单链表的具体实现
Posted zhaobinyouth
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了单链表的具体实现相关的知识,希望对你有一定的参考价值。
1. Linklist设计要点
- 类模板,通过头结点访问后继节点
- 定义内部节点类型Node,用于描述数据域和指针域
- 实现线性表关键操作如增、减、查等
2、Linklist的定义
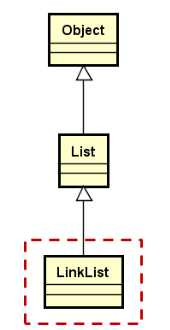

3.代码
1 #ifndef LINKLIST_H 2 #define LINKLIST_H 3 #include "List.h" 4 namespace DataStructureLib 5 { 6 template <typename T> 7 8 class LinkList:public List<T> 9 { 10 protected: 11 struct Node{ 12 T value; 13 Node* next; 14 }; 15 16 mutable Node m_header;//头结点 、mutable为了让get函数中的const属性导致的&m_header(编译器认为是要修改成员变量)mutable就允许const成员函数取地址 17 int m_length;//链表的长度 18 19 Node* position(int i) const//返回第i和元素的指针 20 { 21 Node* ret=&m_header; 22 23 for(int p=0;p<i;p++) 24 { 25 ret=ret->next; 26 } 27 28 return ret;//要查找的元素地址保存在该节点的next指针域中 29 } 30 31 public: 32 LinkList() 33 { 34 m_header.next=NULL; 35 m_length=0; 36 } 37 38 bool insert(int index, const T& elem)//思路:1.找到index位置处的元素;2.在该元素尾部insert新元素 39 { 40 bool ret=(index<=m_length)&&(index>=0); 41 42 Node* NewNode=new Node ; 43 44 if (ret) 45 { 46 if (NULL!=NewNode) 47 { 48 NewNode->value=elem; 49 50 Node* indexNode=position(index); 51 NewNode->next=indexNode->next; 52 indexNode->next=NewNode; 53 54 m_length++; 55 } 56 else{ 57 throw("has Not enougth memory to insert new element ..."); 58 } 59 60 } 61 return ret; 62 } 63 64 bool remove(int index) 65 { 66 bool ret=((index<=m_length)&&(index>=0)); 67 68 if (ret) 69 { 70 Node* CurrentNode=position(index); 71 Node* toDelNode=CurrentNode->next; 72 CurrentNode->next=toDelNode->next; 73 74 delete toDelNode ; 75 m_length--; 76 } 77 78 return ret; 79 } 80 81 bool set(int index,const T& e) 82 { 83 bool ret=((0<=index)&&(index<=m_length)); 84 85 if (ret) 86 { 87 Node* CurrentNode=position(index); 88 CurrentNode->next->value=e; 89 } 90 91 return ret; 92 } 93 94 bool get(int index, T& elem) const 95 { 96 bool ret=((index<=m_length)&&(index>=0)); 97 98 if (ret) 99 { 100 Node* CurrentNode=position(index); 101 elem= CurrentNode->next->value; 102 } 103 104 return ret; 105 } 106 107 int getlength() const 108 { 109 return m_length; 110 111 } 112 113 void clear() 114 { 115 116 117 while (m_header.next) 118 { 119 Node* toDelNode=m_header.next; 120 m_header.next=toDelNode->next; 121 delete toDelNode; 122 } 123 m_length=0; 124 } 125 }; 126 127 } 128 129 #endif
测试代码:
1 #include<iostream> 2 #include "object.h" 3 #include "SeqList.h" 4 #include "LinkList.h" 5 6 using namespace std; 7 using namespace DataStructureLib; 8 9 10 int main(int argc, char const *argv[]) 11 { 12 LinkList<int> linklist; 13 linklist.insert(0,1); 14 linklist.insert(1,2); 15 linklist.insert(2,3); 16 linklist.insert(3,4); 17 linklist.insert(4,5); 18 linklist.insert(5,6); 19 20 for (int i=0;i<linklist.getlength();i++) 21 { 22 int tmp; 23 linklist.get(i,tmp); 24 cout<<tmp<<‘ ‘; 25 } 26 27 linklist.insert(0,0); 28 29 system("pause"); 30 return 0; 31 }
以上是关于单链表的具体实现的主要内容,如果未能解决你的问题,请参考以下文章