Android-Button及其子类详解
Posted 帅次
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了Android-Button及其子类详解相关的知识,希望对你有一定的参考价值。
Button
Button是用户可以点击或单击以执行操作的用户界面元素。
1、在xml中添加按钮
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_margin="@dimen/dimen_20"
android:gravity="center_horizontal"
android:orientation="vertical">
<Button
android:id="@+id/btn_1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="@dimen/text_size_18"
android:padding="@dimen/dimen_10"
android:background="@color/color_188FFF"
android:backgroundTint="@null"
android:textColor="@color/white"
android:text="普通按钮没钮权" />
<!--带边框的文本背景色渐变-->
<Button
android:id="@+id/btn_2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="背景色渐变的按钮"
android:layout_marginTop="@dimen/dimen_20"
android:padding="@dimen/dimen_10"
android:textSize="@dimen/text_size_18"
android:textColor="@color/white"
android:background="@drawable/bg_btn_frame_gradient"/>
<Button
android:id="@+id/btn_3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="点击变蓝,哇哈哈"
android:layout_marginTop="@dimen/dimen_20"
android:padding="@dimen/dimen_10"
android:onClick="btn3OnClick"
android:textSize="@dimen/text_size_18"
android:textColor="@color/white"
android:background="@drawable/bg_btn_selector_bg"/>
<TextView
android:id="@+id/tv_1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="@dimen/text_size_18"
android:layout_marginTop="@dimen/dimen_20"
android:padding="@dimen/dimen_10"
android:background="@color/color_ff0000"
android:backgroundTint="@null"
android:textColor="@color/white"
android:text="TextView点击来袭" />
</LinearLayout>
@drawable/bg_btn_frame_gradient内容:
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<!--radius四个圆角统一设置,也可以单独对某一个圆角设置。例:topLeftRadius-->
<corners android:radius="8dp"/>
<!--边框宽度width、颜色color-->
<stroke android:width="1dp" android:color="@color/color_ff0000" />
<gradient
android:startColor="@color/color_188FFF"
android:centerColor="@color/color_FF773D"
android:endColor="@color/color_ff0000"
android:type="linear"
/>
</shape>
@drawable/bg_btn_selector_bg内容:
<?xml version ="1.0" encoding ="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<!--radius四个圆角统一设置,也可以单独对某一个圆角设置。例:topLeftRadius-->
<corners android:radius="8dp"/>
<!--未点击显示颜色-->
<item android:state_pressed="false"
android:drawable="@color/color_FF773D"/>
<!--点击显示颜色-->
<item android:state_pressed="true"
android:drawable="@color/color_188FFF"/>
</selector>
2、在代码中实现Button的OnClick事件
package com.scc.demo;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
public class ButtonActivity extends AppCompatActivity implements View.OnClickListener {
private Button btn_1,btn_2,btn_3;
private TextView tv_1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.view_button);//加载布局文件
initViewButton();
}
private void initViewButton(){
btn_1 = findViewById(R.id.btn_1);
btn_2 = findViewById(R.id.btn_2);
btn_3 = findViewById(R.id.btn_3);
tv_1 = findViewById(R.id.tv_1);
btn_1.setOnClickListener(this);
tv_1.setOnClickListener(this);
btn_3.setOnClickListener(this);
btn_2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(ButtonActivity.this,"btn_2点击事件",Toast.LENGTH_SHORT).show();
}
});
btn_2.setOnLongClickListener(new View.OnLongClickListener() {
@Override
public boolean onLongClick(View v) {
Toast.makeText(ButtonActivity.this,"btn_2长按点击事件",Toast.LENGTH_SHORT).show();
return false;
}
});
}
public void btn3OnClick(View view){
Toast.makeText(this,"btn_3点击事件",Toast.LENGTH_SHORT).show();
}
@Override
public void onClick(View v) {
switch (v.getId()){
case R.id.btn_1:
Toast.makeText(this,"btn_1点击事件",Toast.LENGTH_SHORT).show();
break;
case R.id.tv_1:
Toast.makeText(this,"TextView点击事件",Toast.LENGTH_SHORT).show();
break;
case R.id.btn_3:
Toast.makeText(this,"tn_3onClick点击事件",Toast.LENGTH_SHORT).show();
break;
default:
break;
}
}
}
2.1、在xml中实现添加android:onClick="btn3OnClick"属性,在代码中实现
public void btn3OnClick(View view){}
同时要满足以下条件:
①方法的修饰符是 public;
②返回值是 void 类型;
③只有一个参数View,这个View就是被点击的这个控件。
2.2、直接实现OnClickListener接口
btn_2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
}
});
2.3、activity实现View.OnClickListener接口
btn_1.setOnClickListener(this);
tv_1.setOnClickListener(this);
btn_3.setOnClickListener(this);
@Override
public void onClick(View v) {
switch (v.getId()){
case R.id.btn_1:
Toast.makeText(this,"btn_1点击事件",Toast.LENGTH_SHORT).show();
break;
case R.id.tv_1:
Toast.makeText(this,"TextView点击事件",Toast.LENGTH_SHORT).show();
break;
case R.id.btn_3:
Toast.makeText(this,"tn_3onClick点击事件",Toast.LENGTH_SHORT).show();
break;
default:
break;
}
}
综上实例:
Button的setOnClickListener优先级比xml中android:onClick高,如果同时设置点击事件,只有setOnClickListener有效。
TextView也可以实现onClick事件,如果部分Button使用麻烦可以考虑使用TextView来代替。
当然,Button生成的按钮功能很强大,它可以通过背景色来设置图片、颜色等,而且Button生成的按钮不仅可以是普通文字按钮,也可以定制成任意形状,并可以随用户的交互动作改变外观。
button设置backgroud无效
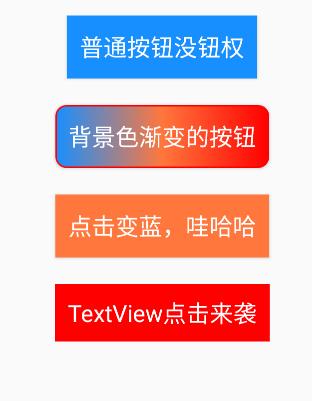
解决方案:
默认的颜色设置来自于res/values/themes.xml将里面的主题由
parent="Theme.MaterialComponents.DayNight.DarkActionBar"
修改为(其他主题也可以)parent="Theme.MaterialComponents.DayNight.DarkActionBar.Bridge"
修改后正常了
RadioButton
RadioButton 是单选按钮控件,多个 RadioButton 控件可以为一组,这一组内的 RadioButton 控件只能有一个被选中,并且只要选择后无法取消。
CheckBox
CheckBox是复选框,是一种特定类型的双状态按钮,可以检查或未选中。
在XML布局文件中,必须为该组单选钮每个按钮指定id属性,否则这组单选钮无法正常工作。
<?xml version="1.0" encoding="utf-8"?>
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_margin="@dimen/dimen_20"
android:orientation="vertical">
<TableRow>
<TextView android:text="爱好:"
android:textSize="@dimen/text_size_18"
android:layout_gravity="center_vertical"/>
<RadioGroup
android:id="@+id/rg"
android:layout_gravity="center_horizontal"
android:orientation="horizontal">
<RadioButton
android:id="@+id/rb_liangpi"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textColor="@color/color_ff0000"
android:textSize="@dimen/text_size_18"
android:text="凉皮" />
<RadioButton
android:id="@+id/rb_roujiamo"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textColor="@color/color_188FFF"
android:textSize="@dimen/text_size_18"
android:text="肉夹馍" />
</RadioGroup>
</TableRow>
<TableRow>
<TextView android:text="调料:"
android:textSize="@dimen/text_size_18"
android:layout_marginTop="5dp"
/>
<LinearLayout android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<CheckBox
android:id="@+id/cb_yan"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="@dimen/text_size_18"
android:textColor="@color/color_188FFF"
android:checked="true"
android:text="加盐" />
<CheckBox
android:id="@+id/cb_cu"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="@dimen/text_size_18"
android:textColor="@color/color_ff0000"
android:text="加醋" />
<CheckBox
android:id="@+id/cb_lajiao"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="@dimen/text_size_18"
android:textColor="@color/color_FF773D"
android:text="加辣椒" />
</LinearLayout>
</TableRow>
<TableRow>
<Button
android:id="@+id/btn_2"
android:layout_width="150dp"
android:layout_height="wrap_content"
android:layout_marginLeft="@dimen/dimen_20"
android:background="@drawable/bg_btn_frame_gradient"
android:padding="@dimen/dimen_10"
android:text="上菜吧你"
android:textColor="@color/white"
android:textSize="@dimen/text_size_18" />
</TableRow>
</TableLayout>
为了监听RadioButton、CheckBox的勾选状态,可以为他们添加事件监听器(RadioGroup.OnCheckedChangeListener和CompoundButton.OnCheckedChangeListener)。此添加事件监听器的方式才会用了"委托式"‘事件处理机制,委托式事件处理机制的原理是,当事件源发生事件变化时,该事件将会激发该事件源上的事件监听器的特定方法。
public class RadioButtonActivity extends AppCompatActivity {
private RadioGroup rg;
private CheckBox cb_yan,cb_cu,cb_lajiao;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.view_radiobutton);//加载布局文件
initViewButton();
}
private void initViewButton(){
rg = findViewById(R.id.rg);
//在选中的单选按钮时注册要调用的回调
rg.setOnCheckedChangeListener(new RadioGroup.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup group, int checkedId) {
if(checkedId==R.id.rb_liangpi){
Toast.makeText(RadioButtonActivity.this,"选择了凉皮",Toast.LENGTH_SHORT).show();
}else{
Toast.makeText(RadioButtonActivity.this,"选择了肉夹馍",Toast.LENGTH_SHORT).show();
}
}
});
cb_yan = findViewById(R.id.cb_yan);
cb_cu = findViewById(R.id.cb_cu);
cb_lajiao = findViewById(R.id.cb_lajiao);
cb_cu.setChecked(true);
cb_yan.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if(isChecked){
Toast.makeText(RadioButtonActivity.this,"选中加盐",Toast.LENGTH_SHORT).show();
}else{
Toast.makeText(RadioButtonActivity.this,"未选中加盐",Toast.LENGTH_SHORT).show();
}
}
});
cb_cu.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if(isChecked){
Toast.makeText(RadioButtonActivity.this,"选中加醋",Toast.LENGTH_SHORT).show();
}else{
Toast.makeText(RadioButtonActivity.this,"未选中加醋",Toast.LENGTH_SHORT).show();
}
}
});
cb_lajiao.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if(isChecked){
Toast.makeText(RadioButtonActivity.this,"选中加辣椒",Toast.LENGTH_SHORT).show();
}else{
Toast.makeText(RadioButtonActivity.this,"未选中加辣椒",Toast.LENGTH_SHORT).show();
}
}
});
}
}
以上是关于Android-Button及其子类详解的主要内容,如果未能解决你的问题,请参考以下文章
Android Studio入门:Android应用界面详解(中)(Android控件详解AdapterView及其子类)