React中的状态管理---Mobx
Posted 前端纸飞机
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了React中的状态管理---Mobx相关的知识,希望对你有一定的参考价值。
Mobx的介绍
Mobx是一个功能强大,上手非常容易的状态管理工具。redux的作者也曾经向大家推荐过它,在不少情况下可以使用Mobx来替代掉redux。
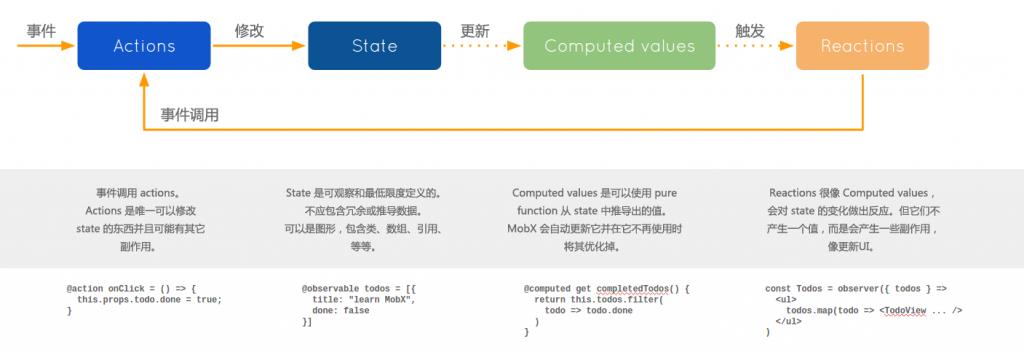
Mobx使用流程
创建项目
npx create-react-app mobx
进入项目
cd mobx
项目抽离
yarn eject
安装mobx mobx-react
yarn add mobx mobx-react
注意: 如果git冲突 解决: 我们要原操作先放到本地暂存盘 git add . git commit -m '安装mobx mobx-react' 注意不要git push
配置装饰器(修饰器 es6 ) babel
yarn add babel-plugin-transform-decorators-legacy -D
yarn add @babel/preset-env -D
yarn add babel-plugin-transform-class-properties -D
yarn add @babel/plugin-proposal-decorators -D
配置package.json
"babel": {
"plugins": [
[
"@babel/plugin-proposal-decorators",
{
"legacy": true
}
],
"transform-class-properties"
],
"presets": [
"react-app",
"@babel/preset-env"
]
},
注意: 以下两个配置顺序不可更改
[
"@babel/plugin-proposal-decorators",
{
"legacy": true
}
],
"transform-class-properties"
项目中 mobx应该怎么用?
新建store目录
src下建立
src store home index.js car index.js index.js
以这种结构建立文件和目录
在主入口文件中 使用 Provider
import store from './store'
import { Provider } from 'mobx-react'
ReactDOM.render(
<Provider store = { store }>
<App />
</Provider>
, document.getElementById('root'));
打造mobx 数据包
import {
observable, computed,action
} from 'mobx'
class Home {
@observable //监听 age
age = 18
@computed //当age发生改变时, 自动触发
get doubleAge(){
return this.age *= 2
}
@action // 用户操作 事件调用
increment(){
this.props.store.home.age ++
console.log( this.props.store.home.age )
//数据请求
fetch('/data/data.json')
.then(res => res.json())
.then( result => console.log( result ))
.catch( error => console.log( error ))
}
}
const home = new Home()
export default home
打造store
store/index.js
import home from './home'
const store = {
//实例
home
}
export default store
组件内使用数据
this.props.store.xxx 可以拿到数据
注意:
- this.porps里面没有找到 @action 装饰器定义的方法, 但是可以直接使用,
- this会丢失 this.props.store.home.increment.bind(this)
在你要使用store的组件上记得做观察
import React,{ Component,Fragment } from 'react'
import { inject,observer } from 'mobx-react'
@inject('store')
@observer
class Count extends Component {
constructor ( props ) {
super( props )
props.store.count.change = props.store.count.change.bind( this )//this丢失的解决
}
increment = () => {
console.log( 'mine' )
this.props.store.count.change()
}
render () {
return (
<Fragment>
<button onClick = { this.increment }>点我+</button>
<p>count:{ this.props.store.count.count} </p>
</Fragment>
)
}
}
export default Count
以上是关于React中的状态管理---Mobx的主要内容,如果未能解决你的问题,请参考以下文章