新手学习使用Java,尝试着做一个项目使用Java做一个视频图像的处理。
Posted
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了新手学习使用Java,尝试着做一个项目使用Java做一个视频图像的处理。相关的知识,希望对你有一定的参考价值。
读取视频然后对每帧图像做检测处理,想问Java怎么实现视频的读取和图片的输出?我的Java版本是jdk1.8.0_91,试着用ImageIO时无法引用又该怎么解决,求帮忙?
Java图像处理技巧四则下面代码中用到的sourceImage是一个已经存在的Image对象
图像剪切
对于一个已经存在的Image对象,要得到它的一个局部图像,可以使用下面的步骤:
//import java.awt.*;
//import java.awt.image.*;
Image croppedImage;
ImageFilter cropFilter;
CropFilter =new CropImageFilter(25,30,75,75); //四个参数分别为图像起点坐标和宽高,即CropImageFilter(int x,int y,int width,int height),详细情况请参考API
CroppedImage= Toolkit.getDefaultToolkit().createImage(new FilteredImageSource(sourceImage.getSource(),cropFilter));
如果是在Component的子类中使用,可以将上面的Toolkit.getDefaultToolkit().去掉。FilteredImageSource是一个ImageProducer对象。
图像缩放
对于一个已经存在的Image对象,得到它的一个缩放的Image对象可以使用Image的getScaledInstance方法:
Image scaledImage=sourceImage. getScaledInstance(100,100, Image.SCALE_DEFAULT); //得到一个100X100的图像
