20175221曾祥杰 实验三《敏捷开发与XP实践》
Posted zxja
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了20175221曾祥杰 实验三《敏捷开发与XP实践》相关的知识,希望对你有一定的参考价值。
实验三《敏捷开发与XP实践》
实验报告封面
课程:Java程序设计 班级:1752班 姓名:曾祥杰 学号:20175221
指导教师:娄嘉鹏 实验日期:2019年4月30日
实验时间:13:45 - 15:25 实验序号:21
实验名称:敏捷开发与XP实践
一.实验内容
1. XP基础
2. XP核心实践
3. 相关工具
二.实验步骤
-
第一部分:
-
要求:参考 http://www.cnblogs.com/rocedu/p/6371315.html#SECCODESTANDARD 安装alibaba 插件,解决代码中的规范问题。
-
在IDEA中使用工具(Code->Reformate Code)把下面代码重新格式化,再研究一下Code菜单,找出一项让自己感觉最好用的功能。提交截图,加上自己学号水印。
1 public class CodeStandard {
2 public static void main(String [] args){
3 StringBuffer buffer = new StringBuffer();
4 buffer.append(‘S‘);
5 buffer.append("tringBuffer");
6 System.out.println(buffer.charAt(1));
7 System.out.println(buffer.capacity());
8 System.out.println(buffer.indexOf("tring"));
9 System.out.println("buffer = " + buffer.toString());
10 if(buffer.capacity()<20)
11 buffer.append("1234567");
12 for(int i=0; i<buffer.length();i++)
13 System.out.println(buffer.charAt(i));
14 }
15 }
- 首先在IDEA中安装alibaba插件, Settings → Plugins → Marketplace ,然后在搜素栏输入 Alibaba ,即可看到 Alibaba Java Code Guidelines 插件,点击Install进行安装,然后重启IDE生效。
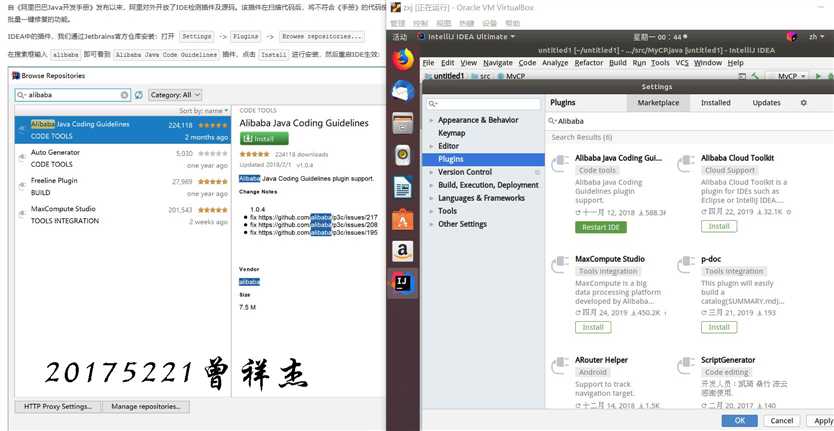
- 使用比较简单:在项目名称上单击右键,在弹出菜单上选择“
编码规约扫描
”
随后即可
- 随后即可根据报错内容进行规范修改
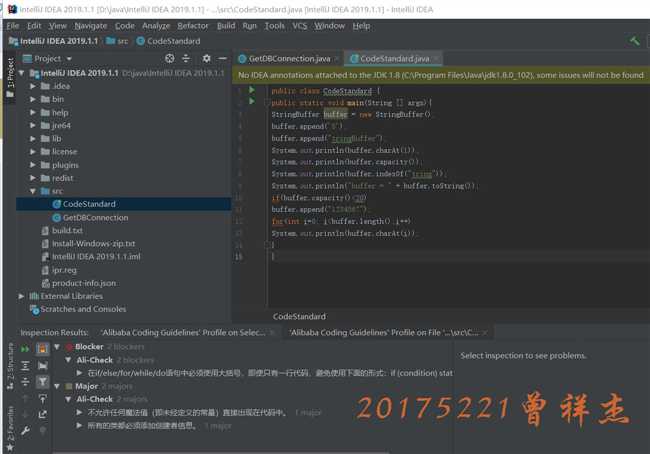
- 我们可以使用 Code → Reformat Code (或 Ctrl + Alt +L )规范缩进,规范后的代码如下:
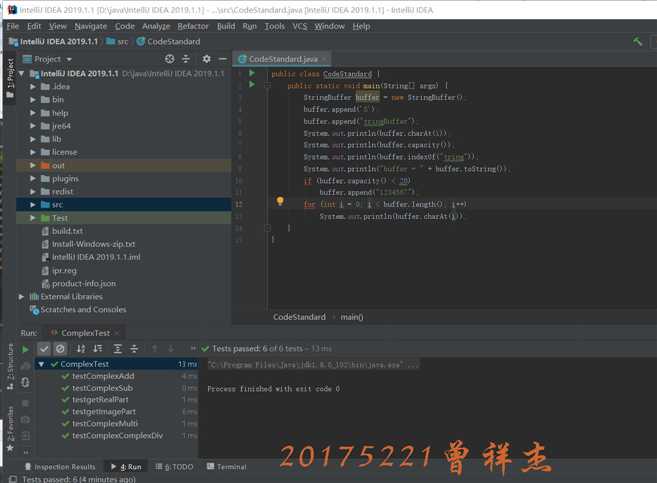
- code菜单中,我感觉最好用的功能就是 Move Statement Up 和 Move Statement Down ,整行移动简直不要太好玩(划掉),太方便!
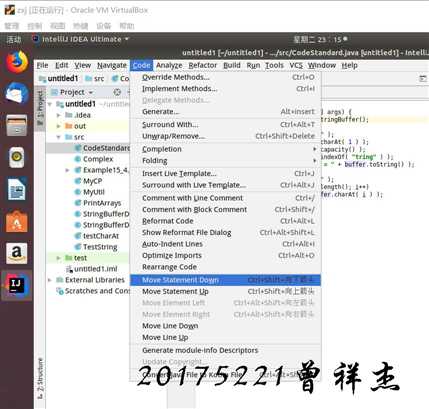
-
第二部分:
-
要求:在码云上把自己的学习搭档加入自己的项目中,确认搭档的项目加入自己后,下载搭档实验二的Complex代码,加入不少于三个JUnit单元测试用例,测试成功后git add .; git commit -m "自己学号 添加内容";git push;
