Hadoop ---- MapReduce
Posted Java到大数据之路
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了Hadoop ---- MapReduce相关的知识,希望对你有一定的参考价值。
MapReduce 思想:分而治之
Map(分):在分的阶段,我们只需要提供Map阶段的逻辑就好,不需要关心原始数据切分几块,以及如何切分,还有使用什么方式读取等,也不需要关系并行任务分配及调度。
Reduce(合):无需关系如何获取Map阶段输出的结果,只需关心如何汇总结果。
WordCount源码分析
1/**
2* Map阶段的业务逻辑
3*/
4public static class TokenizerMapper extends Mapper<Object, Text, Text, IntWritable> {
5 private static final IntWritable one = new IntWritable(1);
6
7 private Text word = new Text();
8
9 /**
10 * Map阶段的业务逻辑就是定义在该方法
11 * @param key: 代指的是一行文本的偏移量
12 * @param value:一行文本
13 * @desc:map接收到一行文本,把文本按照指定分隔符切分,然后得到单词
14 * @desc:map最终输出 :<单词,1>
15 */
16 public void map(Object key, Text value, Mapper<Object, Text, Text, IntWritable>.Context context) throws IOException, InterruptedException {
17 StringTokenizer itr = new StringTokenizer(value.toString());
18 //迭代
19 while (itr.hasMoreTokens()) {
20 //将单词放到word的Text对象里面
21 this.word.set(itr.nextToken());
22 //map最终输出 :<单词,1>
23 context.write(this.word, one);
24 }
25 }
26 }
27
28/**
29* Reduce 阶段的业务逻辑
30**/
31 public static class IntSumReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
32 private IntWritable result = new IntWritable();
33
34 /**
35 * Reduce 阶段的业务逻辑就是在reduce方法
36 * @param key:map阶段的key(就是单词)
37 * @param values:就是迭代器
38 * @desc:数据在map阶段结束后,mr框架进行的shuffle操作
39 * @desc:value是一组key相同的value(map阶段输出的value)的集合
40 */
41 public void reduce(Text key, Iterable<IntWritable> values, Reducer<Text, IntWritable, Text, IntWritable>.Context context) throws IOException, InterruptedException {
42 int sum = 0;
43 for (IntWritable val : values)
44 sum += val.get();
45 this.result.set(sum);
46 context.write(key, this.result);
47 }
48 }
49
50
51public static void main(String[] args) throws Exception {
52 Configuration conf = new Configuration();
53 String[] otherArgs = (new GenericOptionsParser(conf, args)).getRemainingArgs();
54 if (otherArgs.length < 2) {
55 System.err.println("Usage: wordcount <in> [<in>...] <out>");
56 System.exit(2);
57 }
58 Job job = Job.getInstance(conf, "word count");
59 job.setJarByClass(WordCount.class);
60 job.setMapperClass(TokenizerMapper.class);
61 job.setCombinerClass(IntSumReducer.class);
62 job.setReducerClass(IntSumReducer.class);
63 job.setOutputKeyClass(Text.class);
64 job.setOutputValueClass(IntWritable.class);
65 // 以上都是获取job对象,并进行相关属性配置
66 for (int i = 0; i < otherArgs.length - 1; i++)
67 //设置任务输入路径
68 FileInputFormat.addInputPath(job, new Path(otherArgs[i]));
69 //设置任务输出路径
70 FileOutputFormat.setOutputPath(job, new Path(otherArgs[otherArgs.length - 1]));
71 // job.waitForCompletion(true) 提交任务
72 System.exit(job.waitForCompletion(true) ? 0 : 1);
73 }
74}
由上面源码可以看到,WordCount统计主要由三个部门组成
Mapper类
Reducer 类
运行作业的代码(dirver)
Mapper类继承了org.apache.hadoop.mapreduce.Mapper;类并重新其中map方法,作用:Map方法其中的逻辑mr程序map阶段的处理逻辑
Reducer继承了org.apache.hadoop.mapreduce.Reducer类并重新写了reduce方法,作用:reduce方法其中的逻辑就是reduce阶段的处理逻辑
hadoop序列化
为什么序列化?为了通过网络传输数据或对象持久化到文件时,需要把对象持久化成二进制的结构。
观察上面源码,发现Mapper和Reducer类都用泛型类型约束,例如Mapper
为什么Hadoop不使用Java的基本类型
序列化在分布式程序中非常重要,在Hadoop中,节点与节点通信是RPC,RPC将消息序列化成二进制发送到远程节点,远程节点收到后反序列化,所以RPC追求如下特点
紧凑:数据更紧凑,重复利用网络带宽
快速:序列化和反序列化的性能开销更低
Hadoop使用自己的序列化格式Writable,他比java的序列化更紧凑速度更快,一个对象使用Serializable序列化后,会携带很多额外的信息,如校验信息,Header,继承体系等
Java基本类型与Hadoop常用序列化类型
MapReduce 编程规范及事例编写
Mapper类
用户自定义一个Mapper类继承Hadoop的类
Mapper的输入数据是KV形式,如下代码,输入数据就是Object Text,即Mapper的泛型的前两个
Map的业务逻辑在map方法里面
Map的输出数据也是KV形式。输出数据就是,Text, IntWritable即Mapper的泛型的后两个,Map的输出数据格式,也是Reduce的输入数据格式
1/**
2* Map阶段的业务逻辑
3*/
4public static class TokenizerMapper extends Mapper<Object, Text, Text, IntWritable> {
5 private static final IntWritable one = new IntWritable(1);
6
7 private Text word = new Text();
8
9 /**
10 * Map阶段的业务逻辑就是定义在该方法
11 * @param key: 代指的是一行文本的偏移量
12 * @param value:一行文本
13 * @desc:map接收到一行文本,把文本按照指定分隔符切分,然后得到单词
14 * @desc:map最终输出 :<单词,1>
15 */
16 public void map(Object key, Text value, Mapper<Object, Text, Text, IntWritable>.Context context) throws IOException, InterruptedException {
17 StringTokenizer itr = new StringTokenizer(value.toString());
18 //迭代
19 while (itr.hasMoreTokens()) {
20 //将单词放到word的Text对象里面
21 this.word.set(itr.nextToken());
22 //map最终输出 :<单词,1>
23 context.write(this.word, one);
24 }
25 }
26 }
Reduce类
用户自定义reducer类继承Hadoop的Reducer类
Reducer的输入数据应用Map的输出数据类型(KV形式)
Reducer的业务逻辑主要在reduce()方法中
reduce()方法是对相同K的一组KV对调用执行过一次
Driver阶段
创建提交YARN集群运行的Job对象,其中封装了MapReduce程序运行所需要的相关参数输入输出路径,也相当于Yarn集群的客户端,主要作用是提交我们的MapReduce程序运行
WordCount代码编写
pom.xml
1<?xml version="1.0" encoding="UTF-8"?>
2<project xmlns="http://maven.apache.org/POM/4.0.0"
3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
4 xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
5 <modelVersion>4.0.0</modelVersion>
6
7 <groupId>com.hhb.hadoop</groupId>
8 <artifactId>com.hhb.hadoop</artifactId>
9 <version>1.0-SNAPSHOT</version>
10
11 <dependencies>
12 <dependency>
13 <groupId>junit</groupId>
14 <artifactId>junit</artifactId>
15 <version>RELEASE</version>
16 </dependency>
17 <dependency>
18 <groupId>org.apache.logging.log4j</groupId>
19 <artifactId>log4j-core</artifactId>
20 <version>2.8.2</version>
21 </dependency>
22
23 <dependency>
24 <groupId>org.apache.hadoop</groupId>
25 <artifactId>hadoop-common</artifactId>
26 <version>2.9.2</version>
27 </dependency>
28 <!-- https://mvnrepository.com/artifact/org.apache.hadoop/hadoop-client -->
29
30 <dependency>
31 <groupId>org.apache.hadoop</groupId>
32 <artifactId>hadoop-client</artifactId>
33 <version>2.9.2</version>
34 </dependency>
35
36 <!-- https://mvnrepository.com/artifact/org.apache.hadoop/hadoop-hdfs -->
37 <dependency>
38 <groupId>org.apache.hadoop</groupId>
39 <artifactId>hadoop-hdfs</artifactId>
40 <version>2.9.2</version>
41 </dependency>
42 </dependencies>
43
44 <!--maven打包插件 -->
45 <build>
46 <plugins>
47 <plugin>
48 <artifactId>maven-compiler-plugin</artifactId>
49 <version>2.3.2</version>
50 <configuration>
51 <source>1.8</source>
52 <target>1.8</target>
53 </configuration>
54 </plugin>
55 <plugin>
56 <artifactId>maven-assembly-plugin</artifactId>
57 <configuration>
58 <descriptorRefs>
59 <descriptorRef>jar-with-dependencies</descriptorRef>
60 </descriptorRefs>
61 </configuration>
62 <executions>
63 <execution>
64 <id>make-assembly</id>
65 <phase>package</phase>
66 <goals>
67 <goal>single</goal>
68 </goals>
69 </execution>
70 </executions>
71 </plugin>
72 </plugins>
73 </build>
74</project>
WordCountMapper.java
1package com.hhb.mapreduce.wc;
2
3import org.apache.hadoop.io.IntWritable;
4import org.apache.hadoop.io.LongWritable;
5import org.apache.hadoop.io.Text;
6import org.apache.hadoop.mapreduce.Mapper;
7
8import java.io.IOException;
9
10/**
11 * 需求:单词计数Map操作
12 *
13 * @author: huanghongbo
14 * @Date: 2020-07-04 18:29
15 * @Description: 操作步骤
16 * 1、继承Mapper
17 * 2、定义泛型,
18 * LongWritable, Text,输入参数的key,value,LongWritable为偏移量,Text一行文本,
19 * Text, IntWritable ,输出单数的key,value,key为单词,value :1 <单词,1>
20 * 3、重写Mapper的map方法
21 */
22public class WordCountMapper extends Mapper<LongWritable, Text, Text, IntWritable> {
23
24 //计数,输出的value
25 private IntWritable one = new IntWritable(1);
26 //单词,输出的key
27 private Text word = new Text();
28
29
30 @Override
31 protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
32 String str = value.toString();
33 String[] words = str.split(" ");
34 for (String s : words) {
35 word.set(s);
36 context.write(word, one);
37 }
38 }
39}
WordCountReducer.java
1package com.hhb.mapreduce.wc;
2
3import org.apache.hadoop.io.IntWritable;
4import org.apache.hadoop.io.Text;
5import org.apache.hadoop.mapreduce.Reducer;
6
7import java.io.IOException;
8
9/**
10 * 需求:单词计数Map操作
11 *
12 * @author: huanghongbo
13 * @Date: 2020-07-04 18:48
14 * @Description: 操作步骤
15 * 1、继承Reducer。
16 * 2、定义泛型,两个key、value
17 * 第一对key value为map的输出数据的类型
18 * 第二对就是reduce的输出数据的数据类型
19 * 3、重写reduce方法
20 */
21public class WordCountReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
22
23 private IntWritable total = new IntWritable();
24
25 /**
26 * 重新reduce方法,这里就是统计单词出现过几次
27 *
28 * @param key map的结果的key
29 * @param values map的结果的key对应的value组成的迭代器
30 * @param context
31 * @throws IOException
32 * @throws InterruptedException
33 * @Description : 如果map输出为hello 1,hello 1,hello 1,reduce 1,hadoop1,reduce 1
34 * 则:
35 * key:hello,values:<1,1,1>
36 * key:reduce,values:<1,1>
37 * key:hadoop1,values:<1>
38 * <p>
39 * reduce 被调用的次数:3次
40 */
41 @Override
42 protected void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
43 int sum = 0;
44 for (IntWritable value : values) {
45 sum += value.get();
46 }
47 total.set(sum);
48 context.write(key, total);
49 }
50}
WordCountDriver.java
1package com.hhb.mapreduce.wc;
2
3import org.apache.hadoop.conf.Configuration;
4import org.apache.hadoop.fs.Path;
5import org.apache.hadoop.io.IntWritable;
6import org.apache.hadoop.io.Text;
7import org.apache.hadoop.mapreduce.Job;
8import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
9import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
10
11import java.io.IOException;
12
13/**
14 * @author: huanghongbo
