Java 学习笔记 - 集合运算
Posted 笑虾
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了Java 学习笔记 - 集合运算相关的知识,希望对你有一定的参考价值。
Java 学习笔记 - 集合运算
List.retainAll 交集
// 测试数据
List<Integer> listA = IntStream.rangeClosed(1,6).boxed().collect(Collectors.toList());
List<Integer> listB = IntStream.rangeClosed(4,10).boxed().collect(Collectors.toList());
// listA 交 listB。
boolean b = listA.retainAll(listB);
System.out.println(b); // 当 listA 发生改变返回 true
System.out.println(listA); // [4, 5, 6]
boolean b1 = listA.retainAll(listA);
System.out.println(b1); // 当 listA 没发生该变返回 false
System.out.println(listA); // [4, 5, 6]
CollectionUtils
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-collections4</artifactId>
<version>4.4</version>
</dependency>
// 测试数据
List<Integer> listA = IntStream.rangeClosed(1,6).boxed().collect(Collectors.toList());
List<Integer> listB = IntStream.rangeClosed(4,10).boxed().collect(Collectors.toList());
// 交集:保留两个集合中都有的元素
Collection<Integer> intersect1 = CollectionUtils.retainAll(listA, listB);
Collection<Integer> intersect2 = CollectionUtils.retainAll(listA, listB);
System.out.println(intersect1); // [4, 5, 6]
System.out.println(intersect2); // [4, 5, 6]
// 并集:合并两个集合
Collection<Integer> union1 = CollectionUtils.union(listA, listB);
Collection<Integer> union2 = CollectionUtils.union(listB, listA);
System.out.println(union1); // [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
System.out.println(union2); // [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
// (交集的)补集
// AB并集 - AB交集
Collection<Integer> disjunction1 = CollectionUtils.disjunction(listA, listB);
Collection<Integer> disjunction2 = CollectionUtils.disjunction(listB, listA);
System.out.println(disjunction1); // [1, 2, 3, 7, 8, 9, 10]
System.out.println(disjunction2); // [1, 2, 3, 7, 8, 9, 10]
//===================================================================
// 差集
// 去掉 A 中,勾结 B 的元素。(对比AB,保留仅在 A 中包含的元素)
Collection<Integer> except1 = CollectionUtils.subtract(listA, listB);
// 去掉 B 中,勾结 A 的元素。(对比AB,保留仅在 B 中包含的元素)
Collection<Integer> except2 = CollectionUtils.subtract(listB, listA);
System.out.println(except1); // [1, 2, 3]
System.out.println(except2); // [7, 8, 9, 10]
Java8 Stream
List<Integer> listA = IntStream.rangeClosed(1, 6).boxed().collect(Collectors.toList());
List<Integer> listB = IntStream.rangeClosed(4, 10).boxed().collect(Collectors.toList());
// 交集
List<Integer> intersection = listA.stream()
.filter(a -> listB.contains(a))
.collect(Collectors.toList());
System.out.println(intersection); // [4, 5, 6]
// 并集
List<Integer> union = Stream.concat(listA.stream(), listB.stream()).distinct().collect(Collectors.toList());
System.out.println(union); // [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
boolean b = listA.addAll(listB);
System.out.println(b); // 如果 listA 有改变 true
List<Integer> collect = listA.stream().distinct().collect(Collectors.toList());
System.out.println(collect); // [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
//===================================================================
// 差集
// 在 A 中去掉 B 也有的元素
List<Integer> subtraction = listA.stream()
.filter(a -> !listB.contains(a))
.collect(Collectors.toList());
System.out.println(subtraction); // [1, 2, 3]
// 在 B 中去掉 A 也有的元素
List<Integer> subtraction2 = listB.stream()
.filter(a -> !listA.contains(a))
.collect(Collectors.toList());
System.out.println(subtraction2); // [7, 8, 9, 10]
参考资料
List.retainAll (Java Platform SE 8 )
Apache Commons 集合工具类:commons-collections
java.util.stream.Stream (Java Platform SE 8 )
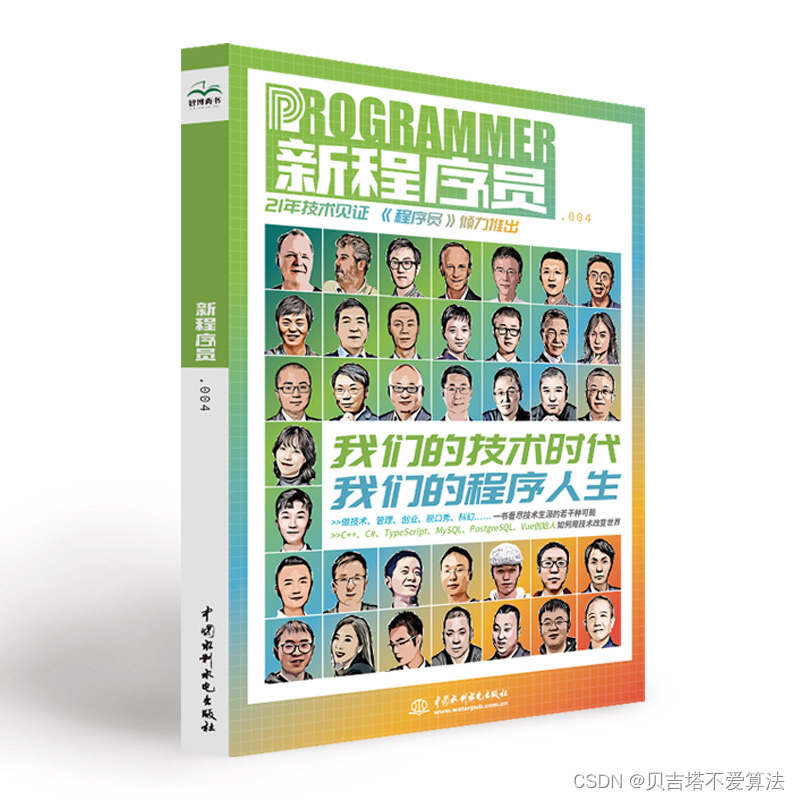

以上是关于Java 学习笔记 - 集合运算的主要内容,如果未能解决你的问题,请参考以下文章