若干经典基础算法题目练习
Posted EbowTang
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了若干经典基础算法题目练习相关的知识,希望对你有一定的参考价值。
练习1,判断是否为素数:
// ConsoleAppIsPrime1.cpp : 定义控制台应用程序的入口点。
//
/*
*函数功能:判断一个输入的数是否为素数
*函数原形:bool Prime( int x )
*参数:int x:将要判断的数
*返回值:bool型变量,判断是否是素数
*备注:需要包含头文件<math.h>
*日期:2014/11/25
*原创:否
*作者:EbowTang
*Email:tangyibiao520@163.com
*/
#include "stdafx.h"
#include "math.h"
#include "iostream"
using namespace std;
bool Prime( int x );
int _tmain(int argc, _TCHAR* argv[])
{
bool flag;
int a;
while (true)
{
cout<<"Please enter a number:";
cin>>a;
flag=Prime(a);
if (flag==true)
cout<<"Prime!!!"<<endl;
else
cout<<"Not Prime!";
}
system("pasue");
return 0;
}
/*原理:将输入的x与2到sqrt(x)整除一遍,若其中任意一个能整除则x不是素数*/
bool Prime( int x )
{
x=abs( x );
if ( x < 1 )
return false;
for (int i = 2; i <= int( sqrt( float( x ))) ; i++ )
{
if ( x % i==0 )//一旦可以整除立马返回他不是素数
return false;
}
return true;
}
练习2,指定范围内的素数:
// ConsoleAppIsPrime.cpp : 定义控制台应用程序的入口点。
//
/*
*函数功能:判断指定范围内素数个数
*函数原形:int Primes( int n ,int m )
*参数:
int n:请输入想要确认的素数范围下限
int m:请输入想要确认的素数范围上限
*返回值:n到m范围内素数的个数
*备注:
*日期:2014/11/25
*原创:是
*作者:EbowTang
*Email:tangyibiao520@163.com
*/
#include "stdafx.h"
#include "iostream"
#include "math.h"
using namespace std;
int Primes( int n ,int m );
bool Prime( int x );
int _tmain(int argc, _TCHAR* argv[])
{
int countprimes=0;
int a=0;
int b=0;
while (true)
{
cout<<"请输入想要确认的素数范围上限:";
cin>> a ;
cout<<"请输入想要确认的素数范围下限:";
cin>> b;
countprimes=Primes(a,b);
cout<<"在 "<< a <<"到 "<< b <<"之间的素数个数为: "<< countprimes<<endl;
}
system("pause");
return 0;
}
bool Prime( int x )//判断素数
{
x=abs( x );
if ( x < 1 )
return false;
for (int i = 2; i <= int( sqrt( float( x ))) ; i++ )
if ( x % i==0 )
return false;
return true;
}
int Primes( int n ,int m )//统计素数
{
int count=0;
bool flag=false;
for (; n <= m ; n++)
{
flag=Prime( n );
if (flag==true)
count++;
}
return count;
}
练习3,某整数是否为2的次幂:
/*
*函数功能:判断一个整数是否为2的次幂
*函数原形:bool IsTwoN(int n);
*参数:int n,要判断的整数
*返回值:bool型变量,表征是与否
*时间复杂度:O(1)
*备注:无
*日期:2014/11/23
*原创:否
*作者:EbowTang
*Email:tangyibiao520@163.com
*/
#include "stdafx.h"
#include "iostream"
#include "math.h"
using namespace std;
bool IsTwoN(int n);
int _tmain(int argc, _TCHAR* argv[])
{
while(1)
{
bool flag;
int m;
cout<<"请输入一个整数:"<<endl;
cin>>m;
flag=IsTwoN(m);
if (flag==true)
cout<<m<<"是2的次幂,恭喜你!"<<endl<<endl;
else
cout<<m<<"不是2的次幂"<<endl<<endl;
}
return 0;
}
bool IsTwoN(int n)
{
//若是,必定与其自身减1后的数相“与”为假,比如8,1000&0111=0000,
//if(n>0&&((n&(n-1))==0))/*第一种方法*/
//如果n==2的log以2为底数的n对数的pow函数后,则是
if (n==pow(2,(int)(log10(n)/log10(2.))))/*第二种方法*/
return true;
else