Image doubledImage=sourceImage. getScaledInstance(sourceImage.getWidth(this)*2,sourceImage.getHeight(this)*2, Image.SCALE_DEFAULT); //得到一个放大两倍的图像,这个程序一般在一个swing的组件中使用,而类Jcomponent实现了图像观察者接口ImageObserver,所有可以使用this。
//其它情况请参考API
灰度变换
下面的程序使用三种方法对一个彩色图像进行灰度变换,变换的效果都不一样。一般而言,灰度变换的算法是将象素的三个颜色分量使用R*0.3+G*0.59+ B*0.11得到灰度值,然后将之赋值给红绿蓝,这样颜色取得的效果就是灰度的。另一种就是取红绿蓝三色中的最大值作为灰度值。java核心包也有一种算法,但是没有看源代码,不知道具体算法是什么样的,效果和上述不同。
/* GrayFilter.java*/
/*@author:cherami */
/*email:cherami@163.net*/
import java.awt.image.*;
public class GrayFilter extends RGBImageFilter
int modelStyle;
public GrayFilter()
modelStyle=GrayModel.CS_MAX;
canFilterIndexColorModel=true;
public GrayFilter(int style)
modelStyle=style;
canFilterIndexColorModel=true;
public void setColorModel(ColorModel cm)
if (modelStyle==GrayModel
else if (modelStyle==GrayModel
public int filterRGB(int x,int y,int pixel)
return pixel;
/* GrayModel.java*/
/*@author:cherami */
