经典排序算法——折半插入排序
Posted 代码民工
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了经典排序算法——折半插入排序相关的知识,希望对你有一定的参考价值。
15
①基本思想
②演示
③算法代码
④性能

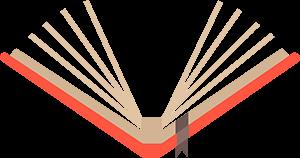
以数组a[8]={8、5、2、4、6、1、7、3}为例,以下仅展示前几次的排序思想
从第二个数据5开始向前插入,low=high=mid=0,a[mid]=8>temp,则high=mid-1=-1,插入点为a[high+1]=a[0]的位置,8后移一位
a[mid]>temp,high=mid-1=-1,插入点为a[high+1]=a[0]的位置,8和5向后移动一位
a[mid]>temp,high=mid-1=0,如下图
a[mid]<temp,low=mid+1=1,插入点为a[high+1]=a[1]的位置,8和5向后移动一位
a[mid]<temp,low=mid+1=2,如下图
a[mid]<temp,low=mid+1=3,如下图
a[mid]>temp,high=mid-1=2,插入点为a[high+1]=a[3]的位置,8向后移动一位
按照这种排序思想可将后面的数据依次进行排序,从而得到一个有序的数组

1#include<iostream>
2using namespace std;
3void BinInsertSort(int a[], int n)
4{
5 int i, j, low, high, mid, temp;
6 for (i = 1; i < n; i++)
7 {
8 temp = a[i];
9 low = 0; high = i-1;
10 while (low <= high)
11 {
12 mid = (low + high) / 2;
13 if (a[mid]>temp)
14 high = mid - 1;
15 else
16 low = mid + 1;
17 }
18 for (j = i - 1; j >= high+1; j-- )
19 {
20 a[j + 1] = a[j];
21 }
22 a[j+1] = temp;
23 }
24}
25int main()
26{
27 int a[8] = { 8,5,2,4,6,1,7,3 };
28 BinInsertSort(a, 8);
29 for (int i = 0; i < 8; i++)
30 {
31 cout << a[i] << " ";
32 }
33
34 return 0;
35}
1def BinInsertSort(nums):
2 for i in range(1,len(nums)):
3 temp=nums[i]
4 low=0
5 high=i-1
6 while low<=high:
7 mid=int((low+high)/2)
8 if nums[mid]>temp:
9 high=mid-1
10 else:low=mid+1
11 j=i-1
12 while j>=high+1:
13 nums[j+1]=nums[j]
14 j-=1
15 nums[j+1]=temp
16a=[8,5,2,4,6,1,7,3]
17BinInsertSort(a)
18print(a)
1package text;
2
3public class BinInsertSort {
4 public static void main(String[] args) {
5 int[] a={8,5,2,4,6,1,7,3};
6 BinInsertSort sorter=new BinInsertSort();
7 sorter.Sort(a);
8 sorter.ShowArr(a);
9 }
10 public void Sort(int a[]){
11 int i,j,low,high,mid,temp;
12 for(i=1;i<a.length;i++){
13 temp=a[i];
14 low=0;high=i-1;
15 while(low<=high){
16 mid=(low+high)/2;
17 if(a[mid]>temp)high=mid-1;
18 else low=mid+1;
19 }
20 for(j=i-1;j>=high+1;j--){
21 a[j+1]=a[j];
22 }
23 a[j+1]=temp;
24 }
25 }
26 public void ShowArr(int a[]){
27 for(int x:a){
28 System.out.print(x+" ");
29 }
30 System.out.println();
31 }
32
33}
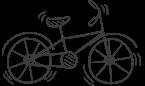
折半插入排序是一种稳定的排序算法,时间复杂度为O(N2),空间复杂度为O(1)
以上是关于经典排序算法——折半插入排序的主要内容,如果未能解决你的问题,请参考以下文章