-
提交搭档项目git log的截图,包含上面git commit的信息,并加上自己的学号水印信息。
- 首先将搭档拉入自己的项目中:管理→仓库成员管理→管理员→添加仓库成员→邀请用户
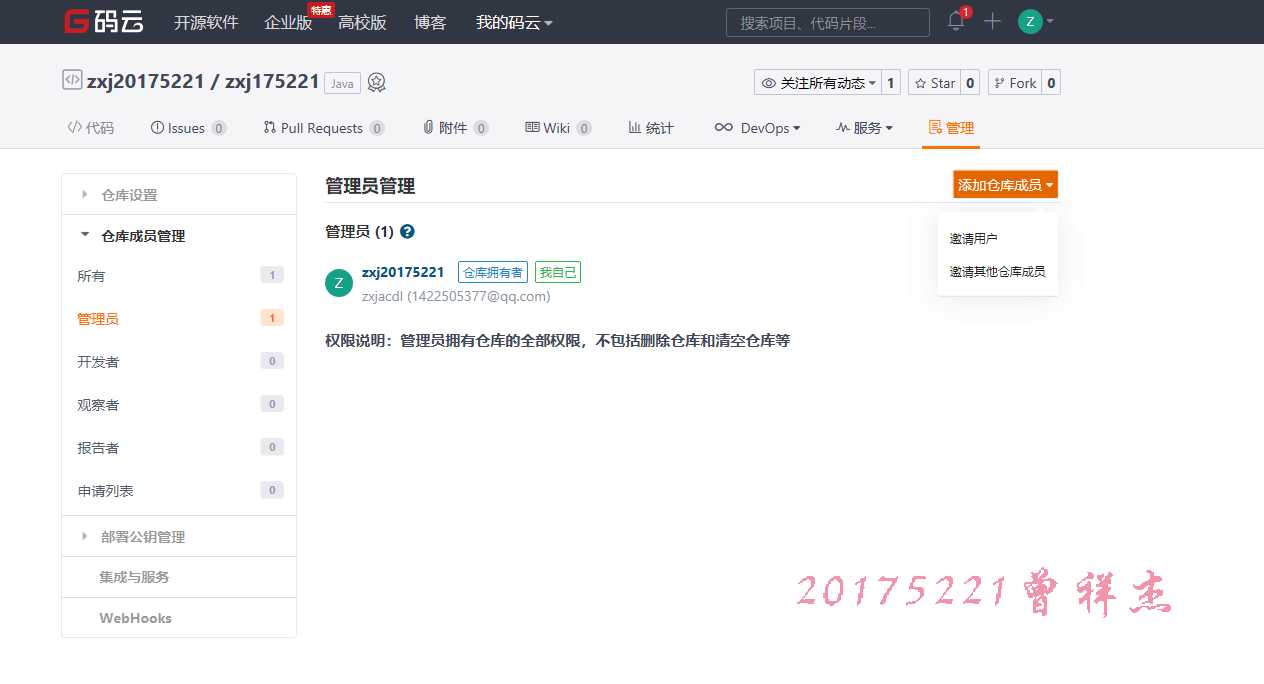
- 产生链接

- 邀请成功
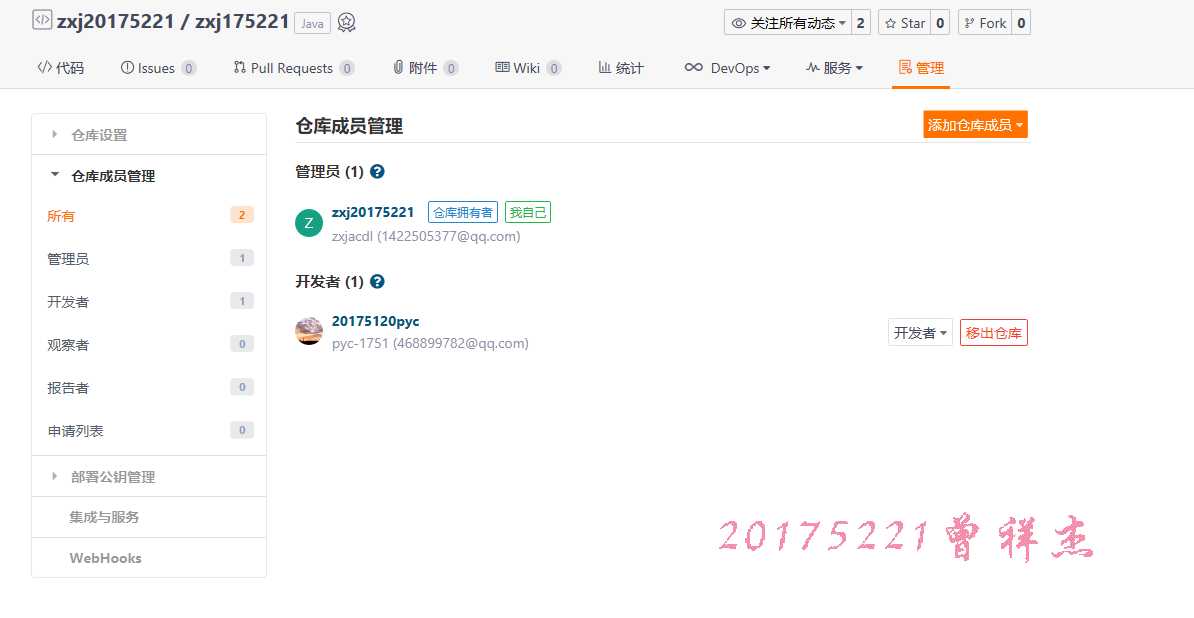
- 下载搭档代码
1 public class Complex {
2 // 定义属性并生成getter,setter
3 double RealPart;
4 double ImagePart;
5
6 // 定义构造函数
7 public Complex(){
8 }
9 public Complex(double R,double I){
10 RealPart=R;
11 ImagePart=I;
12 }
13
14 public static double RealPart(double v) {
15 return 1;
16 }
17
18 public static double ImagePart(double v) {
19 return 0;
20 }
21
22 //Override Object
23 public boolean equals(Complex m){
24 if(m.RealPart==this.RealPart&&m.ImagePart==this.ImagePart){
25 return true;
26 }
27 else{
28 return false;
29 }
30 }
31 public String toString(){
32 if (this.RealPart != 0 && this.ImagePart > 0) {
33 return this.RealPart + " + " + this.ImagePart + "i";
34 } else if (this.RealPart != 0 && this.ImagePart == 0) {
35 return String.valueOf(this.RealPart);
36 } else if (this.RealPart != 0 && this.ImagePart < 0) {
37 return this.RealPart + " - " + -this.ImagePart + "i";
38 } else if (this.RealPart == 0 && this.ImagePart != 0) {
39 return this.ImagePart + "i";
40 } else {
41 return "0";
42 }
43 }
44
45 // 定义公有方法:加减乘除
46 Complex ComplexAdd(Complex a){
47 return new Complex(this.RealPart + a.RealPart, this.ImagePart + a.ImagePart);
48 }
49 Complex ComplexSub(Complex a){
50 return new Complex(this.RealPart - a.RealPart, this.ImagePart - a.ImagePart);
51 }
52 Complex ComplexMulti(Complex a){
53 return new Complex(this.RealPart * a.RealPart - this.ImagePart * a.ImagePart,
54 this.ImagePart * a.RealPart + this.RealPart * a.ImagePart);
