通过删除字母匹配到字典里最长单词--双指针or自动机预处理?
Posted C_YCBX Py_YYDS
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了通过删除字母匹配到字典里最长单词--双指针or自动机预处理?相关的知识,希望对你有一定的参考价值。
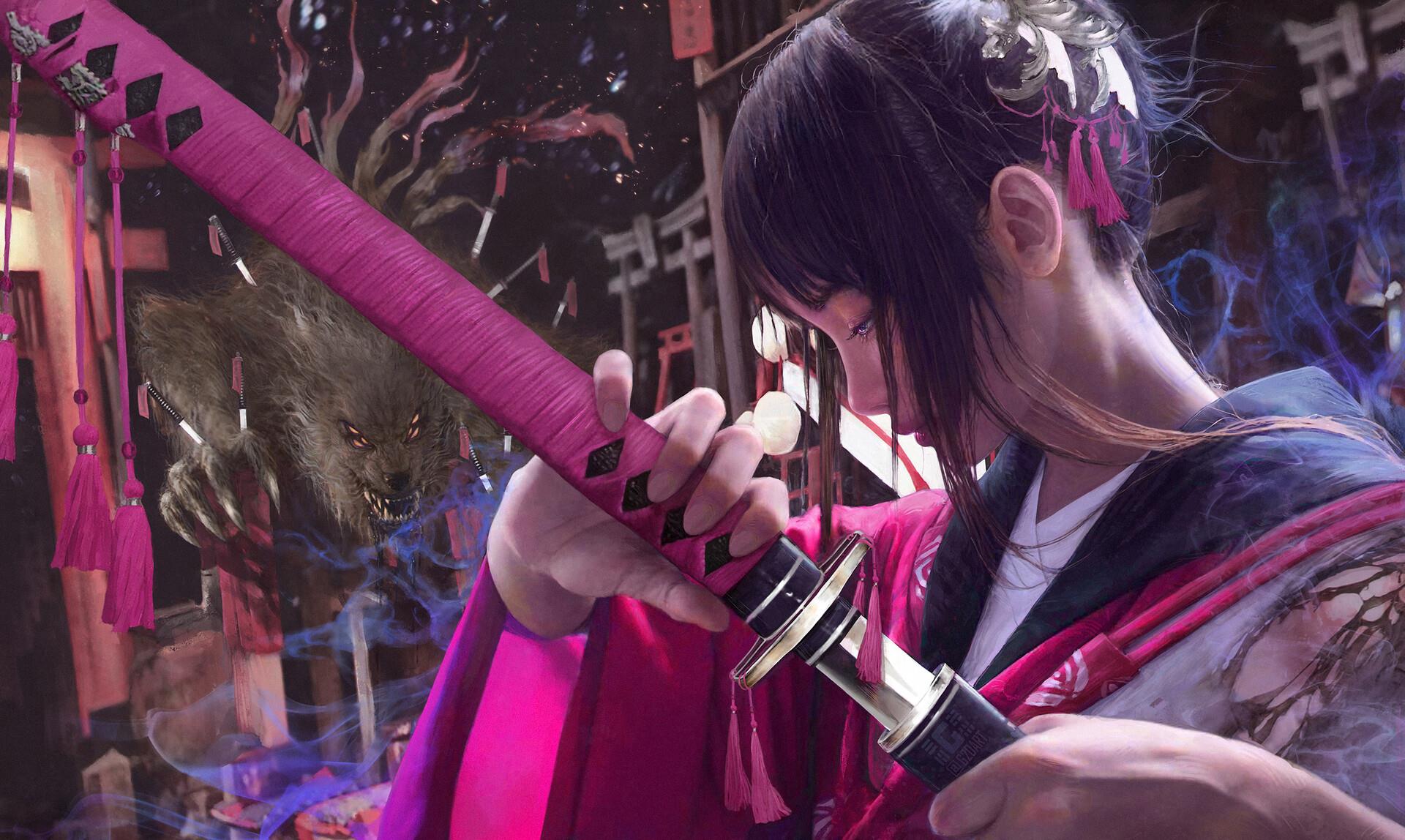
题目
解题思路
只要排好序后,后面的过程就是搜索某个字符串是否属于s的子序列。
- 最直接的方式搜索某个字符串是否是s的子序列的方式就是通过双指针。
- 可以通过数组对s字符串预处理得出它的下标数组,之后根据下标数组快速判断。
代码
- 双指针方式
class Solution {
public:
string findLongestWord(string s, vector<string>& dictionary) {
sort(dictionary.begin(), dictionary.end(), [](const string& a, const string& b) {
return a.size() == b.size() ? a < b : a.size() > b.size();
});
for (auto&& w : dictionary) {
/*双指针操作,由于是判断w是否可以通过删除s串的某些字符得到,
我们分别对dictionary和s取一个指针用于记录下标的遍历情况,
一旦字符相等则同时++,否则只对i++,
如果扫描完了s字符串,j指针仍然未扫描完,则继续扫描下一字符串*/
for (int i = 0, j = 0; i < s.size(); i++) {
j += (s[i] == w[j]);
if (j == w.size()) return w;
}
}
return "";
}
};
- 预处理方式
关键操作在strstr()函数(有点类似于自动机的方式处理)
class Solution {
public:
vector<int> mmp[26]; //存下s的所有字符的对应下标
int curIndex[26]; //用于记录mmp的上一次下标选择,方便下一次选择
string findLongestWord(string s, vector<string>& dictionary) {
for(int i = 0;i<s.size();i++){ //更新mmp数组
mmp[s[i]-'a'].emplace_back(i);
} //自定义排序字符串
sort(dictionary.begin(),dictionary.end(),[](auto&& a, auto&& b){return a.size() > b.size() || a.size() == b.size() && a < b;});
//从大到小查找字典中符合str子串的串,每次调用strstr前把curIndex重置一遍
for(int i=0;i<dictionary.size();i++){
memset(curIndex,0,sizeof(curIndex));
if(strstr(dictionary[i])){
return dictionary[i];
}
}
return "";
}
//根据mmp和curIndex辅助进行查找字典中的字符串是否符合要求。
bool strstr(const string& s){
int pIndex = -1; //关键遍历的三个变量
//pIndex:该字符串的上个字符在字符串s中的下标位置
for(auto&&t:s){ //newIndex:当前这个字符在字符串s中的位置。
//curIndex:表示字符串s中某个字符可选的下一个下标。
if(!mmp[t-'a'].empty()){
int newIndex = INT_MIN;
while(curIndex[t-'a']<mmp[t-'a'].size()&&newIndex<pIndex){
newIndex = mmp[t-'a'][curIndex[t-'a']];
curIndex[t-'a']++;
}
if(newIndex>pIndex)
pIndex = newIndex;
else return false;
}else return false;
}
return true;
}
};
以上是关于通过删除字母匹配到字典里最长单词--双指针or自动机预处理?的主要内容,如果未能解决你的问题,请参考以下文章
Leetcode刷题100天—524. 通过删除字母匹配到字典里最长单词(双指针)—day38
leetcode 524. 通过删除字母匹配到字典里最长单词双指针,在不同字符串中同向查找
leetcode 524. 通过删除字母匹配到字典里最长单词双指针,在不同字符串中同向查找
leetcode 524. 通过删除字母匹配到字典里最长单词双指针在不同字符串中同向查找