pandas的数据结构(Series和DataFrame)
Posted yinziming
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了pandas的数据结构(Series和DataFrame)相关的知识,希望对你有一定的参考价值。
1,Series创建
1)列表+[索引], 索引不填时默认从0-N
from pandas import Series , DataFrame
weight = [3.5065, 3.4882, 3.4849, 3.4885, 3.4942 ]
ses = Series(data = weight)
print (ses)
2)列表+索引
from pandas import Series , DataFrame
weight = ["重量/g", 3.5065, 3.4882, 3.4849, 3.4885, 3.4942 ]
index = ["批次", 1, 2, 3, 4, 5, ]
ses2 = Series(data = weight, index = index)
print (ses2)
3)采用字典创建// 字典中的key转化为Series中的index, value转化为data值//
from pandas import Series,DataFrame
weight_dic = {
"批次":"重量/g", 1: 3.5065, 2:3.4882, 3:3.4849, 4:3.4885 , 5:3.4942
}
ses3 = Series(data = weight_dic)
print (ses3)
=============分割线================
2, DataFrame
1)通过二维数组创建, [index], [columns]可选,若无,则默认0-N
from pandas import Series,DataFrame
weights = [
[3.5065, 3.4882, 3.4849, 3.4885, 3.4942],
[3.5086, 3.5085, 3.4943, 3.5032, 3.4884, ],
[3.5009, 3.4884, 3.5012, 3.5104, 3.5184 ,],
]
df = DataFrame(data = weights)
print (df)
2)增加index, columns
from pandas import Series,DataFrame
weights = [
[3.5065, 3.5086, 3.5009 ],
[3.4882, 3.5085, 3.4884 ],
[3.4849 , 3.4943, 3.5012 ],
[3.4885 , 3.5032, 3.5104 ],
[3.4942 , 3.4884, 3.5184],
]
index = [ 1, 2, 3, 4, 5, ]
columns = ["A样品重量/g", "B样品重量/g", "C样品重量/g", ]
df2 = DataFrame(data = weights, index=index, columns=columns )
print (df2)
3)通过字典创建 字典的key转化为columns, value转化为data值、
value以list形式存储,可增加index行索引
weight_dic = {
"A样品重量/g": [3.5065, 3.4882, 3.4849, 3.4885, 3.4942],
"B样品重量/g": [3.5086, 3.5085, 3.4943, 3.5032, 3.4884 ],
"C样品重量/g": [ 3.5009, 3.4884, 3.5012, 3.5104, 3.5184 ]
}
print (df3)
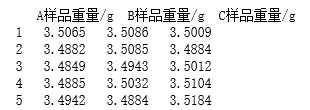
index_list = ["批次1", "批次2", "批次3", "批次4", "批次5"]
weight_dic = {
"A样品重量/g": Series(data = [3.5065, 3.4882, 3.4849, 3.4885, 3.4942], index = index_list),
"B样品重量/g": Series(data = [3.5086, 3.5085, 3.4943, 3.5032, 3.4884 ],index = index_list),
"C样品重量/g": Series(data = [ 3.5009, 3.4884, 3.5012, 3.5104, 3.5184 ],index = index_list)
}
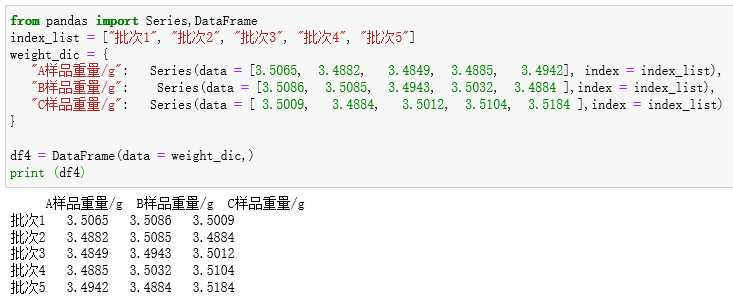
以上是关于pandas的数据结构(Series和DataFrame)的主要内容,如果未能解决你的问题,请参考以下文章