swing计算器
Posted tookkke
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了swing计算器相关的知识,希望对你有一定的参考价值。
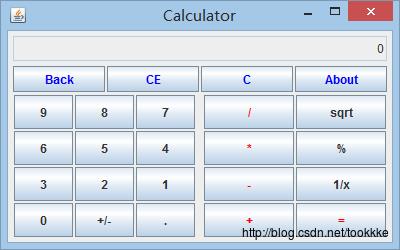
//MyCalculator.java
package test3;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.GridLayout;
import java.awt.KeyEventPostProcessor;
import java.awt.KeyboardFocusManager;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.math.BigDecimal;
import java.math.BigInteger;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.SwingConstants;
import javax.swing.border.EmptyBorder;
public class MyCalculator extends JFrame
JPanel mainPanel=new JPanel();
private JTextField textFiled;
private String a;
private char c='#';
private JButton numButton[]=new JButton[10];
public final static String About="Version : 1.0.0\\n"
+"Arthur : kkke\\n"
+"csdnBlog: http://blog.csdn.net/tookkke/article/\\n";
private void initText()
textFiled.setText("0");
private void initFrame()
setSize(400, 250);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
getContentPane().add(mainPanel,BorderLayout.CENTER);
mainPanel.setBorder(new EmptyBorder(5, 5, 5, 5));
mainPanel.setLayout(new BorderLayout(0, 5));
private class MyActionListener implements ActionListener
private String num;
public MyActionListener(int num)
this.num=Integer.toString(num);
@Override
public void actionPerformed(ActionEvent e)
if(textFiled.getText().equals("0"))textFiled.setText(num);
else textFiled.setText(textFiled.getText()+num);
public MyCalculator()
super("Calculator");
initFrame();
JPanel panel = new JPanel();
mainPanel.add(panel, BorderLayout.NORTH);
panel.setLayout(new BorderLayout(0, 0));
textFiled = new JTextField();
textFiled.setHorizontalAlignment(SwingConstants.RIGHT);
textFiled.setEditable(false);
textFiled.setPreferredSize(new Dimension(0, 25));
panel.add(textFiled, BorderLayout.NORTH);
JPanel PCenter = new JPanel();
mainPanel.add(PCenter, BorderLayout.CENTER);
PCenter.setLayout(new BorderLayout(3, 3));
JPanel PNorth = new JPanel();
PCenter.add(PNorth, BorderLayout.NORTH);
PNorth.setLayout(new GridLayout(1, 4, 2, 0));
JButton btnBack = new JButton("Back");
btnBack.setForeground(Color.blue);
btnBack.addActionListener(new ActionListener()
@Override
public void actionPerformed(ActionEvent e)
String text=textFiled.getText();
if(text.equals("0"))
if(c!='#')
c='#';
textFiled.setText(a);
else
if(text.length()==1)textFiled.setText("0");
else
textFiled.setText(text.substring(0,text.length()-1));
if(textFiled.getText().equals("-"))textFiled.setText("0");
);
PNorth.add(btnBack);
JButton btnCE = new JButton("CE");
btnCE.setForeground(Color.blue);
btnCE.addActionListener(new ActionListener()
@Override
public void actionPerformed(ActionEvent e)
c='#';
textFiled.setText("0");
);
PNorth.add(btnCE);
JButton btnC = new JButton("C");
btnC.setForeground(Color.blue);
btnC.addActionListener(new ActionListener()
@Override
public void actionPerformed(ActionEvent e)
textFiled.setText("0");
);
PNorth.add(btnC);
JButton btnAbout = new JButton("About");
btnAbout.addActionListener(new ActionListener()
@Override
public void actionPerformed(ActionEvent e)
JOptionPane.showMessageDialog(MyCalculator.this, About, "About", JOptionPane.INFORMATION_MESSAGE);
);
btnAbout.setForeground(Color.blue);
JPanel PWest = new JPanel();
PWest.setLayout(new GridLayout(4, 3, 2, 2));
PNorth.add(btnAbout);
for(int i=9;i>=0;i--)
numButton[i]=new JButton(Integer.toString(i));
numButton[i].addActionListener(new MyActionListener(i));
PWest.add(numButton[i]);
JPanel PEX = new JPanel();
PEX.setLayout(new GridLayout(1, 2, 8, 0));
PCenter.add(PEX, BorderLayout.CENTER);
JButton btnPositive = new JButton("+/-");
btnPositive.addActionListener(new ActionListener()
@Override
public void actionPerformed(ActionEvent e)
String text=textFiled.getText();
if(!text.equals("0"))
if(text.charAt(0)=='-')textFiled.setText(text.substring(1));
else textFiled.setText("-"+text);
);
PWest.add(btnPositive);
JButton btnDot = new JButton(".");
PWest.add(btnDot);
btnDot.addActionListener(new ActionListener()
@Override
public void actionPerformed(ActionEvent e)
if(!textFiled.getText().contains("."))textFiled.setText(textFiled.getText()+".");
);
PEX.add(PWest, BorderLayout.WEST);
JPanel PEast = new JPanel();
PEast.setLayout(new GridLayout(4, 2, 2, 2));
