Python - Class and Objects
Posted
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了Python - Class and Objects相关的知识,希望对你有一定的参考价值。
Object-oriented programming
Python is OOP, Usually, each object definition corresponds to some object or concept in the real world and the functions that operate on that object correspond to the ways real-world objects interact.
In python, every value is actually an object. An object has a state and a collection of methods that it can perform.
The state of an object represents those things that the object knows about itself.
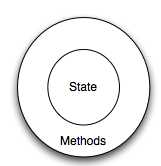
User Defined Classes
1 class Point: 3 """ Point class for representing and manipulating x,y coordinates. """ 4 5 def __init__(self): 6 """ Create a new point at the origin """ 7 self.x = 0 8 self.y = 0
Every class should have a method with the special name _init_. This is Initializer method often called constructor.
A method behaves like a function but it is invoked on a specific instance.
The _str_ method is responsible for returning a string representation as defined by the class creator.
class Point: """ Point class for representing and manipulating x,y coordinates. """ def __init__(self, initX, initY): """ Create a new point at the origin """ self.x = initX self.y = initY
def _str_(self):
return "x=" + str(self.x) + ", y=" + str(self.y)
def getX(self):
return self.x
def getY(self):
return self.y
def distanceFromOrigin(self):
return ((self.x ** 2) + (self.y ** 2)) ** 0.5
def halfway(self, target):
mx = (self.x + target.x) / 2
my = (self.y + target.y) / 2
return Point(mx, my)
def distance(point1, point2):
xdiff = point2.getX() - point1.getX()
ydiff = point2.getY() - point1.getY()
dist = math.sqrt(xdiff ** 2 + ydiff ** 2)
return dist
p = Point(7,6) # Instantiate an object of type Point q = Point(2,2) # and make a second point mid = p.halfway(q)
print(mid)
print(mid.getX())
print(mid.getY()) print(p)
print(p.getX())
print(p.getY())
print(p.distanceFromOrigin()) print(q) print(distance(p,q)) print(p is q)
以上是关于Python - Class and Objects的主要内容,如果未能解决你的问题,请参考以下文章
hausaufgabe--python 39 -- objects and class
016: class, objects and instance: method
UML Class and Object Diagrams Overview
Java6.2 类和对象 Class and Object 03 修饰符