15 * @Date: 2020-07-04 19:27
16 * @Description: dirver类
17 * 1. 获取配置文件对象,获取job对象实例
18 * 2. 指定程序jar的本地路径
19 * 3. 指定Mapper/Reducer类
20 * 4. 指定Mapper输出的kv数据类型
21 * 5. 指定最终输出的kv数据类型
22 * 6. 指定job处理的原始数据路径
23 * 7. 指定job输出结果路径
24 * 8. 提交作业
25 */
26public class WordCountDriver {
27
28 public static void main(String[] args) throws IOException, ClassNotFoundException, InterruptedException {
29 //1. 获取配置文件对象,获取job对象实例
30 Configuration conf = new Configuration();
31 Job job = Job.getInstance(conf, "WordCountDriver");
32 //2. 指定程序jar的本地路径
33 job.setJarByClass(WordCountDriver.class);
34 //3. 指定Mapper/Reducer类
35 job.setMapperClass(WordCountMapper.class);
36 job.setReducerClass(WordCountReducer.class);
37 //4. 指定Mapper输出的kv数据类型
38 job.setMapOutputKeyClass(Text.class);
39 job.setMapOutputValueClass(IntWritable.class);
40 //5. 指定最终输出的kv数据类型
41 job.setOutputKeyClass(Text.class);
42 job.setOutputValueClass(IntWritable.class);
43 //6. 指定job处理的原始数据路径 /Users/baiwang/Desktop/hhb.txt
44 FileInputFormat.setInputPaths(job, new Path(args[0]));
45 //7. 指定job输出结果路径 /Users/baiwang/Desktop/hhb.txt
46 FileOutputFormat.setOutputPath(job, new Path(args[1]));
47 //8. 提交作业
48 boolean flag = job.waitForCompletion(true);
49 //0:表示JVM正常退出,1:表示JVM异常退出
50 System.exit(flag ? 0 : 1);
51 }
52
53}
该方式运行需要在配置args启动参数
1//在idea的启动配置里。Program arguments里添加
2/Users/baiwang/Desktop/hhb.txt /Users/baiwang/Desktop/hhb
查看结果
1/Users/baiwang/Desktop/hhb/part-r-00000
Yarn集群启动
打包后路径:/Users/baiwang/myproject/hadoop/target/com.hhb.hadoop-1.0-SNAPSHOT.jar
hadoop jar wc.jar com.lagou.wordcount.WordcountDriver /wcinput/wc.txt /wcouput
序列化Writable接口
实现Writable接口序列化的步骤
实现Writable接口
反序列化时,需要反射调用空参构造方法,必须有空参构造方法
重新序列化方法,write
重新发序列化方法 readFields
反序列化的字段顺序和序列化的字段顺序必须一致
方便展示结果,重新toString()方法
如果自定义的Bean对象需要放在Mapper的输出的kv的k,那么必须实现Comparable接口,应为MapReduce中的Shuffle过程要求对key排序
需求:统计每台智能音箱设备内容播放时长
原始日志格式:
1001 001577c3 kar_890809 120.196.100.99 1116 954 200
2日志id 设备id appkey(合作硬件厂商) 网络ip 自有内容时长(秒) 第三方内 容时长(秒) 网络状态码
输出结果
1001577c3 11160 9540 20700
2设备id 自有内容时长(秒) 第三方内容时长(秒) 总时长
自定义对象实现Writable接口
1package com.hhb.mapreduce.speak;
2
3import org.apache.hadoop.io.Writable;
4
5import java.io.DataInput;
6import java.io.DataOutput;
7import java.io.IOException;
8
9/**
10 * 输出结果:
11 * <p>
12 * 001577c3 11160 9540 20700
13 * 设备id 自有内容时长(秒) 第三方内容时长(秒) 总时长
14 *
15 * @author: huanghongbo
16 * @Date: 2020-07-05 10:38
17 * @Description:
18 */
19public class SpeakBean implements Writable {
20
21
22 /**
23 * 自有时长内容
24 */
25 private long selfDuration;
26 /**
27 * 第三方时长内容
28 */
29 private long thirdPartDuration;
30 /**
31 * 总时长内容
32 */
33 private long sumDuration;
34
35 public SpeakBean() {
36 }
37
38 public SpeakBean(long selfDuration, long thirdPartDuration) {
39 this.selfDuration = selfDuration;
40 this.thirdPartDuration = thirdPartDuration;
41 this.sumDuration = selfDuration + thirdPartDuration;
42 }
43
44 public long getSelfDuration() {
45 return selfDuration;
46 }
47
48 public SpeakBean setSelfDuration(long selfDuration) {
49 this.selfDuration = selfDuration;
50 return this;
51 }
52
53 public long getThirdPartDuration() {
54 return thirdPartDuration;
55 }
56
57 public SpeakBean setThirdPartDuration(long thirdPartDuration) {
58 this.thirdPartDuration = thirdPartDuration;
59 return this;
60 }
61
62 public long getSumDuration() {
63 return sumDuration;
64 }
65
66 public SpeakBean setSumDuration(long sumDuration) {
67 this.sumDuration = sumDuration;
68 return this;
69 }
70
71 /**
72 * 重新序列化方法
73 *
74 * @param dataOutput
75 * @throws IOException
76 */
77 @Override
78 public void write(DataOutput dataOutput) throws IOException {
79 dataOutput.writeLong(selfDuration);
80 dataOutput.writeLong(thirdPartDuration);
81 dataOutput.writeLong(sumDuration);
82 }
83
84 /**
85 * 重新反序列化方法
86 *
87 * @param dataInput
88 * @throws IOException
89 */
90 @Override
91 public void readFields(DataInput dataInput) throws IOException {
92 selfDuration = dataInput.readLong();
93 thirdPartDuration = dataInput.readLong();
94 sumDuration = dataInput.readLong();
95
96 }
97
98 @Override
99 public String toString() {
100 return selfDuration + " " + thirdPartDuration + " " + sumDuration;
101 }
102}
Mapper
1package com.hhb.mapreduce.speak;
2
3import org.apache.hadoop.io.LongWritable;
4import org.apache.hadoop.io.Text;
5import org.apache.hadoop.mapreduce.Mapper;
6
7import java.io.IOException;
8
9/**
10 * @author: huanghongbo
11 * @Date: 2020-07-05 10:37
12 * @Description:
13 */
14public class SpeakMapper extends Mapper<LongWritable, Text, Text, SpeakBean> {
15
16
17 private Text k = new Text();
18
19 private SpeakBean speakBean = new SpeakBean();
20
21 /**
22 * 文本格式
23 * 01 a00df6s kar 120.196.100.99 384 33 200
24 * 日志id 设备id appkey(合作硬件厂商) 网络ip 自有内容时长(秒) 第三方内 容时长(秒) 网络状态码
25 *
26 * @param key
27 * @param value
28 * @param context
29 * @throws IOException
30 * @throws InterruptedException
31 */
32 @Override
33 protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
34 //取出每行的数据
35 String[] fileds = value.toString().split(" ");
36 Long selfDuration = Long.valueOf(fileds[fileds.length - 3]);
37 Long thirdPartDuration = Long.valueOf(fileds[fileds.length - 2]);
38 speakBean.setSelfDuration(selfDuration);
39 speakBean.setThirdPartDuration(thirdPartDuration);
40 speakBean.setSumDuration(selfDuration + thirdPartDuration);
41 k.set(fileds[1]);
42 context.write(k, speakBean);
43 }
44}
Reducer
1package com.hhb.mapreduce.speak;
2
3import org.apache.hadoop.io.Text;
4import org.apache.hadoop.mapreduce.Reducer;
5
6import java.io.IOException;
7
8/**
9 * @author: huanghongbo
10 * @Date: 2020-07-05 10:54
11 * @Description:
12 */
13public class SpeakReducer extends Reducer<Text, SpeakBean, Text, SpeakBean> {
14
15 private SpeakBean speakBean = new SpeakBean();
16
17 @Override
18 protected void reduce(Text key, Iterable<SpeakBean> values, Context context) throws IOException, InterruptedException {
19
20 long selfDuration = 0L;
21 long thirdPartDuration = 0L;
22 for (SpeakBean speakBean : values) {
23 selfDuration += speakBean.getSelfDuration();
24 thirdPartDuration += speakBean.getThirdPartDuration();
25 }
26 speakBean.setSelfDuration(selfDuration);
27 speakBean.setThirdPartDuration(thirdPartDuration);
28 speakBean.setSumDuration(selfDuration + thirdPartDuration);
29 context.write(key, speakBean);
30 }
31}
Driver
1package com.hhb.mapreduce.speak;
2
3import org.apache.hadoop.conf.Configuration;
4import org.apache.hadoop.fs.Path;
5import org.apache.hadoop.io.Text;
6import org.apache.hadoop.mapreduce.Job;
7import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
8import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
9
10import java.io.IOException;
11
12/**
13 * @author: huanghongbo
14 * @Date: 2020-07-05 10:58
15 * @Description:
16 */
17public class SpeakDriver {
18
19
20 public static void main(String[] args) throws IOException, ClassNotFoundException, InterruptedException {
21 // 1. 获取配置文件对象,获取job对象实例
22 Configuration configuration = new Configuration();
23 Job job = Job.getInstance(configuration, "SpeakDriver");
24 // 2. 指定程序jar的本地路径
25 job.setJarByClass(SpeakDriver.class);
26 // 3. 指定Mapper/Reducer类
27 job.setMapperClass(SpeakMapper.class);
28 job.setReducerClass(SpeakReducer.class);
29 // 4. 指定Mapper输出的kv数据类型
30 job.setMapOutputKeyClass(Text.class);
31 job.setMapOutputValueClass(SpeakBean.class);
32 // 5. 指定最终输出的kv数据类型
33 job.setOutputKeyClass(Text.class);
34 job.setOutputValueClass(SpeakBean.class);
35 // 6. 指定job处理的原始数据路径
36 FileInputFormat.setInputPaths(job, new Path(args[0]));
37 // 7. 指定job输出结果路径
38 FileOutputFormat.setOutputPath(job, new Path(args[1]));
39 // 8. 提交作业
40 boolean flag = job.waitForCompletion(true);
41 System.exit(flag ? 0 : 1);
42
43 }
44}
mr编程技巧
结合业务设计Map输出的key和value,利用key相同则调用同一个reduce的特点
map方法获取只是一行为本数据,尽量不做聚合运算
reduce()方法的参数要清楚含义
MapReduce原理分析
MapTask运行机制
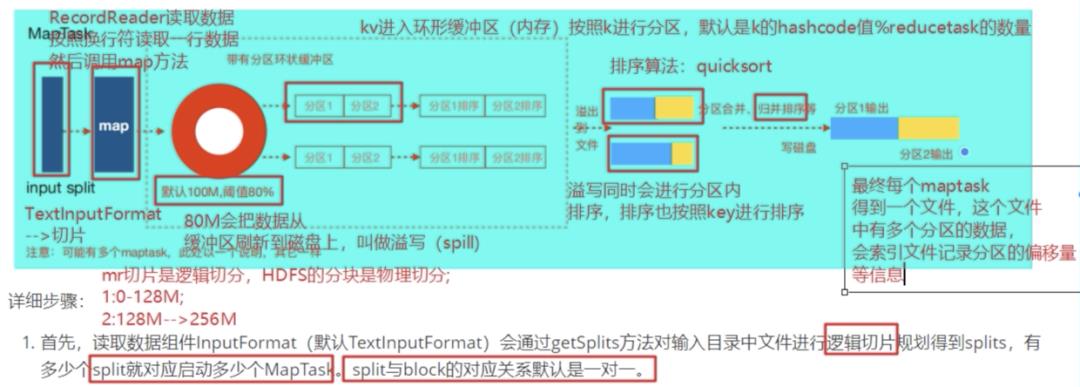
详细步骤:
首先,读取数据组建InputFormat(默认TextInputFormat)会通过getSplits方法对输入目录中的文件进行逻辑切片规划得到splits,有多少个split就有对应启动多少个MapTask,每片默认128M,split与block对应关系默认一对一。
将输入文件切分为splits之后,由RecordReader对象(默认LineRecordReader)进行读取,以 作为分隔符,读取一行数据,返回
。key表示没行字符偏移量。value表示这一行文本内容 读取split返回
,进入用户自己继承的Mapper类中,执行用户重写的map函数。RecordReader读取一行这里调用一次 map逻辑完,将map的每条结果通过context.write()进行collect数据收集。在collect中。会对去进行分区处理,默认使用HashPartioner,MapReduce提供Partitioner接口,他的作用就是根据key或value及reduce的数量来决定当前的这对输出数据最终应该交由那个ReduceTask处理,默认对 key hash后再议ReduceTask数量取模,默认的取模方式只是为了平均reduce的处理能力,如果用户对Partitioneer有需求,可以定制并设置到job上。
接下来,会叫数据写到内存中,内存中这片区域叫做环形缓冲区,缓冲区的作用就是批量收集map结果,减少磁盘IO。我们的key/value对以及Partition的结果都会被写入缓冲区。当然写入之 前,key与value值都会被序列化成字节数组。
环形缓冲区其实是一个数组,数组中存放着key、value的序列化数据和key、value的元数据信 息,包括partition、key的起始位置、value的起始位置以及value的长度。环形结构是一个抽象概 念。
缓冲区是有大小限制,默认是100MB。当map task的输出结果很多时,就可能会撑爆内存,所以 需要在一定条件下将缓冲区中的数据临时写入磁盘,然后重新利用这块缓冲区。这个从内存往磁盘 写数据的过程被称为Spill,中文可译为溢写。这个溢写是由单独线程来完成,不影响往缓冲区写 map结果的线程。溢写线程启动时不应该阻止map的结果输出,所以整个缓冲区有个溢写的比例 spill.percent。这个比例默认是0.8,也就是当缓冲区的数据已经达到阈值(buffer size * spill percent = 100MB * 0.8 = 80MB),溢写线程启动,锁定这80MB的内存,执行溢写过程。Map task的输出结果还可以往剩下的20MB内存中写,互不影响。
当溢写线程启动后,需要对这80MB空间内的key做排序(Sort)。排序是MapReduce模型默认的行为!
