设计模式学习笔记——外观模式
Posted x_k
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了设计模式学习笔记——外观模式相关的知识,希望对你有一定的参考价值。
外观模式
外观模式(Facade),为子系统中的一组接口提供一个一致的界面,此模式定义了一个高层接口,这个接口使得这一子系统更加容易使用。结构图
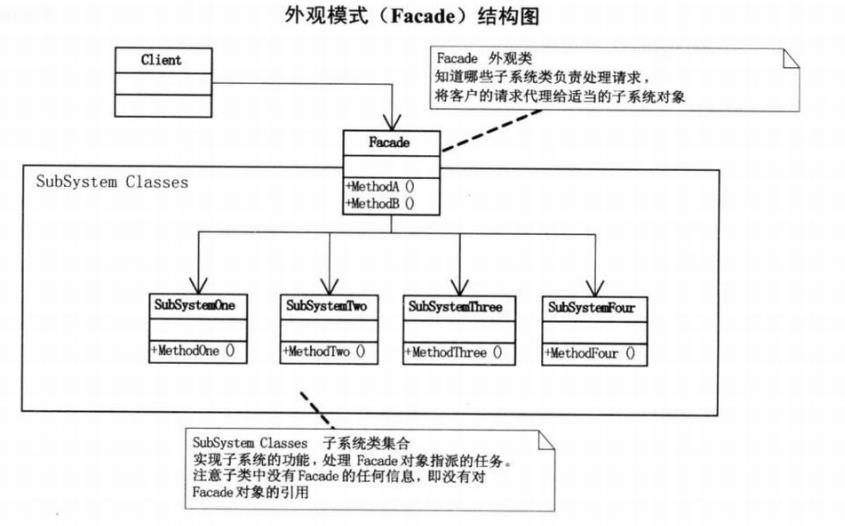
代码实现
子系统:public class SubSystemOne
public void methodOne()
System.out.println("子系统一,方法一");
public class SubSystemTwo
public void methodTwo()
System.out.println("子系统二,方法二");
public class SubSystemThree
public void methodThree()
System.out.println("子系统三,方法三");
外观类Facade:
/**
* 外观类
* @author xukai
* 2016年3月15日 下午3:08:01
*/
public class Facade
SubSystemOne one;
SubSystemTwo two;
SubSystemThree three;
public Facade()
one = new SubSystemOne();
two = new SubSystemTwo();
three = new SubSystemThree();
public void methodA()
System.out.println("组合A:");
one.methodOne();
two.methodTwo();
public void methodB()
System.out.println("组合B:");
two.methodTwo();
three.methodThree();
客户端:
public class Client
public static void main(String[] args)
Facade facade = new Facade();
facade.methodA();
facade.methodB();
demo
问题:炒股未使用外观模式
股民直接和众多股票直接进行接触。 结构图: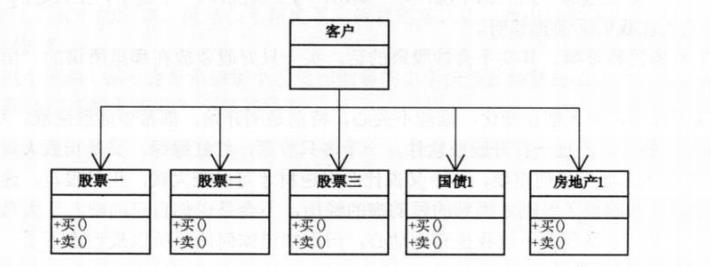
股票1:
/**
* 股票1
*
* @author xukai 2016年3月15日 下午3:32:22
*/
public class Stock1
public void sell()
System.out.println(getClass().getSimpleName() + "售出");
public void buy()
System.out.println(getClass().getSimpleName() + "买入");
股票2:
/**
* 股票2
* @author xukai
* 2016年3月15日 下午3:32:41
*/
public class Stock2
public void sell()
System.out.println(getClass().getSimpleName() + "售出");
public void buy()
System.out.println(getClass().getSimpleName() + "买入");
国债类:
/**
* 国债类1
* @author xukai 2016年3月15日 下午3:37:03
*/
public class NationDebt1
public void sell()
System.out.println(getClass().getSimpleName() + "售出");
public void buy()
System.out.println(getClass().getSimpleName() + "买入");
房地产:
/**
* 房地产1
* @author xukai 2016年3月15日 下午3:36:48
*/
public class Realty1
public void sell()
System.out.println(getClass().getSimpleName() + "售出");
public void buy()
System.out.println(getClass().getSimpleName() + "买入");
客户端,股民进行操作:
package com.xk.day0315.facade.demo;
public class DemoClient
public static void main(String[] args)
Stock1 stock1 = new Stock1();
Stock2 stock2 = new Stock2();
NationDebt1 debt1 = new NationDebt1();
Realty1 realty1 = new Realty1();
// 买入
stock1.buy();
stock2.buy();
debt1.buy();
realty1.buy();
// 卖出
stock1.sell();
stock2.sell();
debt1.sell();
realty1.sell();
操作很复杂,并且对客户来说,任务太重。
使用外观模式
添加基金类,将所有的股票都由基金管理,结构图: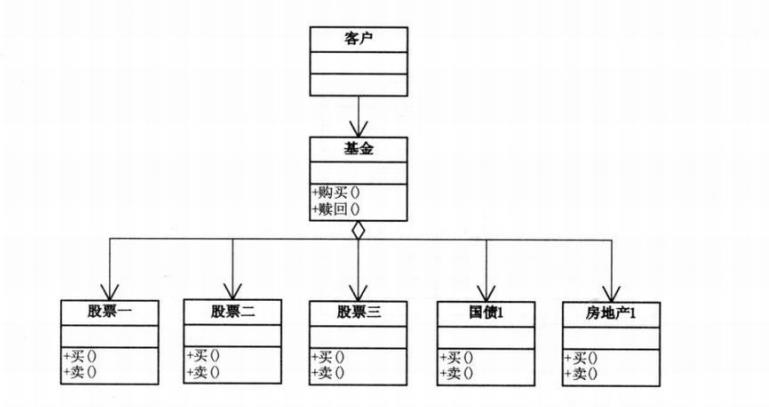
/**
* 基金类
*
* @author xukai 2016年3月15日 下午3:40:03
*/
public class Fund
Stock1 stock1;
Stock2 stock2;
NationDebt1 debt1;
Realty1 realty1;
public Fund()
stock1 = new Stock1();
stock2 = new Stock2();
debt1 = new NationDebt1();
realty1 = new Realty1();
public void buyFund()
stock1.buy();
stock2.buy();
debt1.buy();
realty1.buy();
public void sellFund()
stock1.sell();
stock2.sell();
debt1.sell();
realty1.sell();
股民,客户端:
public class DemoClient2
public static void main(String[] args)
Fund fund = new Fund();
// 购买
fund.buyFund();
// 售出
fund.sellFund();
控制台输出:
Stock1买入
Stock2买入
NationDebt1买入
Realty1买入
Stock1售出
Stock2售出
NationDebt1售出
Realty1售出
总结
1.在设计初期,应该有意识的将不同的两个层分离。如:数据访问层和业务逻辑层、业务逻辑层和表现层 2.子系统会因为不断地重构而变复杂,增加Facade可以提供简单的接口,减少依赖。 3.大型系统的遗留问题,创建Facade类,提供比较清晰简单的接口,新系统与Facade对象交互,Facade对象与遗留代码交互复杂工作。以上是关于设计模式学习笔记——外观模式的主要内容,如果未能解决你的问题,请参考以下文章