JButton btnDevide = new JButton("/");
btnDevide.setForeground(Color.red);
btnDevide.addActionListener(new ActionListener()
@Override
public void actionPerformed(ActionEvent e)
a=textFiled.getText();
c='/';
textFiled.setText("0");
);
PEast.add(btnDevide);
JButton btnSqrt = new JButton("sqrt");
btnSqrt.addActionListener(new ActionListener()
@Override
public void actionPerformed(ActionEvent e)
textFiled.setText(BigDecimal.valueOf(Math.sqrt(Double.parseDouble(textFiled.getText()))).stripTrailingZeros().toPlainString());
);
PEast.add(btnSqrt);
JButton btnMultiply = new JButton("*");
btnMultiply.setForeground(Color.red);
btnMultiply.addActionListener(new ActionListener()
@Override
public void actionPerformed(ActionEvent e)
a=textFiled.getText();
c='*';
textFiled.setText("0");
);
PEast.add(btnMultiply);
JButton btnMod = new JButton("%");
PEast.add(btnMod);
btnMod.addActionListener(new ActionListener()
@Override
public void actionPerformed(ActionEvent e)
a=textFiled.getText();
c='%';
textFiled.setText("0");
);
JButton btnSubtract = new JButton("-");
btnSubtract.addActionListener(new ActionListener()
@Override
public void actionPerformed(ActionEvent e)
a=textFiled.getText();
c='-';
textFiled.setText("0");
);
btnSubtract.setForeground(Color.red);
PEast.add(btnSubtract);
JButton btnReverse = new JButton("1/x");
btnReverse.addActionListener(new ActionListener()
@Override
public void actionPerformed(ActionEvent e)
try
textFiled.setText(new BigDecimal("1").divide(new BigDecimal(textFiled.getText())).stripTrailingZeros().toPlainString());
catch(ArithmeticException e1)
textFiled.setText(new BigDecimal("1").divide(new BigDecimal(textFiled.getText()),15,BigDecimal.ROUND_HALF_UP).stripTrailingZeros().toPlainString());
);
PEast.add(btnReverse);
JButton btnAdd = new JButton("+");
btnAdd.setForeground(Color.red);
btnAdd.addActionListener(new ActionListener()
@Override
public void actionPerformed(ActionEvent e)
a=textFiled.getText();
c='+';
textFiled.setText("0");
);
PEast.add(btnAdd);
JButton btnEnter = new JButton("=");
btnEnter.setForeground(Color.red);
btnEnter.addActionListener(new ActionListener()
@Override
public void actionPerformed(ActionEvent e)
switch(c)
case '+':
textFiled.setText(new BigDecimal(a).add(new BigDecimal(textFiled.getText())).stripTrailingZeros().toPlainString());
break;
case '-':
textFiled.setText(new BigDecimal(a).subtract(new BigDecimal(textFiled.getText())).stripTrailingZeros().toPlainString());
break;
case '*':
textFiled.setText(new BigDecimal(a).multiply(new BigDecimal(textFiled.getText())).stripTrailingZeros().toPlainString());
break;
case '/':
try
textFiled.setText(new BigDecimal(a).divide(new BigDecimal(textFiled.getText())).stripTrailingZeros().toPlainString());
catch(ArithmeticException e1)
try
textFiled.setText(new BigDecimal(a).divide(new BigDecimal(textFiled.getText()),15,BigDecimal.ROUND_HALF_UP).stripTrailingZeros().toPlainString());
catch(ArithmeticException e2)
JOptionPane.showMessageDialog(null, "除数不能为0");
break;
case '%':
a=a.split("[.]")[0];
String b=textFiled.getText().split("[.]")[0];
try
textFiled.setText(new BigInteger(a).mod(new BigInteger(b)).toString());
catch(ArithmeticException e2)
JOptionPane.showMessageDialog(null, "除数不能为0");
break;
c='#';
);
PEast.add(btnEnter);
PEX.add(PEast, BorderLayout.EAST);
KeyboardFocusManager.getCurrentKeyboardFocusManager().addKeyEventPostProcessor(new KeyEventPostProcessor()
private long lastPress=0;
@Override
public boolean postProcessKeyEvent(KeyEvent e)
if(e.getID()==KeyEvent.KEY_PRESSED)
long time=System.currentTimeMillis();
if(time-lastPress>68)
lastPress=time;
if(Character.isDigit(e.getKeyChar()))
numButton[e.getKeyChar()-'0'].doClick();
else if(e.getKeyCode()==KeyEvent.VK_BACK_SPACE)btnBack.doClick();
else switch(e.getKeyChar())
case '.':
btnDot.doClick();
break;
case '+':
btnAdd.doClick();
break;
case '-':
btnSubtract.doClick();
break;
case '*':
btnMultiply.doClick();
break;
case '/':
btnDevide.doClick();
break;
case '%':
btnMod.doClick();
break;
case '=':
case '\\n':
btnEnter.doClick();
break;
return false;
);
public static void main(String[] args)
MyCalculator myCalculator=new MyCalculator();
myCalculator.setVisible(true);
myCalculator.requestFocus();
myCalculator.initText();
以上是关于swing计算器的主要内容,如果未能解决你的问题,请参考以下文章