Eclipse+Java+Swing+Mysql实现超市管理系统
Posted 水坚石青
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了Eclipse+Java+Swing+Mysql实现超市管理系统相关的知识,希望对你有一定的参考价值。
目录
一、系统介绍
1.开发环境
开发工具:Eclipse2021
JDK版本:jdk1.8
mysql版本:8.0.13
2.技术选型
Java+Swing+Mysql
3.系统功能
1.登录系统
2.查询商品
3.添加商品
4.修改商品
5.删除商品
二、系统展示
1.登录系统
2.系统主页
3.查询商品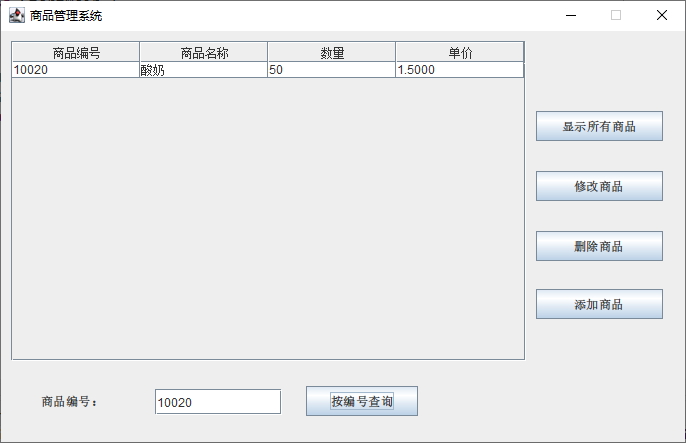
4.修改商品
5.添加商品
三、部分代码
GoodsXG.java
package com.ynavc.Vive;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JTextField;
import com.ynavc.Bean.Goods;
import com.ynavc.Controller.Updata;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
public class GoodsXG extends JFrame {
private JTextField id, name, num, price;
private JButton button;
private JButton button_1;
int goodsid;
public GoodsXG(Goods goods) {
super("商品管理系统");
this.setBounds(0, 0, 400, 450);
this.setLocationRelativeTo(null);// 让窗口在屏幕中间显示
this.setResizable(false);// 让窗口大小不可改变
getContentPane().setLayout(null);
JLabel label = new JLabel("商品编号:");
label.setBounds(85, 89, 87, 22);
getContentPane().add(label);
id = new JTextField();
id.setBounds(147, 90, 142, 21);
getContentPane().add(id);
id.setColumns(10);
JLabel label_1 = new JLabel("商品名称");
label_1.setBounds(85, 139, 87, 22);
getContentPane().add(label_1);
name = new JTextField();
name.setColumns(10);
name.setBounds(147, 140, 142, 21);
getContentPane().add(name);
JLabel label_2 = new JLabel("数量:");
label_2.setBounds(85, 193, 87, 22);
getContentPane().add(label_2);
num = new JTextField();
num.setColumns(10);
num.setBounds(147, 194, 142, 21);
getContentPane().add(num);
JLabel label_3 = new JLabel("单价:");
label_3.setBounds(85, 241, 87, 22);
getContentPane().add(label_3);
price = new JTextField();
price.setColumns(10);
price.setBounds(147, 242, 142, 21);
getContentPane().add(price);
goodsid = goods.getGoodsID();
id.setText(Integer.toString(goods.getGoodsID()));
name.setText(goods.getGoodsName());
num.setText(Integer.toString(goods.getNum()));
price.setText(goods.getPrice());
button = new JButton("确定");
button.setBounds(78, 317, 93, 23);
getContentPane().add(button);
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
String addId = id.getText();
String addName = name.getText();
String addNum = num.getText();
String addPrice = price.getText();
if (addName.equals("") || addName.equals("") || addNum.equals("") || addPrice.equals("")) {
JOptionPane.showMessageDialog(null, "请完整输入要修改的数据");
} else {
String sql = "UPDATE goods SET " + "Goodsid='" + addId + "',Goodsname='" + addName + "',num='"
+ addNum + "',price='" + addPrice + "'where goodsid=" + goodsid;
int result = Updata.addData(sql);
if (result > 0) {
JOptionPane.showMessageDialog(null, "修改成功!");
JOptionPane.showMessageDialog(null, "记得刷新一下哦!");
dispose();
// GoodsManage i = new GoodsManage();
// i.setVisible(true);
} else {
JOptionPane.showMessageDialog(null, "修改失败!");
}
}
}
});
button_1 = new JButton("取消");
button_1.setBounds(208, 317, 93, 23);
getContentPane().add(button_1);
button_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
dispose();
}
});
}
public static void main(String[] args) {
GoodsXG g = new GoodsXG(null);
g.setVisible(true);
}
}
GoodsManagement.java
package com.ynavc.Vive;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.ScrollPaneConstants;
import javax.swing.table.DefaultTableModel;
import com.ynavc.Bean.Goods;
import com.ynavc.Controller.Select;
