上证指数
Posted 佩雷尔曼的信徒
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了上证指数相关的知识,希望对你有一定的参考价值。
1.数据处理模块
- 保存为 sh.py,保存位置 …\\Lib\\site-packages,这样以后调用直接 import sh.py 即可
# -*- coding: utf-8 -*- # sh.py class sh: # 本地数据库连接信息初始化,用 pyodbc 连接只需三个信息 def __init__(self, user=\'sa\', password=\'123456\', dsn=\'XiTongDSN\'): self.user = user self.password = password self.dsn = dsn import sqlalchemy # 建立数据库连接引擎,存取数据库需用到 self.engine = sqlalchemy.create_engine(\'mssql+pyodbc://\'+self.user+\':\'+self.password+\'@\'+self.dsn) # 从 tushare.org 下载上证指数 sh 数据 def get_url(self): import tushare import pandas dataframe = tushare.get_hist_data(\'sh\') # 由于 dataframe 存入 SQL Server 时 index 会报错,那就灵活处理下 # 把 index 复制成列 date 插到最后一列 index = list(dataframe[\'open\'].index) dataframe[\'date\'] = pandas.Series(index, index) pandas.DataFrame(dataframe, index) # 我只需列(开盘,最高,收盘,最低,成交量,日期),其他列统统删掉好了 dataframe.drop([\'price_change\', \'p_change\', \'ma5\', \'ma10\', \'ma20\', \'v_ma5\', \'v_ma10\', \'v_ma20\'], axis=1, inplace=True) # 将处理完的 dataframe 存入到本地数据库 dataframe.to_sql(\'sh\', self.engine, if_exists=\'replace\', index=False) # 调用本地数据库上证指数数据 sh def get_sql(self): import pandas connection = self.engine.connect() data = pandas.read_sql_table(\'sh\', connection) # 做处理时,由于 date 不连续且无什么意义,直接用计数当 date 用好了 index = list(data[\'open\'].index) o = data[\'open\'] h = data[\'high\'] c = data[\'close\'] l = data[\'low\'] volume = data[\'volume\'] return index, o, h, c, l, volume
- 调用 sh.py
# -*- coding: utf-8 -*- # 清屏 import os clearscreen = os.system(\'cls\') # 调用 sh.py 模块 import sh # 获取 sh 数据,先模块实例化 SH = sh.sh() \'\'\' # 下载数据到本地数据库 SH.get_url() \'\'\' # 调取数据库表 sh 数据 index, o, h, c, l, volume = SH.get_sql()
2.图形绘制模块
# -*- coding: utf-8 -*- # 清屏 import os clearscreen = os.system(\'cls\') # 调用模块 import sh # 获取 sh 数据,先模块实例化 SH = sh.sh() \'\'\' # 下载数据到本地数据库 SH.get_url() \'\'\' # 调取数据库表 sh 数据 index, o, h, c, l, volume = SH.get_sql() # 这是暴力的分割线~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ # 载入绘图模块 import matplotlib.pyplot as plot from matplotlib.finance import candlestick_ochl # 根据需要按列合并成一个 quotes = [] for i in range(0, len(index)): quotes.append((index[i], o[i], c[i], h[i], l[i], volume[i])) # 获取图表实例 figure = plot.figure(\'Made by DengChaohai\') # 上图 subplot1 = figure.add_subplot(211, title=\'Index of Shanghai\', xlabel=\'index of date\', ylabel=\'index of Shanghai\', xlim=[min(index), max(index)]) plot.grid(True, axis=\'both\') # K 线图 candlestick_ochl(subplot1, quotes, colorup=\'r\', colordown=\'g\') # 成交量 bar 图,为了好看点,进行了缩放处理 b11 = subplot1.bar(left=index, height=[i*100/min(volume) for i in volume], bottom=0, width=1, color=\'c\', edgecolor=\'c\') # 下图 subplot2 = figure.add_subplot(212, title=\'Singal of