nio原理和示例代码
Posted wenbinshen
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了nio原理和示例代码相关的知识,希望对你有一定的参考价值。
我正在为学习大数据打基础中,为了手撸rpc框架,需要懂得nio的原理,在搞懂nio框架前,我会带着大家手撸一些比较底层的代码,当然今后当我们学会了框架,这些繁琐的代码也就不用写了,但是学一学底层的代码也是有好处的嘛。
java.nio全称java non-blocking IO(实际上是 new io),是指jdk1.4 及以上版本里提供的新api(New IO) ,为所有的原始类型(boolean类型除外)提供缓存支持的数据容器,使用它可以提供非阻塞式的高伸缩性网络。
前面我写的socket的服务端与客户端的通信是线程阻塞的,这在实际应用场景中并不竟如人意,我们更多需要的是异步操作,用户无感知,当我们在操作主线程的时候,一些通信相关的线程不应该阻塞我们的主线程。我们需要传送数据,我们只要将请求发送出去,这时候具体的发送细节就应该交由底层的操作系统帮我们完成,我们应该可以操作主线程继续完成其他事情。nio就为我们解决这些事情提供了很好的办法。
学会nio之前我们需要了解这几个概念:
Channel:
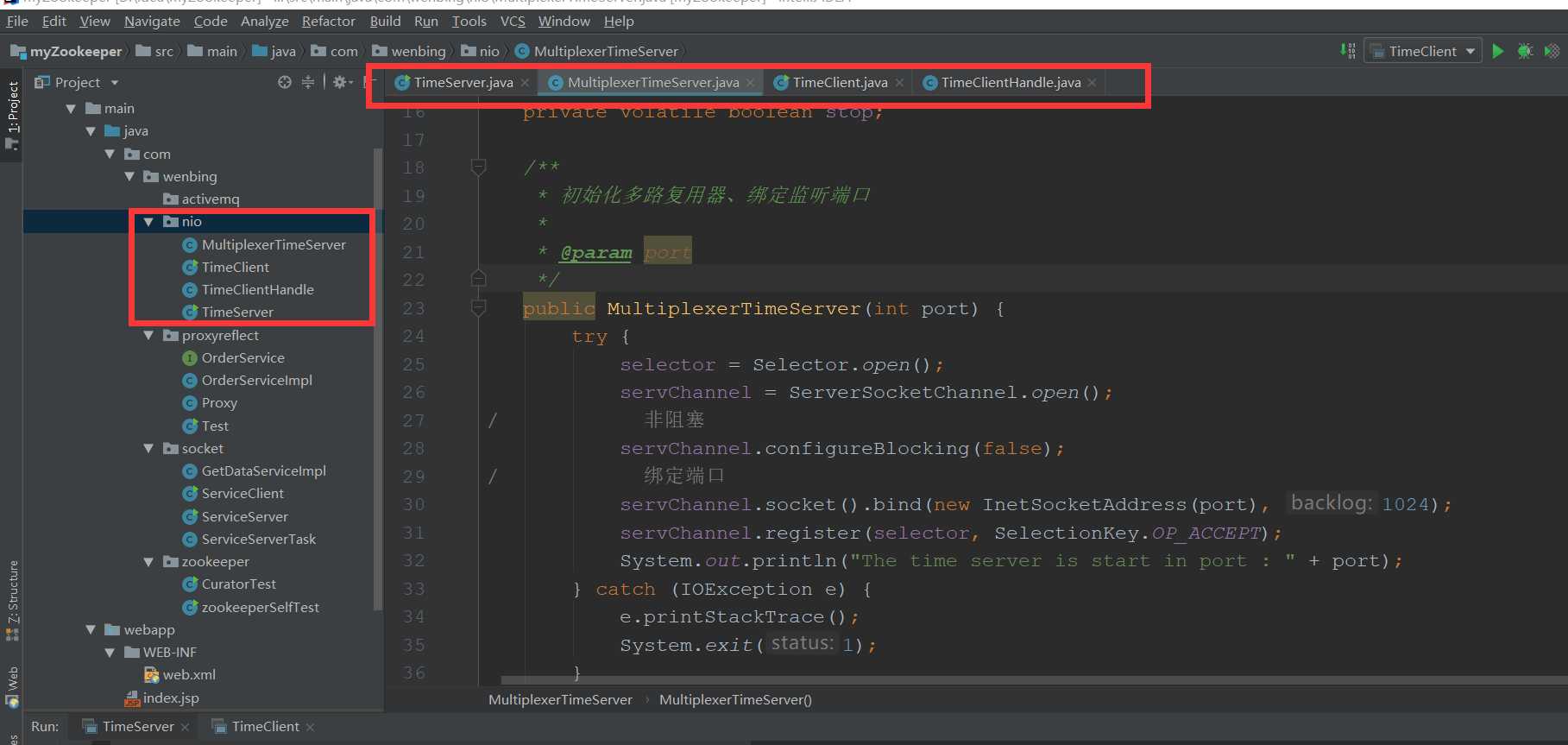
先定义一个TimeServer
package com.wenbing.nio; public class TimeServer { public static void main(String[] args) { int port = 8085; if (args != null && args.length < 0) { port = Integer.valueOf(args[0]); } MultiplexerTimeServer timeServer = new MultiplexerTimeServer(port); new Thread(timeServer,"NIO-MultiplexerTimeServer-001").start(); } }
再定义一个MultiplexerTimeServer去实现Runnable接口,每个通信的操作交由这一个线程去完成。
package com.wenbing.nio; import java.io.IOException; import java.net.InetSocketAddress; import java.nio.ByteBuffer; import java.nio.channels.*; import java.util.Iterator; import java.util.Set; public class MultiplexerTimeServer implements Runnable { private Selector selector; private ServerSocketChannel servChannel; private volatile boolean stop; /** * 初始化多路复用器、绑定监听端口 * * @param port */ public MultiplexerTimeServer(int port) { try { selector = Selector.open(); servChannel = ServerSocketChannel.open(); // 非阻塞 servChannel.configureBlocking(false); // 绑定端口 servChannel.socket().bind(new InetSocketAddress(port), 1024); servChannel.register(selector, SelectionKey.OP_ACCEPT); System.out.println("The time server is start in port : " + port); } catch (IOException e) { e.printStackTrace(); System.exit(1); } } public void stop() { this.stop = true; } @Override public void run() { while (!stop) { try { selector.select(1000); // 查询存在的活跃的key Set<SelectionKey> selectedKeys = selector.selectedKeys(); // 迭代所有活跃的key,进行操作 Iterator<SelectionKey> it = selectedKeys.iterator(); SelectionKey key = null; while (it.hasNext()) { key = it.next(); // 拿到某个key后,就将其从迭代器里除去 it.remove(); try { handleInput(key); } catch (Exception e) { if (key != null) { key.cancel(); if (key.channel() != null) { key.channel().close(); } } } } } catch (Throwable t) { t.printStackTrace(); } } // 