如果job设置过Combiner,那么现在就是使用Combiner的时候了。将有相同key的key/value对的 value加起来,减少溢写到磁盘的数据量。Combiner会优化MapReduce的中间结果,所以它在整 个模型中会多次使用。
那哪些场景才能使用Combiner呢?从这里分析,Combiner的输出是Reducer的输入,Combiner 绝不能改变最终的计算结果。Combiner只应该用于那种Reduce的输入key/value与输出key/value 类型完全一致,且不影响最终结果的场景。比如累加,最大值等。Combiner的使用一定得慎重, 如果用好,它对job执行效率有帮助,反之会影响reduce的最终结果。
合并溢写文件:每次溢写会在磁盘上生成一个临时文件(写之前判断是否有combiner),如果 map的输出结果真的很大,有多次这样的溢写发生,磁盘上相应的就会有多个临时文件存在。当 整个数据处理结束之后开始对磁盘中的临时文件进行merge合并,因为最终的文件只有一个,写入 磁盘,并且为这个文件提供了一个索引文件,以记录每个reduce对应数据的偏移量。
MapTask并行度
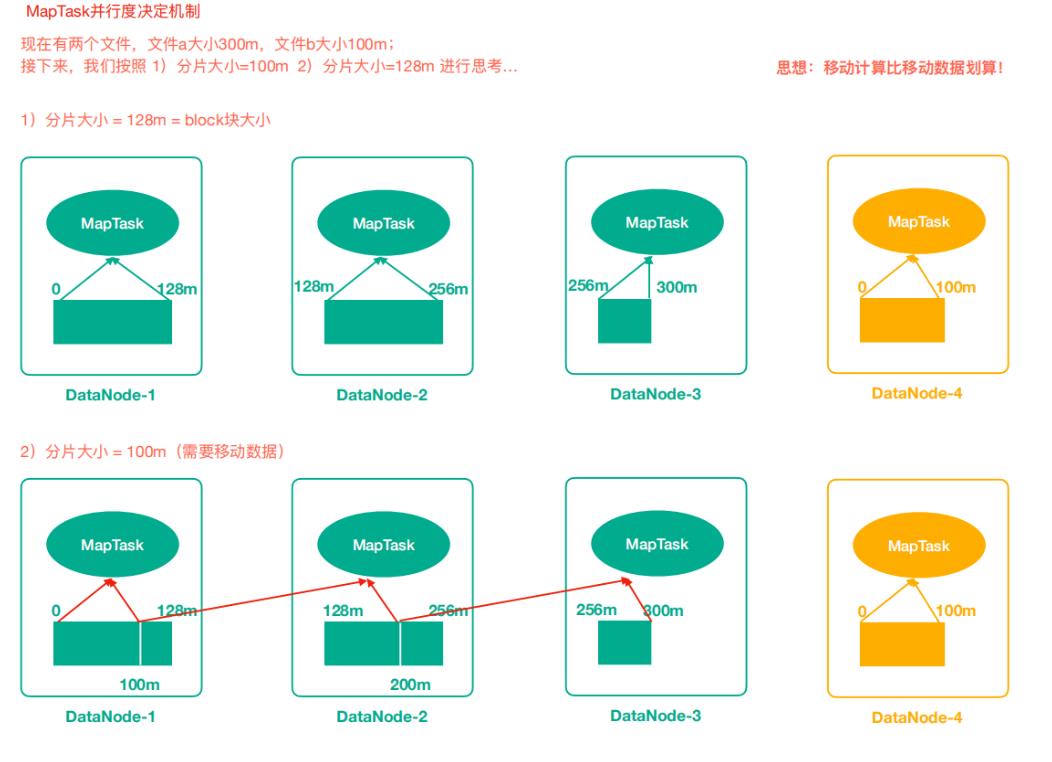
切片大小默认等于Block大小,splitSize = BlockSize,BlockSize = 128M;
问题:a文件300M,b文件100M,两个文件都存入到HDFS,并作为某个mr任务的输入数据,现在这个mr任务的split已经MapTask的并行度是多少?
切片的计算方式:按照文件逐个计算
a文件:0-128M,128-256M,256-300M
b文件:0-100M
总共是4个split,MapTask的并行度=4。
MapTask的并行度是不是越多越好?
不是的,如果一个文件比128M大一点点,也会被当成一个切片,这个大小的比例是1.1
MR框架在并行运算同时也会消耗更多的资源,并行度越高资源消耗越多,假设129M文件两个切片。一个128M一个1M,对于1M切片的MapTask来说, 太浪费资源。
切片源码解读,FileInputFarmat 的 getSplits方法
1/**
2 * Generate the list of files and make them into FileSplits.
3 * @param job the job context
4 * @throws IOException
5 */
6 public List<InputSplit> getSplits(JobContext job) throws IOException {
7 StopWatch sw = new StopWatch().start();
8 //获取切片的最小值, getFormatMinSplitSize()返回的是1, getMinSplitSize(job)返回的是0或1,所有minSize即切片的最小值为1
9 long minSize = Math.max(getFormatMinSplitSize(), getMinSplitSize(job));
10 //切片的最大值,Long的最大值
11 long maxSize = getMaxSplitSize(job);
12
13 // generate splits
14 List<InputSplit> splits = new ArrayList<InputSplit>();
15 //返回输入路径文件的状态信息,封装到FileStatus。
16 List<FileStatus> files = listStatus(job);
17 //循环,切片是针对文件一个一个计算处理,而不是累计计算
18 for (FileStatus file: files) {
19 //获取文件路径
20 Path path = file.getPath();
21 //获取文件大小
22 long length = file.getLen();
23 if (length != 0) {
24 //准备块文件信息
25 BlockLocation[] blkLocations;
26 if (file instanceof LocatedFileStatus) {
27 blkLocations = ((LocatedFileStatus) file).getBlockLocations();
28 } else {
29 FileSystem fs = path.getFileSystem(job.getConfiguration());
30 blkLocations = fs.getFileBlockLocations(file, 0, length);
31 }
32 //判断文件是否可切分
33 if (isSplitable(job, path)) {
34 //获取文件的块大小
35 long blockSize = file.getBlockSize();
36 //后去切片大小,返回的就是128M。
37 long splitSize = computeSplitSize(blockSize, minSize, maxSize);
38
39 long bytesRemaining = length;
40 // SPLIT_SLOP = 1.1,也就是说,如果一个文件是129M,129/128 是< 1.1
41 //这种情况走下面的if方法
42 while (((double) bytesRemaining)/splitSize > SPLIT_SLOP) {
43 //计算本次循环的开始偏移量,起始值:length = bytesRemaining,所以第一次为0
44 int blkIndex = getBlockIndex(blkLocations, length-bytesRemaining);
45 //切片,并添加到splits集合里面,
46 //path:路径,length-bytesRemaining:本次循环的开始偏移量;splitSize:片大小。128M
47 splits.add(makeSplit(path, length-bytesRemaining, splitSize,
48 blkLocations[blkIndex].getHosts(),
49 blkLocations[blkIndex].getCachedHosts()));
50 //bytesRemaining 减去本次切片出去的大小,方便计算下次循环的开始偏移量
51 bytesRemaining -= splitSize;
52 }
53 // 当((double) bytesRemaining)/splitSize < 1.1的时候。直接生成一个切片
54 if (bytesRemaining != 0) {
55 int blkIndex = getBlockIndex(blkLocations, length-bytesRemaining);
56 splits.add(makeSplit(path, length-bytesRemaining, bytesRemaining,
57 blkLocations[blkIndex].getHosts(),
58 blkLocations[blkIndex].getCachedHosts()));
59 }
60 } else { // not splitable
61 if (LOG.isDebugEnabled()) {
62 // Log only if the file is big enough to be splitted
63 if (length > Math.min(file.getBlockSize(), minSize)) {
64 LOG.debug("File is not splittable so no parallelization "
65 + "is possible: " + file.getPath());
66 }
67 }
68 splits.add(makeSplit(path, 0, length, blkLocations[0].getHosts(),
69 blkLocations[0].getCachedHosts()));
70 }
71 } else {
72 //Create empty hosts array for zero length files
73 splits.add(makeSplit(path, 0, length, new String[0]));
74 }
75 }
76 // Save the number of input files for metrics/loadgen
77 job.getConfiguration().setLong(NUM_INPUT_FILES, files.size());
78 sw.stop();
79 if (LOG.isDebugEnabled()) {
80 LOG.debug("Total # of splits generated by getSplits: " + splits.size()
81 + ", TimeTaken: " + sw.now(TimeUnit.MILLISECONDS));
82 }
83 return splits;
84 }
85
86
87
88 /**
89 *List input directories.
90 * Subclasses may override to, e.g., select only files matching a regular
91 * expression.
92 *
93 * @param job the job to list input paths for
94 * @return array of FileStatus objects
95 * @throws IOException if zero items.
96 */
97 protected List<FileStatus> listStatus(JobContext job
98 ) throws IOException {
99 //得到的是输入数据的路径
100 Path[] dirs = getInputPaths(job);
101 if (dirs.length == 0) {
102 throw new IOException("No input paths specified in job");
103 }
104
105 // 根据文件系统校验一下
106 TokenCache.obtainTokensForNamenodes(job.getCredentials(), dirs,
107 job.getConfiguration());
108
109 // 是否需要递归遍历目录下所有文件
110 boolean recursive = getInputDirRecursive(job);
111
112 // creates a MultiPathFilter with the hiddenFileFilter and the
113 // user provided one (if any).
