Python基础--丰富的else语句/简洁的with语句/字典dict{}/集合set{}
Posted 吴英强
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了Python基础--丰富的else语句/简洁的with语句/字典dict{}/集合set{}相关的知识,希望对你有一定的参考价值。
丰富的else语句
要么怎样,要么不怎样(if)
干完了能怎样,干不完就别想怎样(for、while循环)
def showMaxFactor(num):
count = num // 2
while count > 1:
print('count = %d' % count)
if num % count == 0:
print('%d最大的约数是%d' %(num, count))
break
count -= 1
else:
print('%d是素数'% num)
num = int(input('请输入一个数:'))
showMaxFactor(num)

没有问题,那就干吧 (异常处理)
try:
#print(int('abc'))
print(int('123'))
except ValueError as reason:
print('出错啦' + str(reason))
else:
print('没有任何异常')

简洁的with语句
try:
f = open('data.txt', 'w')
for each_line in f:
print(each_line)
except OSError as reason:
print('出错啦' + str(reason))
finally:
f.close()
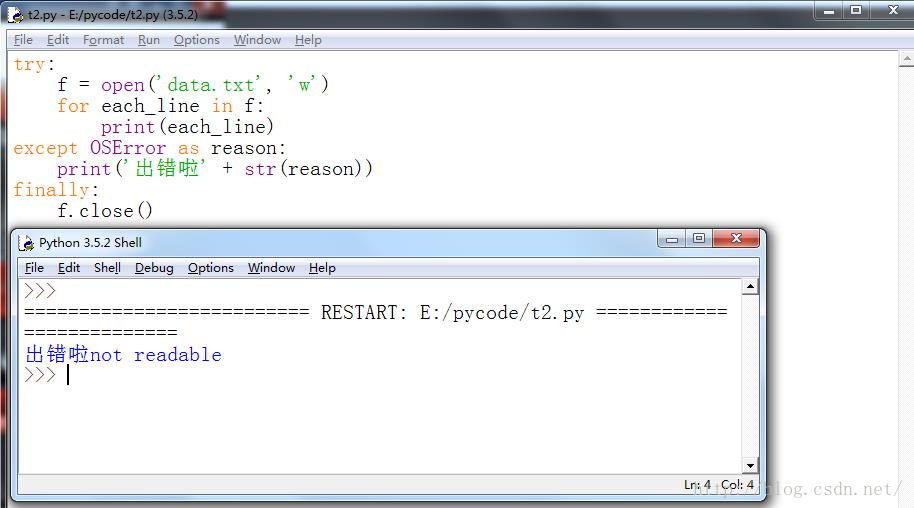
try:
with open('data.txt', 'w') as f:
for each_line in f:
print(each_line)
except OSError as reason:
print('出错啦' + str(reason))

使用with后,自动调用close()。
字典dict
>>> brand = ['lining', 'nike', 'adidas']
>>> slogan = ['Anything is possible', 'Just do it', 'Impossible is nothing']
>>> print('lining slogan is :', slogan[brand.index('lining')])
lining slogan is : Anything is possible
>>> dict1 = 'lining': 'Anything is possible', 'nike':'Just do it', 'adidas':'Impossible is nothing'
>>> print('lining slogan is:', dict1['lining'])
lining slogan is: Anything is possible
>>>

>>> dict1 =
>>> dict1.fromkeys((1, 2, 3))
1: None, 2: None, 3: None
>>> dict1.fromkeys((1, 2, 3), 'number')
1: 'number', 2: 'number', 3: 'number'
>>> dict1.fromkeys((1, 2, 3), 'one', 'two', 'three')
Traceback (most recent call last):
File "<pyshell#20>", line 1, in <module>
dict1.fromkeys((1, 2, 3), 'one', 'two', 'three')
TypeError: fromkeys expected at most 2 arguments, got 4
>>> dict1.fromkeys((1, 2, 3), ('one', 'two', 'three'))
1: ('one', 'two', 'three'), 2: ('one', 'two', 'three'), 3: ('one', 'two', 'three')
>>>
>>> dict2 =
>>> dict2 = dict2.fromkeys(range(5), '赞')
>>> dict2
0: '赞', 1: '赞', 2: '赞', 3: '赞', 4: '赞'
>>> for eachkey in dict2.keys():
print(eachkey)
0
1
2
3
4
>>>
>>> for eachvalue in dict2.values():
print(eachvalue)
赞
赞
赞
赞
赞
>>> for eachitem in dict2.items():
print(eachitem)
(0, '赞')
(1, '赞')
(2, '赞')
(3, '赞')
(4, '赞')
>>> print(dict2[4])
赞
>>> print(dict2[5])
Traceback (most recent call last):
File "<pyshell#41>", line 1, in <module>
print(dict2[5])
KeyError: 5
>>> dict2.get(5)
>>> print(dict2.get(5))
None
>>> dict2.get(5, 'haha')
'haha'
>>> dict2.get(4, 'haha')
'赞'
>>> 4 in dict2
True
>>> 5 not in dict2
True
>>> 5 in dict2
False
>>> dict2.clear()
>>> dict2
>>>
>>> a = 'name':'wuyq'
>>> b =a
>>> a
'name': 'wuyq'
>>> b
'name': 'wuyq'
>>> a =
>>> a
>>> b
'name': 'wuyq'
>>>
>>>
>>> a = b
>>> a
'name': 'wuyq'
>>> b
'name': 'wuyq'
>>> a.clear()
>>> a
>>> b
>>>
>>> a = 1:'one', 2:'two', 3:'three'
>>> b = a.copy()
>>> c = a
>>> a
1: 'one', 2: 'two', 3: 'three'
>>> b
1: 'one', 2: 'two', 3: 'three'
>>> c
1: 'one', 2: 'two', 3: 'three'
>>> id(a)
58001288
>>> id(b)
55237704
>>> id(c)
58001288
>>> c[4] = 'four'
>>> c
1: 'one', 2: 'two', 3: 'three', 4: 'four'
>>> a
1: 'one', 2: 'two', 3: 'three', 4: 'four'
>>> b
1: 'one', 2: 'two', 3: 'three'
>>>
>>> a.pop(2)
'two'
>>> a
1: 'one', 3: 'three', 4: 'four'
>>> a.popitem()
(1, 'one')
>>> a
3: 'three', 4: 'four'
>>> a.setdefault('xiaobai')
>>> a
3: 'three', 4: 'four', 'xiaobai': None
>>> a.setdefault(5, 'five')
'five'
>>> a
3: 'three', 4: 'four', 'xiaobai': None, 5: 'five'
>>> 字典没有顺序的概念
KeyboardInterrupt
>>>
>>> a
3: 'three', 4: 'four', 'xiaobai': None, 5: 'five'
>>> b = 'xiaobai':'dog'
>>> a.update(b)
>>> a
3: 'three', 4: 'four', 'xiaobai': 'dog', 5: 'five'
>>>
集合set
数据唯一,不可以重复,不支持下表索引。元素是无序的
>>> num =
>>> type(num)
<class 'dict'>
>>> num2 = 1, 2, 3, 4, 5
>>> type(num2)
<class 'set'>
>>> num2 = 1, 2, 3, 4, 5, 4, 3, 2,1
>>> num2
1, 2, 3, 4, 5
>>> num2[2]
Traceback (most recent call last):
File "<pyshell#30>", line 1, in <module>
num2[2]
TypeError: 'set' object does not support indexing
>>> set1 = set([1, 2, 3, 4])
>>> set1
1, 2, 3, 4
>>>