return false;
}
练习4,整数的二进制数中1的个数:
/*
*函数功能:求取整数对应二进制数中1的个数
*函数原形:int Count(int z);
*参数:int z,要计算的整数
*返回值:返回整数对应二进制数中1的个数
*时间复杂度:O(1)
*备注:无
*日期:2014/11/23
*原创:否
*作者:EbowTang
*Email:tangyibiao520@163.com
*/
#include "stdafx.h"
#include "iostream"
using namespace std;
int Count(int z);
int main()
{
int number;
int z;
char asn='n';
do
{
cout<<"请输入一个整数:"<<endl;
cin>>z;
number=Count(z);
cout<<"该整数对应二进制数1的个数为:"<<endl;
cout<<number<<endl;
cout<<"是否继续?(y/n)";
cin>>asn;
} while (asn=='y');
return 0;
}
int Count(int v)
{
int num=0;
while (v)
{
//第一种算法:复杂度较低
// num+=v & 0x01;
// v >>=1;
//第二种算法:
// v&=(v-1);
// num++;
/*第三种算法:算法复杂度较高*/
if(v%2==1)
num++;
v=v/2;
}
return num;
}
练习5,统计指定数据出现次数:
// ConsoleAppDigitCount.cpp : 定义控制台应用程序的入口点。
//
/*
*问题描述:用随机函数生成100个在100到999之间的整数,设计程序统计这些三位数中十位是分别是0-9出现次数
*问题示例:无
*函数功能:统计三位数中十位数0-9出现的次数
*函数原形:void DigitCount(int num[], int count[])
*参数:int num[], 欲统计的数组,int count[]存储统计结果
*返回值: 无
*时间复杂度:O(n)
*备注:无
*日期:2014/12/31
*算法描述:
*/
#include "stdafx.h"
#include "iostream"
#include <stdlib.h>
#include <time.h>
#include <string.h>
using namespace std;
#define MAX 100
using namespace std;
/*
*函数功能:生成100到999的随机整数数
*函数原形:void RandNumIn(int num[])
*参数:int num[],用于存储随机整数
*返回值:无
*/
void RandNumIn(int num[])
{
srand((unsigned)time(NULL)); //设置随机种子
for( int i=0; i<MAX; i++)
num[i]=100+rand()%900; //生成100到999的随机整数数
}
/*
*函数功能:输出生成的100个随机整数
*函数原形:void output(int num[])
*参数:int num[],即将输出的整数
*返回值:无
*/
void RandNumOut(int num[])
{
int count=0;
for( int i=0; i<MAX; i++)
{
cout<<num[i]<<" ";
count++;
if(0==count%10)//每个10个数字就换行
cout<<endl;
}
cout<<endl;
}
/*
*函数功能:统计三位数中十位数0-9出现的次数
*函数原形:void DigitCount(int num[], int count[])
*参数:int num[], 欲统计的数组,int count[]存储统计结果
*返回值: 无
*/
void DigitCount(int num[], int count[])
{
int mod;
for(int i=0; i<MAX; i++)
{
mod=num[i]/10%10; //得到十位数字
count[mod]++;
}
}
int _tmain(int argc, _TCHAR* argv[])
{
int num[MAX], count[10];
memset(count, 0, 10*sizeof(int)); /* 初始化统计数组 */
memset(num, 0, 100*sizeof(int)); /* 初始化随机数组 */
cout<<"100个随机整数如下:"<<endl;
RandNumIn(num);//生成100个随机数
RandNumOut(num);//输出100个随机数
DigitCount(num, count);//统计十位数的出现个数
for(int i=0; i<10; i++)
cout<<"十位数"<<i<<":"<<count[i]<<endl;
cout<<endl;
cout<<"其中十位数为0的数为:"<<endl;
for (int i=0;i<MAX;i++)
{
if (num[i]/10%10==0)
{
cout<<num[i]<<" ";
}
}
cout<<endl;
system("pause");
return 0;
}
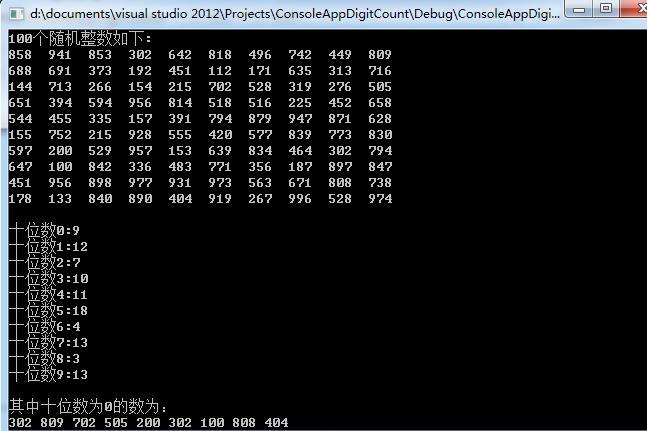
练习6,进制的转换:
// ConsoleAppHexadecimalTrans.cpp : 定义控制台应用程序的入口点。
//
/*
*问题描述:将十进制转换成二进制或者八进制,16进制
*问题示例:输入50 2,输出110010
*函数功能:
*函数原形:void HexadecimalTrans(int n, int d)
*参数:int n,十进制整数 int d,进制数
*返回值:无
*时间复杂度:
