[JUnit] JUnit5 基础 4 - Maven 集成,参数化测试,重复测试 [完]
Posted GoldenaArcher
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了[JUnit] JUnit5 基础 4 - Maven 集成,参数化测试,重复测试 [完]相关的知识,希望对你有一定的参考价值。
[JUnit] JUnit5 基础 4 - Maven 集成,参数化测试,重复测试 [完]
前面的系列:
- [JUnit] JUnit5 基础 1 - Junit5 结构 与 断言的使用
- [JUnit] JUnit5 基础 2 - 生命周期注解 和 DisplayName 注解
- [JUnit] JUnit5 基础 3 - 依赖注入,假设(assume),开启/禁用测试 与 嵌套测试
Maven 集成
Maven 是一个自动化的管理工具,它主要的目的有:
- 描述软件应该怎样被构建(build)
- 描述项目的依赖关系(dependency)
Maven 使用 XML 文件去进行项目管理,一般的入口文件名为 pom.xml
。
VSCode 集成 Maven 的步骤之前写过笔记:[VSCode] Java JDK/JRE 的配置及 Maven 初始化项目,这里不多赘述。
Junit5 依赖
想要运行 Junit5,Maven 必须至少配置以下三个依赖:
-
junit-jupiter-api
定义了 Junit 的 API
-
junit-jupiter-engine
用于执行 Junit 的测试案例
-
maven-surefire-plugin
用于在 maven 构件的时候运行 Junit
以及,Junit5 需要 JAVA8 以上才能运行,如果想要兼容 Junit4,则需要使用额外的依赖。一个个单独配置相对而言比较麻烦,Junit 也提供了一个 集合器(aggregator):JUnit Jupiter (Aggregator),集合器 集成了其他需要的 Junit 依赖,包括:
- junit-jupiter-api
- junit-jupiter-params
- junit-jupiter-engine
- junit-bom
参数化测试
除了基础的三个依赖之外,参数化测试还需要 junit-jupiter-params 依赖才能实现,这也是推荐使用 JUnit Jupiter (Aggregator) 的原因。
使用 @ValueSource 进行参数化测试
@ValueSource
会将一组数组传给 @ParameterizedTest
,它接受的参数类型包括:
- short
- byte
- int
- long
- float
- double
- char
- java.lang.String
- java.lang.Class
假设这里有一个类包含一个函数测试一个整数是否是奇数,测试方法实现如下:
package com.example;
import static org.junit.jupiter.api.Assertions.assertTrue;
import org.junit.jupiter.api.Test;
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.ValueSource;
public class OddNumberTest {
@ParameterizedTest
@ValueSource(ints = {1,3,5,7,10})
@Test
void testOddNumber(int number) {
OddNumber oddNum = new OddNumber();
assertTrue(oddNum.isOdd(number));
}
}
运行结果如下:
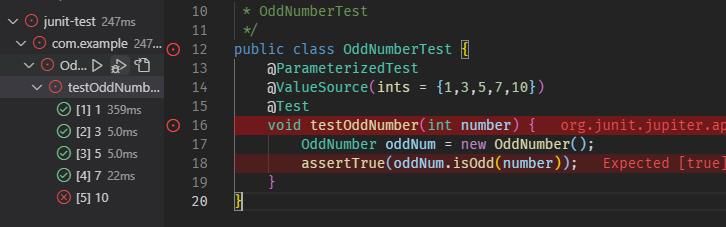
可以看到,奇数的测试案例都通过了,但是偶数的测试案例都失败了。
使用 @EnumSource 进行参数化测试
@EnumSource
接受一组 enum 的实例,如:
package com.example;
public enum ChessPiece {
QUEEN,
KING,
KNIGHT,
PAWN,
ROOK,
}
测试案例:
package com.example;
import static org.junit.jupiter.api.Assertions.assertNotNull;
