JavaScript实现树的深度优先遍历和广度优先遍历
Posted Yolanda_NuoNuo
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了JavaScript实现树的深度优先遍历和广度优先遍历相关的知识,希望对你有一定的参考价值。
javascript实现树的深度优先遍历和广度优先遍历~
一、来棵树
1、tree.js
const tree = {
val: 'a',
children: [
{
val: 'b',
children: [
{
val: 'd',
children: []
},
{
val: 'e',
children: []
},
{
val: 'f',
children: []
}
]
},
{
val: 'c',
children: [
{
val: 'g',
children: []
},
{
val: 'h',
children: []
},
{
val: 'i',
children: []
}
]
}
]
}
module.exports = {
tree
}
2、给它画出来~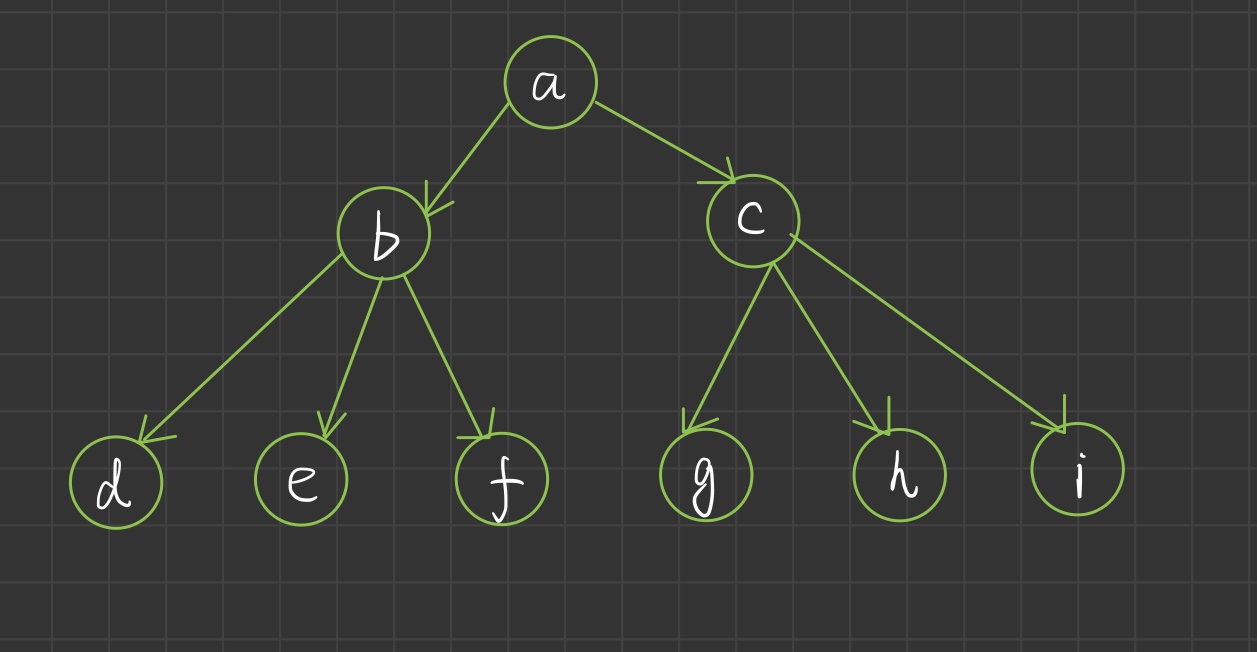
二、深度优先遍历
1、画图
2、代码实现
const { tree } = require('./tree_data')
const dfs = root => {
if(!root) return;
console.log(root.val);
root.children.forEach(child => {
dfs(child)
})
}
dfs(tree)
// a b d e f c g h i
三、树的广度优先遍历
1、画图
2、编码实现
const { tree } = require('./tree_data')
const bfs = root => {
if(!root) return;
const queue = [root];
while(queue.length) {
const top = queue.shift();
console.log(top.val);
top.children.forEach(child => {
queue.push(child)
})
}
}
bfs(tree)
// a b c d e f g h i
写完啦~
以上是关于JavaScript实现树的深度优先遍历和广度优先遍历的主要内容,如果未能解决你的问题,请参考以下文章