*备注:无
*日期:2014/11/30
*算法描述:
*/
#include "stdafx.h"
#include <iostream>
using namespace std;
int flag=1;
void HexadecimalTrans(int n, int d)
{
int mod;
mod=n%d; //n表示数字,d表示进制
n=n/d;
while(flag && n) //辗转相除
HexadecimalTrans(n,d);
switch(mod)
{
case 10:
cout<<"A";
break;
case 11:
cout<<"B";
break;
case 12:
cout<<"C";
break;
case 13:
cout<<"D";
break;
case 14:
cout<<"E";
break;
case 15:
cout<<"F";
break;
default:
cout<<mod; //二进制和八进制均在这里输出,mod保存了辗转相除的每次结果
}
flag=0;
}
int _tmain(int argc, _TCHAR* argv[])
{
int n, d;
cout<<"输入十进制数字:"<<endl;
cin>>n;
cout<<"请输入将要进行的进制转换(2,8,16):"<<endl;
cin>>d;
HexadecimalTrans(n,d); //调用转换函数
cout<<endl;
system("pause");
return 0;
}
练习7,查找指定数据的位置(暴力搜索):
// ConsoleAppSpecifiedSearch.cpp : 定义控制台应用程序的入口点。
//
/*
*问题描述:暴力搜索遍历查找,从100个随机函数中(100-999)查找指定数字的位置
*问题示例:
*函数功能:查找指定的数
*函数原形:int FindNum(int num[], int x)
*参数:int num[],要查找的数组, int x,要查找的数
*返回值:数字的位置
*时间复杂度:O()
*备注:无
*日期:2014/7/30
*算法描述:
*/
#include "stdafx.h"
#include <iostream>
#include <stdlib.h>
#include <time.h>
using namespace std;
#define MAX 100
/*
*函数功能:产生指定的随机数
*函数原形:void RandNumIn(int num[])
*参数:int num[],产生的随机数保存到数组num中
*返回值:无
*/
void RandNumIn(int num[])
{
int i;
srand((unsigned)time(NULL)); //得到随机种子
for(i=0; i<MAX; i++)
num[i]=100+rand()%900; //生成100--999以内的随机三位整数
}
/*
*函数功能:输出产生的随机数
*函数原形:void RandNumOut(int num[])
*参数:int num[],将要输出的数组
*返回值:无
*/
void RandNumOut(int num[])
{
int count=0;
for(int i=0; i<MAX; i++)
{
cout<<num[i]<<" ";
count++;
if(0==count%10)
cout<<endl;
}
}
/*
*函数功能:查找指定的数
*函数原形:int FindNum(int num[], int x)
*参数:int num[],要查找的数组, int x,要查找的数
*返回值:数字的位置
*/
int FindNum(int num[], int x)
{
for(int i=0; i<MAX; i++)
if(x == num[i]) //遍历查找指定数字的位置
return i;
return 0;
}
int _tmain(int argc, _TCHAR* argv[])
{
int x, pos, num[MAX]; //设置存储数组
RandNumIn(num);
cout<<"随机生成的数字: "<<endl;
RandNumOut(num);
cout<<"请输入要查找的三位整数"<<endl;
cin>>x;
pos=FindNum(num, x); //调用查找函数
if(pos)
cout<<"太好了!"<<x<< "的位置在: "<< pos<<endl;
else
cout<<"抱歉!"<<x<< "并未找到! "<<endl;
system("pause");
return 0;
}
练习8,二分法查找指定数据位置:
// ConsoleAppSpecifiedSearch.cpp : 定义控制台应用程序的入口点。
//
/*
*问题描述:二分法遍历查找,从100个随机函数中(100-999)查找指定数字的位置
*问题示例:
*函数功能:二分查找法查找指定的数
*函数原形:int SpecialFindNum(int num[], int x, int low, int high)
*参数:
int num[],要查找的数组,
int x,要查找的数
int low, 查找的起始位置
int high,查找的末端位置
*返回值:数字的位置
*时间复杂度:O()
*备注:无
*日期:2014/7/30
*算法描述:
*/
#include "stdafx.h"
#include <iostream>
#include <stdlib.h>
#include <time.h>
using namespace std;
#define MAX 101
/*
*函数功能:产生指定的随机数
*函数原形:void RandNumIn(int num[])
*参数:int num[],产生的随机数保存到数组num中
*返回值:无
*/
void RandNumIn(int num[])
{
int i;