114 //过滤器
115 List<PathFilter> filters = new ArrayList<PathFilter>();
116 filters.add(hiddenFileFilter);
117 PathFilter jobFilter = getInputPathFilter(job);
118 if (jobFilter != null) {
119 filters.add(jobFilter);
120 }
121 PathFilter inputFilter = new MultiPathFilter(filters);
122 //获取到所有输入文件的状态信息,大小,block块、
123 List<FileStatus> result = null;
124
125 int numThreads = job.getConfiguration().getInt(LIST_STATUS_NUM_THREADS,
126 DEFAULT_LIST_STATUS_NUM_THREADS);
127 StopWatch sw = new StopWatch().start();
128 if (numThreads == 1) {
129 result = singleThreadedListStatus(job, dirs, inputFilter, recursive);
130 } else {
131 Iterable<FileStatus> locatedFiles = null;
132 try {
133 LocatedFileStatusFetcher locatedFileStatusFetcher = new LocatedFileStatusFetcher(
134 job.getConfiguration(), dirs, recursive, inputFilter, true);
135 locatedFiles = locatedFileStatusFetcher.getFileStatuses();
136 } catch (InterruptedException e) {
137 throw new IOException("Interrupted while getting file statuses");
138 }
139 result = Lists.newArrayList(locatedFiles);
140 }
141
142 sw.stop();
143 if (LOG.isDebugEnabled()) {
144 LOG.debug("Time taken to get FileStatuses: "
145 + sw.now(TimeUnit.MILLISECONDS));
146 }
147 LOG.info("Total input files to process : " + result.size());
148 return result;
149 }
MapTask并行度取决于split的数量,而split的大小默认是Block大小,所以块的数量约等于分片的数量
ReduceTask工作机制
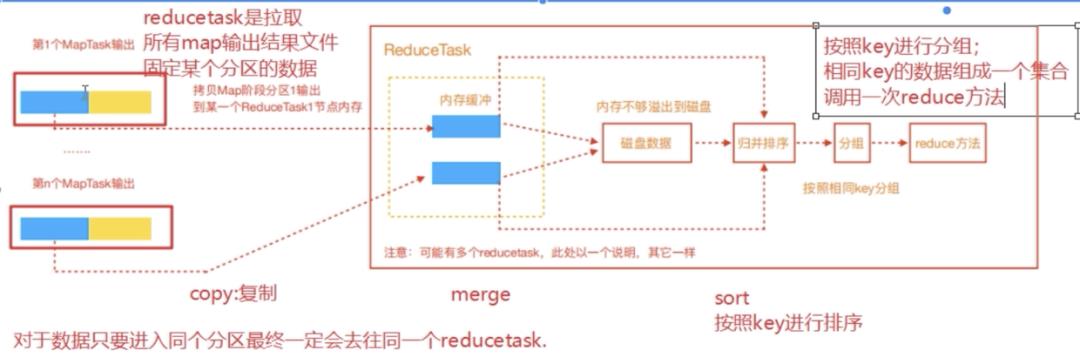
详细步骤
Copy阶段,简单地拉取数据。Reduce进程启动一些数据copy线程(Fetcher),通过HTTP方式请求 maptask获取属于自己的文件。
Merge阶段。这里的merge如map端的merge动作,只是数组中存放的是不同map端copy来的数 值。Copy过来的数据会先放入内存缓冲区中,这里的缓冲区大小要比map端的更为灵活。merge 有三种形式:内存到内存;内存到磁盘;磁盘到磁盘。默认情况下第一种形式不启用。当内存中的 数据量到达一定阈值,就启动内存到磁盘的merge。与map 端类似,这也是溢写的过程,这个过 程中如果你设置有Combiner,也是会启用的,然后在磁盘中生成了众多的溢写文件。第二种 merge方式一直在运行,直到没有map端的数据时才结束,然后启动第三种磁盘到磁盘的merge 方式生成最终的文件。
合并排序。把分散的数据合并成一个大的数据后,还会再对合并后的数据排序。
对排序后的键值对调用reduce方法,键相等的键值对调用一次reduce方法,每次调用会产生零个 或者多个键值对,最后把这些输出的键值对写入到HDFS文件中。
ReduceTask并行度
ReduceTask并行度同样影响整个Job的执行并发度和效率,但是与MapTask并行度有切片数决定不通。ReduceTask的数量是可以直接通过手动设置的
注意:
ReduceTask=0,表示没有Reduce阶段,输出文件数和MapTask数量保持一致
ReduceTask数量不设置默认是1,输出文件数量为1
如果数据分布不均匀,可能在Reduce阶段产生数据倾斜,就是某个ReduceTask处理的数据量远远大于其他节点,就是数据分配不均匀导致的。
Shuffle机制
map阶段处理的数据如何传递给reduce阶段,是MapReduce框架中最关键的一个流程,这个流程就叫shuffle。
shuffle: 洗牌、发牌——(核心机制:数据分区,排序,分组,combine,合并等过程)
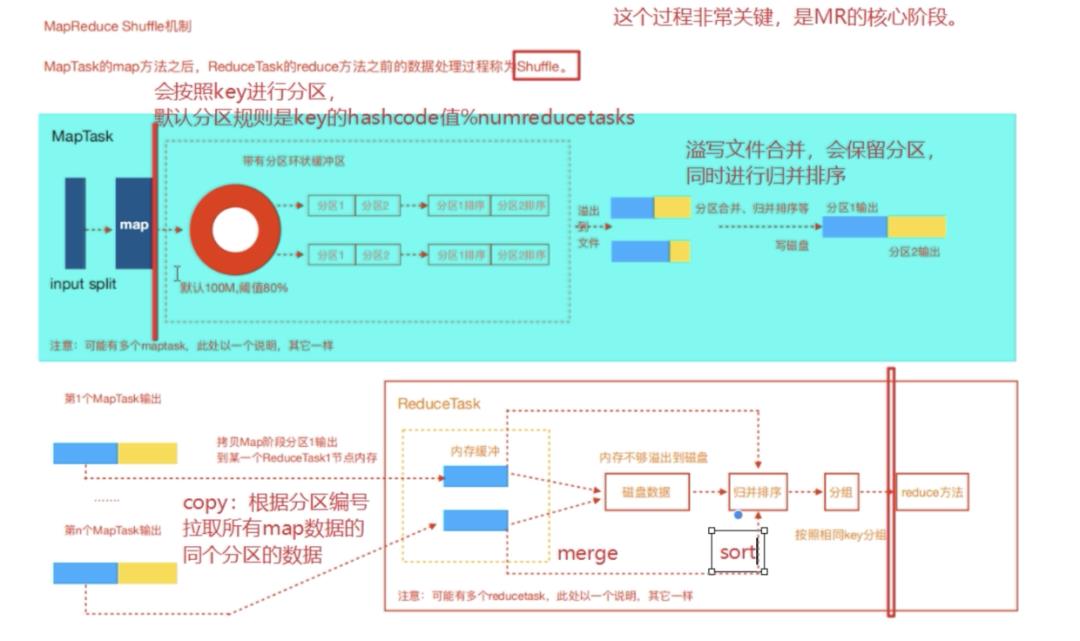
只要key相同,根据默认的shuffle机制,一定去的是同一个分区,也就是同一个ReduceTash
MapReduce的分区与reduceTash数量
在MapReduce中,我们通过指定分区,会将同一个分区的数据发送到同一个reduce中进行处理,默认是相同的key去同一个分区。
如何保证相同的key的数据去往同一个reduce呢?只需要保证相同key的数据分发到同一个区就可以了,也就是MapReduce的Shuffle机制默认的规则
源码分区:
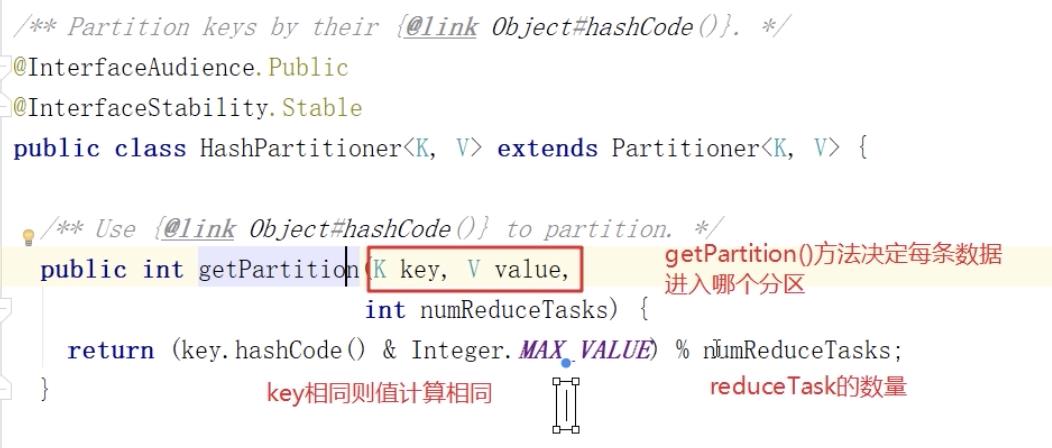
自定义分区
CustomPartition
1package com.hhb.mapreduce.partition;
2
3import org.apache.hadoop.io.Text;
4import org.apache.hadoop.mapreduce.Partitioner;
5
6/**
7 * @author: huanghongbo
8 * @Date: 2020-07-05 16:07
9 * @Description: 需求:
10 * 按照不同的appkey把记录输出到不同的分区中
11 * <p>
12 * 001 001577c3 kar_890809 120.196.100.99 1116 954 200
13 * 日志id 设备id appkey(合作硬件厂商) 网络ip 自有内容时长(秒) 第三方内 容时长(秒) 网络状态码
14 */
15public class CustomPartition extends Partitioner<Text, PartitionBean> {
16 @Override
17 public int getPartition(Text text, PartitionBean partitionBean, int numPartitions) {
18 if (text.toString().equals("kar")) {
19 return 1;
20 } else if (text.toString().equals("pandora")) {
21 return 2;
22 } else {
23 return 0;
24 }
25 }
26}
PartitionBean
1package com.hhb.mapreduce.partition;
2
3import org.apache.hadoop.io.Writable;
4import java.io.DataInput;
5import java.io.DataOutput;
6import java.io.IOException;
7
8/**
9 * @author: huanghongbo
10 * @Date: 2020-07-05 16:07
11 * @Description: 需求:
12 * * 按照不同的appkey把记录输出到不同的分区中
13 * * <p>
14 * * 001 001577c3 kar_890809 120.196.100.99 1116 954 200
15 * * 日志id 设备id appkey(合作硬件厂商) 网络ip 自有内容时长(秒) 第三方内 容时长(秒) 网络状态码
16 */
17public class PartitionBean implements Writable {
18
19
20 /**
21 * 日志ID
22 */
23 private String id;
24
25 /**
26 * 设别ID
27 */
28 private String deviceId;
29
30 /**
31 * appKey
32 */
33 private String appKey;
34
35 private String ip;
36
37 private String selfDuration;
38
39 private String thirdPartDuration;
40
41
42 public String getId() {
43 return id;
44 }
45
46 public PartitionBean setId(String id) {
47 this.id = id;
48 return this;
49 }
50
51 public String getDeviceId() {
52 return deviceId;
53 }
54
55 public PartitionBean setDeviceId(String deviceId) {
56 this.deviceId = deviceId;
57 return this;
58 }
59
60 public String getAppKey() {
61 return appKey;
62 }
63
64 public PartitionBean setAppKey(String appKey) {
65 this.appKey = appKey;
66 return this;
67 }
68
69 public String getIp() {
70 return ip;
71 }
72
73 public PartitionBean setIp(String ip) {
74 this.ip = ip;
75 return this;
76 }
77
78 public String getSelfDuration() {
79 return selfDuration;
80 }
81
82 public PartitionBean setSelfDuration(String selfDuration) {
83 this.selfDuration = selfDuration;
84 return this;
85 }
86
87 public String getThirdPartDuration() {
88 return thirdPartDuration;
89 }
90
91 public PartitionBean setThirdPartDuration(String thirdPartDuration) {
92 this.thirdPartDuration = thirdPartDuration;
93 return this;
94 }
95
96 @Override
97 public String toString() {
98 return id + " " + deviceId + " " + appKey + " " + ip + " " + selfDuration + " " + thirdPartDuration;
99 }
100
101 @Override
102 public void write(DataOutput output) throws IOException {
103 output.writeUTF(id);
104 output.writeUTF(deviceId);
105 output.writeUTF(appKey);
106 output.writeUTF(ip);
107 output.writeUTF(selfDuration);
108 output.writeUTF(thirdPartDuration);
109
110 }
111
112 @Override
113 public void readFields(DataInput input) throws IOException {
114 this.id = input.readUTF();
115 this.deviceId = input.readUTF();
116 this.appKey = input.readUTF();
117 this.ip = input.readUTF();
118 this.selfDuration = input.readUTF();
119 this.thirdPartDuration = input.readUTF();
120 }
121}
PartitionDriver
1package com.hhb.mapreduce.partition;
2
3import java.io.IOException;
4
5import org.apache.hadoop.conf.Configuration;
6import org.apache.hadoop.fs.Path;
7import org.apache.hadoop.io.Text;
8import org.apache.hadoop.mapreduce.Job;
9import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
10import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
11
12/**
13 * @author: huanghongbo
14 * @Date: 2020-07-05 16:07
15 * @Description: 需求:
16 * 按照不同的appkey把记录输出到不同的分区中
17 * <p>
18 * 001 001577c3 kar_890809 120.196.100.99 1116 954 200
19 * 日志id 设备id appkey(合作硬件厂商) 网络ip 自有内容时长(秒) 第三方内 容时长(秒) 网络状态码
20 */
21public class PartitionDriver {
22
23 public static void main(String[] args) throws IOException, ClassNotFoundException, InterruptedException {
24
25 // 1. 获取配置文件对象,获取job对象实例
26 Configuration configuration = new Configuration();
27 Job job = Job.getInstance(configuration, "PartitionDriver");
28 // 2. 指定程序jar的本地路径
29 job.setJarByClass(PartitionDriver.class);
30 // 3. 指定Mapper/Reducer类
31 job.setMapperClass(PartitionMapper.class);
32 job.setReducerClass(PartitionReducer.class);
33 // 4. 指定Mapper输出的kv数据类型
34 job.setMapOutputKeyClass(Text.class);
35 job.setMapOutputValueClass(PartitionBean.class);
36 // 5. 指定最终输出的kv数据类型
37 job.setOutputKeyClass(Text.class);
38 job.setOutputValueClass(PartitionBean.class);
39 // 6. 指定job处理的原始数据路径
40 FileInputFormat.setInputPaths(job, new Path(args[0]));
41 // 7. 指定job输出结果路径
42 FileOutputFormat.setOutputPath(job, new Path(args[1]));
43 // 设置ReduceTask数量
44 job.setNumReduceTasks(3);
45 // 设置自定义分区
46 job.setPartitionerClass(CustomPartition.class);
47 // 8. 提交作业
48 boolean flag = job.waitForCompletion(true);
49 System.exit(flag ? 0 : 1);
50 }
51}
PartitionMapper
1package com.hhb.mapreduce.partition;
2
3import org.apache.hadoop.io.LongWritable;
4import org.apache.hadoop.io.Text;
5import org.apache.hadoop.mapreduce.Mapper;
6
7import java.io.IOException;
8
9/**
10 * @author: huanghongbo
11 * @Date: 2020-07-05 16:07
12 * @Description: 需求:
13 * * 按照不同的appkey把记录输出到不同的分区中
14 * * <p>
15 * * 001 001577c3 kar_890809 120.196.100.99 1116 954 200
16 * * 日志id 设备id appkey(合作硬件厂商) 网络ip 自有内容时长(秒) 第三方内 容时长(秒) 网络状态码
17 */
18public class PartitionMapper extends Mapper<LongWritable, Text, Text, PartitionBean> {
19
20
21 private PartitionBean bean = new PartitionBean();
22
23 private Text text = new Text();
24
25 @Override
