设计模式
Posted 吼怠
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了设计模式相关的知识,希望对你有一定的参考价值。
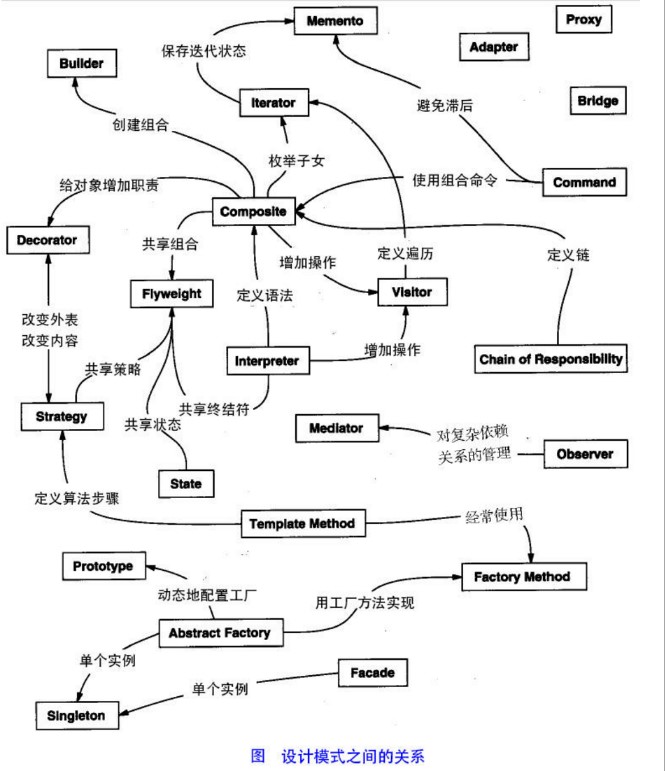
五种创建型模式
1、工厂模式
2、抽象工厂模式
3、单例模式
4、建造者模式
概述:相比较工厂模式而言,就是建造模式可以一次建造很多个对象,工厂模式一次只能建造一个。
<wiz_code_mirror>
xxxxxxxxxx
15
1
public class Builder {
2
3
private List<Sender> list = new ArrayList<Sender>();
4
5
public void produceMailSender(int count){
6
for(int i=0; i<count; i++){
7
list.add(new MailSender());
8
}
9
}
10
public void produceSmsSender(int count){
11
for(int i=0; i<count; i++){
12
list.add(new SmsSender());
13
}
14
}
15
}
<wiz_code_mirror>
xxxxxxxxxx
7
1
public class Test {
2
3
public static void main(String[] args) {
4
Builder builder = new Builder();
5
builder.produceMailSender(10);
6
}
7
}
5、原型模式
原型模式的思想就是将一个对象作为原型,对其进行复制、克隆产生一个和元对象类似的新对象,在Java中,复制对象就是通过clone()实现的,先创建一个原型类:
<wiz_code_mirror>
xxxxxxxxxx
6
1
public class Prototype implements Cloneable{
2
public Object clone() throws CloneNotSupportedException{
3
Prototype proto = (Prototype)super.clone();
4
return proto;
5
}
6
}
进行实现Cloneable接口,完成克隆,因为Cloneable接口是一个空接口,因此实现类的方法名称可以任意,但是在实现的内部就是必须调用super.clone()方法。
对于对象的复制,主要分为浅复制和深复制两种:
浅复制:将一个对象复制后,基本数据类型的变量会重新创建,而对于引用类型,还是指向原来对象
深复制:当然和浅复制有区别就是完全的复制,一般需要采用流的形式读入当前对象的二进制输入,再写出二进制数据对应的对象。
<wiz_code_mirror>
xxxxxxxxxx
42
1
public class Prototype implements Cloneable,Serializable{
2
private static final long serialVersionUID = 1L;
3
private String string;
4
5
private SerializableObject obj;
6
7
/*浅复制*/
8
public Object clone() throws CloneNotSupportedException{
9
Prototype proto = (Prototype) super.clone();
10
return proto;
11
}
12
13
/*深复制*/
14
public Object deepclone() throws IOException ,ClassNotFoundException{
15
16
/*写入当前对象的二进制流*/
17
ByteArrayOutputStream bos = new ByteArrayOutputStream();
18
ObjectOutputStream oos = new ObjectOutputStream(bos);
19
oos.writeObject(this);
20