/*email:cherami@163.net*/
import java.awt.image.*;
public class GrayModel extends ColorModel
public static final int CS_MAX=0;
public static final int CS_FLOAT=1;
ColorModel sourceModel;
int modelStyle;
public GrayModel(ColorModel sourceModel)
super(sourceModel.getPixelSize());
this.sourceModel=sourceModel;
modelStyle=0;
public GrayModel(ColorModel sourceModel,int style)
super(sourceModel.getPixelSize());
this.sourceModel=sourceModel;
modelStyle=style;
public void setGrayStyle(int style)
modelStyle=style;
protected int getGrayLevel(int pixel)
if (modelStyle==CS_MAX)
return Math.max(sourceModel.getRed(pixel),Math.max(sourceModel.getGreen(pixel),sourceModel.getBlue(pixel)));
else if (modelStyle==CS_FLOAT)
return (int)(sourceModel.getRed(pixel)*0.3+sourceModel.getGreen(pixel)*0.59+sourceModel.getBlue(pixel)*0.11);
else
return 0;
public int getAlpha(int pixel)
return sourceModel.getAlpha(pixel);
public int getRed(int pixel)
return getGrayLevel(pixel);
public int getGreen(int pixel)
return getGrayLevel(pixel);
public int getBlue(int pixel)
return getGrayLevel(pixel);
public int getRGB(int pixel)
int gray=getGrayLevel(pixel);
return (getAlpha(pixel)<<24)+(gray<<16)+(gray<<8)+gray;
如果你有自己的算法或者想取得特殊的效果,你可以修改类GrayModel的方法getGrayLevel()。
色彩变换
根据上面的原理,我们也可以实现色彩变换,这样的效果就很多了。下面是一个反转变换的例子:
/* ReverseColorModel.java*/
/*@author:cherami */
/*email:cherami@163.net*/
import java.awt.image.*;
public class ReverseColorModel extends ColorModel
ColorModel sourceModel;
public ReverseColorModel(ColorModel sourceModel)
super(sourceModel.getPixelSize());
this.sourceModel=sourceModel;
public int getAlpha(int pixel)
return sourceModel.getAlpha(pixel);
public int getRed(int pixel)
return ~sourceModel.getRed(pixel);
public int getGreen(int pixel)
return ~sourceModel.getGreen(pixel);
public int getBlue(int pixel)
return ~sourceModel.getBlue(pixel);