55 }
56 Complex ComplexDiv(Complex a){
57 return new Complex((this.ImagePart * a.ImagePart + this.RealPart * a.RealPart) / (a.ImagePart * a.ImagePart + a.RealPart * a.RealPart),
58 (this.RealPart * a.ImagePart - this.ImagePart * a.RealPart) / (a.ImagePart * a.ImagePart + a.RealPart * a.RealPart));
59 }
60 }
1 import junit.framework.TestCase;
2 import org.junit.Test;
3
4 public class ComplexTest extends TestCase {
5 Complex a = new Complex(0.0, 2.0);
6 Complex b = new Complex(-1.0, -1.0);
7 Complex c = new Complex(1.0,2.0);
8 @Test
9 public void testgetRealPart() throws Exception {
10 assertEquals(1.0, Complex.RealPart(0.0));
11 assertEquals(1.0, Complex.RealPart(-1.0));
12 assertEquals(1.0, Complex.RealPart(1.0));
13 }
14 @Test
15 public void testgetImagePart() throws Exception {
16 assertEquals(0.0, Complex.ImagePart(2.0));
17 assertEquals(0.0, Complex.ImagePart(-1.0));
18 assertEquals(0.0, Complex.ImagePart(2.0));
19 }
20 @Test
21 public void testComplexAdd() throws Exception {
22 assertEquals("-1.0 + 1.0i", a.ComplexAdd(b).toString());
23 assertEquals("1.0 + 4.0i", a.ComplexAdd(c).toString());
24 assertEquals("1.0i", b.ComplexAdd(c).toString());
25 }
26 @Test
27 public void testComplexSub() throws Exception {
28 assertEquals("1.0 + 3.0i", a.ComplexSub(b).toString());
29 assertEquals("-1.0", a.ComplexSub(c).toString());
30 assertEquals("-2.0 - 3.0i", b.ComplexSub(c).toString());
31 }
32 @Test
33 public void testComplexMulti() throws Exception {
34 assertEquals("2.0 - 2.0i", a.ComplexMulti(b).toString());
35 assertEquals("-4.0 + 2.0i", a.ComplexMulti(c).toString());
