Spring boot crud 和 swagger使用
Posted xiaoduup
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了Spring boot crud 和 swagger使用相关的知识,希望对你有一定的参考价值。
文章目录
spring boot swagger
上一节 Spring boot logging
源码地址
https://gitee.com/mayun_xiaodu/spring-boot-all
正文
swagger快速上手
- spring boot 集成
1. pom依赖
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
2. swagger配置类
@Configuration
@EnableSwagger2
public class Swagger2Config
@Bean
public Docket docket()
return new Docket(DocumentationType.SWAGGER_2)
.enable(true)
.apiInfo(new ApiInfoBuilder()
.description("swagger-test")
.version("1.0")
.title("swagger-title")
.contact(new Contact("xiaodu", "http://www.baidu.com", "xiaoduup@aliyun.com"))
.build())
.select()
.apis(RequestHandlerSelectors.basePackage("com.xiaodu.swagger"))
.paths(PathSelectors.any())
.build();
@EnableSwagger2 开启swageer
DocumentationType.SWAGGER_2 指定使用swagger2,
description api文档描述
version 版本
title 文档标题
contact 作者联系信息
basePackage 扫描使用了swagger的包;
swagger 注解doc描述使用
swagger 常用注解描述
## controller 层使用
@Api: 标注在controller 类上;表示对整个类对应的资源文档说明
(属性)
- String value 已经废弃,推荐使用 tags
- String[] tags api文档标签,对应ui上的资源名称,默认会使用类名
- String produces 服务器返回的mediaType
- String consumes 服务器接收的mediaType
- boolean hidden 是否隐藏资源
@ApiOperation:标注在请求方法上,表示对请求方法的
- String value 对请求的简单描述
- String notes 对请求的详细描述
- String[] tags 对应@Api的tags ;属于那个 @api
- Class<?> response 响应类型
- httpMethod 请求方法 GET", "HEAD", "POST", "PUT", "DELETE", "OPTIONS" and "PATCH".
- produces 服务器返回的mediaType
- consumes 服务器接收的mediaType
- boolean hidden 是否隐藏资源
@ApiParam
- name 参数名称
- value 参数描述
- defaultValue 默认值
- allowableValues 参数允许的值
- required 参数是否必需
- example 示例
- type 参数类型
- allowEmptyValue 是否允许空值
-
@ApiResponses : 返回可能的一组响应
@ApiResponse
- code 响应code码
- message 响应描述信息
- response 响应类型
- responseHeaders 响应头
@ApiImplicitParams: 一组对请求参数的描述
@ApiImplicitParam :对请求参数的描述;当请求参数不是一个可描述的时候使用 同@ApiParam 作用一样
- name 参数名称
- value 参数描述
- dateTypeClass 参数类型
- paramType 参数的参数类型的位置; 可选值 path, query, body, header,
- type 参数类型
## 实体bean使用
@ApiModel
- value 模型的备用名称
- description 模型的详细描述
@ApiModelProperty
- value 对属性的简要描述
- name 文档中属性的名称
- required 是否必需
- example 属性值的示例
(以上只是简单对常用的注解和属性进行了描述,代码中有使用示例)
1. 使用swagger对实体bean描述
@ApiModel(description = "订单实体bean")
public class OrderEntity
@ApiModelProperty(value = "订单编号")
private String id;
@ApiModelProperty(value = "订单名称")
private String name;
@ApiModelProperty(value = "订单创建时间")
private Date createTime;
...