import com.ynavc.Controller.Updata;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.awt.event.ActionEvent;
public class GoodsManage extends JFrame {
private JTextField textField;
Select select = new Select();
Updata updata = new Updata();
Object[] header = { "商品编号", "商品名称", "数量", "单价" };
String sql = "SELECT goodsID,goodsname,num,price FROM goods";
Object[][] data = select.getGoods(sql);
DefaultTableModel df = new DefaultTableModel(data, header);
int v = ScrollPaneConstants.VERTICAL_SCROLLBAR_AS_NEEDED;
int h = ScrollPaneConstants.HORIZONTAL_SCROLLBAR_AS_NEEDED;
public GoodsManage() {
super("商品管理系统");
this.setBounds(0, 0, 700, 450);
this.setLocationRelativeTo(null);// 让窗口在屏幕中间显示
this.setResizable(false);// 让窗口大小不可改变
getContentPane().setLayout(null);
JTable jTable = new JTable(df);
JScrollPane jsp = new JScrollPane(jTable, v, h);
jsp.setBounds(10, 10, 515, 320);
getContentPane().add(jsp);
JButton button_1 = new JButton("显示所有商品");
button_1.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String sql = "SELECT goodsID,goodsname,num,price FROM goods";
Object[][] data = Select.getGoods(sql);
df.setDataVector(data, header);
}
});
button_1.setBounds(535, 80, 127, 30);
getContentPane().add(button_1);
JButton button_2 = new JButton("修改商品");
button_2.setBounds(535, 140, 127, 30);
getContentPane().add(button_2);
button_2.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
if (jTable.getSelectedColumn() < 0) {
JOptionPane.showMessageDialog(null, "请选择要修改的数据!");
} else {
int goodsID = Integer.parseInt(jTable.getValueAt(jTable.getSelectedRow(), 0).toString());
String name = jTable.getValueAt(jTable.getSelectedRow(), 1).toString();
int num = Integer.parseInt(jTable.getValueAt(jTable.getSelectedRow(), 2).toString());
String price = jTable.getValueAt(jTable.getSelectedRow(), 3).toString();
Goods goods = new Goods(goodsID, name, num, price);
GoodsXG goodsXG = new GoodsXG(goods);
goodsXG.setVisible(true);
}
}
});
JButton button_3 = new JButton("删除商品");
button_3.setBounds(535, 200, 127, 30);
getContentPane().add(button_3);
button_3.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
if (jTable.getSelectedColumn() < 0) {
JOptionPane.showMessageDialog(null, "请选中要删除的数据!");
} else {
int goodsID = Integer.parseInt(jTable.getValueAt(jTable.getSelectedRow(), 0).toString());
String sql = "delete from goods where goodsid=" + goodsID;
int result = updata.addData(sql);
if (result > 0) {
JOptionPane.showMessageDialog(null, "删除成功!");
JOptionPane.showMessageDialog(null, "记得刷新一下哦!");
} else {
JOptionPane.showMessageDialog(null, "删除失败!");
}
}
}
});
JButton button_4 = new JButton("添加商品");
button_4.setBounds(535, 258, 127, 30);
getContentPane().add(button_4);
button_4.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
GoodsADD goodsAdd = new GoodsADD();
goodsAdd.setVisible(true);
}
});
JLabel label = new JLabel("商品编号:");
label.setBounds(40, 354, 112, 32);
getContentPane().add(label);
textField = new JTextField();
textField.setBounds(154, 358, 127, 26);
getContentPane().add(textField);
textField.setColumns(10);
JButton button = new JButton("按编号查询");
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
String sql = "SELECT goodsID,goodsname,num,price FROM goods WHERE goodsid LIKE '%" + textField.getText()
+ "%'";
Object[][] data = Select.getGoods(sql);
df.setDataVector(data, header);
