EAMCSCRIPT6
Posted xkjlive
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了EAMCSCRIPT6相关的知识,希望对你有一定的参考价值。
1. 知识全景图
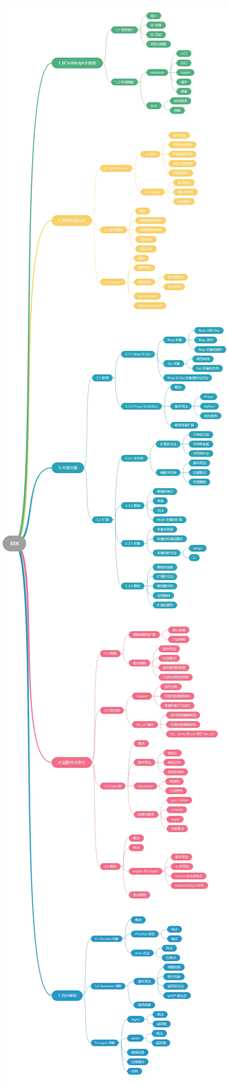
2. ES6简要知识归类
2.1. 概述
ECMAScript 6 目前基本成为业界标准,它的普及速度比 ES5 要快很多,主要原因是现代浏览器对 ES6 的支持相当迅速,尤其是 Chrome 和 Firefox 浏览器,已经支持 ES6 中绝
大多数的特性。
2.2. let、const 和 block 作用域
2.2.1. let 允许创建块级作用域,ES6 推荐在函数中使用 let 定义变量,而非 var:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <script> var a = 2; //var 全局变量,使用var声明的变的作用域或提升 { let a = 3; //let 具有块级作用域 console.log("let: "+a); //3 } console.log("var: "+a); //2 </script> </head> <body> </body> </html>
2.2.2. const
同样在块级作用域有效的另一个变量声明方式是 const,它可以声明一个常量。ES6 中,const 声明的常量类似于指针,它指向某个引用,也就是说这个「常量」并非一成不变的,如:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <script> { const ARR = [5,6]; console.log("before: " + ARR); //[5,6] ARR.push(7); console.log("after: " + ARR); //[5,6,7] //ARR = 10; 报错了,语法变得严格了,提示已经命名变量了。 typeError } </script> </head> <body> </body> </html>
有几个点需要注意:
let 关键词声明的变量不具备变量提升(hoisting)特性
let 和 const 声明只在最靠近的一个块中(花括号内)有效
当使用常量 const 声明时,请使用大写变量,如:CAPITAL_CASING
const 在声明时必须被赋值
2.3. 箭头函数(Arrow Functions)
ES6 中,箭头函数就是函数的一种简写形式,使用括号包裹参数,跟随一个 =>,紧接着是函数体
var getPrice = function() { return 4.55; }; // Implementation with Arrow Function var getPrice = () => 4.55;
传统写法:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <script> var getPrice = function() { return 4.55; }; console.log(getPrice()); </script> </head> <body> </body> </html>
箭头函数写法:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <script> // var getPrice = function() { // return 4.55; // }; // console.log(getPrice()); let getPrice = () => 4.55 // ()代表函数参数,=>后面代表函数体 console.log(getPrice()); </script> </head> <body> </body> </html>
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <script> // var getPrice = function() { // return 4.55; // }; // console.log(getPrice()); let getPrice = () => 4.55; console.log(getPrice()); let getSum = (a,b) => a+b; console.log(getSum(2,3)); </script> </head> <body> </body> </html>
需要注意的是,上面例子中的 getPrice 箭头函数采用了简洁函数体,它不需要 return 语句,下面这个例子使用的是正常函数体:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <script> // var getPrice = function() { // return 4.55; // }; // console.log(getPrice()); let getPrice = () => 4.55; console.log(getPrice()); let getSum = (a,b) => a+b; console.log(getSum(2,3)); let arr = [‘apple‘,‘banana‘,‘orange‘]; let breakfast = arr.map(fruit => { return fruit + ‘s‘; }); console.log(breakfast); </script> </head> <body> </body> </html>
当然,箭头函数不仅仅是让代码变得简洁,函数中 this 总是绑定总是指向对象自身。具体可以看看下面几个例子:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <script> // var getPrice = function() { // return 4.55; // }; // console.log(getPrice()); let getPrice = () => 4.55; console.log(getPrice()); let getSum = (a,b) => a+b; console.log(getSum(2,3)); let arr = [‘apple‘,‘banana‘,‘orange‘]; let breakfast = arr.map(fruit => { return fruit + ‘s‘; }); console.log(breakfast); function person() { this.age = 0; setInterval(function growUp() { //非严格模式下,growUp()函数的this指向window对象 this.age++; },1000); } var person = new person(); console.log(person); </script> </head> <body> </body> </html>
我们经常需要使用一个变量来保存 this,然后在 growUp 函数中引用
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <script> // var getPrice = function() { // return 4.55; // }; // console.log(getPrice()); // let getPrice = () => 4.55; // console.log(getPrice()); // // let getSum = (a,b) => a+b; // console.log(getSum(2,3)); // // let arr = [‘apple‘,‘banana‘,‘orange‘]; // let breakfast = arr.map(fruit => { // return fruit + ‘s‘; // }); // console.log(breakfast); // function person() { // this.age = 0; // // setInterval(function growUp() { // //非严格模式下,growUp()函数的this指向window对象 // this.age++; // },1000); // } // var person = new person(); // console.log(person); function Person() { var self = this; self.age = 0; setInterval(function growUp() { self.age++; },1000); } // function Person() { // this.age = 0; // // setInterval(()=>{ // // his 指向person对象 // this.age++; // },1000); // } var person = new Person(); console.log(person.age); </script> </head> <body> </body> </html>
而使用箭头函数可以省却这个麻烦:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <script> // var getPrice = function() { // return 4.55; // }; // console.log(getPrice()); // let getPrice = () => 4.55; // console.log(getPrice()); // // let getSum = (a,b) => a+b; // console.log(getSum(2,3)); // // let arr = [‘apple‘,‘banana‘,‘orange‘]; // let breakfast = arr.map(fruit => { // return fruit + ‘s‘; // }); // console.log(breakfast); // function person() { // this.age = 0; // // setInterval(function growUp() { // //非严格模式下,growUp()函数的this指向window对象 // this.age++; // },1000); // } // var person = new person(); // console.log(person); // function Person() { // var self = this; // self.age = 0; // // setInterval(function growUp() { // self.age++; // },1000); // } function Person() { this.age = 0; setInterval(()=>{ // his 指向person对象 this.age++; },1000); } var person = new Person(); console.log(person.age); </script> </head> <body> </body> </html>
2.3. 函数参数默认值
以上是关于EAMCSCRIPT6的主要内容,如果未能解决你的问题,请参考以下文章