ES6 Promise 用法讲解(转)
Posted 呗儿
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了ES6 Promise 用法讲解(转)相关的知识,希望对你有一定的参考价值。
Promise是一个构造函数,自己身上有all、reject、resolve这几个眼熟的方法,原型上有then、catch等同样很眼熟的方法。
var p = new Promise(function(resolve, reject){
//做一些异步操作
setTimeout(function(){
console.log(\'执行完成\');
resolve(\'随便什么数据\');
}, 2000);
});
function runAsync(){
var p = new Promise(function(resolve, reject){
//做一些异步操作
setTimeout(function(){
console.log(\'执行完成\');
resolve(\'随便什么数据\');
}, 2000);
});
return p;
}
runAsync()
runAsync().then(function(data){ console.log(data); //后面可以用传过来的数据做些其他操作 //...... });
function runAsync(callback){ setTimeout(function(){ console.log(\'执行完成\'); callback(\'随便什么数据\'); }, 2000); } runAsync(function(data){ console.log(data); });
链式操作的用法
runAsync1() .then(function(data){ console.log(data); return runAsync2(); }) .then(function(data){ console.log(data); return runAsync3(); }) .then(function(data){ console.log(data); });
这样能够按顺序,每隔两秒输出每个异步回调中的内容,在runAsync2中传给resolve的数据,能在接下来的then方法中拿到。运行结果如下:
猜猜runAsync1、runAsync2、runAsync3这三个函数都是如何定义的?没错,就是下面这样
function runAsync1(){ var p = new Promise(function(resolve, reject){ //做一些异步操作 setTimeout(function(){ console.log(\'异步任务1执行完成\'); resolve(\'随便什么数据1\'); }, 1000); }); return p; } function runAsync2(){ var p = new Promise(function(resolve, reject){ //做一些异步操作 setTimeout(function(){ console.log(\'异步任务2执行完成\'); resolve(\'随便什么数据2\'); }, 2000); }); return p; } function runAsync3(){ var p = new Promise(function(resolve, reject){ //做一些异步操作 setTimeout(function(){ console.log(\'异步任务3执行完成\'); resolve(\'随便什么数据3\'); }, 2000); }); return p; }
在then方法中,你也可以直接return数据而不是Promise对象,在后面的then中就可以接收到数据了,比如我们把上面的代码修改成这样:
runAsync1() .then(function(data){ console.log(data); return runAsync2(); }) .then(function(data){ console.log(data); return \'直接返回数据\'; //这里直接返回数据 }) .then(function(data){ console.log(data); });
那么输出就变成了这样:
reject的用法
function getNumber(){ var p = new Promise(function(resolve, reject){ //做一些异步操作 setTimeout(function(){ var num = Math.ceil(Math.random()*10); //生成1-10的随机数 if(num<=5){ resolve(num); } else{ reject(\'数字太大了\'); } }, 2000); }); return p; } getNumber() .then( function(data){ console.log(\'resolved\'); console.log(data); }, function(reason, data){ console.log(\'rejected\'); console.log(reason); } );


catch的用法
getNumber() .then(function(data){ console.log(\'resolved\'); console.log(data); }) .catch(function(reason){ console.log(\'rejected\'); console.log(reason); });
效果和写在then的第二个参数里面一样。不过它还有另外一个作用:在执行resolve的回调(也就是上面then中的第一个参数)时,如果抛出异常了(代码出错了),那么并不会报错卡死js,而是会进到这个catch方法中。请看下面的代码:
getNumber() .then(function(data){ console.log(\'resolved\'); console.log(data); console.log(somedata); //此处的somedata未定义 }) .catch(function(reason){ console.log(\'rejected\'); console.log(reason); });

all的用法
Promise .all([runAsync1(), runAsync2(), runAsync3()]) .then(function(results){ console.log(results); });
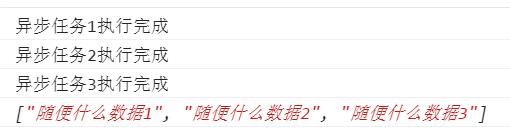
race的用法
Promise .race([runAsync1(), runAsync2(), runAsync3()]) .then(function(results){ console.log(results); });
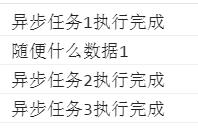
//请求某个图片资源 function requestImg(){ var p = new Promise(function(resolve, reject){ var img = new Image(); img.onload = function(){ resolve(img); } img.src = \'xxxxxx\'; }); return p; } //延时函数,用于给请求计时 function timeout(){ var p = new Promise(function(resolve, reject){ setTimeout(function(){ reject(\'图片请求超时\'); }, 5000); }); return p; } Promise .race([requestImg(), timeout()]) .then(function(results){ console.log(results); }) .catch(function(reason){ console.log(reason); });

以上是关于ES6 Promise 用法讲解(转)的主要内容,如果未能解决你的问题,请参考以下文章