gin接收参数和返回数据
Posted issue是fw
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了gin接收参数和返回数据相关的知识,希望对你有一定的参考价值。
返回JSON数据
方式一
用map
作序列化
package main
import(
"github.com/gin-gonic/gin"
)
func main()
r := gin.Default()
r.GET("/json",func(c *gin.Context)
c.JSON(200,gin.H
"name":"cl",
"message":"kkk",
"age":"19",
)
)
r.Run()
其中gin.H
是一种生成map[string]interface
的快捷方式
方式二
返回struct
作序列化
package main
import (
"github.com/gin-gonic/gin"
"net/http"
)
func main()
r := gin.Default()
type msg struct
Name string
Message string
Age int
r.GET("/struct_json",func(c *gin.Context)
data := msg"cl","消息",19
c.JSON(http.StatusOK,data )
)
r.Run()
这里需要注意的是结构体的字段必须是以大写字母开头(对外暴露),否则接收不到
如果实在想返回首字母为小写,可以使用标签来解决
type msg struct
Name string `json:"name"`
Message string
Age int
接收GET请求参数
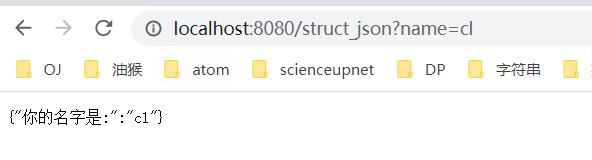
package main
import (
"github.com/gin-gonic/gin"
"net/http"
)
func main()
r := gin.Default()
r.GET("/struct_json",func(c *gin.Context)
name := c.Query("name")
c.JSON(http.StatusOK,gin.H
"你的名字是:":name,
)
)
r.Run()
除了Query
函数,还有下面两种方法取值
name := c.DefaultQuery("name","默认值") //若不存在name参数,返回默认值(即第二个参数)
name,ok := c.GetQuery("name") //ok表示是否存在name参数,不存在返回false
接收FORM表单参数
前端代码为
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<form action="/login" method="post" >
<label for="username">username: </label>
<input type="text" name="username" id="username">
<label for="password">password: </label>
<input type="password" name="password" id="password">
<input type="submit">
</form>
</body>
</html>
package main
import (
"fmt"
"github.com/gin-gonic/gin"
"net/http"
)
func main()
r := gin.Default()
r.LoadHTMLFiles("./login.html")//解析网页
r.GET("/login",func(c *gin.Context)//get请求就返回网页
c.HTML( http.StatusOK,"login.html",nil )
)
r.POST("/login",func(c *gin.Context)//post请求就接收参数并打印出来
username := c.PostForm("username")
password := c.PostForm("password")
fmt.Println("接收到参数username="+username+",password="+password )
)
r.Run()


类似上面的,除了PostForm
方法外,还有GetPostForm
和DefaultPostForm
获取url中的参数(restful风格)
如图所示,访问路径形如http://localhost:8080/user/username/password
其中username
和password
是参数名,实际值需要获取
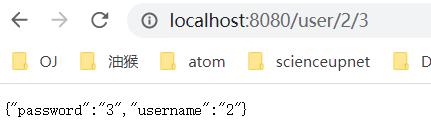
package main
import (
"github.com/gin-gonic/gin"
"net/http"
)
func main()
r := gin.Default()
r.GET("/user/:username/:password",func(c *gin.Context)
username := c.Param("username")
password := c.Param("password")
c.JSON( http.StatusOK,gin.H
"username":username,
"password":password,
)
)
r.Run()
gin参数绑定
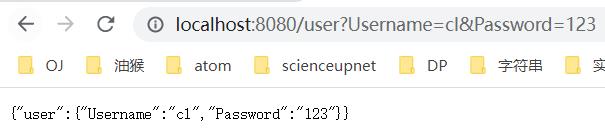
先定义结构体,然后直接用结构体的字段一次性匹配多个参数会更加方便
package main
import (
"github.com/gin-gonic/gin"
"net/http"
)
type User struct
Username string ``
Password string ``
func main()
r := gin.Default()
r.GET("/user",func(c *gin.Context)
var me User
err := c.ShouldBind( &me )
if err != nil
c.JSON(http.StatusBadRequest,gin.H
"error":err.Error(),
)
else
c.JSON(http.StatusOK,gin.H
"user":me,
)
)
r.Run()
以上是关于gin接收参数和返回数据的主要内容,如果未能解决你的问题,请参考以下文章