Buy or Sell\', xlabel=\'index of date\', ylabel=\'index of Shanghai\', xlim=[min(index), max(index)]) plot.grid(True, axis=\'both\') # open 线图 subplot2.plot(o, \'g\') # 自定义加权价 weighting price wp = [] for i in range(0, len(index)): wp.append(o[i]*2/7+c[i]*3/7+h[i]/7+l[i]/7) # weighting price 线图 subplot2.plot(wp, \'r\') # 差价 bar 图,为好看同意进行了缩放 subplot2.bar(left=index, height=[(o[i]-wp[i])*10 for i in range(0, len(index))], bottom=0, width=1, color=\'c\', edgecolor=\'c\')
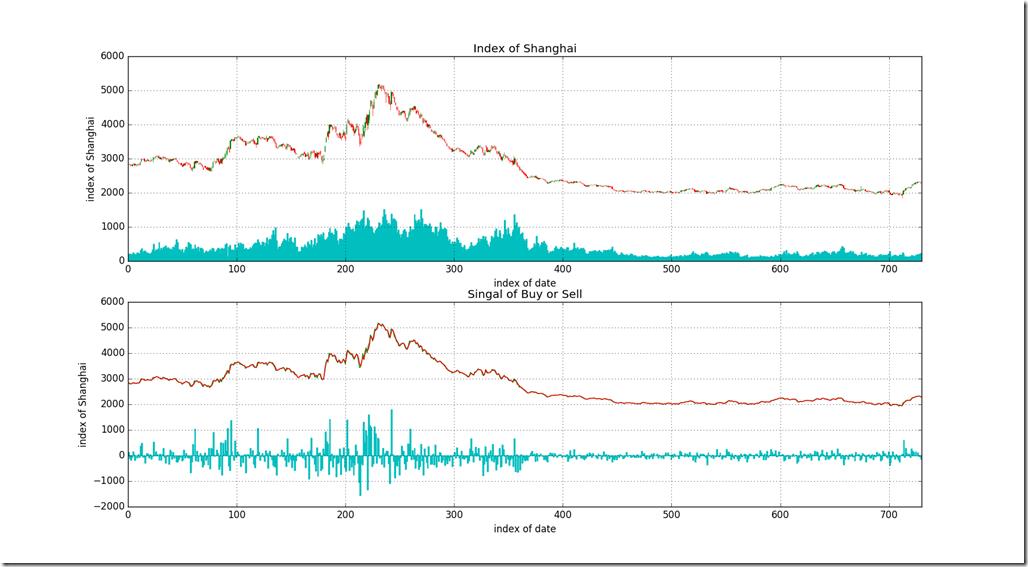
3.买卖信号模块
- 保存为 bs.py,保存位置 …\\Lib\\site-packages,这样以后调用直接 import bs.py 即可
# -*- coding: utf-8 -*- class bs: # 数据初始化 def __init__(self, index, o, wp): self.index = index self.o = o self.wp = wp # 买点发生器 def buy(self): bindex = [] bo = [] bkey = [0] for i in range(1, len(self.index)): # 买点条件 if (self.o[i] > self.wp[i]) and (self.o[i-1] < self.wp[i-1]): bindex.append(self.index[i]) bo.append(self.o[i]) bkey.append(1) else: bkey.append(0) # 返回买点的位置,用于画图,bkey 则是插入回数据库 sh 用,为后续交易铺垫 return bindex, bo, bkey def sell(self): sindex = [] so = [] skey = [0] for i in range(1, len(self.index)): if (self.o[i] < self.wp[i]) and (self.o[i-1] > self.wp[i-1]): sindex.append(self.index[i]) so.append(self.o[i]) skey.append(1) else: skey.append(0) return sindex, so, skey
- 调用效果
# -*- coding: utf-8 -*- # 清屏 import os clearscreen = os.system(\'cls\') # 调用模块 import sh # 获取 sh 数据,先模块实例化 SH = sh.sh() \'\'\' # 下载数据到本地数据库 SH.get_url() \'\'\' # 调取数据库表 sh 数据 index, o, h, c, l, volume = SH.get_sql() # 这是暴力的分割线~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ # 载入绘图模块 import matplotlib.pyplot as plot from matplotlib.finance import candlestick_ochl # 根据需要按列合并成一个 quotes = [] for i in range(0, len(index)): quotes.append((index[i], o[i], c[i], h[i], l[i], volume[i])) # 获取图表实例 figure = plot.figure(\'Made by DengChaohai\') # 上图 subplot1 = figure.add_subplot(211, title=\'Index of Shanghai\', xlabel=\'index of date\', ylabel=\'index of Shanghai\', xlim=[min(index), max(index)]) plot.grid(True, axis=\'both\') # K 线图 candlestick_ochl(subplot1, quotes, colorup=\'r\', colordown=\'g\') # 成交量 bar 图,为了好看点,进行了缩放处理 b11 = subplot1.bar(left=index, height=[i*100/min(volume) for i in volume], bottom=0, width=1, color=\'c\', edgecolor=\'c\') # 下图 subplot2 = figure.add_subplot(212, title=\'Singal of Buy or Sell\', xlabel=\'index of date\', ylabel=\'index of Shanghai\', xlim=[min(index), max(index)]) plot.grid(True, axis=\'both\') # open 线图 subplot2.plot(o, \'g\') # 自定义加权价 weighting price wp = [] for i in range(0, len(index)): wp.append(o[i]*2/7+c[i]*3/7+h[i]/7+l[i]/7) # weighting price 线图 subplot2.plot(wp, \'r\') # 差价 bar 图,为好看同意进行了缩放 subplot2.bar(left=index, height=[(o[i]-wp[i])*10 for i in range(0, len(index))], bottom=0, width=1, color=\'c\', edgecolor=\'c\') # 这是暴力的分割线~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ import bs BS = bs.bs(index, o, wp) bindex, bo, bkey = BS.buy() subplot2.plot(bindex, bo, \'ro\') sindex, so, skey = BS.sell() subplot2.plot(sindex, so, \'go\')
- 承上,把 buy sell 信号存回表 sh
