六SpringSecurity Web权限方案—— 基于数据库实现权限认证
Posted 上善若水
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了六SpringSecurity Web权限方案—— 基于数据库实现权限认证相关的知识,希望对你有一定的参考价值。
一、 基于数据库实现权限认证
1.1、项目结构
1.2、添加实体类
package com.xbmu.entity;
import lombok.Data;
@Data
public class Users
private Integer id;
private String username;
private String password;
package com.xbmu.entity;
import lombok.Data;
@Data
public class Role
private Long id;
private String name;
package com.xbmu.entity;
import lombok.Data;
@Data
public class Menu
private Long id;
private String name;
private String url;
private Long parentId;
private String permission;
1.3、编写接口与实现类
package com.xbmu.mapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.xbmu.entity.Menu;
import com.xbmu.entity.Role;
import com.xbmu.entity.Users;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public interface UserMapper extends BaseMapper<Users>
/**
* 根据用户id 查询用户角色
* @param userId 用户id
* @return
*/
List<Role> selectRoleByUserId(Long userId);
/**
* 根据用户id 查询菜单
* @param userId
* @return
*/
List<Menu> selectMenuByUserId(Long userId);
上述接口需要进行多表管理查询:
需要在resource/mapper
目录下自定义UserMapper.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.xbmu.mapper.UserMapper">
<!--根据用户id 查询角色消息-->
<select id="selectRoleByUserId" resultType="com.xbmu.entity.Role">
SELECT
r.id,
r.NAME
FROM
role r
INNER JOIN role_user ru ON ru.rid = r.id
WHERE
ru.uid = #0
</select>
<!-- 根据用户id 查询权限消息 -->
<select id="selectMenuByUserId" resultType="com.xbmu.entity.Menu">
SELECT
m.id,
m.NAME,
m.url,
m.parentid,
m.permission
FROM
menu m
INNER JOIN role_menu rm ON m.id = rm.mid
INNER JOIN role r ON r.id = rm.rid
INNER JOIN role_user ru ON r.id = ru.rid
WHERE
ru.uid = #0
</select>
</mapper>
package com.xbmu.service;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.xbmu.entity.Menu;
import com.xbmu.entity.Role;
import com.xbmu.entity.Users;
import com.xbmu.mapper.UserMapper;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.authority.AuthorityUtils;
import org.springframework.security.core.authority.SimpleGrantedAuthority;
import org.springframework.security.core.userdetails.User;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.core.userdetails.UsernameNotFoundException;
import org.springframework.stereotype.Service;
import java.util.ArrayList;
import java.util.List;
@Service
@Slf4j
public class LoginService implements UserDetailsService
@Autowired
private UserMapper userMapper;
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException
QueryWrapper<Users> wrapper = new QueryWrapper<>();
wrapper.eq("username",username);
Users users = userMapper.selectOne(wrapper);
// 判断用户对象是否为空
if(null == users)
throw new UsernameNotFoundException("用户名不存在!");
// 获取到用户角色,菜单列表
List<Role> roles = userMapper.selectRoleByUserId(Long.valueOf(users.getId()));
List<Menu> menus = userMapper.selectMenuByUserId(Long.valueOf(users.getId()));
// 声明一个集合
List<GrantedAuthority> grantedAuthorityList = new ArrayList<>();
// 处理角色
for (Role role : roles)
SimpleGrantedAuthority simpleGrantedAuthority = new SimpleGrantedAuthority("ROLE_".concat(role.getName()));
grantedAuthorityList.add(simpleGrantedAuthority);
// 处理权限
for (Menu menu : menus)
grantedAuthorityList.add(new SimpleGrantedAuthority(menu.getPermission()));
// 将用户角色,权限添加到当前用户
return new User(users.getUsername(),users.getPassword(),grantedAuthorityList);
1.4、在配置文件中添加映射
在配置文件中 application.yml
添加
mybatis-plus:
mapper-locations: classpath:mapper/*.xml
1.5、修改访问配置类
1.6、使用管理员与非管理员进行测试
管理员账号登录
非管理员账号登录,会提示403,没有权限。
1.7、自定义403页面
1.7.1、修改访问配置类
http.exceptionHandling().accessDeniedPage("/unAuth.html");
1.7.2、测试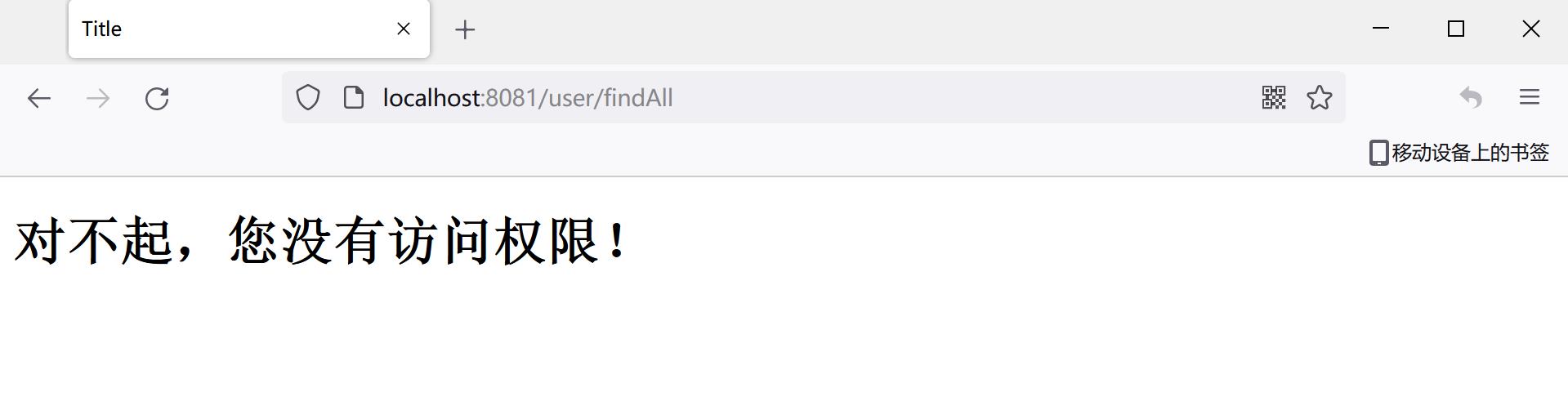
以上是关于六SpringSecurity Web权限方案—— 基于数据库实现权限认证的主要内容,如果未能解决你的问题,请参考以下文章
[SpringSecurity]web权限方案_用户授权_自定义403页面
五SpringSecurity Web权限方案——自定义登录页面与权限访问控制