21
/*读取二进制流产生的新对象*/
22
ByteArrayInputStream bis = new ByteArrayInputStream(bos.toByteArray());
23
ObjectInputStream ois = new ObjectInputStream(bis);
24
return ois.readObject();
25
}
26
27
public String getString(){
28
return string;
29
}
30
31
public void setString(String string){
32
this.string = string;
33
}
34
35
public SerializableObject getObj(){
36
return obj;
37
}
38
39
public void setObj(SerializableObject obj){
40
this.obj = obj;
41
}
42
}
七种结构模式
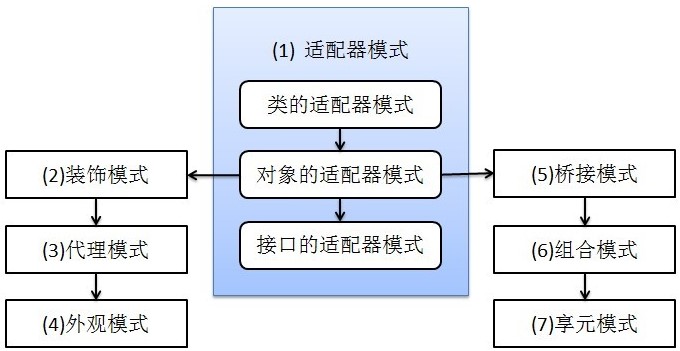
适配器模式
对于适配模式可以分为类的适配器模式、对象的适配器模式、接口的适配器模式
类的适配器模式:
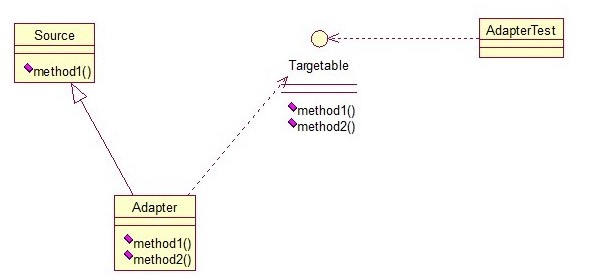
<wiz_code_mirror>
xxxxxxxxxx
32
1
public class Source {
2
3
public void method1() {
4
System.out.println("this is original method!");
5
}
6
}
7
8
public interface Targetable {
9
10
/* 与原类中的方法相同 */
11
public void method1();
12
13
/* 新类的方法 */
14
public void method2();
15
}
16
17
public class Adapter extends Source implements Targetable {
18
19
@Override
20
public void method2() {
21
System.out.println("this is the targetable method!");
22
}
23
}
24
25
public class AdapterTest {
26
27
public static void main(String[] args) {
28
Targetable target = new Adapter();
29
target.method1();
30
target.method2();
31
}
32
}
对象的适配
基本思路类的适配是类似的,就是把source类不继承了,而是把source类进行实例,包含在内部。
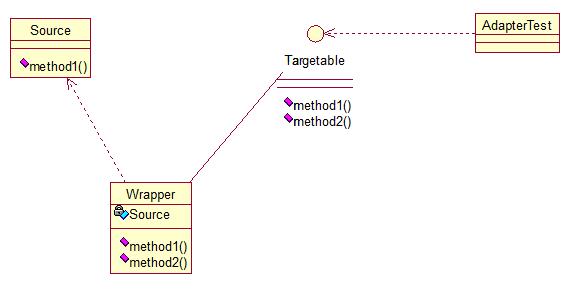
<wiz_code_mirror>
xxxxxxxxxx
29
1
public class Wrapper implements Targetable {
2
3
private Source source;
4
5
public Wrapper(Source source){
6
super();
7
this.source = source;
8
}
9
@Override
10
public void method2() {
11
System.out.println("this is the targetable method!");
12
}
13
14
@Override
15
public void method1() {
16
source.method1();
17
}
18
}
19
20
测试类:
21
public class AdapterTest {
22
23
public static void main(String[] args) {
24
Source source = new Source();
25
Targetable target = new Wrapper(source);
26
target.method1();