# 这是暴力的分割线~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ # 把 bkey skey 当作列存回 sh 数据表 # 先合并新的 dataframe import pandas # 提取旧表 oldsh = pandas.read_sql(\'sh\', SH.engine.connect()) oldsh[\'buy\'] = pandas.Series(bkey, list(oldsh[\'open\'].index)) oldsh[\'sell\'] = pandas.Series(skey, list(oldsh[\'open\'].index))
pandas.DataFrame(oldsh, bkey) pandas.DataFrame(oldsh, skey) # 存回新表 oldsh.to_sql(\'sh\', SH.engine, if_exists=\'replace\', index=False)
4.交易测试模块
# -*- coding: utf-8 -*- ######################################################################## class trade: """交易模块""" #---------------------------------------------------------------------- def __init__(self, money = 1000000, rate = 0.01): """初始函数""" \'\'\'本金\'\'\' self.money = money \'\'\'比例,即每次买股票的钱占本金的比例\'\'\' self.rate = rate \'\'\'每次买股票的钱\'\'\' self.moneybuy = money * rate \'\'\'持有股票,初始为 0 \'\'\' self.stockhold = 0 \'\'\'计数,用于往前一级级跳数\'\'\' self.k = 0 \'\'\'用于绘制资金曲线\'\'\' self.amount = [0] #---------------------------------------------------------------------- def trade(self, index = [1, 2, 3, 4, 5], buy = [1, 0, 0, 1, 0], sell = [0, 1, 1, 0, 1], price = [0.1, 0.2, 0.3, 0.4, 0.5]): """交易函数""" \'\'\'遍历买点\'\'\' for i in range(self.k, len(buy) - 1): \'\'\'如果出现买点,则\'\'\' if buy[i] == 1: \'\'\'钱减少\'\'\' self.money = self.money - self.moneybuy \'\'\'股票增加\'\'\' self.stockhold = self.moneybuy / price[i] \'\'\'如果股票持有数不空,遍历卖点\'\'\' while self.stockhold: \'\'\'k + 1 因为买入后第二天才能卖\'\'\' for i in range(self.k + 1, len(sell) - 1): \'\'\'如果碰到卖点,则\'\'\' if sell[i] == 1: \'\'\'钱增加\'\'\' self.money = self.money + self.stockhold * price[i] \'\'\'股票清空\'\'\' self.stockhold = 0 \'\'\'计数加一,从第二天起重新遍历买点\'\'\' self.k = self.k + 1 \'\'\'用于绘制资金曲线\'\'\' self.amount.append(self.money) \'\'\'跳出 for 循环\'\'\' break \'\'\'返回钱数\'\'\' return self.money, self.amount
- 调用
# -*- coding: utf-8 -*- \'\'\'添加文件路径\'\'\' import sys sys.path.append(\'D:\\360data\\重要数据\\桌面\\sh\') """调用 sh.py 模块""" import sh Sh = sh.sh() Index, Open, High, Close, Low, Volume = Sh.get_sql() """调用 bs.py 模块""" import bs Bs = bs.bs(Index, Open, Close) a, b, c = Bs.buy() d, e, f = Bs.sell() """调用 trade.py 模块""" import trade Trade = trade.trade(money=1000000, rate=0.05) Money, Amount = Trade.trade(index = Index, buy = c, sell = f, price = Open) print(\'total money = \', Money) \'\'\'绘制资金曲线\'\'\' import matplotlib.pyplot as plot plot.plot(Amount, \'g-\')
以上是关于上证指数的主要内容,如果未能解决你的问题,请参考以下文章