第二周:神经网络的编程基础----------3Python Basics with numpy (optional)
Posted
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了第二周:神经网络的编程基础----------3Python Basics with numpy (optional)相关的知识,希望对你有一定的参考价值。
Python Basics with numpy (optional)
Welcome to your first (Optional) programming exercise of the deep learning specialization. In this assignment you will:
- Learn how to use numpy.
- Implement some basic core deep learning functions such as the softmax, sigmoid, dsigmoid, etc...
- Learn how to handle data by normalizing inputs and reshaping images.
- Recognize the importance of vectorization.
- Understand how python broadcasting works.
This assignment prepares you well for the upcoming assignment. Take your time to complete it and make sure you get the expected outputs when working through the different exercises. In some code blocks, you will find a "#GRADED FUNCTION: functionName" comment. Please do not modify it. After you are done, submit your work and check your results. You need to score 70% to pass. Good luck :) !
中文翻译------------>
Welcome to your first assignment. This exercise gives you a brief introduction to Python. Even if you‘ve used Python before, this will help familiarize you with functions we‘ll need.
Instructions:
You will be using Python 3.
Avoid using for-loops and while-loops, unless you are explicitly told to do so.
Do not modify the (# GRADED FUNCTION [function name]) comment in some cells. Your work would not be graded if you change this. Each cell containing that comment should only contain one function.
After coding your function, run the cell right below it to check if your result is correct.
After this assignment you will:
Be able to use iPython Notebooks
Be able to use numpy functions and numpy matrix/vector operations
Understand the concept of "broadcasting"
Be able to vectorize code
Let‘s get started!
中文翻译------------>
--------------------------------------------------------------------------------------------------------------------------------------
About iPython Notebooks
iPython Notebooks are interactive coding environments embedded in a webpage. You will be using iPython notebooks in this class. You only need to write code between the ### START CODE HERE ### and ### END CODE HERE ### comments. After writing your code, you can run the cell by either pressing "SHIFT"+"ENTER" or by clicking on "Run Cell" (denoted by a play symbol) in the upper bar of the notebook.
We will often specify "(≈ X lines of code)" in the comments to tell you about how much code you need to write. It is just a rough estimate, so don‘t feel bad if your code is longer or shorter.
中文翻译------------>
### START CODE HERE ### (≈ 1 line of code) test = "Hello World" ### END CODE HERE ###
print ("test: " + test)
What you need to remember:
Run your cells using SHIFT+ENTER (or "Run cell")
Write code in the designated areas using Python 3 only
Do not modify the code outside of the designated areas
中文翻译------------>
--------------------------------------------------------------------------------------------------------------------------------------
1 - Building basic functions with numpy
Numpy is the main package for scientific computing in Python. It is maintained by a large community (www.numpy.org). In this exercise you will learn several key numpy functions such as np.exp, np.log, and np.reshape. You will need to know how to use these functions for future assignments.
1.1 - sigmoid function, np.exp()
Before using np.exp(), you will use math.exp() to implement the sigmoid function. You will then see why np.exp() is preferable to math.exp().
Exercise: Build a function that returns the sigmoid of a real number x. Use math.exp(x) for the exponential function.
Reminder: sigmoid(x)=is sometimes also known as the logistic function. It is a non-linear function used not only in Machine Learning (Logistic Regression), but also in Deep Learning.
To refer to a function belonging to a specific package you could call it using package_name.function(). Run the code below to see an example with math.exp().
中文翻译------------>
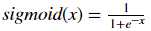
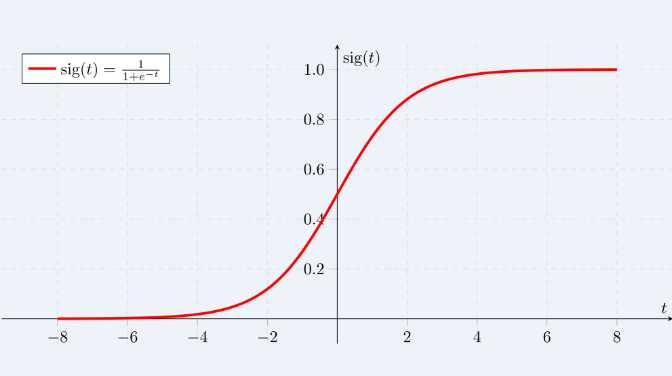
要引用属于特定包的函数, 可以使用 package_name.function()来调用它。运行下面的代码以查看math. exp () 的示例。
# GRADED FUNCTION: basic_sigmoid import math def basic_sigmoid(x): """ Compute sigmoid of x. Arguments: x -- A scalar Return: s -- sigmoid(x) """ ### START CODE HERE ### (≈ 1 line of code) s = 1/(1+math.exp(-x)) ### END CODE HERE ### return s
basic_sigmoid(3)
Expected Output:
basic_sigmoid(3) | 0.9525741268224334 |
### One reason why we use "numpy" instead of "math" in Deep Learning ### x = [1, 2, 3] basic_sigmoid(x) # you will see this give an error when you run it, because x is a vector.
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-6-2e11097d6860> in <module>()
1 ### One reason why we use "numpy" instead of "math" in Deep Learning ###
2 x = [1, 2, 3]
----> 3 basic_sigmoid(x) # you will see this give an error when you run it, because x is a vector.
<ipython-input-4-951c5721dbfa> in basic_sigmoid(x)
15
16 ### START CODE HERE ### (≈ 1 line of code)
---> 17 s = 1/(1+math.exp(-x))
18 ### END CODE HERE ###
19
TypeError: bad operand type for unary -: ‘list‘
In fact, if x=(x1,x2,...,xn) is a row vector then np.exp(x)will apply the exponential function to every element of x. The output will thus be: np.exp(x)=(ex1,ex2,...,exn)
import numpy as np # example of np.exp x = np.array([1, 2, 3]) print(np.exp(x)) # result is (exp(1), exp(2), exp(3))
result:
[ 2.71828183 7.3890561 20.08553692]
# example of vector operation x = np.array([1, 2, 3]) print (x + 3)
result:
[4 5 6]
--------------------------------------------------------------------------------------------------------------------------------------
Any time you need more info on a numpy function, we encourage you to look at the official documentation.
You can also create a new cell in the notebook and write np.exp? (for example) to get quick access to the documentation.
Instructions: x could now be either a real number, a vector, or a matrix. The data structures we use in numpy to represent these shapes (vectors, matrices...) are called numpy arrays. You don‘t need to know more for now.


# GRADED FUNCTION: sigmoid import numpy as np # this means you can access numpy functions by writing np.function() instead of numpy.function() def sigmoid(x): """ Compute the sigmoid of x Arguments: x -- A scalar or numpy array of any size Return: s -- sigmoid(x) """ ### START CODE HERE ### (≈ 1 line of code) s = 1/(1+np.exp(-x)) ### END CODE HERE ### return s
x = np.array([1, 2, 3])
# x=[1,2,3] #这种写法会报错
sigmoid(x)
array([ 0.73105858, 0.88079708, 0.95257413])
Expected Output:
sigmoid([1,2,3]) | array([ 0.73105858, 0.88079708, 0.95257413]) |