二叉搜索树
Posted ZDF0414
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了二叉搜索树相关的知识,希望对你有一定的参考价值。
二叉搜索树的性质:(1)每个节点都有一个作为搜索依据的关键码(key),所有节点的关键码互不相同。
(2)左子树上所有节点的关键码(key)都小于根节点的关键码(key)。
(3)右子树上所有节点的关键码(key)都大于根节点的关键码(key)。
(4)左右子树都是二叉搜索树。
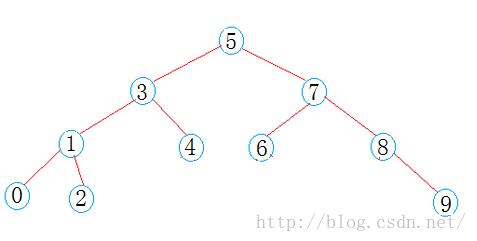
缺陷:在特殊情况下,会退化成单链表的形式,若还以搜索树来看待问题,效率就会很低.
如:在二叉搜索树中插入0、1、2、3、4
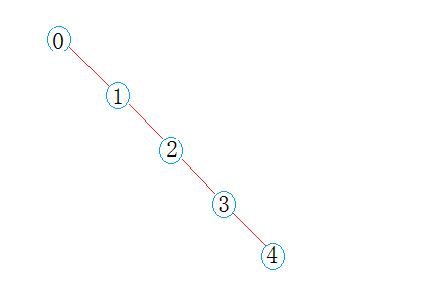
#pragma once
#include<iostream>
template<class K,class V>
struct TreeNode
TreeNode<K, V>* _left;
TreeNode<K, V>* _right;
K _key;
V _value;
TreeNode<K, V>(const K&key, const V&value)
: _left(NULL)
, _right(NULL)
, _key(key)
, _value(value)
;
template<class K,class V>
class BSTree
typedef TreeNode<K, V> Node;
public:
BSTree()
:_root(NULL)
bool Insert(const K&key, const V&value)
if (_root == NULL)
_root = new Node(key, value);
return true;
Node*parent = NULL;
Node*cur = _root;
while (cur)
if (cur->_key < key)
parent = cur;
cur = cur->_right;
else if (cur->_key>key)
parent = cur;
cur = cur->_left;
else
return false;
if (parent->_key < key)
parent->_right = new Node(key, value);
else
parent->_left = new Node(key, value);
bool InsertR(const K&key, const V&value)
return _InsertR(_root, key, value);
Node* Find(const K&key)
if (_root == NULL)
return NULL;
Node* ret = _root;
while (ret)
if (ret->_key > key)
ret = ret->_left;
else if (ret->_key < key)
ret = ret->_right;
else
return ret;
return NULL;
void Remove(K key)
if (_root == NULL)
return ;
Node*parent = NULL;
Node* del = _root;
//1、找到要删除结点的位置
while (del)
if (del->_key < key)
parent = del;
del = del->_right;
else if (del->_key>key)
parent = del;
del = del->_left;
else
break;
if (del)
//2、要删除的结点的左结点为空
if (del->_left == NULL)
if (del == _root)
_root = del->_right;
else if (del == parent->_left)
parent->_left = del->_right;
else
parent->_right = del->_right;
delete del;
del = NULL;
//3、要删除的结点的右结点为空
else if (del->_right == NULL)
if (del == _root)
_root = del->_left;
else if (del == parent->_left)
parent->_left = del->_left;
else
parent->_right = del->_left;
delete del;
//4、要删除的结点的左右都不为空
else
Node* curR = del->_right;
Node*parent = del;
while (curR->_left)
parent = curR;
curR = curR->_left;//找到删除节点右子树中最左边的结点
swap(del->_key, curR->_key);
swap(del->_value, curR->_value);
if (parent->_left == curR)
parent->_left = curR->_right;
else
parent->_right = curR->_right;
delete curR;
curR = NULL;
void RemoveR(K key)
_RemoveR(_root, key);
//cout << endl;
void InSort()
_InSort(_root);
cout << endl;
protected:
bool _InsertR(Node* &root,const K&key, const V&value)
if (root == NULL)
root = new Node(key, value);
return true;
if (root->_key < key)
_InsertR(root->_right, key, value);
else if (root->_key>key)
_InsertR(root->_left, key, value);
return false;
void _InSort(Node*root)
if (root == NULL)
return;
_InSort(root->_left);
cout << root->_key << " ";
_InSort(root->_right);
bool _RemoveR(Node* &root, K key)
if (root == NULL)
return false;
if (root->_key < key)
_RemoveR(root->_right, key);
else if (root->_key > key)
_RemoveR(root->_left, key);
else
Node*del = root;
if (root->_left == NULL)
root = root->_right;
else if (root->_right == NULL)
root = root->_left;
else
Node*curR = root->_right;
Node*curRL = curR->_left;
Node*parent = root;
while (curRL)
parent = curRL;
curRL = curRL->_left;
swap(root->_key, curRL->_key);
if (parent->_left == curRL)
parent->_left = curRL->_right;
else
parent->_right = curRL->_left;
del = curRL;
delete del;
del = NULL;
private:
Node* _root;
;
要解决此问题,即引入了平衡二叉树(AVL树),即根据结点的平衡因子对树进行调节。
以上是关于二叉搜索树的主要内容,如果未能解决你的问题,请参考以下文章