public int getRGB(int pixel)
return (getAlpha(pixel)<<24)+(getRed(pixel)<<16)+(getGreen(pixel)<<8)+getBlue(pixel);
/* ReverseColorModel.java*/
/*@author:cherami */
/*email:cherami@163.net*/
import java.awt.image.*;
public class ReverseFilter extends RGBImageFilter
public ReverseFilter()
canFilterIndexColorModel=true;
public void setColorModel(ColorModel cm)
substituteColorModel(cm,new ReverseColorModel(cm));
public int filterRGB(int x,int y,int pixel)
return pixel;
要想取得自己的效果,需要修改ReverseColorModel.java中的三个方法,getRed、getGreen、getBlue。
下面是上面的效果的一个总的演示程序。
/*GrayImage.java*/
/*@author:cherami */
/*email:cherami@163.net*/
import java.awt.*;
import java.awt.image.*;
import javax.swing.*;
import java.awt.color.*;
public class GrayImage extends JFrame
Image source,gray,gray3,clip,bigimg;
BufferedImage bimg,gray2;
GrayFilter filter,filter2;
ImageIcon ii;
ImageFilter cropFilter;
int iw,ih;
public GrayImage()
ii=new ImageIcon(\"images/11.gif\");
source=ii.getImage();
iw=source.getWidth(this);
ih=source.getHeight(this);
filter=new GrayFilter();
filter2=new GrayFilter(GrayModel.CS_FLOAT);
gray=createImage(new FilteredImageSource(source.getSource(),filter));
gray3=createImage(new FilteredImageSource(source.getSource(),filter2));
cropFilter=new CropImageFilter(5,5,iw-5,ih-5);
clip=createImage(new FilteredImageSource(source.getSource(),cropFilter));
bigimg=source.getScaledInstance(iw*2,ih*2,Image.SCALE_DEFAULT);
MediaTracker mt=new MediaTracker(this);
mt.addImage(gray,0);
try
mt.waitForAll();
catch (Exception e)
参考技术A 我个人比较喜欢杜老师的教学视频不错,正在B站上看mysql学习数据库这块儿,零基础可以看看他最新版本的Java教程,一共316集。动力节点的java基础教程159集,20年最新升级为全新的java基础316集,可以通过蛙课网获取哦
那是学的不精,天天动脑,可以到学习下,掌握下,补缺补漏,这样就会减轻大脑负担的 参考技术B ffmpeg 必须了,多媒体处理的好选择,建议多看看经典的视频教程,比如java301集。 参考技术C 旧版的杜老师的视频教程是17年的所用的是jdk8。而最新的视频教程是今年的用的是jdk13。而且视频中的知识点都是最新的,你可以去B站上了解一下。动力节点的java基础教程159集,20年最新升级为全新的java基础316集,可以通过蛙课网获取哦
