Java:LeetCode题目1~5试做
Posted fxalll
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了Java:LeetCode题目1~5试做相关的知识,希望对你有一定的参考价值。
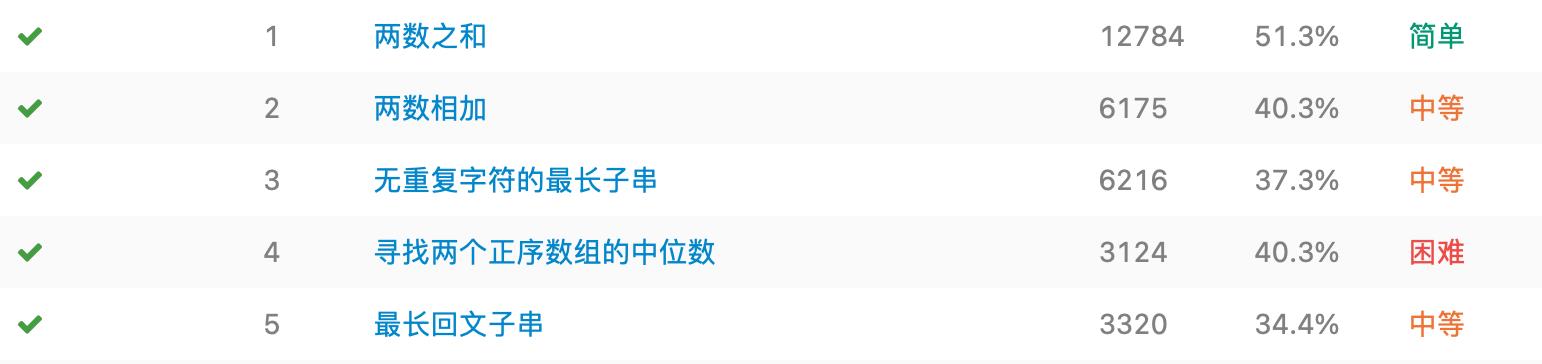
两数之和
给定一个整数数组 nums 和一个整数目标值 target,请你在该数组中找出 和为目标值 target 的那 两个 整数,并返回它们的数组下标。
你可以假设每种输入只会对应一个答案。但是,数组中同一个元素在答案里不能重复出现。
你可以按任意顺序返回答案。
package LeetCode;
import java.util.HashMap;
import java.util.Map;
public class S1 {
public static int[] twoNum(int[] nums,int target){
Map<Integer,Integer> map = new HashMap<>();
map.put(nums[0],0);
for (int i = 1;i < nums.length;i++){
int matchNum = target - nums[i];
if (map.containsKey(matchNum)){
int index1 = i;
int index2 = map.get(matchNum);
return new int[] {index1,index2};
}else {
map.put(nums[i],i);
}
}
return null;
}
}
两数相加
给你两个 非空 的链表,表示两个非负的整数。它们每位数字都是按照 逆序 的方式存储的,并且每个节点只能存储 一位 数字。
请你将两个数相加,并以相同形式返回一个表示和的链表。
你可以假设除了数字 0 之外,这两个数都不会以 0 开头。
(此题有参考)
class ListNode {
int val;
ListNode next;
ListNode() {}
ListNode(int val) { this.val = val; }
ListNode(int val, ListNode next) { this.val = val; this.next = next; }
}
public class S2 {
public static ListNode addTwoNumbers(ListNode l1, ListNode l2) {
ListNode root = new ListNode(0);
ListNode cursor = root;
int carry = 0;
while (l1 != null || l2 != null || carry != 0){
int l1Val = l1 != null ? l1.val : 0;
int l2Val = l2 != null ? l2.val : 0;
int sumVal = l1Val + l2Val + carry;
carry = sumVal / 10;
ListNode sumNode = new ListNode(sumVal % 10);
cursor.next = sumNode;
cursor = sumNode;
if (l1 != null) l1 = l1.next;
if (l2 != null) l2 = l2.next;
}
return root.next;
}
public static void main(String[] args) {
ListNode res = addTwoNumbers(new ListNode(2,new ListNode(4,new ListNode(3))),new ListNode(5,new ListNode(6,new ListNode(4))));
System.out.println(res.val);
System.out.println(res.next.val);
System.out.println(res.next.next.val);
}
}
无重复字符的最长子串
给定一个字符串,请你找出其中不含有重复字符的 最长子串 的长度。
class Solution {
public int lengthOfLongestSubstring(String s) {
String[] res = s.split("");
Set<String> get = new HashSet<>();
Set<Integer> number = new HashSet<>();
number.add(0);
int num = 0;
int i = 0;
int j = 0;
while (i<s.length()) {
if (!get.contains(res[i])){
get.add(res[i]);
num++;
number.add(num);
i++;
}else {
number.add(num);
j++;
i = j;
num = 0;
get = new HashSet<>();
}
}
if (Collections.max(number) > 0){
num = Collections.max(number);
}
System.out.println(num);
return num;
}
}
寻找两个正序数组的中位数
给定两个大小分别为 m 和 n 的正序(从小到大)数组 nums1 和 nums2。请你找出并返回这两个正序数组的 中位数 。
class Solution {
public double findMedianSortedArrays(int[] nums1, int[] nums2) {
int number = nums1.length + nums2.length;
int[] num = new int[number];
System.arraycopy(nums1,0,num,0,nums1.length);
System.arraycopy(nums2,0,num,nums1.length,nums2.length);
Arrays.sort(num);
for (int i = 0; i < num.length; i++) {
System.out.println(num[i]);
}
if (number % 2 == 0){
System.out.println((double) (num[(number/2)-1]+num[number/2])/2);
return (double)(num[(number/2)-1]+num[number/2])/2;
}else {
return num[number/2];
}
}
}
最长回文子串
给你一个字符串 s,找到 s 中最长的回文子串。
package LeetCode;
import com.sun.jdi.Value;
import java.util.*;
public class S5 {
public static String longestPalindrome(String s) {
String[] res = s.split("");
Map<Integer,String> word = new HashMap<>();
int num = 0;
for (int i = 0; i < res.length; i++) {
for (int j = res.length - 1; j >= i; j--) {
if (Objects.equals(res[i], res[j])) {
int k = i + 1;
boolean judge = true;
String trueWord = "";
while (k < j){
if (Objects.equals(res[k], res[j-k+i])){
k++;
}else {
judge = false;
trueWord = "";
break;
}
}
if (judge){
for (int l = i; l <= j; l++) {
trueWord = trueWord + res[l];
}
word.put(num,trueWord);
num++;
}
}
}
}
int max = word.get(0).length();
int maxIn = 0;
for (int i = 1; i < num; i++) {
if (max < word.get(i).length()){
max = word.get(i).length();
maxIn = i;
}
}
System.out.println(word.get(maxIn));
return word.get(maxIn);
}
public static void main(String[] args) {
longestPalindrome("我正在上海在海上吃蜜蜂蜂蜜蛋糕");
}
}
(此题我写的这个代码能做出正确答案,但是耗时稍微长了一点,还可以再改进)
以上是关于Java:LeetCode题目1~5试做的主要内容,如果未能解决你的问题,请参考以下文章
LeetCode 518. 零钱兑换 II c++/java详细题解
LeetCode 61. 旋转链表 c++/java详细题解