srand((unsigned)time(NULL)); //得到随机种子
for(i=1; i<MAX; i++)
num[i]=100+rand()%900; //生成100--999以内的随机三位整数
}
/*
*函数功能:输出产生的随机数
*函数原形:void RandNumOut(int num[])
*参数:int num[],将要输出的数组
*返回值:无
*/
void RandNumOut(int num[])
{
int count=0;
for(int i=1; i<MAX; i++)
{
cout<<num[i]<<" ";
count++;
if(0==count%10)
cout<<endl;
}
}
/*
*函数功能:快速排序法
*函数原形:void QuickSort(int num[], int low, int high)
*参数:
int num[],要排序的数组,
int low, 查找的起始位置
int high,查找的末端位置
*返回值:无
*/
void QuickSort(int num[], int low, int high)
{
int l, h;
if(low<high)
{
l=low;
h=high;
num[0]=num[l];
while(l<h)
{
while(l<h && num[h]>=num[0])
h--; //利用快速排序是数据有序
num[l]=num[h];
while(l<h && num[l]<=num[0])
l++;
num[h]=num[l];
}
num[l]=num[0];
QuickSort(num, low, l-1);
QuickSort(num, l+1, high);
}
}
/*
*函数功能:二分查找法查找指定的数
*函数原形:int SpecialFindNum(int num[], int x, int low, int high)
*参数:
int num[],要查找的数组,
int x,要查找的数
int low, 查找的起始位置
int high,查找的末端位置
*返回值:数字的位置
*/
int SpecialFindNum(int num[], int x, int low, int high)
{
int mid; //中间位置
while(low<=high)
{
mid=(low+high)/2; /* 找到 */
if(x==num[mid])
return mid;
else if(x<num[mid]) //两边的游标不停往中间移动比较
high=mid-1;
else
low=mid+1;
}
return 0;
}
int _tmain(int argc, _TCHAR* argv[])
{
int x, pos, num[MAX];
RandNumIn(num);
cout<<"排序前:"<<endl;
RandNumOut(num);
QuickSort(num, 1, MAX-1);
cout<<"排序后:"<<endl;
RandNumOut(num);
cout<<"请输入要查找的数:"<<endl;
cin>>x;
pos=SpecialFindNum(num, x, 1, MAX-1); //调用查找函数
if(pos)
cout<<"太好了!"<<x<< "的位置在: "<< pos<<endl;
else
cout<<"抱歉"<<x<< "没有找到 "<<endl;
system("pause");
return 0;
}
练习9,字符串中指定数据出现次数
// ConsoleAppCharCount.cpp : 定义控制台应用程序的入口点。
//
/*
*问题描述:设计一个程序,统计随机给出的字符串的数字的个数,字母的个数,特殊字符的个数
*问题示例:123asd,数字3,字母3,特殊字符0
*函数功能:统计字符串中的数据
*函数原形:void CharCal(char *str, int count[])
*参数:char *str,欲统计的字符串, int count[],用于存储统计结果
*返回值:无
*时间复杂度:O()
*备注:无
*日期:2014/7/30
*算法描述:
*/
#include "stdafx.h"
#include <iostream>
#include <string.h>
#define MAX 1024
using namespace std;
/*
*函数功能:统计字符串中的数据
*函数原形:void CharCal(char *str, int count[])
*参数:char *str,欲统计的字符串, int count[],用于存储统计结果
*返回值:无
*/
void CharCal(char *str, int count[])
{
while(*str)
{
if((*str>='0')&&(*str<='9')) //统计数字型字符
count[0]++;
else if(((*str>='a')&&(*str<='z')) || ((*str>='A')&&(*str<='Z'))) //统计字符型字符
count[1]++;
else //其他特殊字符
count[2]++;
str++;//指针移动
}
}
/*
*函数功能:输出统计结果
*函数原形:void CharCount( int count[])
*参数: int count[],存储统计结果的数组
*返回值:无
*/
void CharCount( int count[])
{
for(int i=0; i<3; i++)
{
switch(i)
{
case 0:
cout<<"数字:"<<count[i]<<endl;
break;
case 1:
cout<<"字符:"<< count[i]<<endl;
break;
case 2:
cout<<"特殊字符:"<<count[i]<<endl;
break;
}
}
}