价格都是国家规定的,你可以到了解下,目前优惠很多,你要读,现在去看看呀
Maven学习笔记-使用Eclipse插件创建Java Webapp项目
使用 Java 的猿们,最多接触的大概就是 web 项目了吧?在学习 Maven 的过程中,首先当然就是创建一个 webapp 项目。
自己在尝试的过程中,碰到了很多的问题。我把详细的过程都记录下来,以便今后参考。
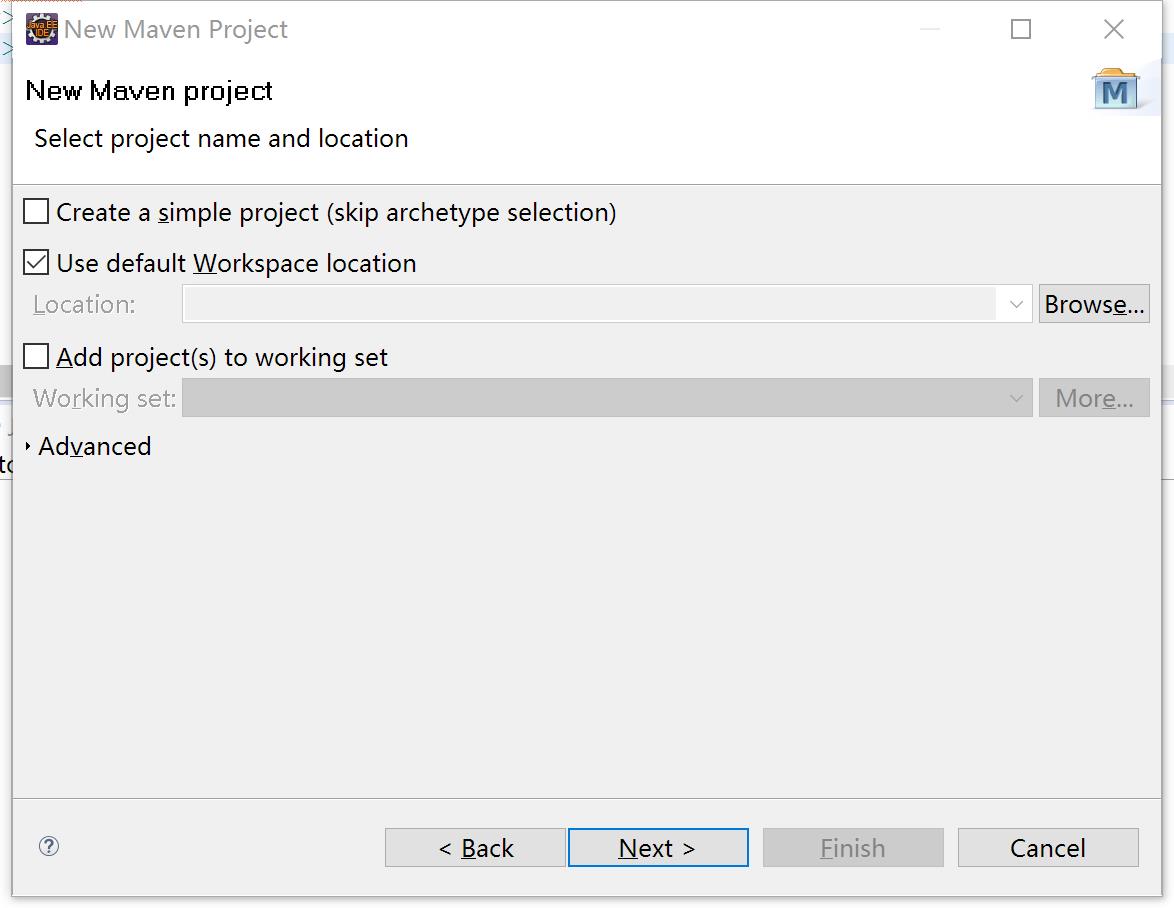
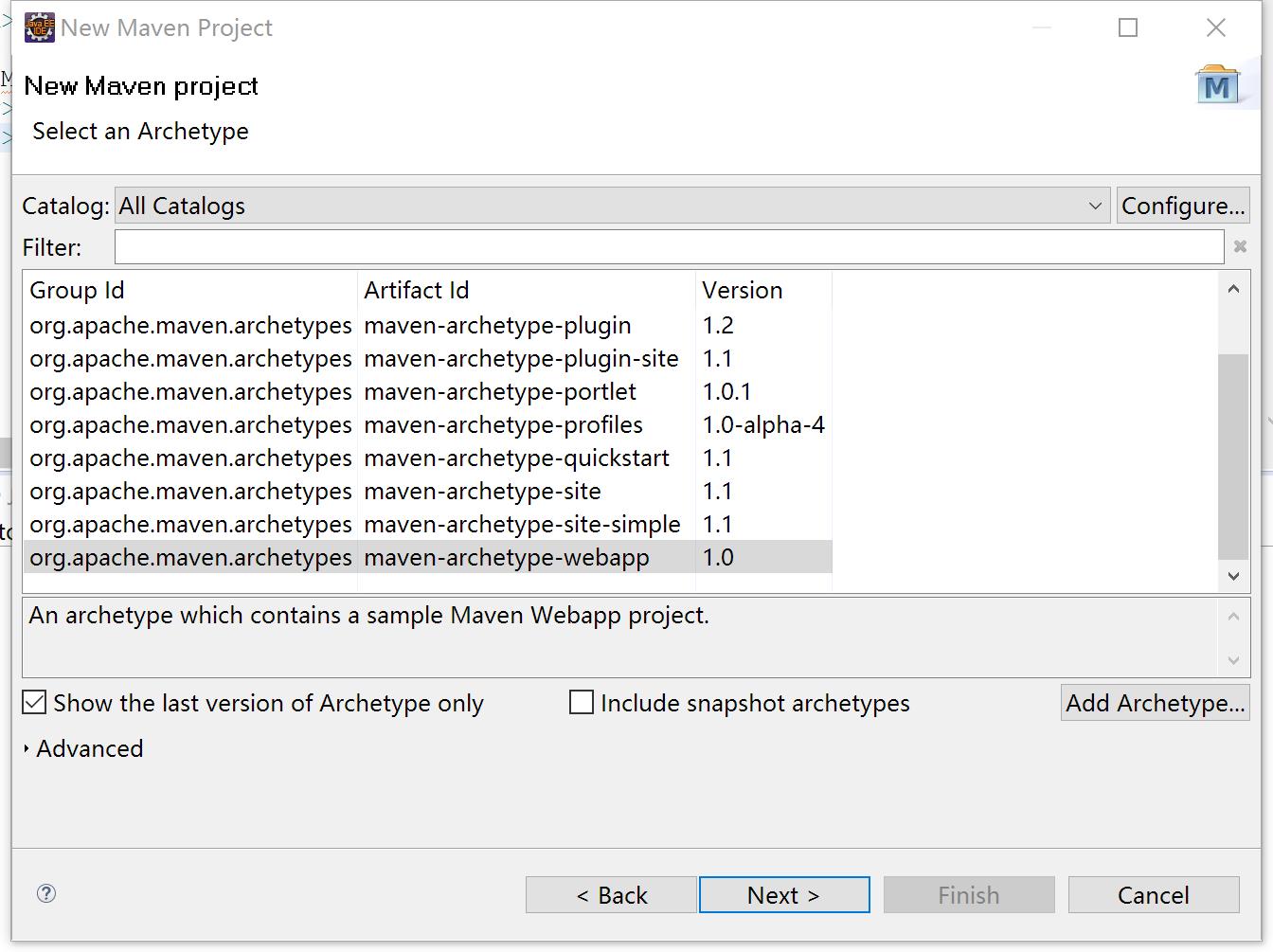
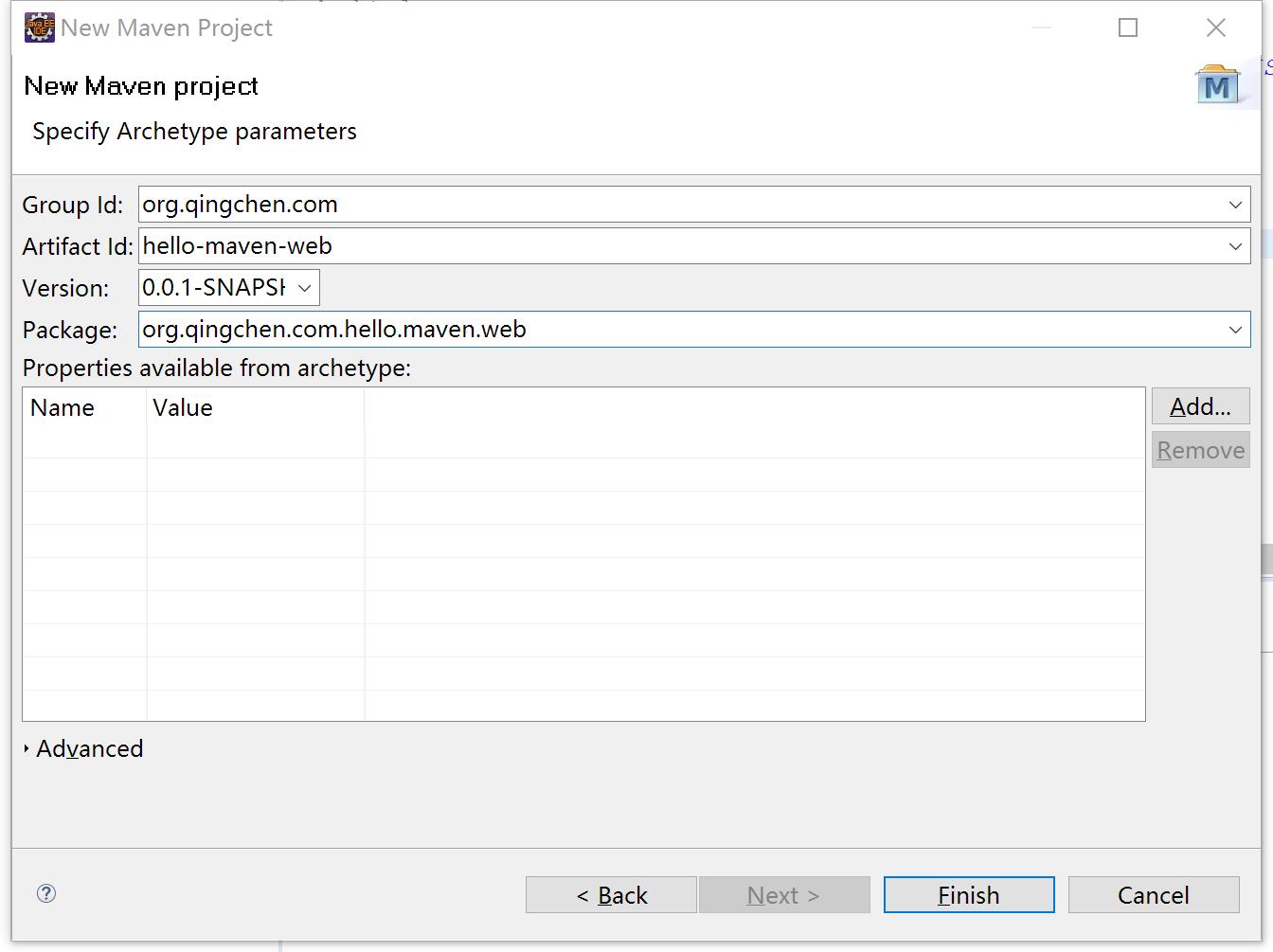
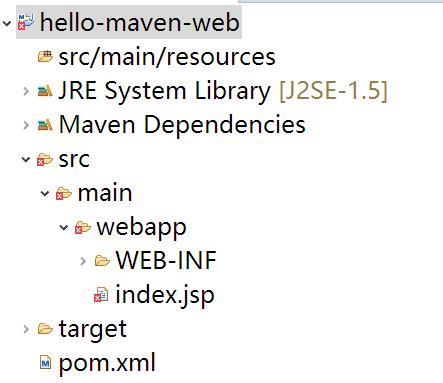
<build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>2.1</version> <configuration> <source>1.7</source> <target>1.7</target> </configuration> </plugin> </plugins> </build>
(也可以通过修改 settings.xml 全局配置以让 Maven 默认设置特定版本的 JRE,具体方法这里先不写了)
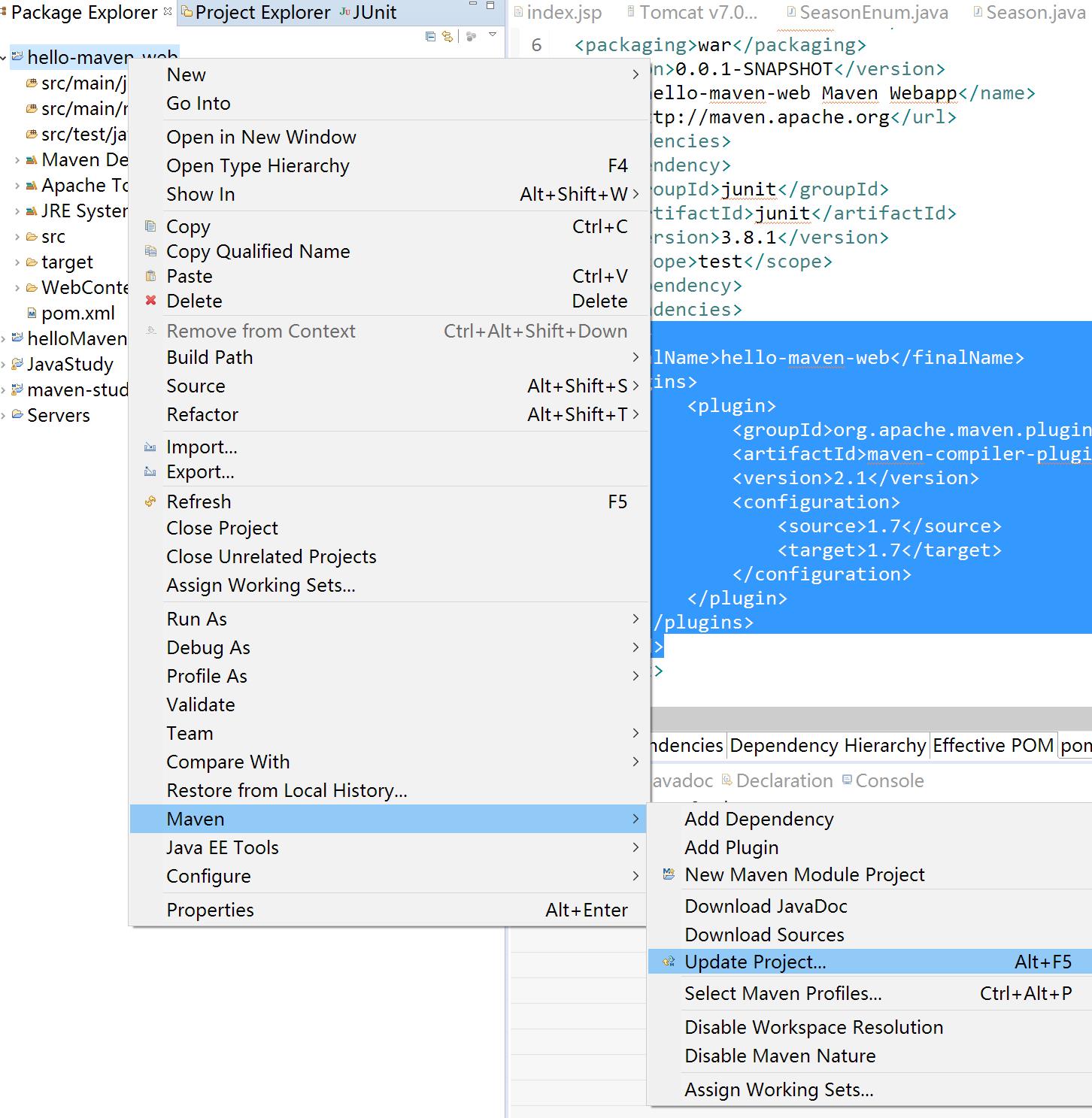

这时只要将 Libraries 标签中的 JRE System Library 设置为本机默认的就可以解决该错误(不知道为什么会这样)
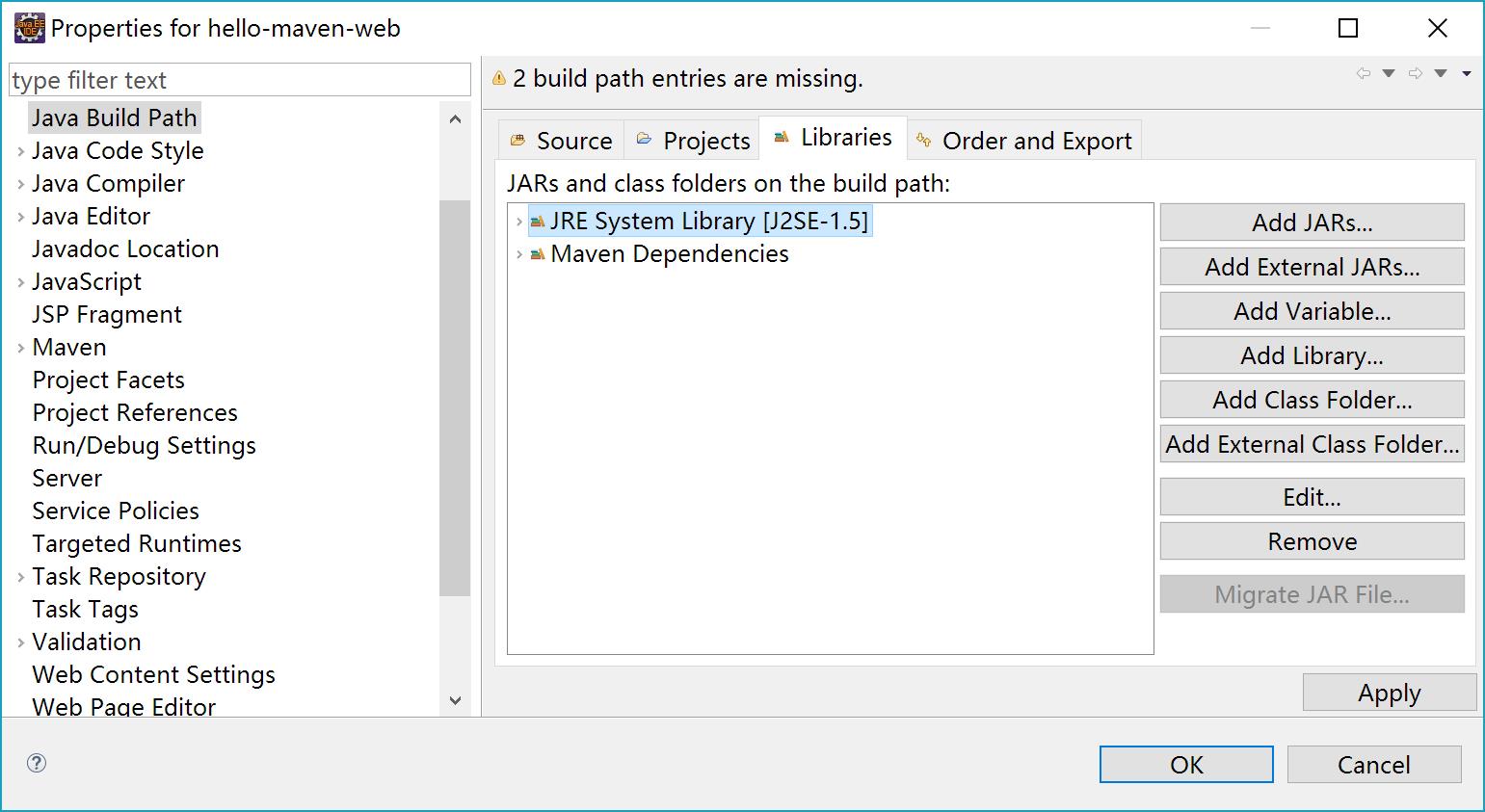
双击 JRE System Library 项,弹出 JRE 设置界面:↓↓↓
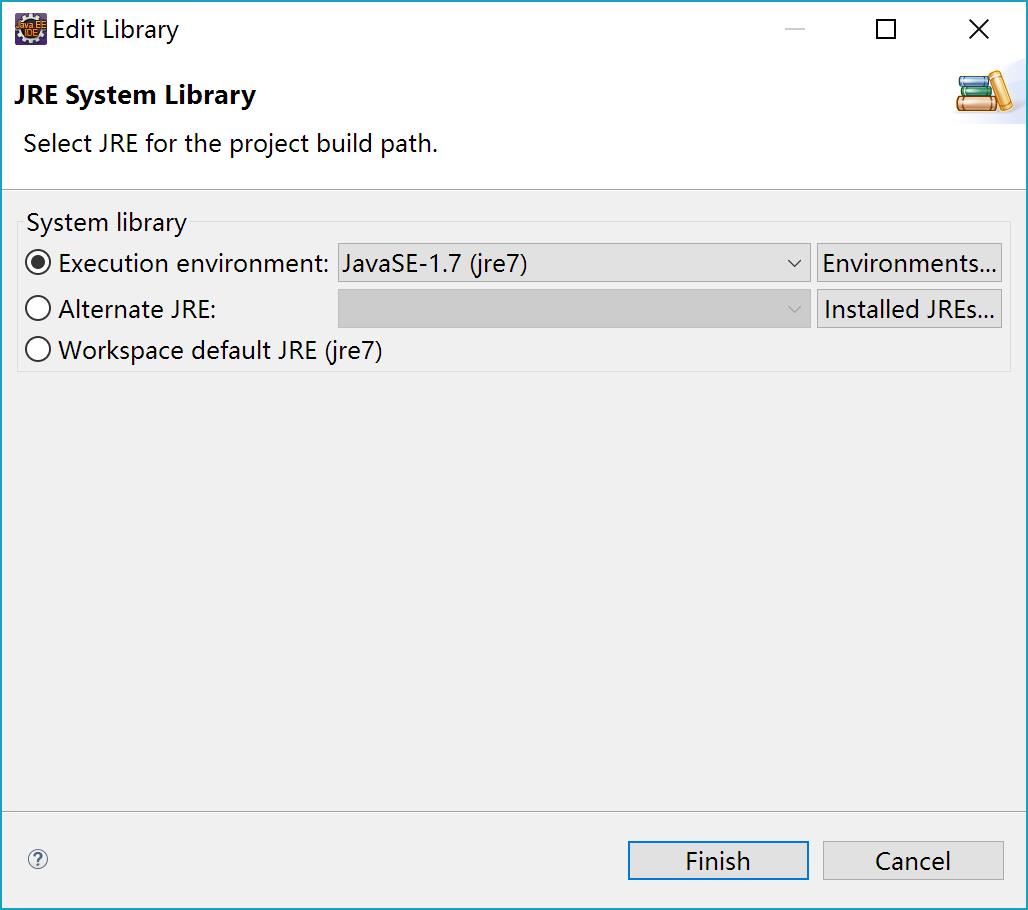
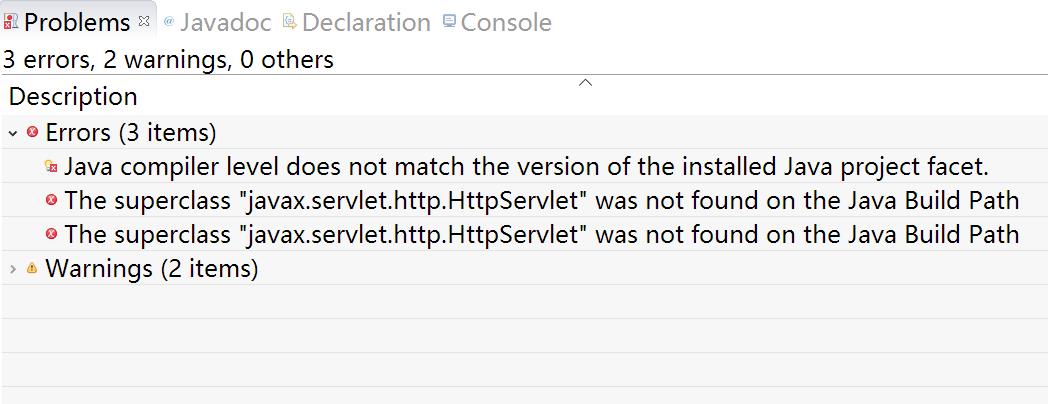
The superclass "javax.servlet.http.HttpServlet" was not found on the Java Build Path
