576. Out of Boundary Paths
Posted ruruozhenhao
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了576. Out of Boundary Paths相关的知识,希望对你有一定的参考价值。
There is an m by n grid with a ball. Given the start coordinate (i,j) of the ball, you can move the ball to adjacent cell or cross the grid boundary in four directions (up, down, left, right). However, you can at most move N times. Find out the number of paths to move the ball out of grid boundary. The answer may be very large, return it after mod 109 + 7.
Example 1:
Input: m = 2, n = 2, N = 2, i = 0, j = 0 Output: 6 Explanation:
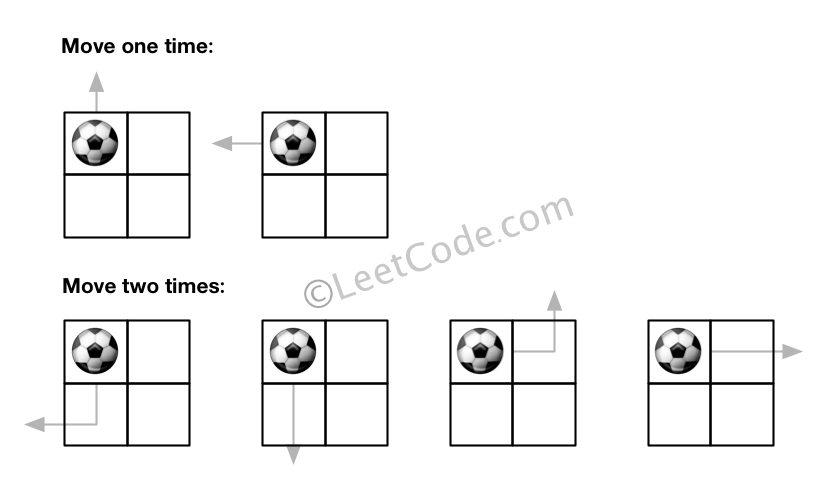
Example 2:
Input: m = 1, n = 3, N = 3, i = 0, j = 1 Output: 12 Explanation:
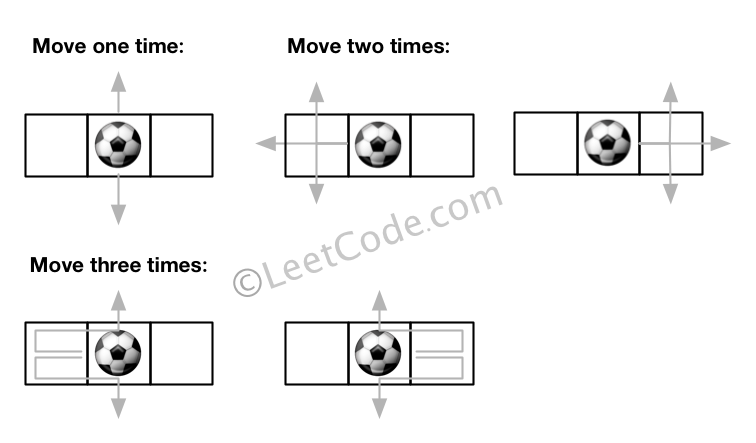
Note:
- Once you move the ball out of boundary, you cannot move it back.
- The length and height of the grid is in range [1,50].
- N is in range [0,50].
Approach #1: DP. [C++]
class Solution { public: int findPaths(int m, int n, int N, int i, int j) { const int mod = 1000000007; vector<vector<vector<int>>> dp(N+1, vector<vector<int>>(m, vector<int>(n, 0))); vector<int> dirs = {1, 0, -1, 0, 1}; for (int s = 1; s <= N; ++s) { for (int x = 0; x < m; ++x) { for (int y = 0; y < n; ++y) { for (int k = 0; k < 4; ++k) { int dx = x + dirs[k]; int dy = y + dirs[k+1]; if (dx < 0 || dy < 0 || dx >= m || dy >= n) dp[s][x][y] += 1; else dp[s][x][y] = (dp[s][x][y] + dp[s-1][dx][dy]) % mod; } } } } return dp[N][i][j]; } };
Analysis:
Observation:
Number of paths start from (i, j) to out of boundary <=> Number of paths start from out of boundary to (i, j).
dp[N][i][j] : Number of paths start from out of boundary to (i, j) by moving N steps.
dp[*][y][x] = 1, if (x, y) are out of boundary
dp[s][i][j] = dp[s-1][i+1][j] + dp[s-1][i-1][j] + dp[s-1][i][j+1] + dp[s-1][i][j-1]
Ans: dp[N][i][j]
Time complexity: O(N*m*n)
Space complexity: O(N*m*n) -> O(m*n)
Reference:
http://zxi.mytechroad.com/blog/dynamic-programming/leetcode-576-out-of-boundary-paths/
以上是关于576. Out of Boundary Paths的主要内容,如果未能解决你的问题,请参考以下文章
leetcode 576. Out of Boundary Paths
第十一周 Leetcode 576. Out of Boundary Paths (HARD) 计数dp
2021-8-15 Out of Boundary Paths
LeetCode 545. Boundary of Binary Tree