26 protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
27 String[] fileds = value.toString().split(" ");
28 bean.setId(fileds[0]);
29 bean.setDeviceId(fileds[1]);
30 bean.setAppKey(fileds[2]);
31 bean.setIp(fileds[3]);
32 bean.setSelfDuration(fileds[4]);
33 bean.setThirdPartDuration(fileds[5]);
34 text.set(fileds[2]);
35 context.write(text, bean);
36 }
37}
PartitionReducer
1package com.hhb.mapreduce.partition;
2
3import org.apache.hadoop.io.Text;
4import org.apache.hadoop.mapreduce.Reducer;
5
6import java.io.IOException;
7
8/**
9 * @author: huanghongbo
10 * @Date: 2020-07-05 16:07
11 * @Description: 需求:
12 * 按照不同的appkey把记录输出到不同的分区中
13 * <p>
14 * 001 001577c3 kar_890809 120.196.100.99 1116 954 200
15 * 日志id 设备id appkey(合作硬件厂商) 网络ip 自有内容时长(秒) 第三方内 容时长(秒) 网络状态码
16 */
17public class PartitionReducer extends Reducer<Text, PartitionBean, Text, PartitionBean> {
18
19
20 @Override
21 protected void reduce(Text key, Iterable<PartitionBean> values, Context context) throws IOException, InterruptedException {
22 for (PartitionBean value : values) {
23 context.write(key, value);
24 }
25 }
26}
总结:
自定义分区数量最好保证分区数量与ReduceTask数量保持一致
如果分区数量不止一个,但是ReduceTask数量1个,此时只会输出一个文件
如果ReduceTask大于分区数量,假设reduceTask为5,此时会输出5个文件,但是有三个文件是空的。
如果ReduceTask小于分区数量,可能会报错。
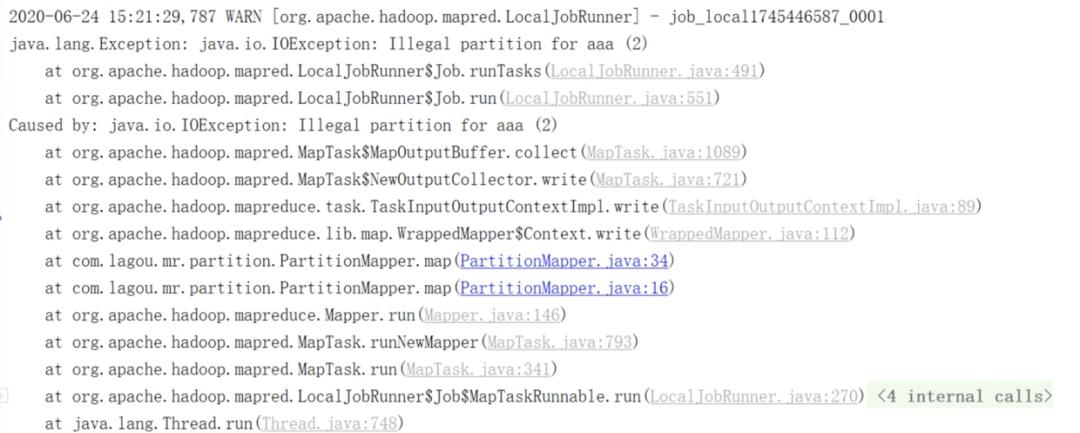
MapReduce中的Combiner
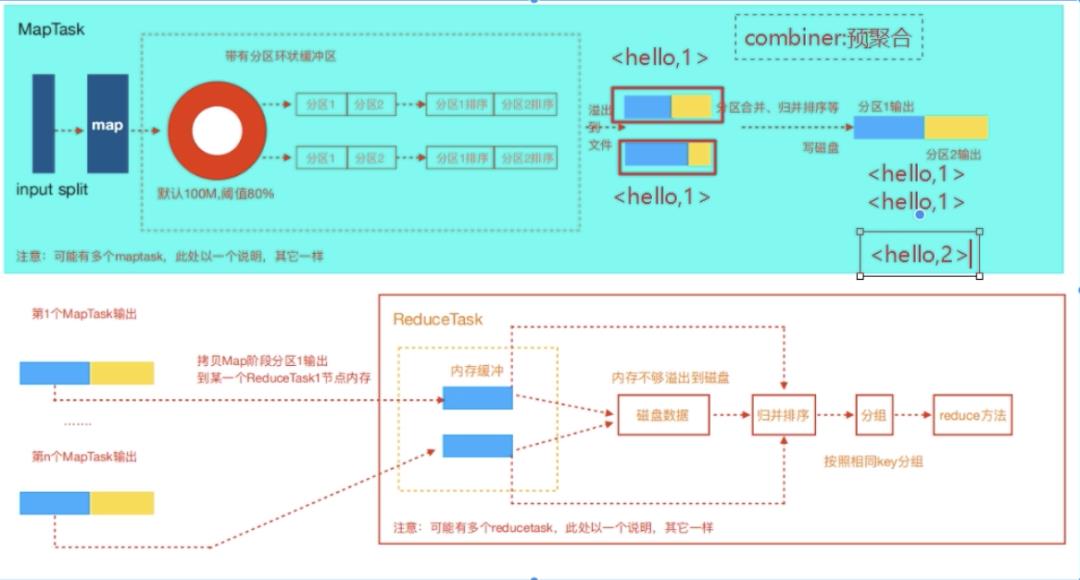
Combiner 是MR程序中Mapper和Reducer之外的一种组件
Combiner组件的父类就是Reducer
Combiner和Reducer的区别在于运行的位置
Combiner是在每一个MapTask的输出进行局部汇总,以减小网络传输量
Combineer能够应用的前提是不能影响最终的业务逻辑,此外,Combiner的输出kv应该跟Reducer的输入的kv类型对应起来
Combiner默认是不使用的,使用的前提就是不能影响最终的运行结果,例如计算平均数,就会影响到最终结果
实例:
wc增加WordCountCombiner
1package com.hhb.mapreduce.combiner;
2
3import org.apache.hadoop.io.IntWritable;
4import org.apache.hadoop.io.Text;
5import org.apache.hadoop.mapreduce.Reducer;
6
7import java.io.IOException;
8
9/**
10 * @author: huanghongbo
11 * @Date: 2020-07-05 17:26
12 * @Description:
13 */
14public class WordCountCombiner extends Reducer<Text, IntWritable, Text, IntWritable> {
15
16
17 private IntWritable total = new IntWritable();
18
19 @Override
20 protected void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
21 int sum = 0;
22 for (IntWritable value : values) {
23 sum += value.get();
24 }
25 total.set(sum);
26 context.write(key, total);
27 }
28}
驱动类:
1package com.hhb.mapreduce.combiner;
2
3import org.apache.hadoop.conf.Configuration;
4import org.apache.hadoop.fs.Path;
5import org.apache.hadoop.io.IntWritable;
6import org.apache.hadoop.io.Text;
7import org.apache.hadoop.mapreduce.Job;
8import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
9import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
10
11import java.io.IOException;
12
13/**
14 * @author: huanghongbo
15 * @Date: 2020-07-04 19:27
16 * @Description: dirver类
17 * 1. 获取配置文件对象,获取job对象实例
18 * 2. 指定程序jar的本地路径
19 * 3. 指定Mapper/Reducer类
20 * 4. 指定Mapper输出的kv数据类型
21 * 5. 指定最终输出的kv数据类型
22 * 6. 指定job处理的原始数据路径
23 * 7. 指定job输出结果路径
24 * 8. 提交作业
25 */
26public class WordCountDriver {
27
28 public static void main(String[] args) throws IOException, ClassNotFoundException, InterruptedException {
29 //1. 获取配置文件对象,获取job对象实例
30 Configuration conf = new Configuration();
31 Job job = Job.getInstance(conf, "WordCountDriver");
32 //2. 指定程序jar的本地路径
33 job.setJarByClass(WordCountDriver.class);
34 //3. 指定Mapper/Reducer类
35 job.setMapperClass(WordCountMapper.class);
36 job.setReducerClass(WordCountReducer.class);
37 //4. 指定Mapper输出的kv数据类型
38 job.setMapOutputKeyClass(Text.class);
39 job.setMapOutputValueClass(IntWritable.class);
40 //5. 指定最终输出的kv数据类型
41 job.setOutputKeyClass(Text.class);
42 job.setOutputValueClass(IntWritable.class);
43
44 //5.1 设置Combiner
45 //如果不设置Combiner,控制台里面输出:
46 // Combine input records=0
47 // Combine output records=0
48 //设置Combiner,控制台里面输出:
49 // Combine input records=11
50 // Combine output records=8
51 //WordCountCombiner 其实和 WordCountReducer 可以改为:
52 // job.setCombinerClass(WordCountReducer.class);
53 job.setCombinerClass(WordCountCombiner.class);
54 //6. 指定job处理的原始数据路径 /Users/baiwang/Desktop/hhb.txt
55 FileInputFormat.setInputPaths(job, new Path(args[0]));
56 //7. 指定job输出结果路径 /Users/baiwang/Desktop/hhb.txt
57 FileOutputFormat.setOutputPath(job, new Path(args[1]));
58 //8. 提交作业
59 boolean flag = job.waitForCompletion(true);
60 //0:表示JVM正常退出,1:表示JVM异常退出
61 System.exit(flag ? 0 : 1);
62 }
63}
MapReduce中的排序
MapTask和ReduceTask均会对数据按照key排序,该操作属于Hadoop默认行为,任何应用程序中的数据均会被排序,而不管业务逻辑是否需要,默认的排序就是按照字典顺序排序,且实现排序的方式是快速排序。
MapTask
它会在处理结果暂时放在缓形缓冲区中,当环形缓冲区使用率达到一定阈值后,在对缓冲区中的数据进行一次快速排序,并将这些有序结果溢写到磁盘上
溢写完毕后,它会对磁盘所有的文件进行归并排序
ReduceTask当所有数据拷贝完成后,ReduceTask统一对内存和磁盘的所有的数据进行一次归并排序
部分排序
MapReduce根据输入记录的健对数据排序,保证输出的每个文件内部有序
全排序
最终输出结果只有一个文件,且文件内部有序。实现方式是只设置- -个ReduceTask。但该方法在 处理大型文件时效率极低,因为- -台机器处理所有文件,完全丧失了MapReduce所提供的并行架 构。
辅助排序
在Reduce端对key进行分组。应用于:在接收的key为bean对象时,想让一个或几个字段相同(全部 字段比较不相同)的key进入到同一个reduce方法时,可以采用分组排序。
二次排序
在自定义排序过程中,如果compareTo中的判断条件为两个即为二次排序。
全排序
代码:
SortBean:
1package com.hhb.mapreduce.sort;
2
3import org.apache.hadoop.io.WritableComparable;
4
5import java.io.DataInput;
6import java.io.DataOutput;
7import java.io.IOException;
8
9/**
10 * @author: huanghongbo
11 * @Date: 2020-07-06 12:50
12 * @Description:
13 */
14public class SortBean implements WritableComparable<SortBean> {
15
16
17 private String id;
18
19 /**
20 * 自有时长内容
21 */
22 private long selfDuration;
23 /**
24 * 第三方时长内容
25 */
26 private long thirdPartDuration;
27 /**
28 * 总时长内容
29 */
30 private long sumDuration;
31
32 @Override
33 public int compareTo(SortBean o) {
34 if (sumDuration > o.getSumDuration()) {
35 return -1;
36 // 当加入第二个判断条件的时候,就是二次排序
37 } else if (sumDuration == o.getSumDuration()) {
38 return 0;
39 } else {
40 return 1;
41 }
42
43 }
44
45 @Override
46 public void write(DataOutput dataOutput) throws IOException {
47 dataOutput.writeUTF(id);
48 dataOutput.writeLong(selfDuration);
49 dataOutput.writeLong(thirdPartDuration);
50 dataOutput.writeLong(sumDuration);
51 }
52
53 @Override
54 public void readFields(DataInput dataInput) throws IOException {
55 this.id = dataInput.readUTF();
56 this.selfDuration = dataInput.readLong();
57 thirdPartDuration = dataInput.readLong();
58 sumDuration = dataInput.readLong();
59 }
60
61
62 public String getId() {
63 return id;
64 }
65
66 public SortBean setId(String id) {
67 this.id = id;
68 return this;
69 }
70
71 public long getSelfDuration() {
72 return selfDuration;
73 }
74
75 public SortBean setSelfDuration(long selfDuration) {
76 this.selfDuration = selfDuration;
77 return this;
78 }
79
80 public long getThirdPartDuration() {
81 return thirdPartDuration;
82 }
83
84 public SortBean setThirdPartDuration(long thirdPartDuration) {
85 this.thirdPartDuration = thirdPartDuration;
86 return this;
87 }
88
89 public long getSumDuration() {
90 return sumDuration;
91 }
92
93 public SortBean setSumDuration(long sumDuration) {
94 this.sumDuration = sumDuration;
95 return this;
96 }
97
98 @Override
99 public String toString() {
100 return id + " " + selfDuration + " " + thirdPartDuration + " " + sumDuration;
101 }
102}
SortMapper
1package com.hhb.mapreduce.sort;
2
3import org.apache.hadoop.io.LongWritable;
4import org.apache.hadoop.io.NullWritable;
5import org.apache.hadoop.io.Text;
6import org.apache.hadoop.mapreduce.Mapper;
7
8import java.io.IOException;
9
10/**
11 * @author: huanghongbo
12 * @Date: 2020-07-06 12:52
13 * @Description:
14 */
15public class SortMapper extends Mapper<LongWritable, Text, SortBean, NullWritable> {
16
17
18 private SortBean sortBean = new SortBean();
19
20 @Override
21 protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
22
23 String[] fileds = value.toString().split(" ");
24 sortBean.setId(fileds[0]);
25 sortBean.setSelfDuration(Long.parseLong(fileds[1]));
26 sortBean.setThirdPartDuration(Long.parseLong(fileds[2]));
27 sortBean.setSumDuration(Long.parseLong(fileds[3]));
28
29 context.write(sortBean, NullWritable.get());
30 }
31}
SortReducer
1package com.hhb.mapreduce.sort;
2
3import org.apache.hadoop.io.NullWritable;
4import org.apache.hadoop.mapreduce.Reducer;
5
6import java.io.IOException;
7
8/**
9 * @author: huanghongbo
10 * @Date: 2020-07-06 12:57
11 * @Description:
12 */
13public class SortReducer extends Reducer<SortBean, NullWritable, SortBean, NullWritable> {