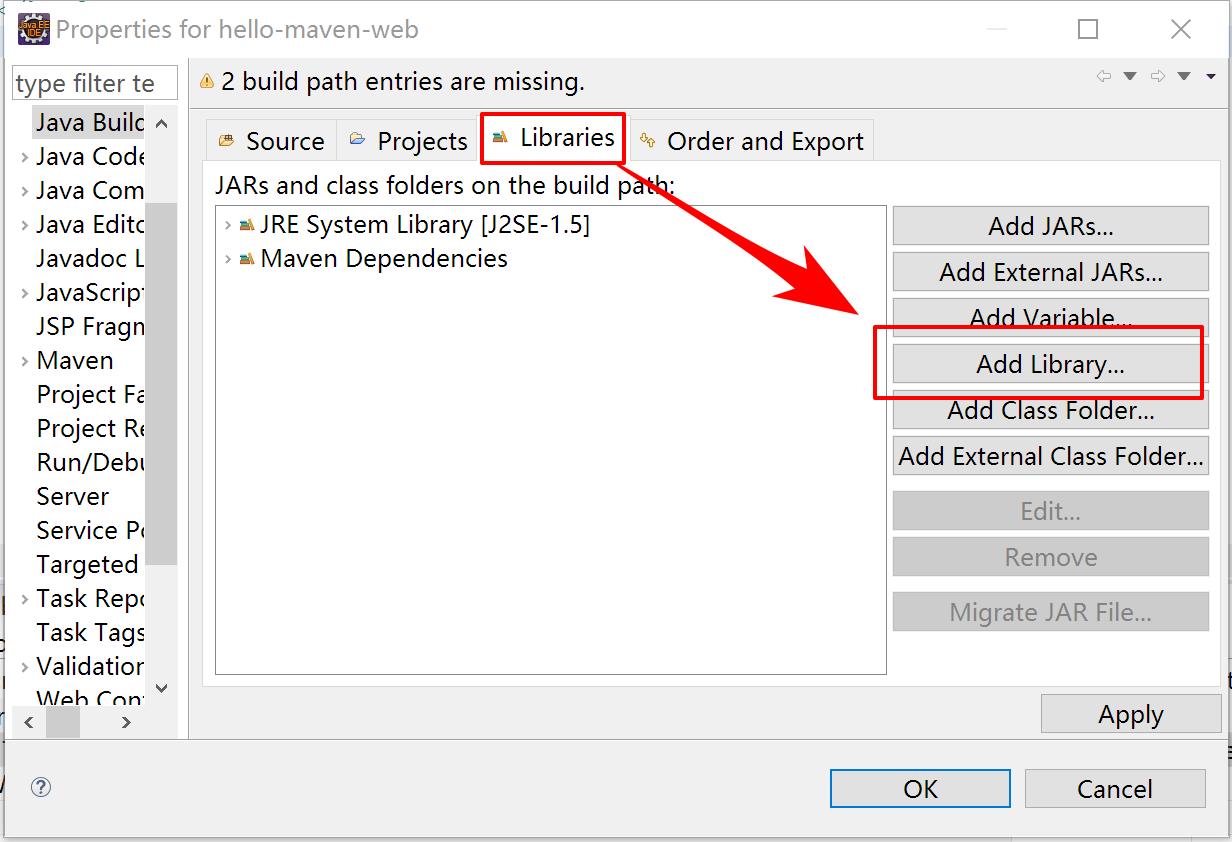
在弹出的对话框中,选择“Server Runtime”。
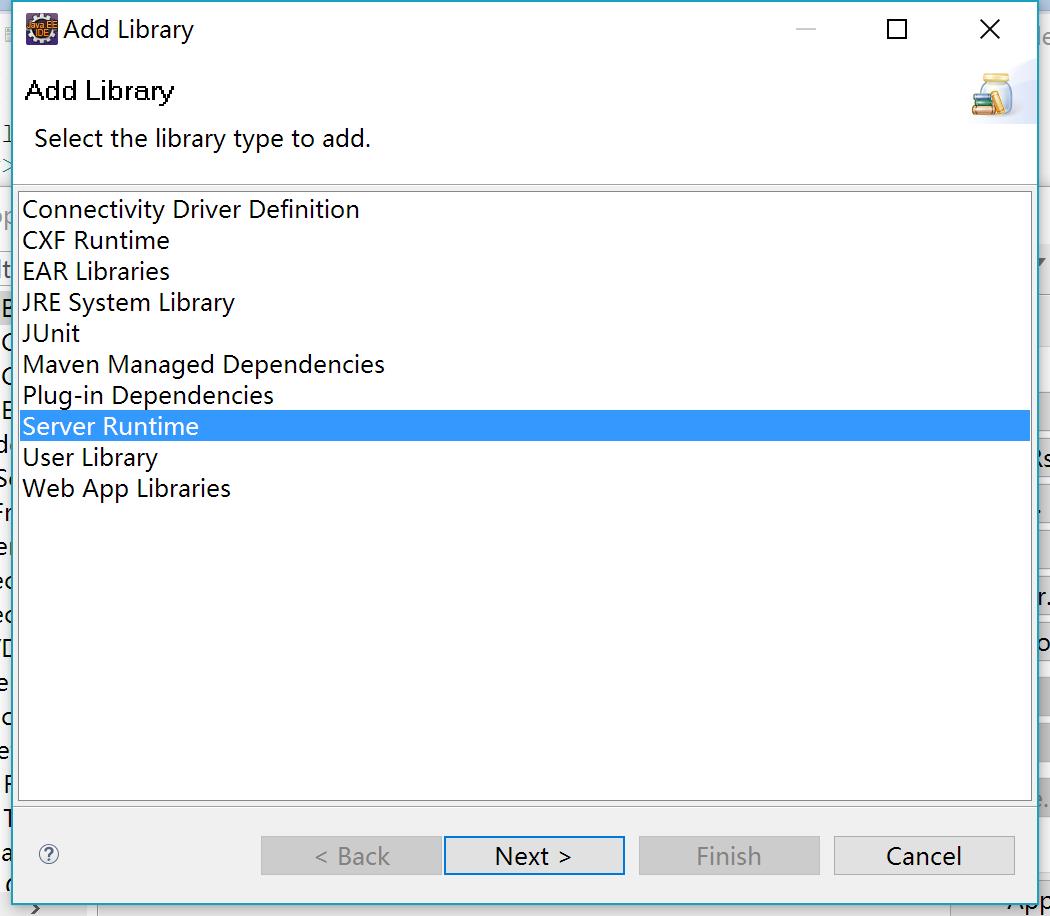
选择 Tomcat 服务器。如果这里没有服务器配置,请事先配置一个 Tomcat 等服务器。
(不知道如何配置服务器的话,就用搜索引擎搜一番)。
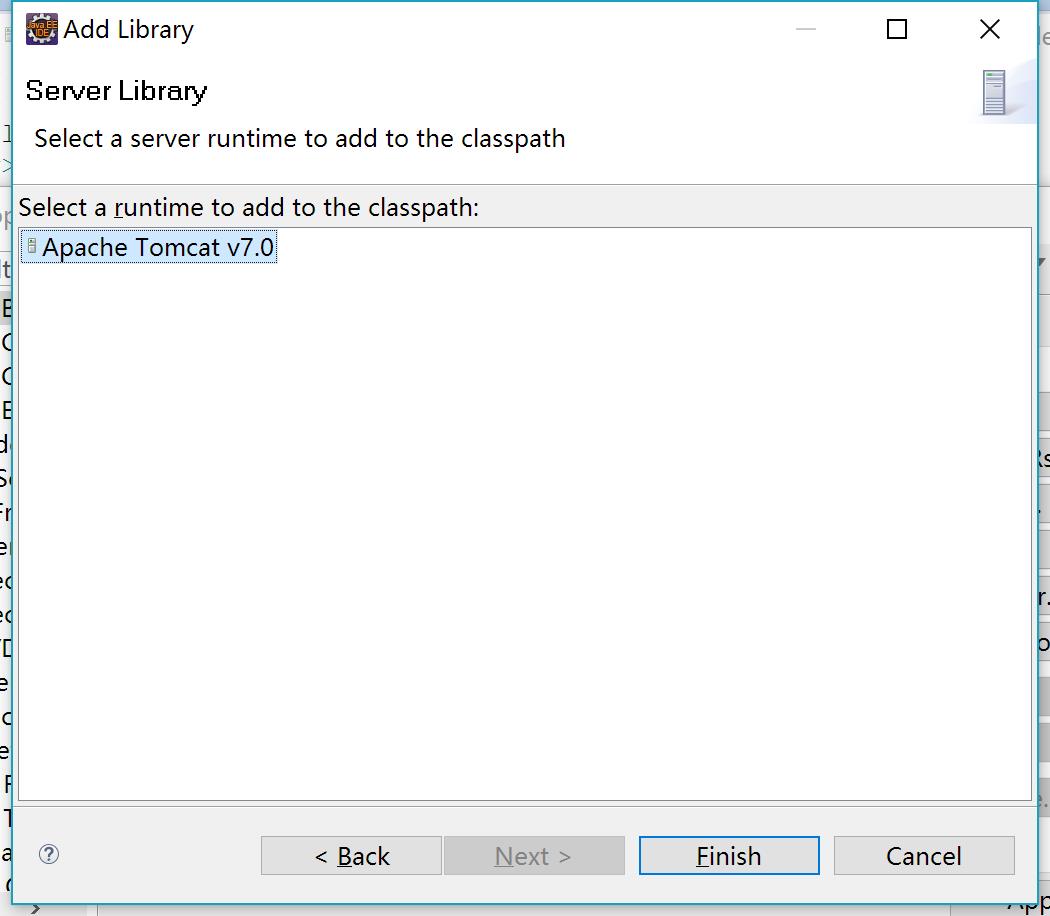
选中 Tomcat,点击 Finish 后,看到工程已经不再有那两个 Servlet 的报错了。
Java compiler level does not match the version of the installed java project facet.
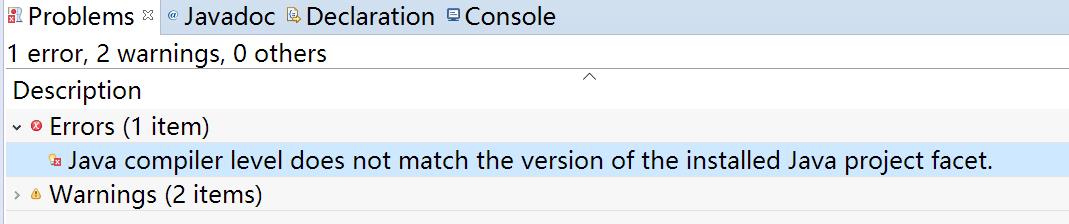
我们需要通过修改 Project Facets 来解决这个错误。
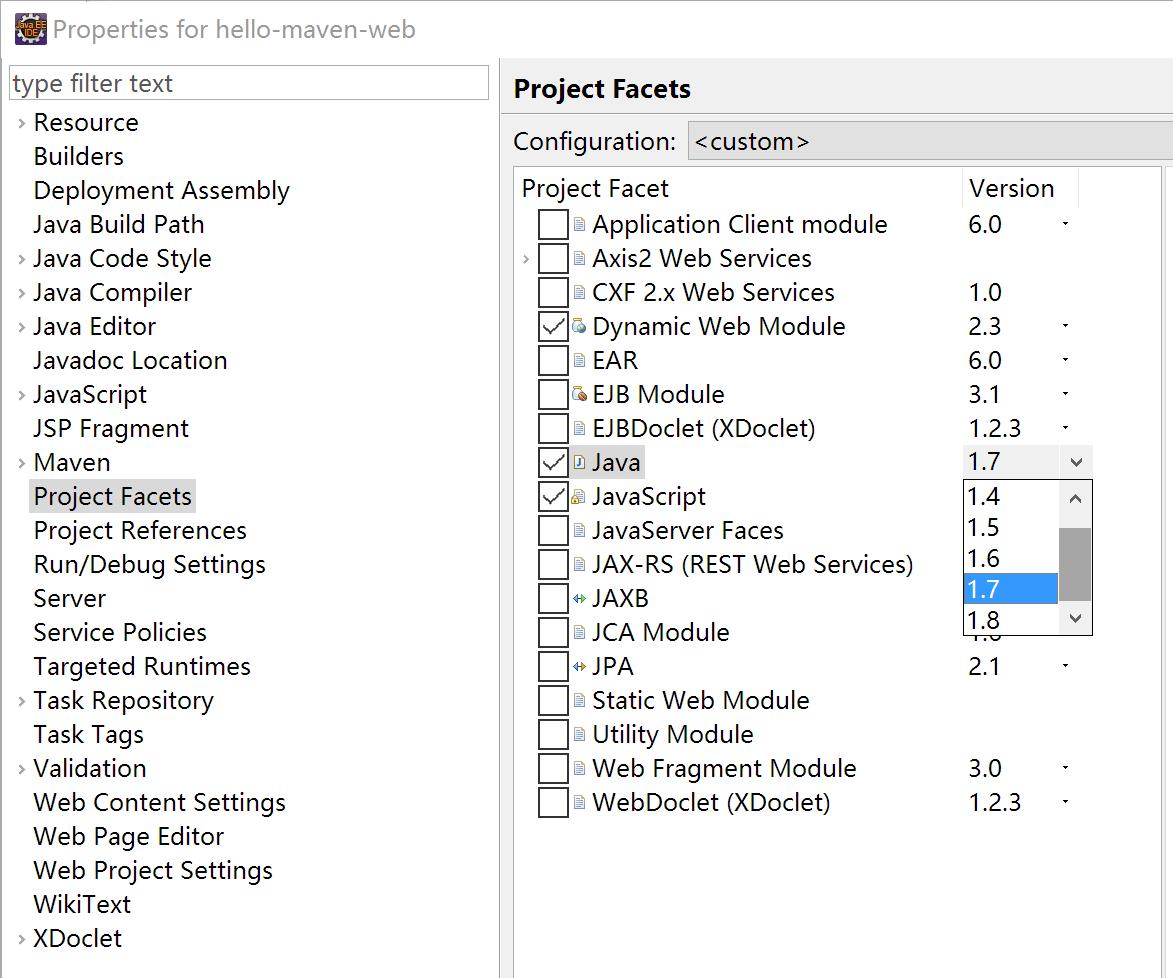
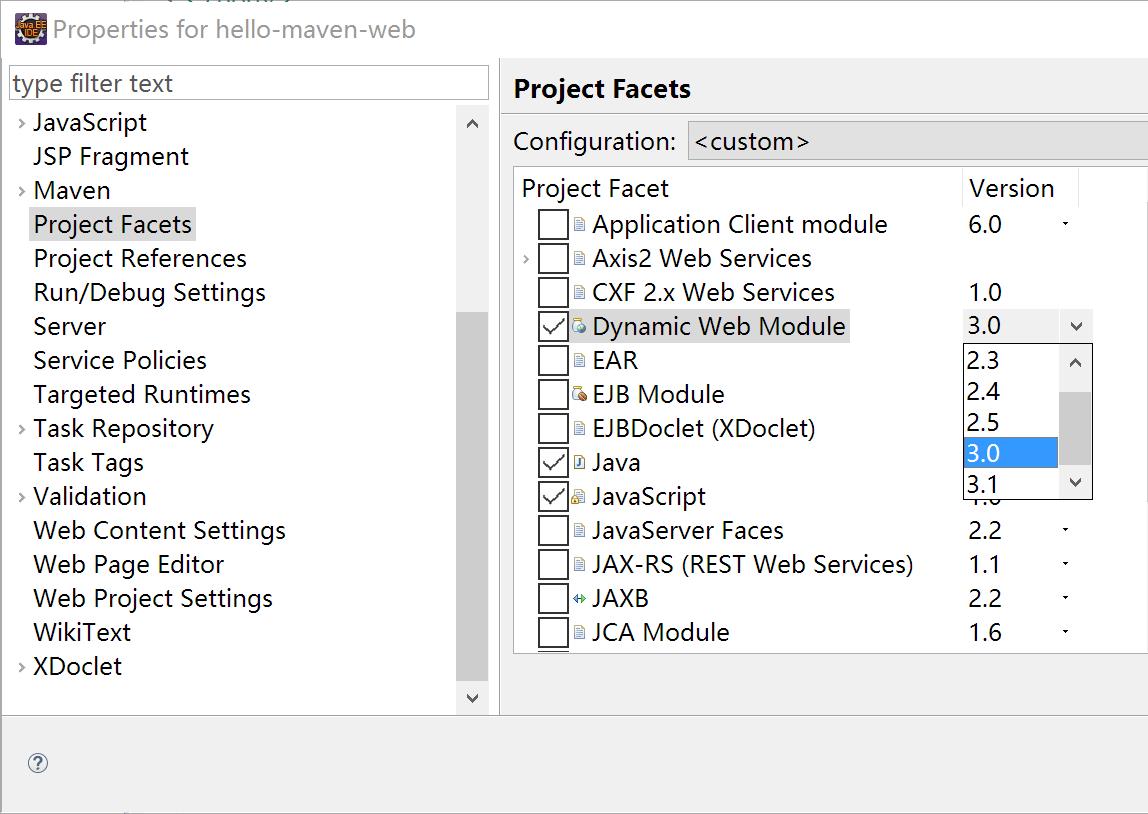
Cannot change version of project facet Dynamic Web Module to 3.0
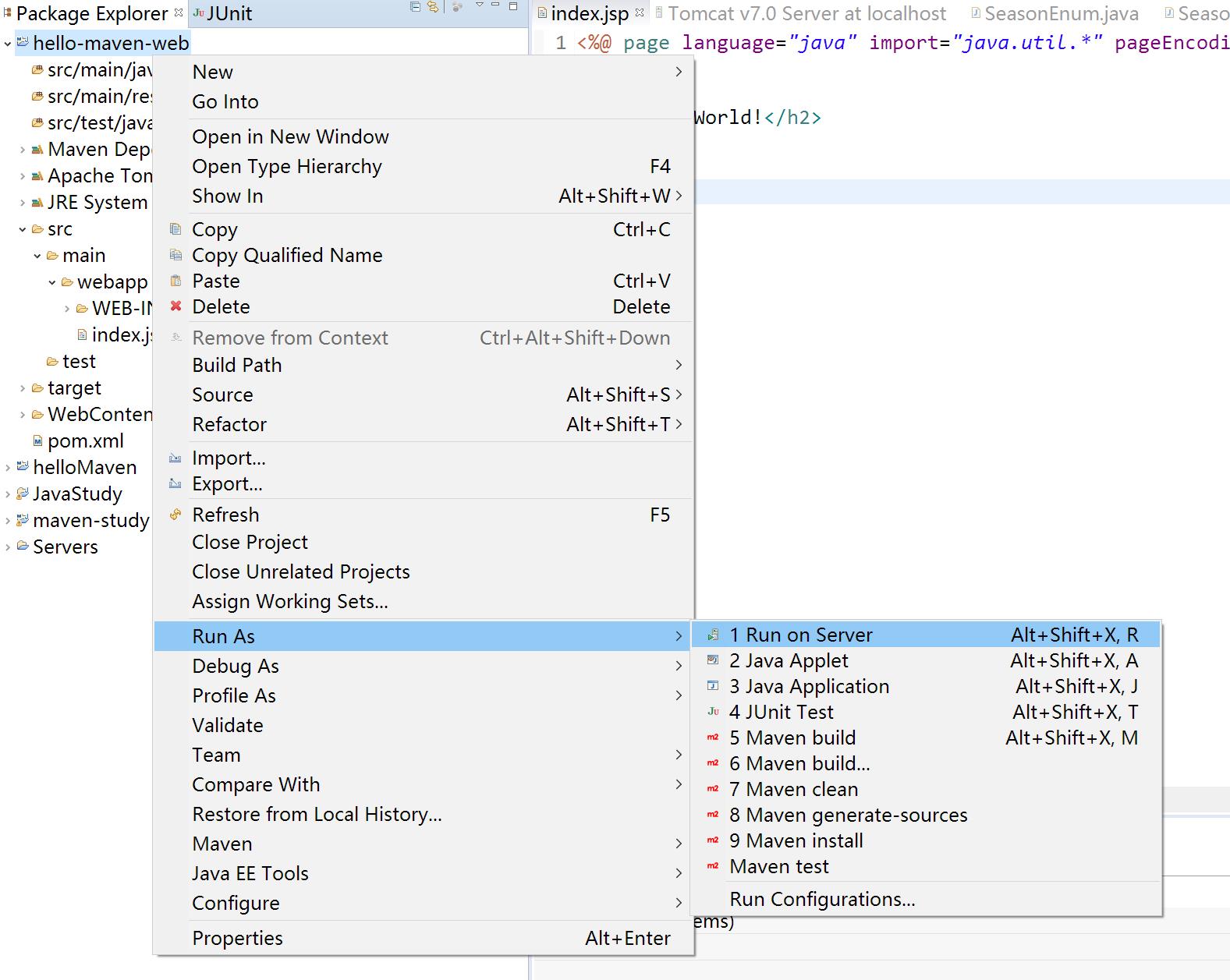
这时项目应该已经配置到服务器中了:
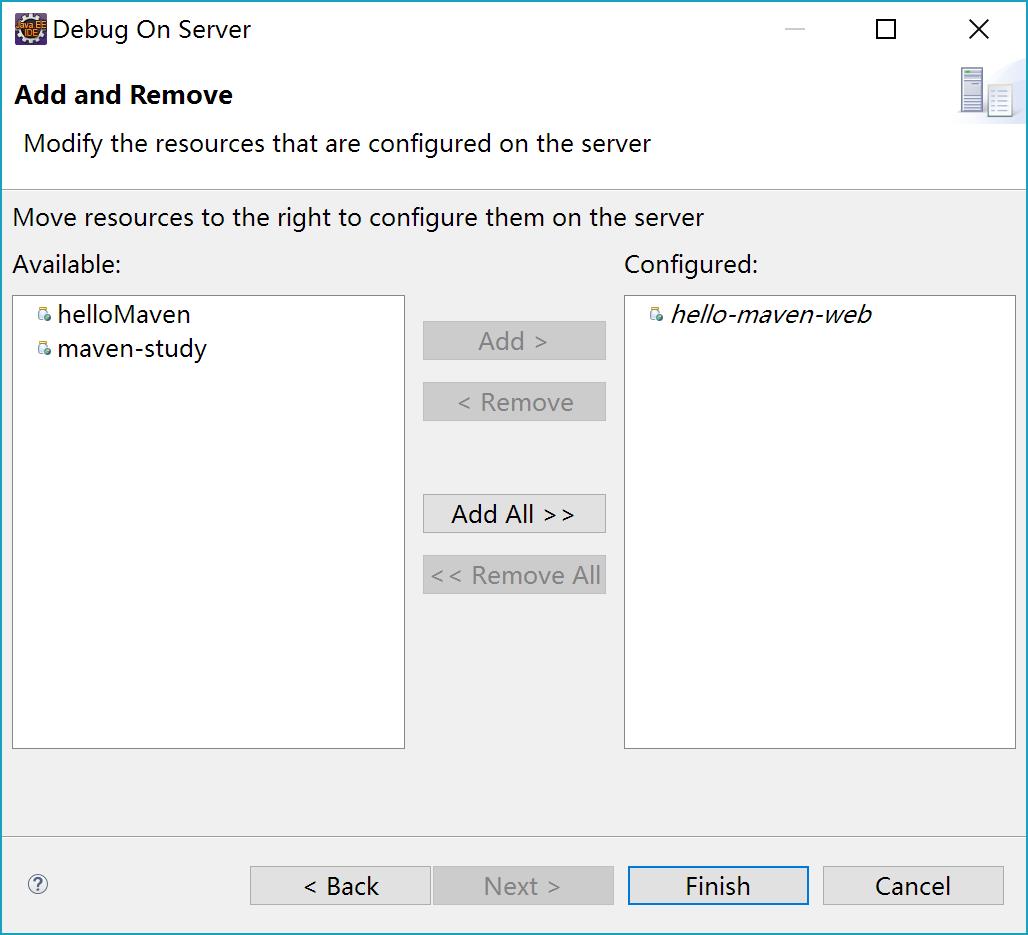
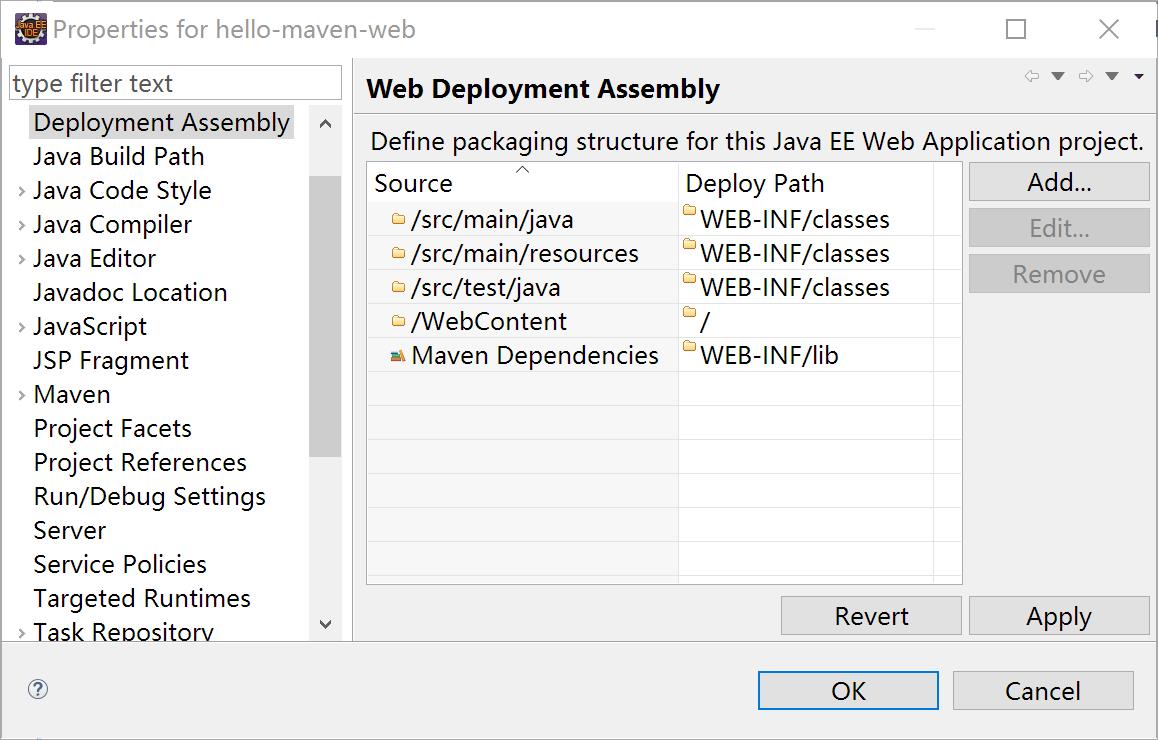
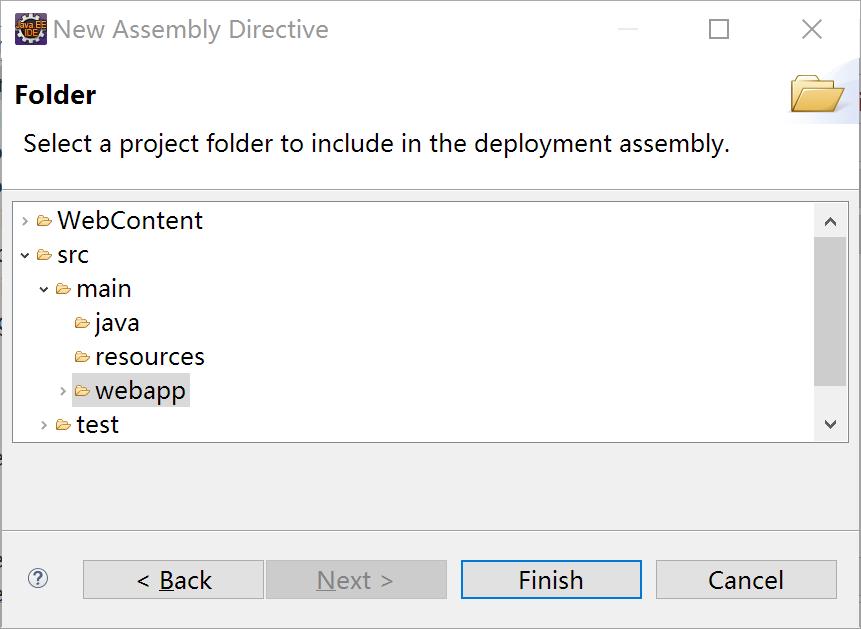
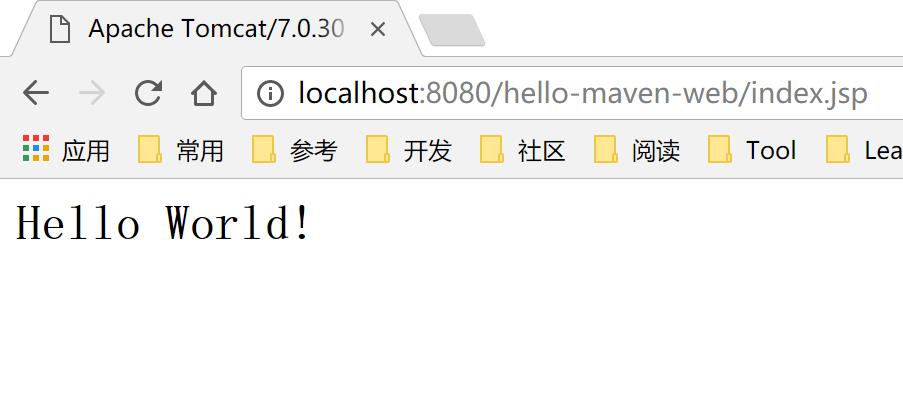
最后,我也不知道为什么,似乎很简单的事情,会变得如此复杂。
以上是关于新手学习使用Java,尝试着做一个项目使用Java做一个视频图像的处理。的主要内容,如果未能解决你的问题,请参考以下文章