Day9-计数排序/平方根/搜索插入位置
Posted spytensor
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了Day9-计数排序/平方根/搜索插入位置相关的知识,希望对你有一定的参考价值。
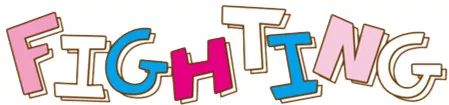
几次面试后深知非科班在基础上的弱势,故决定自2018年5月7日起刷一波算法和数据结构,编程语言为:Python。
(6)排序算法-计数排序
算法思想:
计数排序的核心在于将输入的数据值转化为键存储在额外开辟的数组空间中。作为一种线性时间复杂度的排序,计数排序要求输入的数据必须是有确定范围的整数。
Python代码:
def countingSort(arr, maxValue):
bucketLen = maxValue+1
bucket = [0]*bucketLen
sortedIndex =0
arrLen = len(arr)
for i in range(arrLen):
if not bucket[arr[i]]:
bucket[arr[i]]=0
bucket[arr[i]]+=1
for j in range(bucketLen):
while bucket[j]>0:
arr[sortedIndex] = j
sortedIndex+=1
bucket[j]-=1
return arr
LeetCode-35 搜索插入位置
问题描述:
给定一个排序数组和一个目标值,在数组中找到目标值,并返回其索引。如果目标值不存在于数组中,返回它将会被按顺序插入的位置。
你可以假设数组中无重复元素。
实现代码:
class Solution:
def searchInsert(self, nums, target):
""" :type nums: List[int] :type target: int :rtype: int """
if target not in nums:
if nums[0] > target:
return 0
elif nums[-1] < target:
return len(nums)
else:
for i in range(len(nums) - 1):
if nums[i] < target and nums[i+1] > target:
return i+1
else:
return nums.index(target)
LeetCode-69 实现求解平方根
问题描述:
实现求解平方根函数。要求:计算并返回x的平方根,其中x是非负整数。由于返回类型是整数,结果只保留整数部分,小数部分舍去。
实现代码:
class Solution:
def mySqrt(self, x):
""" :type x: int :rtype: int """
y = 1.0
while abs(y * y - x) > 1e-6:
y = (y + x/y)/2
return int(y)
Python算法实现之---冒泡排序
深度学习开发环境配置--win10+Tensorflow-GPU
以上是关于Day9-计数排序/平方根/搜索插入位置的主要内容,如果未能解决你的问题,请参考以下文章
LeetCode与《代码随想录》数组篇:做题笔记与总结-Java版
常考排序算法总结(插入排序,希尔排序,快速排序,归并排序,计数排序)