14
15 @Override
16 protected void reduce(SortBean key, Iterable<NullWritable> values, Context context) throws IOException, InterruptedException {
17 //有可能存在总时长一样,导致合并成一个key,所以遍历values
18 for (NullWritable value : values) {
19 context.write(key, value);
20 }
21 }
22}
SortDriver
1package com.hhb.mapreduce.sort;
2
3import org.apache.hadoop.conf.Configuration;
4import org.apache.hadoop.fs.Path;
5import org.apache.hadoop.io.NullWritable;
6import org.apache.hadoop.mapreduce.Job;
7import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
8import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
9
10
11import java.io.IOException;
12
13/**
14 * @author: huanghongbo
15 * @Date: 2020-07-06 12:59
16 * @Description:
17 */
18public class SortDriver {
19
20
21 public static void main(String[] args) throws IOException, ClassNotFoundException, InterruptedException {
22
23
24 Configuration configuration = new Configuration();
25 Job job = Job.getInstance(configuration, "SortDriver");
26 job.setJarByClass(SortDriver.class);
27 job.setMapperClass(SortMapper.class);
28 job.setReducerClass(SortReducer.class);
29 job.setMapOutputKeyClass(SortBean.class);
30 job.setMapOutputValueClass(NullWritable.class);
31 job.setOutputKeyClass(SortBean.class);
32 job.setOutputValueClass(NullWritable.class);
33 job.setNumReduceTasks(1);
34 FileInputFormat.setInputPaths(job, new Path("/Users/baiwang/myproject/hadoop/src/main/data/mr-writable案例/输出"));
35 FileOutputFormat.setOutputPath(job, new Path("/Users/baiwang/myproject/hadoop/src/main/data/mr-writable案例/输出/sortoutput"));
36 boolean flag = job.waitForCompletion(true);
37 System.exit(flag ? 0 : 1);
38
39 }
40}
总结:
自定义对象作为Map的key输出的时候,需要实现WritableComparable接口,重新方法
再次理解reduce方法,reduce方法是map输出kv中的key相同的kv中v组成的集合。调用一次reduce方法,遍历所有的values得到所有的key
默认的reduceTask数量是一个
对于全排序,ReduceTask只有一个
分区排序(默认的分区规则,区内有序)
GroupingComparator
GroupingComparator是MapReduce当中reduce端的一个功能组件,主要的作用是决定那些数据作为一组,调用一次reduce逻辑,默认是不同的key,做为不同的组,每个组调用一次reduce逻辑。我们可以自定义GroupingComparator实现不同的key在同一个组
原始数据
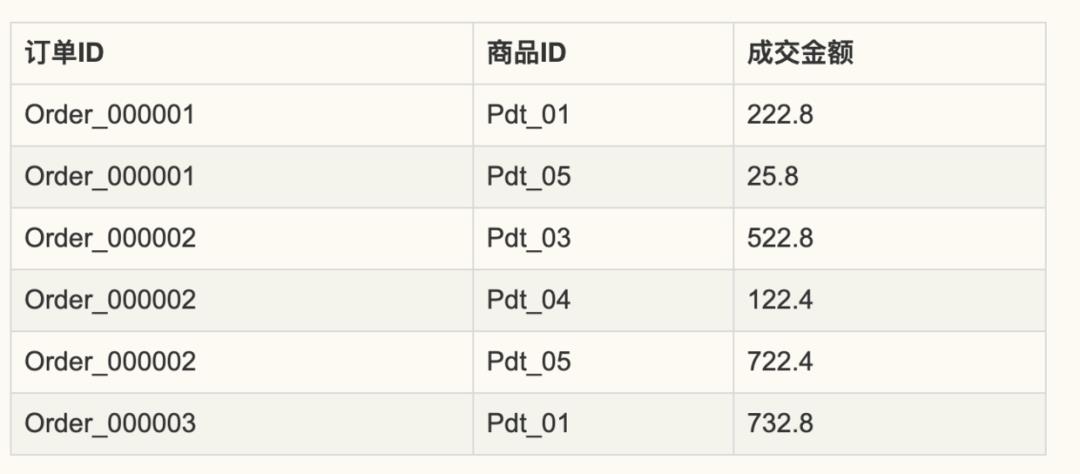
需要求出每一个订单中成交金额最大的一笔交易。
实现思路:
Mapper
读取指定的一行文本,切分字段
将订单ID和金额封装为Bean对象,Bean对象的排序规则指定为先按照订单ID排序,订单ID相同在按照金额降序。
map方法输出,bean对象
Shuffle
指定分区器,保证相同ID去同一个分区
Reduce
每个reduce方法写出一组key的第一个
GroupingComparator
保证一个分区里面的相同的key 的 Id去同一个分组
GroupBean:
1package com.hhb.mapreduce.group;
2
3import org.apache.hadoop.io.WritableComparable;
4
5import java.io.DataInput;
6import java.io.DataOutput;
7import java.io.IOException;
8
9/**
10 * @author: huanghongbo
11 * @Date: 2020-07-06 13:53
12 * @Description:
13 */
14public class GroupBean implements WritableComparable<GroupBean> {
15
16 private String id;
17
18 private Double price;
19
20 public String getId() {
21 return id;
22 }
23
24 public GroupBean setId(String id) {
25 this.id = id;
26 return this;
27 }
28
29 public Double getPrice() {
30 return price;
31 }
32
33 public GroupBean setPrice(Double price) {
34 this.price = price;
35 return this;
36 }
37
38 @Override
39 public int compareTo(GroupBean o) {
40 if (price > o.getPrice()) {
41 return -1;
42 } else if (price < o.getPrice()) {
43 return 1;
44 }
45 return 0;
46// int result = id.compareTo(o.getId());
47// if (result == 0) {
48// result = -price.compareTo(o.getPrice());
49// }
50// return result;
51 }
52
53 @Override
54 public void write(DataOutput dataOutput) throws IOException {
55 dataOutput.writeUTF(id);
56 dataOutput.writeDouble(price);
57 }
58
59 @Override
60 public void readFields(DataInput dataInput) throws IOException {
61 id = dataInput.readUTF();
62 price = dataInput.readDouble();
63 }
64
65 @Override
66 public String toString() {
67 return id + " " + price;
68 }
69}
CustomGroupPartition:
1package com.hhb.mapreduce.group;
2
3import org.apache.hadoop.io.NullWritable;
4import org.apache.hadoop.mapreduce.Partitioner;
5
6
7/**
8 * @author: huanghongbo
9 * @Date: 2020-07-06 14:07
10 * @Description:
11 */
12public class CustomGroupPartition extends Partitioner<GroupBean, NullWritable> {
13
14 @Override
15 public int getPartition(GroupBean groupBean, NullWritable nullWritable, int numPartitions) {
16 return (groupBean.getId().hashCode() & Integer.MAX_VALUE) % numPartitions;
17 }
18}
CustomGroupingComparator:
1package com.hhb.mapreduce.group;
2
3import org.apache.hadoop.io.WritableComparable;
4import org.apache.hadoop.io.WritableComparator;
5
6/**
7 * @author: huanghongbo
8 * @Date: 2020-07-06 14:12
9 * @Description:
10 */
11public class CustomGroupingComparator extends WritableComparator {
12
13 public CustomGroupingComparator() {
14 super(GroupBean.class, true);
15 }
16
17 @Override
18 public int compare(WritableComparable a, WritableComparable b) {
19 GroupBean a1 = (GroupBean) a;
20 GroupBean b1 = (GroupBean) b;
21 return a1.getId().compareTo(b1.getId());
22 }
23}
GroupMapper:
1package com.hhb.mapreduce.group;
2
3import org.apache.hadoop.io.LongWritable;
4import org.apache.hadoop.io.NullWritable;
5import org.apache.hadoop.io.Text;
6import org.apache.hadoop.mapreduce.Mapper;
7
8import java.io.IOException;
9
10
11/**
12 * @author: huanghongbo
13 * @Date: 2020-07-06 13:56
14 * @Description:
15 */
16public class GroupMapper extends Mapper<LongWritable, Text, GroupBean, NullWritable> {
17
18 private GroupBean groupBean = new GroupBean();
19
20
21 @Override
22 protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
23 String[] fileds = value.toString().split(" ");
24 groupBean.setId(fileds[0]);
25 groupBean.setPrice(Double.parseDouble(fileds[2]));
26 context.write(groupBean, NullWritable.get());
27 }
28}
GroupReduce:
1package com.hhb.mapreduce.group;
2
3import org.apache.hadoop.io.NullWritable;
4import org.apache.hadoop.mapreduce.Reducer;
5
6import java.io.IOException;
7
8/**
9 * @author: huanghongbo
10 * @Date: 2020-07-06 13:59
11 * @Description:
12 */
13public class GroupReducer extends Reducer<GroupBean, NullWritable, GroupBean, NullWritable> {
14
15 private int index = 0;
16
17 @Override
18 protected void reduce(GroupBean key, Iterable<NullWritable> values, Context context) throws IOException, InterruptedException {
19 index++;
20 System.err.println("这是第: " + index + "次进入reduce");
21 int i = 0;
22 for (NullWritable value : values) {
23 System.err.println(key.toString());
24 if (i == 0) {
25 context.write(key, NullWritable.get());
26 }
27 i++;
28 }
29
30 }
31}
GroupDriver:
1package com.hhb.mapreduce.group;
2
3import org.apache.hadoop.conf.Configuration;
4import org.apache.hadoop.fs.Path;
5import org.apache.hadoop.io.NullWritable;
6import org.apache.hadoop.mapreduce.Job;
7import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
8import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
9
10import java.io.IOException;
11
12/**
13 * @author: huanghongbo
14 * @Date: 2020-07-06 14:03
15 * @Description:
16 */
17public class GroupDriver {
18
19
20 public static void main(String[] args) throws IOException, ClassNotFoundException, InterruptedException {
21 Configuration configuration = new Configuration();
22 Job job = Job.getInstance(configuration, "GroupDriver");
23 job.setJarByClass(GroupDriver.class);
24 job.setMapperClass(GroupMapper.class);
25 job.setReducerClass(GroupReducer.class);
26 job.setMapOutputKeyClass(GroupBean.class);
27 job.setMapOutputValueClass(NullWritable.class);
28 job.setOutputKeyClass(GroupBean.class);
29 job.setOutputValueClass(NullWritable.class);
30 job.setPartitionerClass(CustomGroupPartition.class);
31 job.setNumReduceTasks(2);
32 job.setGroupingComparatorClass(CustomGroupingComparator.class);
33 FileInputFormat.setInputPaths(job, new Path("/Users/baiwang/myproject/hadoop/src/main/data/GroupingComparator"));
34 FileOutputFormat.setOutputPath(job, new Path("/Users/baiwang/myproject/hadoop/src/main/data/GroupingComparator/out"));
35 boolean flag = job.waitForCompletion(true);
36 System.exit(flag ? 0 : 1);
37 }
38}
MapReduce读取和输出数据
InputFormat
常见的子类:
TextInputFormat(普通文件文件,默认读取方式)map输入默认的key是LongWritable,偏移量
KeyValueInputFormat(也是普通文本。但是是读取一行数据,按照指定的分隔符,把数据封装成kv),默认的key就是就是一行的第一个参数,value就是第二个参数。
NLineInputFormat(读取数据按照行进行划分分片)
CombineTextInputFormat(合并小文件,避免启动过多MapTask任务)
自定义InputFormat
CombineTextInputFormat案例