int _tmain(int argc, _TCHAR* argv[])
{
char str[MAX]; //定义存储输入字符串的数组
int count[3]; //用于存储统计结果0->数字; 1->字符; 2->其他
memset(count, 0, 3*sizeof(int)); //初始化统计数组
cout<<"输入字符串: "<<endl;
cin>>str;
CharCal(str, count);//统计
CharCount(count);//输出显示
system("pause");
return 0;
}
练习10,逆序输出字符串
// ConsoleAppCharTest.cpp : 定义控制台应用程序的入口点。
//
/*
*问题描述:输入字符串,将其逆序输出
*问题示例:输入,asdfgh,输出hgfdsa
*函数功能:逆序输出字符串的内容
*函数原形:void Reverse( char* s, int left, int right );
*参数:
char *,欲逆序的字符串,
int left, 逆序的左起点
int right,逆序的右尾点
*返回值:无
*时间复杂度:O(n)
*备注:无
*日期:2014/7/30
*算法描述:
*/
#include "stdafx.h"
#include<iostream>
using namespace std;
void Reverse( char* s, int left, int right );
int _tmain(int argc, _TCHAR* argv[])
{
int len;
char a[100];
while(1)
{
cout<<"请输入字符串a:"<<endl;
cin>>a;
len=strlen(a);
Reverse(a,0,len-1);
for(int i=0;i<len;i++)
cout<<a[i];
cout<<endl;
}
return 0;
}
//算法一: 对字符串s在指定区间left和right之间进行逆序,递归法
void Reverse( char* s, int left, int right )
{
if(left >= right)
return;
char t = s[left] ;
s[left] = s[right] ;
s[right] = t ;
Reverse(s, left + 1, right - 1) ;
}
/*
//算法二:与上面的方法没有太大区别
void Reverse( char* s, int left, int right )
{
while( left < right )
{
char t = s[left] ;
s[left++] = s[right] ;
s[right--] = t ;
}
}*/
练习11,字符串包含判断
// ConsoleAppCharTest.cpp : 定义控制台应用程序的入口点。
//
/*
*问题描述:输入两个字符串,判断相互之间字符串是否包含
*问题示例:输入,asdfgh,输出asd,结果,包含!
*函数功能:判断两个字符串是否包含
*函数原形:bool IsContain(char *pStrA, char *pStrB);
*参数:
char *pStrA, 第一个字符串
char *pStrB,第二个字符串
*返回值:布尔变量
*时间复杂度:O(n)
*备注:无
*日期:2014/7/30
*算法描述:
*/
#include "stdafx.h"
#include "iostream"
using namespace std;
bool IsContain(char *pStrA, char *pStrB);
int _tmain(int argc, _TCHAR* argv[])
{
char a[10],b[10];
bool flag;
while(true)
{
cout<<"Please Enter characters A:"<<endl;
cin>>a;
cout<<"Please Enter characters B:"<<endl;
cin>>b;
flag=IsContain(a, b);
if (flag==false)
cout<<"Not Contain!"<<endl;
else
cout<<"Contain!"<<endl;
}
return 0;
}
//算法1
bool IsContain(char *pStrA, char *pStrB)
{
int lenA = strlen(pStrA);
int lenB = strlen(pStrB);
int i,j;
char temp;
for( i = 0; i < lenA; i++)
{//直接对字符数组A进行循环移位再立马进行字符包含判断
temp = pStrA[0];
for( j = 0; j < lenA - 1; j++)
pStrA[ lenA - 1] = pStrA[j + 1];
pStrA[j] = temp;
if(strncmp(pStrA, pStrB, lenB) == 0)
return true;
}
//若果执行到最后都还没有匹配成功,则返回false
if(i == lenA)
return false;
}/*
//算法2
bool IsContain(char *pStrA, char *pStrB)
{
int lenp = strlen(pStrA);
char *temp = (char*)malloc(2 * lenp + 1);//动态分配内存
strcpy_s(temp,2 * lenp + 1,pStrA);
strcpy_s(temp + lenp, 2 * lenp + 1,pStrA);
if(strstr(temp, pStrB))
return TRUE;
else
return FALSE;
}*/
以上是关于若干经典基础算法题目练习的主要内容,如果未能解决你的问题,请参考以下文章