GO语言系列
Posted 麦克叔叔每晚10点说
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了GO语言系列相关的知识,希望对你有一定的参考价值。
来看一些实际的代码示例及运行输出。
HelloWorld程序
package main
import "fmt"
func main() {
fmt.Println("hello world")
}
输出:
$ go run hello-world.go
hello world
$ go build hello-world.go
$ ls
hello-worldhello-world.go
$ ./hello-world
hello world
值运算
package main
import "fmt"
func main() {
fmt.Println("go" + "lang")
fmt.Println("1+1 =", 1+1)
fmt.Println("7.0/3.0 =", 7.0/3.0)
fmt.Println(true && false)
fmt.Println(true || false)
fmt.Println(!true)
}
输出:
$ go run values.go
golang
1+1 = 2
7.0/3.0 = 2.3333333333333335
false
true
false
变量
package main
import "fmt"
func main() {
var a = "initial"
fmt.Println(a)
var b, c int = 1, 2
fmt.Println(b, c)
var d = true
fmt.Println(d)
var e int
fmt.Println(e)
f := "short"
fmt.Println(f)
}
输出:
$ go run variables.go
initial
1 2
true
0
Short
常量
package main
import "fmt"
import "math"
const s string = "constant"
func main() {
fmt.Println(s)
const n = 500000000
const d = 3e20 / n
fmt.Println(d)
fmt.Println(int64(d))
fmt.Println(math.Sin(n))
}
输出:
$ go run constant.go
constant
6e+11
600000000000
-0.28470407323754404
For循环
package main
import "fmt"
func main() {
i := 1
for i <= 3 {
fmt.Println(i)
i = i + 1
}
for j := 7; j <= 9; j++ {
fmt.Println(j)
}
for {
fmt.Println("loop")
break
}
for n := 0; n <= 5; n++ {
if n%2 == 0 {
continue
}
fmt.Println(n)
}
}
输出:
$ go run for.go
1
2
3
7
8
9
loop
1
3
5
发个小广告!!!走过路过,不要错过!新书来啦!!!
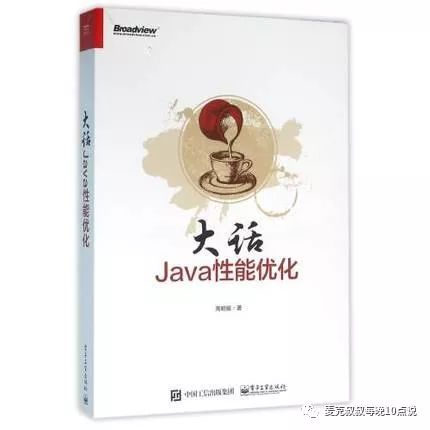
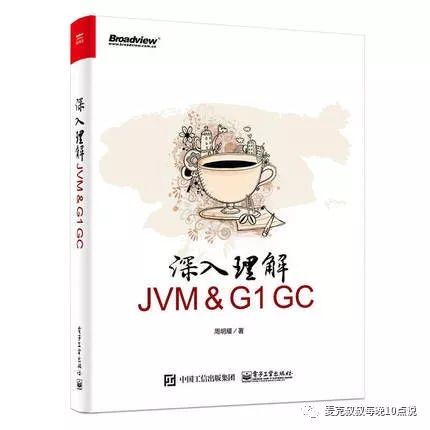
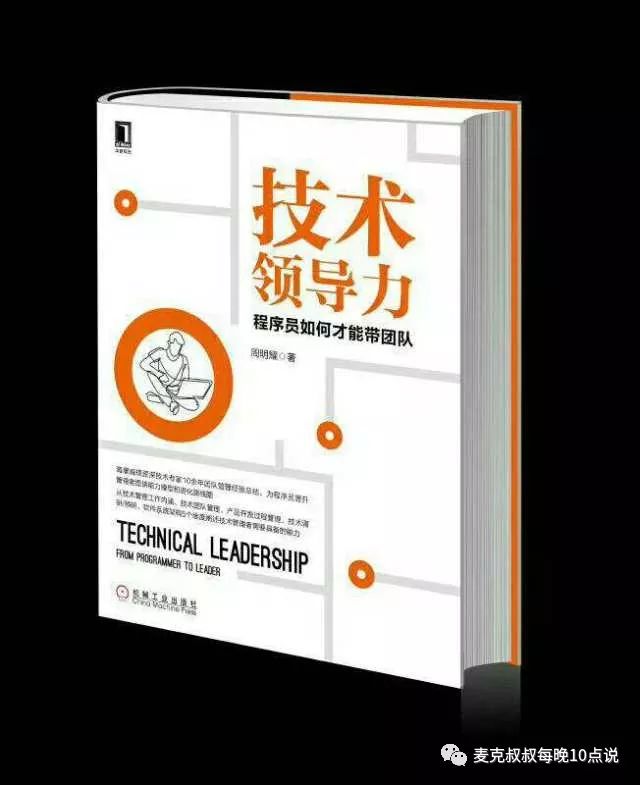
这里有你想买的书!
http://product.dangdang.com/23949549.html#ddclick_reco_reco_relate
麦克叔叔每晚十点说
以上是关于GO语言系列的主要内容,如果未能解决你的问题,请参考以下文章