36 assertEquals("1.0 - 3.0i", b.ComplexMulti(c).toString());
37 }
38 @Test
39 public void testComplexComplexDiv() throws Exception {
40 assertEquals("-1.0 + 1.0i", a.ComplexDiv(b).toString());
41 assertEquals("0.8 - 0.4i", a.ComplexDiv(c).toString());
42 assertEquals("-0.6 - 0.2i", b.ComplexDiv(c).toString());
43 }
44 }
- 测试截图:

- 然后虚拟机里 mkdir 20175221zxj 新建文件夹以方便区分, git clone 搭档码云链接 ,将代码推到搭档码云:
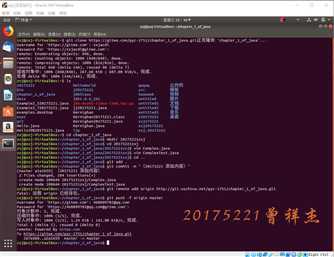
- 推送成功截图

-
第三部分:
-
要求:完成重构内容的练习,下载搭档的代码,至少进行三项重构,提交重构后代码的截图,加上自己的学号水印。提交搭档的码云项目链接。
- 我们先看看重构的概念:重构(Refactor),就是在不改变软件外部行为的基础上,改变软件内部的结构,使其更加易于阅读、易于维护和易于变更 。
- 重构中一个非常关键的前提就是“不改变软件外部行为”,它保证了我们在重构原有系统的同时,不会为原系统带来新的BUG,以确保重构的安全。
- 如何保证不改变软件外部行为?重构后的代码要能通过单元测试。
- 如何使其更加易于阅读、易于维护和易于变更 ?设计模式给出了重构的目标。
- -修改软件的四种动机:
①增加新功能;
②原有功能有BUG;
③改善原有程序的结构;
④优化原有系统的性能 。
- -需要重构的地方:
①代码重复;
②方法过长;
③参数列过长;
④条件逻辑过度复杂;
⑤分支语句。
- -一个完整的重构流程包括
①从版本控制系统代码库中Check out code;
②读懂代码(包括测试代码);
③发现bad smell;
④Refactoring;
⑤运行所有的Unit Tests;
⑥往代码库中Check in code。
- 常用使用方法:
- refactor->Rename 给类、包、方法、变量改名字
- refactor->Encapsulate Field 封装
- Source->Generate toString() toString()方法
- refactor->Extract Method 提炼出重复的代码
-
我继续以搭档的 Complex 代码为例进行重构

-
代码中存在的问题:
- 许多地方命名时未采用驼峰形式
- 类未添加创建者信息
- 类方法等未遵从javadoc规范
- 重构后代码如下:
1 /**
2 * @author 20175221 zxj
3 * @date 2019/5/1
4 */
5 @SuppressWarnings("ALL")
6 public class Complex {
7
8 double realpart;
9 double imagepart;
10
11 public Complex() {
12 }
13
14 public Complex(double r, double i) {
15 realpart = r;
16 imagepart = i;
17 }
18
19 public static double realpart(double v) {
20 return 1;
21 }
22
23 public static double imagepart(double v) {
24 return 0;
25 }
26
