使用Spring MVC统一异常处理实战
Posted
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了使用Spring MVC统一异常处理实战相关的知识,希望对你有一定的参考价值。
1 描写叙述
在J2EE项目的开发中。无论是对底层的数据库操作过程。还是业务层的处理过程,还是控制层的处理过程,都不可避免会遇到各种可预知的、不可预知的异常须要处理。每一个过程都单独处理异常,系统的代码耦合度高,工作量大且不好统一。维护的工作量也非常大。
那么,能不能将全部类型的异常处理从各处理过程解耦出来,这样既保证了相关处理过程的功能较单一,也实现了异常信息的统一处理和维护?答案是肯定的。
2 分析
Spring MVC处理异常有3种方式:
(1)使用Spring MVC提供的简单异常处理器SimpleMappingExceptionResolver。
(2)实现Spring的异常处理接口HandlerExceptionResolver 自己定义自己的异常处理器;
(3)使用@ExceptionHandler注解实现异常处理;
3 实战
3.1 引言
为了验证Spring MVC的3种异常处理方式的实际效果。我们须要开发一个測试项目,从Dao层、Service层、Controller层分别抛出不同的异常。然后分别集成3种方式进行异常处理,从而比較3种方式的优缺点。
3.2 实战项目
3.2.1 项目结构
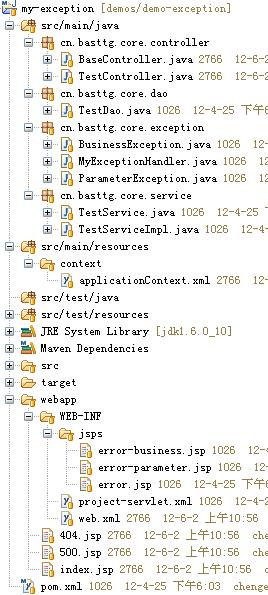
3.2.2 Dao层代码
3.2.3 Service层代码
3.2.4 Controller层代码
3.2.5 JSP页面代码
3.3 集成异常处理
3.3.1 使用SimpleMappingExceptionResolver实现异常处理
1、在Spring的配置文件applicationContext.xml中添加下面内容:
在J2EE项目的开发中。无论是对底层的数据库操作过程。还是业务层的处理过程,还是控制层的处理过程,都不可避免会遇到各种可预知的、不可预知的异常须要处理。每一个过程都单独处理异常,系统的代码耦合度高,工作量大且不好统一。维护的工作量也非常大。
那么,能不能将全部类型的异常处理从各处理过程解耦出来,这样既保证了相关处理过程的功能较单一,也实现了异常信息的统一处理和维护?答案是肯定的。
以下将介绍使用Spring MVC统一处理异常的解决和实现过程。
2 分析
Spring MVC处理异常有3种方式:
(1)使用Spring MVC提供的简单异常处理器SimpleMappingExceptionResolver。
(2)实现Spring的异常处理接口HandlerExceptionResolver 自己定义自己的异常处理器;
(3)使用@ExceptionHandler注解实现异常处理;
3 实战
3.1 引言
为了验证Spring MVC的3种异常处理方式的实际效果。我们须要开发一个測试项目,从Dao层、Service层、Controller层分别抛出不同的异常。然后分别集成3种方式进行异常处理,从而比較3种方式的优缺点。
3.2 实战项目
3.2.1 项目结构
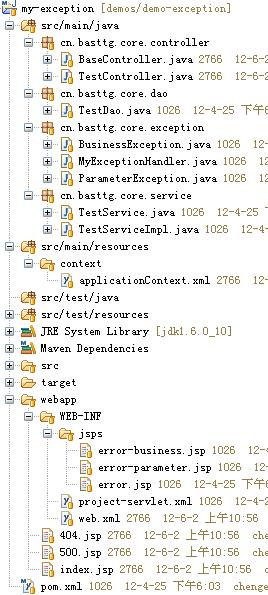
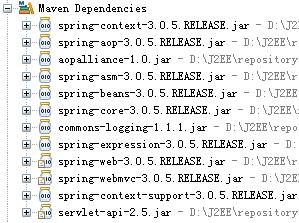
3.2.2 Dao层代码
- @Repository("testDao")
- public class TestDao {
- public void exception(Integer id) throws Exception {
- switch(id) {
- case 1:
- throw new BusinessException("12", "dao12");
- case 2:
- throw new BusinessException("22", "dao22");
- case 3:
- throw new BusinessException("32", "dao32");
- case 4:
- throw new BusinessException("42", "dao42");
- case 5:
- throw new BusinessException("52", "dao52");
- default:
- throw new ParameterException("Dao Parameter Error");
- }
- }
- }
3.2.3 Service层代码
- public interface TestService {
- public void exception(Integer id) throws Exception;
- public void dao(Integer id) throws Exception;
- }
- @Service("testService")
- public class TestServiceImpl implements TestService {
- @Resource
- private TestDao testDao;
- public void exception(Integer id) throws Exception {
- switch(id) {
- case 1:
- throw new BusinessException("11", "service11");
- case 2:
- throw new BusinessException("21", "service21");
- case 3:
- throw new BusinessException("31", "service31");
- case 4:
- throw new BusinessException("41", "service41");
- case 5:
- throw new BusinessException("51", "service51");
- default:
- throw new ParameterException("Service Parameter Error");
- }
- }
- @Override
- public void dao(Integer id) throws Exception {
- testDao.exception(id);
- }
- }
3.2.4 Controller层代码
- @Controller
- public class TestController {
- @Resource
- private TestService testService;
- @RequestMapping(value = "/controller.do", method = RequestMethod.GET)
- public void controller(HttpServletResponse response, Integer id) throws Exception {
- switch(id) {
- case 1:
- throw new BusinessException("10", "controller10");
- case 2:
- throw new BusinessException("20", "controller20");
- case 3:
- throw new BusinessException("30", "controller30");
- case 4:
- throw new BusinessException("40", "controller40");
- case 5:
- throw new BusinessException("50", "controller50");
- default:
- throw new ParameterException("Controller Parameter Error");
- }
- }
- @RequestMapping(value = "/service.do", method = RequestMethod.GET)
- public void service(HttpServletResponse response, Integer id) throws Exception {
- testService.exception(id);
- }
- @RequestMapping(value = "/dao.do", method = RequestMethod.GET)
- public void dao(HttpServletResponse response, Integer id) throws Exception {
- testService.dao(id);
- }
- }
3.2.5 JSP页面代码
- <%@ page contentType="text/html; charset=UTF-8"%>
- <html>
- <head>
- <title>Maven Demo</title>
- </head>
- <body>
- <h1>全部的示例</h1>
-
<h3>[url=./dao.do?
id=1]Dao正常错误[/url]</h3>
- <h3>[url=./dao.do?id=10]Dao參数错误[/url]</h3>
- <h3>[url=./dao.do?id=]Dao未知错误[/url]</h3>
- <h3>[url=./service.do?id=1]Service正常错误[/url]</h3>
- <h3>[url=./service.do?id=10]Service參数错误[/url]</h3>
- <h3>[url=./service.do?id=]Service未知错误[/url]</h3>
- <h3>[url=./controller.do?id=1]Controller正常错误[/url]</h3>
- <h3>[url=./controller.do?id=10]Controller參数错误[/url]</h3>
- <h3>[url=./controller.do?id=]Controller未知错误[/url]</h3>
- <h3>[url=./404.do?id=1]404错误[/url]</h3>
- </body>
- </html>
3.3 集成异常处理
3.3.1 使用SimpleMappingExceptionResolver实现异常处理
1、在Spring的配置文件applicationContext.xml中添加下面内容:
- <bean class="org.springframework.web.servlet.handler.SimpleMappingExceptionResolver">
- <!-- 定义默认的异常处理页面,当该异常类型的注冊时使用 -->
- <property name="defaultErrorView" value="error"></property>
- <!-- 定义异常处理页面用来获取异常信息的变量名,默认名为exception -->
- <property name="exceptionAttribute" value="ex"></property>
- <!-- 定义须要特殊处理的异常,用类名或全然路径名作为key,异常也页名作为值 -->
-
<property
以上是关于使用Spring MVC统一异常处理实战的主要内容,如果未能解决你的问题,请参考以下文章
Spring MVC学习—项目统一异常处理机制详解与使用案例