去掉列表中的重复元素
>>> num1 = [1, 2, 3, 4, 5,0, 5, 3, 1]
>>> num1
[1, 2, 3, 4, 5, 0, 5, 3, 1]
>>> tmp = []
>>> for each in num1:
if each not in tmp:
tmp.append(each)
>>> tmp
[1, 2, 3, 4, 5, 0]
>>> num1
[1, 2, 3, 4, 5, 0, 5, 3, 1]
>>> num1 = list(set(num1))
>>> num1
[0, 1, 2, 3, 4, 5]
>>>
>>>
>>> 1 in num2
True
>>>
>>> '1' in num2
False
>>>
>>> num2.add(6)
>>> num2
1, 2, 3, 4, 5, 6
>>> num2.remove(1)
>>> num2
2, 3, 4, 5, 6
>>>
>>> num3 = frozenset([1, 2, 3])
>>> num3.add(5)
Traceback (most recent call last):
File "<pyshell#57>", line 1, in <module>
num3.add(5)
AttributeError: 'frozenset' object has no attribute 'add'
>>>
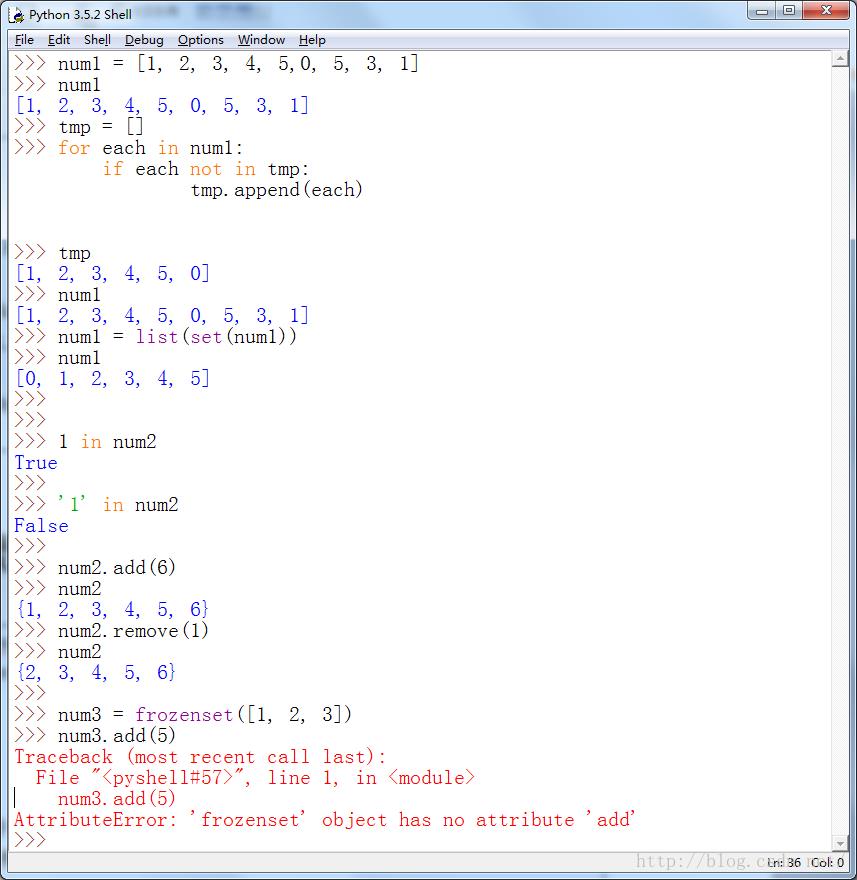
以上是关于Python基础--丰富的else语句/简洁的with语句/字典dict{}/集合set{}的主要内容,如果未能解决你的问题,请参考以下文章
Python--21 丰富的else语句与简洁的else语句