MR框架默认的TextInputFormat切片机制按文件划分切片,文件无论多小,都是单独一个切片,然后有MapTask处理,如果有大量小文件,就会对应启动大量的MapTask,而每个MapTask处理量很小,时间浪费在了初始化、资源启动回收等阶段,这个方式导致资源利用率不高。
CombineTextInputFormat用于小文件过多的场景,它可以将多个小文件从逻辑上划分成一个切片,这个多个小文件就可以交给一个MapTask处理,提高资源利用率。
需求 :将输入数据中的多个小文件合并为一个切片处理
运行WordCount案例,准备多个小文件 具体使用方式
1// 如果不设置InputFormat,它默认用的是TextInputFormat.class
2job.setInputFormatClass(CombineTextInputFormat.class);
3//虚拟存储切片最大值设置4m
4CombineTextInputFormat.setMaxInputSplitSize(job, 4194304);
CombineTextInputFormat切片原理
切片生成过程分为两个部分,虚拟存储过程和切片过程
假设设置setMaxInputSplitSize值为4M,
四个小文件
1.t x t -> 2M
2.txt -> 7M
3.txt -> 0.3M
4.txt -> 8.2M
虚拟存储过程:把输入目录下面所有的文件大小,依次和设置的setMaxInputSplitSize值进行过比较,如果不大于设置的最大值,逻辑上划分一个块。如果输入文件大于设置的最大值且大于两倍,那么以最大值切割一块,当剩余数据台下超过设置的最大值,但是不大于最大值的两杯,将文件均分成两块(防止出去太小的块)
1.t x t -> 2M 2M<4M,单独一个块
2.txt -> 7M 7M>4M,但是不大于两倍,两个3.5M的文件块
3.txt -> 0.3M 0.3M<4M,单独一个块
4.txt -> 8.2M 8.2M >4M,大于两倍,一个4M的文件,两个2.1的文件
所以虚拟块信息:2M,3.5M,3.5M,0.3M,4M,2.1M,2.1M,共七个虚拟块
切片过程
判断虚拟存储文件大小是否大于setMaxInputSplitSize,大于等于单独形成一个切片
如果不大于,则跟下一个虚拟存储文件合并,共同形成一个切片
所以切片为:(2M+3.5M) + (3.5M+0.3M+4M) + (2.1M+2.1M)三个切片
注意:虚拟存储切片最大值设置最好根据实际的小文件大小情况设置具体的值
自定义InputFormat
HDFS还是MapReduce,在处理小文件时效率都非常低,但又难免面临处理大量小文件的场景,
此时,就需要有相应解决方案。可以自定义InputFormat实现小文件的合并。
需求:
将多个小文件合并成一个SequenceFile文件(SequenceFile文件是Hadoop用来存储二进制形式的 key-value对的文件格式),SequenceFile里面存储着多个文件,存储的形式为文件路径+名称为 key,文件内容为value。
结果
得到一个合并了多个小文件的SequenceFile文件 整体思路
定义一个类继承FileInputFormat
重写isSplitable()指定为不可切分;重写createRecordReader()方法,创建自己的
RecorderReader对象
改变默认读取数据方式,实现一次读取一个完整文件作为kv输出;
Driver指定使用的InputFormat类型
CustomInputFormat
1package com.hhb.mapreduce.sequence;
2
3
4import org.apache.hadoop.fs.Path;
5import org.apache.hadoop.io.BytesWritable;
6import org.apache.hadoop.io.Text;
7import org.apache.hadoop.mapreduce.InputSplit;
8import org.apache.hadoop.mapreduce.JobContext;
9import org.apache.hadoop.mapreduce.RecordReader;
10import org.apache.hadoop.mapreduce.TaskAttemptContext;
11import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
12
13import java.io.IOException;
14
15
16/**
17 * @author: huanghongbo
18 * @Date: 2020-07-06 19:21
19 * @Description:
20 */
21public class CustomInputFormat extends FileInputFormat<Text, BytesWritable> {
22
23
24 /**
25 * 判断文件是否需要切片,当前操作是一次读取整个文件,不需要切片
26 *
27 * @param context
28 * @param filename
29 * @return
30 * @throws IOException
31 */
32 @Override
33 protected boolean isSplitable(JobContext context, Path filename) {
34 return false;
35 }
36
37 /**
38 * 创建自定义的RecordReader,用来读取数据
39 *
40 * @param split
41 * @param context
42 * @return
43 * @throws IOException
44 * @throws InterruptedException
45 */
46 @Override
47 public RecordReader<Text, BytesWritable> createRecordReader(InputSplit split, TaskAttemptContext context) throws IOException, InterruptedException {
48 CustomRecordReader customRecordReader = new CustomRecordReader();
49 customRecordReader.initialize(split, context);
50 return customRecordReader;
51 }
52}
CustomRecordReader
1package com.hhb.mapreduce.sequence;
2
3import org.apache.hadoop.conf.Configuration;
4import org.apache.hadoop.fs.FSDataInputStream;
5import org.apache.hadoop.fs.FileSystem;
6import org.apache.hadoop.fs.Path;
7import org.apache.hadoop.io.BytesWritable;
8import org.apache.hadoop.io.IOUtils;
9import org.apache.hadoop.io.Text;
10import org.apache.hadoop.mapreduce.InputSplit;
11import org.apache.hadoop.mapreduce.RecordReader;
12import org.apache.hadoop.mapreduce.TaskAttemptContext;
13import org.apache.hadoop.mapreduce.lib.input.FileSplit;
14
15import java.io.IOException;
16
17/**
18 * @author: huanghongbo
19 * @Date: 2020-07-06 19:23
20 * @Description: 负责读取数据,一次将整个文本数据读取完,封装成kv输出
21 */
22public class CustomRecordReader extends RecordReader<Text, BytesWritable> {
23
24
25 private FileSplit fileSplit;
26
27 private Configuration configuration;
28
29 private Text key = new Text();
30
31 private BytesWritable value = new BytesWritable();
32
33 //用来判断是否读取过文件的表示
34 private boolean flag = true;
35
36 /**
37 * 初始化数据,把切片和上下文提升为全局属性
38 *
39 * @param split
40 * @param context
41 * @throws IOException
42 * @throws InterruptedException
43 */
44 @Override
45 public void initialize(InputSplit split, TaskAttemptContext context) throws IOException, InterruptedException {
46 fileSplit = (FileSplit) split;
47 configuration = context.getConfiguration();
48 }
49
50 /**
51 * 读取数据
52 *
53 * @return
54 * @throws IOException
55 * @throws InterruptedException
56 */
57 @Override
58 public boolean nextKeyValue() throws IOException, InterruptedException {
59 if (flag) {
60 //创建一个字节数组,长度为文件的大小
61 byte[] bytes = new byte[(int) fileSplit.getLength()];
62 //获取文件路径
63 Path path = fileSplit.getPath();
64 //获取文件信息
65 FileSystem fileSystem = path.getFileSystem(configuration);
66 //获取输入流
67 FSDataInputStream fsDataInputStream = fileSystem.open(path);
68 //将输入流的数组复制到bytes中
69 IOUtils.readFully(fsDataInputStream, bytes, 0, bytes.length);
70 key.set(fileSplit.getPath().toString());
71 value.set(bytes, 0, bytes.length);
72 flag = false;
73 fsDataInputStream.close();
74 return true;
75 }
76 return false;
77 }
78
79 /**
80 * 获取当前的key
81 *
82 * @return
83 * @throws IOException
84 * @throws InterruptedException
85 */
86 @Override
87 public Text getCurrentKey() throws IOException, InterruptedException {
88 return key;
89 }
90
91 @Override
92 public BytesWritable getCurrentValue() throws IOException, InterruptedException {
93 return value;
94 }
95
96 /**
97 * 获取进度
98 *
99 * @return
100 * @throws IOException
101 * @throws InterruptedException
102 */
103 @Override
104 public float getProgress() throws IOException, InterruptedException {
105 return 0;
106 }
107
108 @Override
109 public void close() throws IOException {
110
111 }
112}
SequenceMapper
1package com.hhb.mapreduce.sequence;
2
3import org.apache.hadoop.io.BytesWritable;
4import org.apache.hadoop.io.Text;
5import org.apache.hadoop.mapreduce.Mapper;
6
7import java.io.IOException;
8
9/**
10 * @author: huanghongbo
11 * @Date: 2020-07-06 19:36
12 * @Description:
13 */
14public class SequenceMapper extends Mapper<Text, BytesWritable, Text, BytesWritable> {
15
16 @Override
17 protected void map(Text key, BytesWritable value, Context context) throws IOException, InterruptedException {
18 // 读取内容直接输出
19 context.write(key, value);
20 }
21}
SequenceReducer
1package com.hhb.mapreduce.sequence;
2
3import org.apache.hadoop.io.BytesWritable;
4import org.apache.hadoop.io.Text;
5import org.apache.hadoop.mapreduce.Reducer;
6
7import java.io.IOException;
8
9/**
10 * @author: huanghongbo
11 * @Date: 2020-07-06 19:38
12 * @Description:
13 */
14public class SequenceReducer extends Reducer<Text, BytesWritable, Text, BytesWritable> {
15
16 @Override
17 protected void reduce(Text key, Iterable<BytesWritable> values, Context context) throws IOException, InterruptedException {
18 //values 的size应该是1,只有一个文件,直接输出第一个文件就好
19 context.write(key, values.iterator().next());
20 }
21}
SequenceDriver
1package com.hhb.mapreduce.sequence;
2
3import com.hhb.mapreduce.wc.WordCountDriver;
4import com.hhb.mapreduce.wc.WordCountMapper;
5import com.hhb.mapreduce.wc.WordCountReducer;
6import org.apache.hadoop.conf.Configuration;
7import org.apache.hadoop.fs.Path;
8import org.apache.hadoop.io.BytesWritable;
9import org.apache.hadoop.io.IntWritable;
10import org.apache.hadoop.io.Text;
11import org.apache.hadoop.mapreduce.Job;
12import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
13import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
14
15import java.io.IOException;
16
17/**
18 * @author: huanghongbo
19 * @Date: 2020-07-06 19:40
20 * @Description:
21 */
22public class SequenceDriver {
23
24
25 public static void main(String[] args) throws IOException, ClassNotFoundException, InterruptedException {
26 //1. 获取配置文件对象,获取job对象实例
27 Configuration conf = new Configuration();
28 Job job = Job.getInstance(conf, "SequenceDriver");
29 //2. 指定程序jar的本地路径
30 job.setJarByClass(SequenceDriver.class);
31 //3. 指定Mapper/Reducer类
32 job.setMapperClass(SequenceMapper.class);
33 job.setReducerClass(SequenceReducer.class);
34 //4. 指定Mapper输出的kv数据类型
35 job.setMapOutputKeyClass(Text.class);
36 job.setMapOutputValueClass(BytesWritable.class);
37 //5. 指定最终输出的kv数据类型
38 job.setOutputKeyClass(Text.class);
39 job.setOutputValueClass(BytesWritable.class);