多路复用器关闭后,所有注册在上面的Channel和Pipe等资源都会被自动去注册并关闭,所以不需要单个关闭 if (selector != null) { try { selector.close(); } catch (IOException e) { e.printStackTrace(); } } } private void handleInput(SelectionKey key) throws IOException { if (key.isValid()) { // 处理新接入的请求消息 if (key.isAcceptable()) { // Accept the new connection ServerSocketChannel ssc = (ServerSocketChannel) key.channel(); SocketChannel sc = ssc.accept(); sc.configureBlocking(false); // Add the new connection to the selector sc.register(selector, SelectionKey.OP_READ); } if (key.isReadable()) { // Read the data SocketChannel sc = (SocketChannel) key.channel(); ByteBuffer readBuffer = ByteBuffer.allocate(1024); int readBytes = sc.read(readBuffer); if (readBytes > 0) { readBuffer.flip(); byte[] bytes = new byte[readBuffer.remaining()]; readBuffer.get(bytes); String body = new String(bytes, "UTF-8"); System.out.println("The time server receive order : " + body); //将当前时间发回去 String currentTime = "QUERY TIME ORDER" .equalsIgnoreCase(body) ? new java.util.Date( System.currentTimeMillis()).toString() : "BAD ORDER"; doWrite(sc, currentTime); } else if (readBytes < 0) { // 对端链路关闭 key.cancel(); sc.close(); } else ; //读到0字节,忽略 } } } private void doWrite(SocketChannel channel, String response) throws IOException{ if (response != null && response.trim().length() > 0) { byte[] bytes = response.getBytes(); ByteBuffer writeBuffer = ByteBuffer.allocate(bytes.length); writeBuffer.put(bytes); writeBuffer.flip(); channel.write(writeBuffer); } } }
定义TimeClient
package com.wenbing.nio; public class TimeClient { /** * * @param args */ public static void main(String[] args) { int port = 8085; if (args != null && args.length > 0) { try { port = Integer.valueOf(args[0]); } catch (NumberFormatException e) { //采用默认值 } } new Thread(new TimeClientHandle("127.0.0.1", port), "TimeClient-001").start(); } }
定义TimeClientHandle同样继承Runnable接口,与上面的MultiplexerTimeServer作用类似。
package com.wenbing.nio; import java.io.IOException; import java.net.InetSocketAddress; import java.nio.ByteBuffer; import java.nio.channels.SelectionKey; import java.nio.channels.Selector; import java.nio.channels.SocketChannel; import java.util.Iterator; import java.util.Set; public class TimeClientHandle implements Runnable { private String host; private int port; private Selector selector; private SocketChannel socketChannel; private volatile boolean