- @ApiModel标注在实体类上进行描述
- @ApiModelProperty 对字段进行描述
2. 使用swagger对请求api描述
以下是一个完整的 crud 操作的controller; 并且添加了swagger文档描述
@RestController
@RequestMapping(value = "/test")
@Api(tags = "test-订单操作") // 标注为swagger的一个资源
public class OrderController
private static final Logger log = LoggerFactory.getLogger(OrderController.class);
// get 请求
@ApiOperation(value = "订单查询", notes = "根据orderId查询订单信息", httpMethod = "GET") // 对请求的表述
@ApiResponses( // 对可能返回的结果response描述
@ApiResponse(code = 200, message = "ok"),
@ApiResponse(code = 500, message = "error")
)
@GetMapping("/order")
public ResultBean<OrderEntity> getName(
@ApiParam(name = "orderId", value = "订单编号", required = true) @RequestParam String orderId) throws Exception
OrderEntity orderEntity = new OrderEntity();
orderEntity.setId(orderId);
orderEntity.setCreateTime(new Date());
orderEntity.setName("xiaomi 11");
return ResultBean.success(orderEntity);
// post 请求
@ApiOperation(value = "添加订单", httpMethod = "POST")
@PostMapping("/order")
public ResultBean<Void> addOrder(@RequestBody OrderEntity orderEntity) throws Exception
log.warn("添加 order = ", orderEntity);
return ResultBean.success(null);
// put 请求
@ApiOperation(value = "更新订单", notes = "根据orderId更新订单信息并返回更新后的订单信息", httpMethod = "PUT")
@PutMapping("/order")
public ResultBean<OrderEntity> updateOrder(@RequestBody OrderEntity orderEntity) throws Exception
log.warn("update order = ", orderEntity);
return ResultBean.success(orderEntity);
// delete 请求
@ApiOperation(value = "删除订单", httpMethod = "DELETE")
@DeleteMapping("order/orderId")
public ResultBean<Void> delOrder(@ApiParam(value = "订单编号")@PathVariable String orderId)
log.warn("delete orderId = ", orderId);
return ResultBean.success(null);
//get 请求; ApiImplicitParam 使用场景