40
41 //设置文件的输入类型
42 job.setInputFormatClass(CustomInputFormat.class);
43
44 //6. 指定job处理的原始数据路径 /Users/baiwang/Desktop/hhb.txt
45 FileInputFormat.setInputPaths(job, new Path("/Users/baiwang/myproject/hadoop/src/main/data/小文件"));
46 //7. 指定job输出结果路径 /Users/baiwang/Desktop/hhb.txt
47 FileOutputFormat.setOutputPath(job, new Path("/Users/baiwang/myproject/hadoop/src/main/data/小文件/seq/输出"));
48 //8. 提交作业
49 boolean flag = job.waitForCompletion(true);
50 //0:表示JVM正常退出,1:表示JVM异常退出
51 System.exit(flag ? 0 : 1);
52 }
53}
自定义OutputFormat
OutputFormat:是MapReduce输出数据的基类,所有MapReduce的数据输出都实现了OutputFormat 抽象类。下面我们介绍几种常见的OutputFormat子类
TextOutputFormat 默认的输出格式是TextOutputFormat,它把每条记录写为文本行。它的键和值可以是任意类型,因为TextOutputFormat调用toString()方 法把它们转换为字符串。
SequenceFileOutputFormat
将SequenceFileOutputFormat输出作为后续MapReduce任务的输入,这是一种好的输出格式, 因为它的格式紧凑,很容易被压缩。
需求分析
要在一个MapReduce程序中根据数据的不同输出两类结果到不同目录,这类输出需求可以通过自定义 OutputFormat来实现。
实现步骤
自定义一个类继承FileOutputFormat。
改写RecordWriter,改写输出数据的方法write()。
Mapper.java
1package com.hhb.mapreduce.sequence.output;
2
3import org.apache.hadoop.io.LongWritable;
4import org.apache.hadoop.io.NullWritable;
5import org.apache.hadoop.io.Text;
6import org.apache.hadoop.mapreduce.Mapper;
7
8import java.io.IOException;
9
10/**
11 * @author: huanghongbo
12 * @Date: 2020-07-06 20:07
13 * @Description:
14 */
15public class OutputMapper extends Mapper<LongWritable, Text, Text, NullWritable> {
16
17
18 //直接输出
19 @Override
20 protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
21 context.write(value, NullWritable.get());
22 }
23}
Reducer
1package com.hhb.mapreduce.sequence.output;
2
3import org.apache.hadoop.io.NullWritable;
4import org.apache.hadoop.io.Text;
5import org.apache.hadoop.mapreduce.Reducer;
6
7import java.io.IOException;
8
9/**
10 * @author: huanghongbo
11 * @Date: 2020-07-06 20:08
12 * @Description:
13 */
14public class OutputReducer extends Reducer<Text, NullWritable, Text, NullWritable> {
15
16 @Override
17 protected void reduce(Text key, Iterable<NullWritable> values, Context context) throws IOException, InterruptedException {
18 context.write(key, NullWritable.get());
19 }
20}
CustomOutputFormat
1package com.hhb.mapreduce.sequence.output;
2
3import org.apache.hadoop.conf.Configuration;
4import org.apache.hadoop.fs.FSDataOutputStream;
5import org.apache.hadoop.fs.FileSystem;
6import org.apache.hadoop.fs.Path;
7import org.apache.hadoop.io.NullWritable;
8import org.apache.hadoop.io.Text;
9import org.apache.hadoop.mapreduce.RecordWriter;
10import org.apache.hadoop.mapreduce.TaskAttemptContext;
11import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
12
13import java.io.IOException;
14
15/**
16 * @author: huanghongbo
17 * @Date: 2020-07-06 20:10
18 * @Description:
19 */
20public class CustomOutputFormat extends FileOutputFormat<Text, NullWritable> {
21
22
23 @Override
24 public RecordWriter<Text, NullWritable> getRecordWriter(TaskAttemptContext context) throws IOException, InterruptedException {
25 //获取配置信息
26 Configuration configuration = context.getConfiguration();
27 //获取文件系统高
28 FileSystem fileSystem = FileSystem.get(configuration);
29 //创建两个输出流
30 FSDataOutputStream lagou = fileSystem.create(new Path("/Users/baiwang/myproject/hadoop/src/main/data/click_log/out/lagou.txt"));
31 FSDataOutputStream other = fileSystem.create(new Path("/Users/baiwang/myproject/hadoop/src/main/data/click_log/out/other.txt"));
32 CustomRecordWriter customRecordWriter = new CustomRecordWriter(lagou, other);
33 return customRecordWriter;
34 }
35}
CustomRecordWriter
1package com.hhb.mapreduce.sequence.output;
2
3import org.apache.hadoop.fs.FSDataOutputStream;
4import org.apache.hadoop.io.IOUtils;
5import org.apache.hadoop.io.NullWritable;
6import org.apache.hadoop.io.Text;
7import org.apache.hadoop.mapreduce.RecordWriter;
8import org.apache.hadoop.mapreduce.TaskAttemptContext;
9
10import java.io.IOException;
11
12/**
13 * @author: huanghongbo
14 * @Date: 2020-07-06 20:11
15 * @Description:
16 */
17public class CustomRecordWriter extends RecordWriter<Text, NullWritable> {
18
19 private FSDataOutputStream lagou;
20
21 private FSDataOutputStream other;
22
23 //对两个输出流赋值
24 public CustomRecordWriter(FSDataOutputStream lagou, FSDataOutputStream other) {
25 this.lagou = lagou;
26 this.other = other;
27 }
28
29 //写数据的路径
30 @Override
31 public void write(Text key, NullWritable value) throws IOException, InterruptedException {
32 String line = key.toString();
33 //如果一行包含lagou,则输出到lgou,否则输出到other
34 if (line.contains("lagou")) {
35 lagou.write(line.getBytes());
36 lagou.write("
".getBytes());
37 } else {
38 other.write(line.getBytes());
39 other.write("
".getBytes());
40 }
41 }
42
43 @Override
44 public void close(TaskAttemptContext context) throws IOException, InterruptedException {
45 IOUtils.closeStream(lagou);
46 IOUtils.closeStream(other);
47 }
48}
Driver.java
1package com.hhb.mapreduce.sequence.output;
2
3import com.hhb.mapreduce.sequence.input.CustomInputFormat;
4import com.hhb.mapreduce.sequence.input.SequenceDriver;
5import com.hhb.mapreduce.sequence.input.SequenceMapper;
6import com.hhb.mapreduce.sequence.input.SequenceReducer;
7import org.apache.hadoop.conf.Configuration;
8import org.apache.hadoop.fs.Path;
9import org.apache.hadoop.io.BytesWritable;
10import org.apache.hadoop.io.NullWritable;
11import org.apache.hadoop.io.Text;
12import org.apache.hadoop.mapreduce.Job;
13import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
14import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
15
16import java.io.IOException;
17
18/**
19 * @author: huanghongbo
20 * @Date: 2020-07-06 20:21
21 * @Description:
22 */
23public class OutputDriver {
24
25
26 public static void main(String[] args) throws IOException, ClassNotFoundException, InterruptedException {
27 //1. 获取配置文件对象,获取job对象实例
28 Configuration conf = new Configuration();
29 Job job = Job.getInstance(conf, "OutputDriver");
30 //2. 指定程序jar的本地路径
31 job.setJarByClass(OutputDriver.class);
32 //3. 指定Mapper/Reducer类
33 job.setMapperClass(OutputMapper.class);
34 job.setReducerClass(OutputReducer.class);
35 //4. 指定Mapper输出的kv数据类型
36 job.setMapOutputKeyClass(Text.class);
37 job.setMapOutputValueClass(NullWritable.class);
38 //5. 指定最终输出的kv数据类型
39 job.setOutputKeyClass(Text.class);
40 job.setOutputValueClass(NullWritable.class);
41
42
43 job.setOutputFormatClass(CustomOutputFormat.class);
44
45
46 //6. 指定job处理的原始数据路径 /Users/baiwang/Desktop/hhb.txt
47 FileInputFormat.setInputPaths(job, new Path("/Users/baiwang/myproject/hadoop/src/main/data/click_log"));
48 //7. 指定job输出结果路径 /Users/baiwang/Desktop/hhb.txt,因为输出的文件不仅仅有laguo.txt等,还有success文件
49 FileOutputFormat.setOutputPath(job, new Path("/Users/baiwang/myproject/hadoop/src/main/data/click_log/out"));
50 //8. 提交作业
51 boolean flag = job.waitForCompletion(true);
52 //0:表示JVM正常退出,1:表示JVM异常退出
53 System.exit(flag ? 0 : 1);
54 }
55}
Shuffle阶段数据压缩
数据压缩有两大好处,节约磁盘空间,加速数据在网络和磁盘上的传输!!
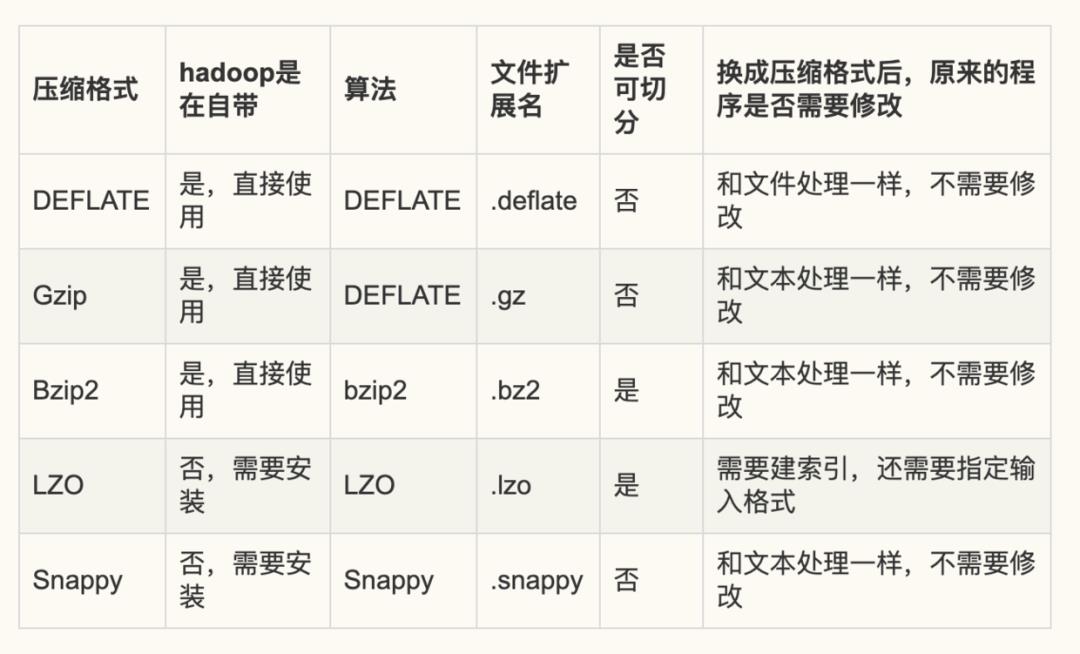
为了支持多种压缩/解压缩算法,Hadoop引入了编码/解编码器
常见的压缩对比分析
压缩的位置:
Map输入端压缩:
此处使用的压缩文件作为Map的输入数据。无需指定编码,Hadoop自动检查文件扩展名,如果压缩方式能够匹配,Hadoop就会选择合适的编解码方式对文件进行压缩和解压。
Map输出端压缩:
Shuffle是Hadoop MR过程中资源消耗最多的阶段,如果数据量过大造成网络传输速度缓慢,可以使用压缩
Reduce输出端压缩:
输出结果数据使用压缩能够减少数据存储的数据量。
压缩配置方式
在驱动代码中通过Configurtaion直接设置,只压缩当前MR任务
1设置map阶段压缩
2Configuration configuration = new Configuration(); configuration.set("mapreduce.map.output.compress","true"); configuration.set("mapreduce.map.output.compress.codec","org.apache.hadoop.io.compress.SnappyCodec");
3设置reduce阶段的压缩 configuration.set("mapreduce.output.fileoutputformat.compress","true"); configuration.set("mapreduce.output.fileoutputformat.compress.type","RECORD" ); configuration.set("mapreduce.output.fileoutputformat.compress.codec","org.apache.hadoop.io.compress.SnappyCodec");
配置mapred-site.xml,该方式所有MR任务都压缩
1<property>
2 <name>mapreduce.output.fileoutputformat.compress</name>
3 <value>true</value>
4</property>
5<property>
6 <name>mapreduce.output.fileoutputformat.compress.type</name>
7 <value>RECORD</value>
8</property>
9<property>
10 <name>mapreduce.output.fileoutputformat.compress.codec</name>
11 <value>org.apache.hadoop.io.compress.SnappyCodec</value>
12</property>
以上是关于Hadoop ---- MapReduce的主要内容,如果未能解决你的问题,请参考以下文章