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.EnumSource;
public class EnumTest {
@ParameterizedTest
@EnumSource(ChessPiece.class)
void testEnum(ChessPiece piece) {
assertNotNull(piece);
}
}
运行结果:
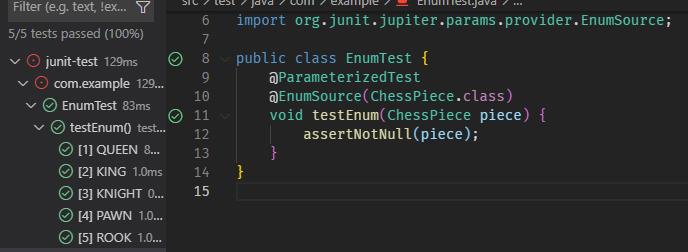
个人觉得 enum 对于多个数据库支持的测试很用帮助。
使用 @MethodSource 进行参数化测试
@MethodSource
支持使用 工厂方法(factory method) 进行传值,注意 工厂方法 必须是一个静态方法,如:
package com.example;
import static org.junit.jupiter.api.DynamicTest.stream;
import java.util.stream.Stream;
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.MethodSource;
public class MethodTest {
@ParameterizedTest
@MethodSource("paramProvider")
void testMethodSource(String arg) {
System.out.println(arg);
}
static Stream<String> paramProvider() {
return Stream.of("para1", "para2");
}
}
运行结果:
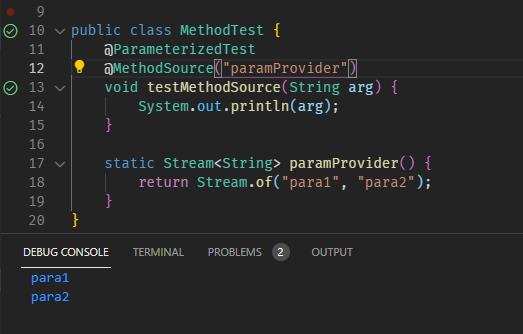
使用 @CsvSource 进行参数化测试
@CsvSource
支持传入一组 逗号分隔 的 键值对数据,如:
package com.example;
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.CsvSource;
public class CsvMethod {
@ParameterizedTest
@CsvSource({"one, 1", "two, 2", "'test, test', 10"})
void testCsvSource(String first, int second) {
System.out.println(first + ", " + second);
}
}
运行结果如下:
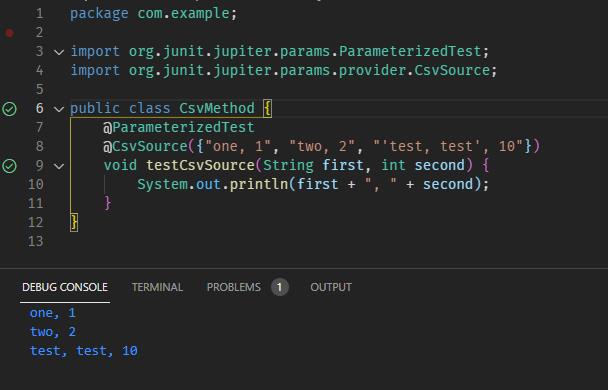
使用 @CsvFileSource 进行参数化测试
@CsvFileSource
与 @CsvSource
几乎一样,只不过 @CsvFileSource
接受的是一个 CSV 文件的路径地址。
重复测试
很多项目在上线前都会进行压力测试,JUnit5 中使用重复测试的方法也很简单:
package com.example;
import org.junit.jupiter.api.DisplayName;
import org.junit.jupiter.api.RepeatedTest;
public class RepeatedTestExample {
@RepeatedTest(name = "{displayName} - {currentRepetition}/{totalRepetitions}",
value = 5)
@DisplayName("Repeated Test")
public void simpleRepeatedTest() {
System.out.println("hello world");
}
}
运行结果:
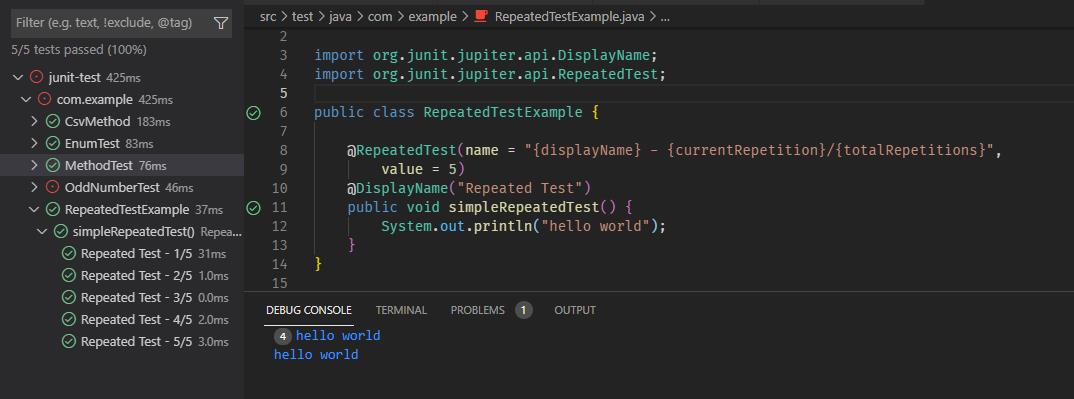
注意使用 displayName
, currentRepetition
, totalRepetitions
关键字可以很方便的标识当前运行的测试。
以上是关于[JUnit] JUnit5 基础 4 - Maven 集成,参数化测试,重复测试 [完]的主要内容,如果未能解决你的问题,请参考以下文章
[JUnit] JUnit5 基础 3 - 依赖注入,假设(assume),开启/禁用测试 与 嵌套测试
JUnit 5 快速上手(从 JUnit 4 到 JUnit 5)
[JUnit] JUnit5 基础 2 - 生命周期注解 和 DisplayName 注解