@ApiOperation(value = "test-ApiImplicitParam", httpMethod = "GET", response = String.class)
@ApiImplicitParams( // 对请求参数的描述;当请求参数不是一个可描述的时候使用 同@ApiParam 作用一样
@ApiImplicitParam(name = "name", value = "名称", dataTypeClass = String.class, paramType = "query"),
@ApiImplicitParam(name = "age", value = "年龄", dataTypeClass = Integer.class, paramType = "query")
)
@GetMapping(value = "/str", consumes = MediaType.ALL_VALUE)
public String getStr(HttpServletRequest request, HttpServletResponse response) throws Exception
return "name = " + request.getParameter("name") + ", age = " + request.getParameter("age");
API描述文档
启动项目后;访问localhost:8080/swagger-ui.html 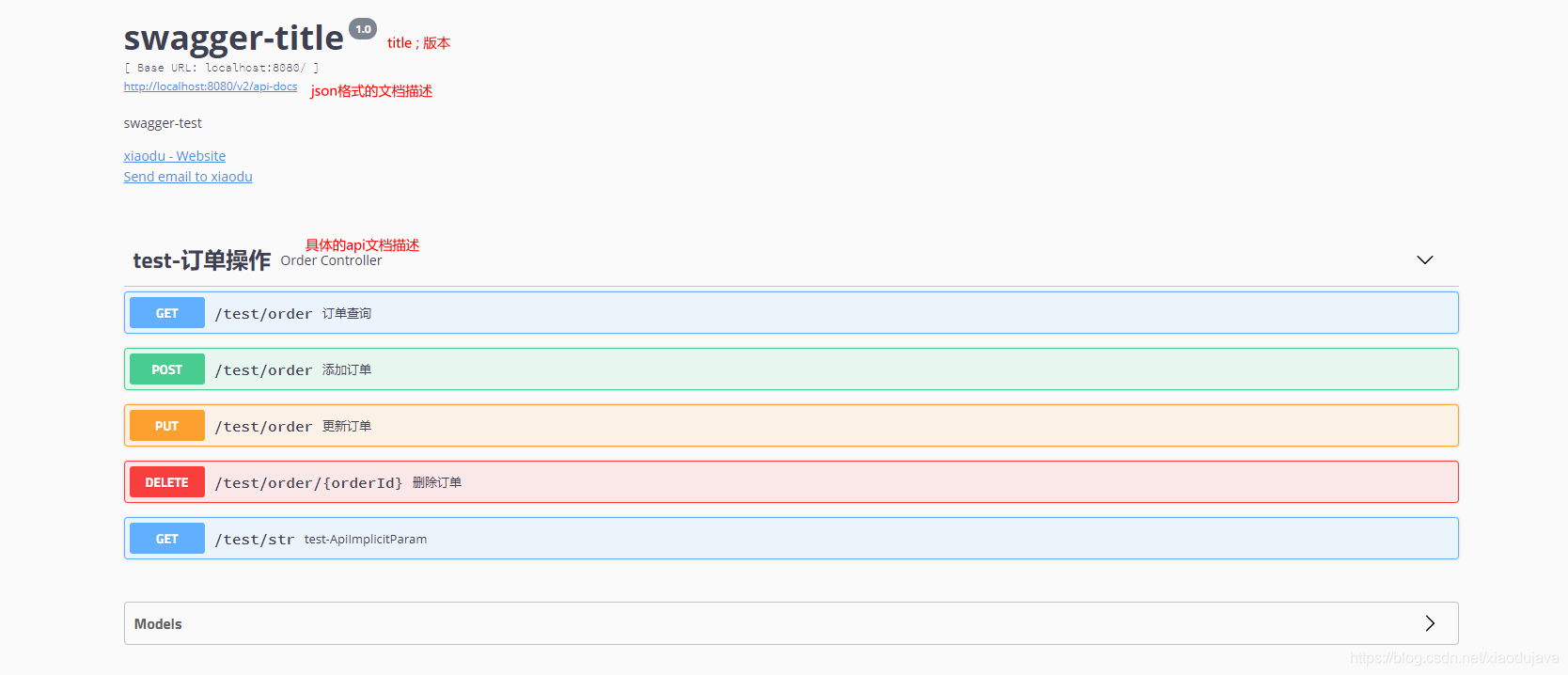 随便点开一个 文档即可看到详细的文档描述; 可以进行模拟http请求; 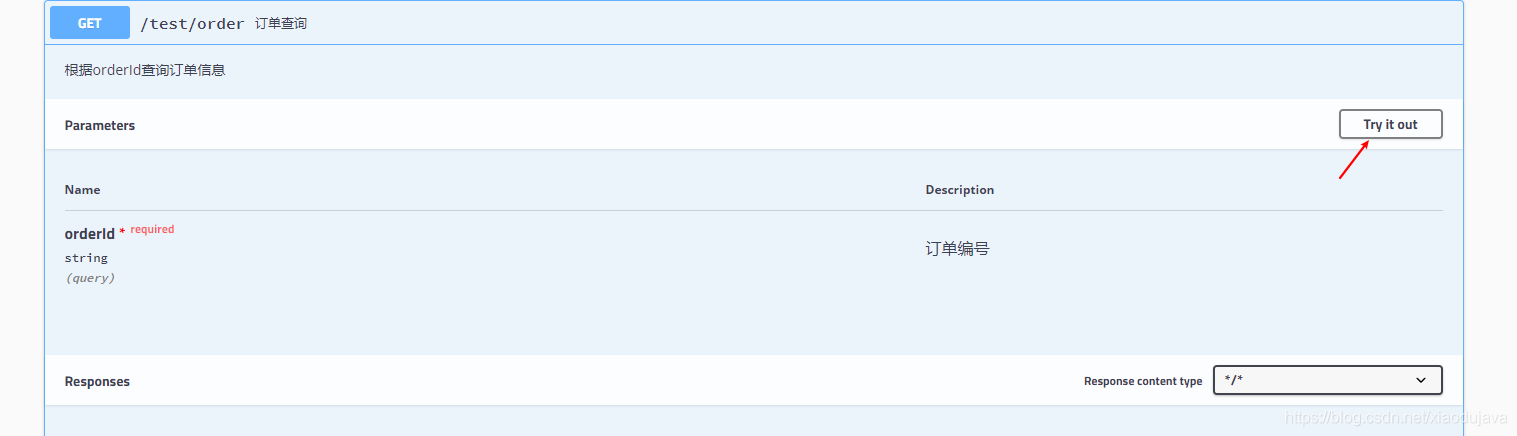 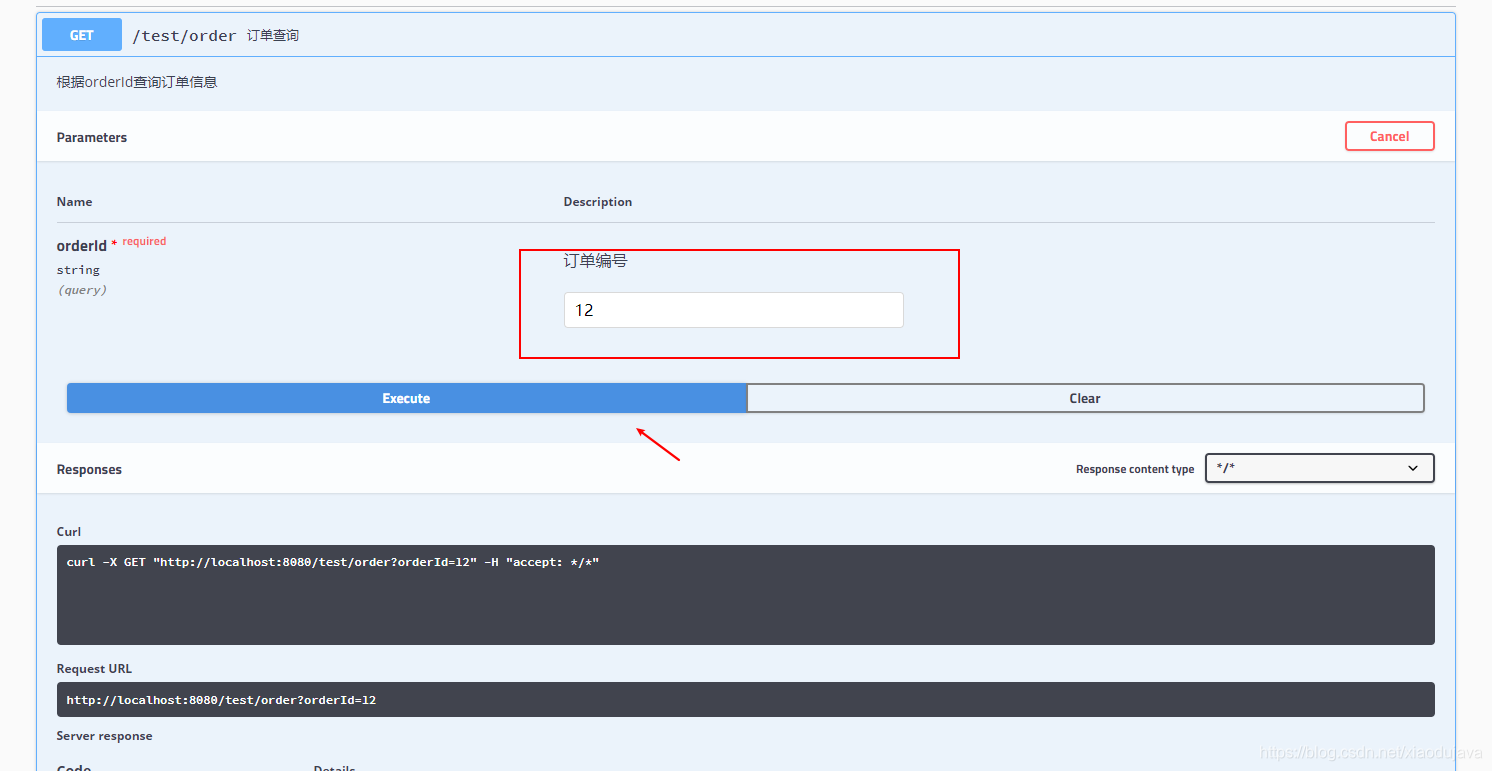
下一节 Spring boot 参数校验
以上是关于Spring boot crud 和 swagger使用的主要内容,如果未能解决你的问题,请参考以下文章