stop; public TimeClientHandle(String host, int port) { this.host = host == null ? "127.0.0.1" : host; this.port = port; try { selector = Selector.open(); socketChannel = SocketChannel.open(); socketChannel.configureBlocking(false); } catch (IOException e) { e.printStackTrace(); System.exit(1); } } @Override public void run() { try { doConnect(); } catch (IOException e) { e.printStackTrace(); System.exit(1); } while (!stop) { try { selector.select(1000); Set<SelectionKey> selectedKeys = selector.selectedKeys(); Iterator<SelectionKey> it = selectedKeys.iterator(); SelectionKey key = null; while (it.hasNext()) { key = it.next(); it.remove(); try { handleInput(key); } catch (Exception e) { if (key != null) { key.cancel(); if (key.channel() != null) { key.channel().close(); } } } } } catch (IOException e) { e.printStackTrace(); System.exit(1); } } } private void doConnect() throws IOException { //如果直接连接成功,则注册到多路复用器上,发送请求消息,读应答 if (socketChannel.connect(new InetSocketAddress(host, port))) { socketChannel.register(selector, SelectionKey.OP_READ); doWriter(socketChannel); } else { socketChannel.register(selector, SelectionKey.OP_CONNECT); } } private void doWriter(SocketChannel sc) throws IOException { byte[] req = "QUERY TIME ORDER".getBytes(); ByteBuffer writeBuffer = ByteBuffer.allocate(1024); writeBuffer.put(req); writeBuffer.flip(); sc.write(writeBuffer); if (!writeBuffer.hasRemaining()) { System.out.println("Send order 2 server succeed."); } } private void handleInput(SelectionKey key) throws IOException { if (key.isValid()) { // 判断连接是否成功 SocketChannel sc = (SocketChannel) key.channel(); if (key.isConnectable()) { if (sc.finishConnect()) { sc.register(selector, SelectionKey.OP_READ); doWriter(sc); } else { System.exit(1);//连接失败,进程退出 } } if (key.isReadable()) { ByteBuffer readBuffer = ByteBuffer.allocate(1024); int readBytes = sc.read(readBuffer); if (readBytes > 0) { readBuffer.flip(); byte[] bytes = new byte[readBuffer.remaining()]; readBuffer.get(bytes); String body = new String(bytes, "UTF-8"); System.out.println("Now is : " + body); this.stop = true; } else if (readBytes < 0) { //对端链路关闭 key.cancel(); sc.close(); } else ;//读到0字节,忽略 } } } }
The time server receive order : QUERY TIME ORDER
Now is : Sun Nov 04 00:10:56 CST 2018
以上是关于nio原理和示例代码的主要内容,如果未能解决你的问题,请参考以下文章
Netty——NIO(Selector处理read事件)代码示例
Netty——NIO(Selector处理read事件)代码示例