}
});
button.setBounds(305, 355, 112, 30);
getContentPane().add(button);
this.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
super.windowClosing(e);
// 加入动作
GoodsManagement m = new GoodsManagement();
m.setVisible(true);
}
});
}
public static void main(String[] args) {
GoodsManage t = new GoodsManage();
t.setVisible(true);
}
}
LoginFrame.java
package com.ynavc.Vive;
import java.awt.BorderLayout;
import java.awt.Container;
import java.awt.FlowLayout;
import java.awt.Frame;
import java.awt.HeadlessException;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
import com.ynavc.Dao.UserDao;
/**
* 登录框架 2019年6月6日18:04:57
*
* @author Administrator
*
*/
public class LoginFrame extends Frame {
private static final long serialVersionUID = 1L;
private JFrame jFrame = new JFrame("登录");
private Container c = jFrame.getContentPane();
private JLabel a1 = new JLabel("用户名");
private JTextField username = new JTextField("admin");
private JLabel a2 = new JLabel("密 码");
private JPasswordField password = new JPasswordField("admin");
private JButton okbtn = new JButton("登录");
private JButton cancelbtn = new JButton("重置");
public LoginFrame() {
// 设置窗体的位置及大小
jFrame.setBounds(600, 200, 300, 220);
// 设置一层相当于桌布的东西
c.setLayout(new BorderLayout());// 布局管理器
// 设置按下右上角X号后关闭
jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 初始化--往窗体里放其他控件
jFrame.setLocationRelativeTo(null);// 窗
// 标题部分
JPanel titlePanel = new JPanel();
titlePanel.setLayout(new FlowLayout());
titlePanel.add(new JLabel("超市管理系统"));
c.add(titlePanel, "North");
// 输入部分-
JPanel fieldPanel = new JPanel();
fieldPanel.setLayout(null);
a1.setBounds(50, 20, 50, 20);
a2.setBounds(50, 60, 50, 20);
fieldPanel.add(a1);
fieldPanel.add(a2);
username.setBounds(110, 20, 120, 20);
password.setBounds(110, 60, 120, 20);
fieldPanel.add(username);
fieldPanel.add(password);
c.add(fieldPanel, "Center");
// 按钮部分
JPanel buttonPanel = new JPanel();
buttonPanel.setLayout(new FlowLayout());
buttonPanel.add(okbtn);
buttonPanel.add(cancelbtn);
c.add(buttonPanel, "South");
// 设置窗体可见
jFrame.setVisible(true);
// 确认按下去获取
okbtn.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
try {
String uname = username.getText();
String pwd = String.valueOf(password.getPassword());
boolean flag = false;
try {
flag = UserDao.login(uname, pwd);
} catch (Exception e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
if (flag) {
jFrame.setVisible(false);
JOptionPane.showMessageDialog(null, "恭喜您,登录成功!");
GoodsManage g = new GoodsManage();
g.setVisible(true);
// 窗口居中
g.setLocationRelativeTo(null);
} else {
JOptionPane.showMessageDialog(null, "登录失败,账号或密码错误");
}
} catch (HeadlessException e1) {
username.setText("");
password.setText("");
JOptionPane.showMessageDialog(null, "登录失败");
}
}
});
// 取消按下去清空
cancelbtn.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
username.setText("");
password.setText("");
}
});
}
}
四、其他
1.更多系统
更多JavaSwing系统请关注专栏。
https://blog.csdn.net/helongqiang/category_6229101.htmlhttps://blog.csdn.net/helongqiang/category_6229101.html
2.源码下载
3.运行项目
请点击以下链接,部署你的项目。
4.备注
如有侵权请联系我删除。
5.支持博主
如果您觉得此文对您有帮助,请点赞加关注加收藏。祝您生活愉快!想要获取其他资源可关注左侧微信公众号获取!
以上是关于Eclipse+Java+Swing+Mysql实现超市管理系统的主要内容,如果未能解决你的问题,请参考以下文章
Eclipse+Java+Swing+Mysql实现超市管理系统
Eclipse+Java+Swing+Mysql实现酒店管理系统
Eclipse+Java+Swing+Mysql实现学生信息管理系统
Eclipse+Java+Swing+Mysql实现进销存管理系统建议收藏