27 public boolean equals(Complex m) {
28 if (m.realpart == this.realpart && m.imagepart == this.imagepart) {
29 return true;
30 } else {
31 return false;
32 }
33 }
34
35 @Override
36 public String toString() {
37 if (this.realpart != 0 && this.imagepart > 0) {
38 return this.realpart + " + " + this.imagepart + "i";
39 } else if (this.realpart != 0 && this.imagepart == 0) {
40 return String.valueOf(this.realpart);
41 } else if (this.realpart != 0 && this.imagepart < 0) {
42 return this.realpart + " - " + -this.imagepart + "i";
43 } else if (this.realpart == 0 && this.imagepart != 0) {
44 return this.imagepart + "i";
45 } else {
46 return "0";
47 }
48 }
49
50 Complex complexAdd(Complex a) {
51 return new Complex(this.realpart + a.realpart, this.imagepart + a.imagepart);
52 }
53
54 Complex complexSub(Complex a) {
55 return new Complex(this.realpart - a.realpart, this.imagepart - a.imagepart);
56 }
57
58 Complex complexmulti(Complex a) {
59 return new Complex(this.realpart * a.realpart - this.imagepart * a.imagepart,
60 this.imagepart * a.realpart + this.realpart * a.imagepart);
61 }
62
63 Complex complexDiv(Complex a) {
64 return new Complex((this.imagepart * a.imagepart + this.realpart * a.realpart) / (a.imagepart * a.imagepart + a.realpart * a.realpart),
65 (this.realpart * a.imagepart - this.imagepart * a.realpart) / (a.imagepart * a.imagepart + a.realpart * a.realpart));
66 }
67 }
以上是关于20175221曾祥杰 实验三《敏捷开发与XP实践》的主要内容,如果未能解决你的问题,请参考以下文章
实验三 敏捷开发与XP实践
实验三 敏捷开发与XP实践
20175312 2018-2019-2 实验三 敏捷开发与XP实践 实验报告
2017-2018-2 20165331 实验三《敏捷开发与XP实践》实验报告
20175325 《JAVA程序设计》实验三《敏捷开发与XP实践》实验报告
2017-2018-2 20165327 实验三《敏捷开发与XP实践》实验报告