使用PyMongo查询MongoDB数据库
Posted Python之刃
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了使用PyMongo查询MongoDB数据库相关的知识,希望对你有一定的参考价值。
-
本文我们介绍如何使用PyMongo库查询MongoDB数据库。 -
本文我们介绍MongoDB中的基本聚合操作。
介绍
目录
-
什么是PyMongo? -
安装步骤 -
将数据插入数据库 -
查询数据库 -
根据字段进行过滤 -
根据比较运算符进行过滤 -
基于逻辑运算符进行过滤 -
常用表达 -
聚合管道 -
尾注
什么是PyMongo?
-
https://courses.analyticsvidhya.com/courses/introduction-to-data-science
安装步骤
pip3 install pymongo
将数据插入数据库
-
导入库并连接到mongo客户端
# 导入所需的库
import pymongo
import pprint
import json
import warnings
warnings.filterwarnings('ignore')
# 连接到mongoclient
client = pymongo.MongoClient('mongodb://localhost:27017')
# 获取数据库
database = client['sample_db']
-
从JSON文件创建集合
-
https://drive.google.com/drive/folders/1XqnNv5msuluuX5JV50sGYC0Uvwa7w2C2?usp=sharing
-
weekly_demand: -
id:每个文档的唯一ID -
week:周号 -
center_id:配送中心的唯一ID -
meal_id:餐的唯一ID -
checkout_price:最终价格,包括折扣,税金和送货费 -
base_price:餐的基本价格 -
emailer_for_promotion:发送电子邮件以促进进餐 -
homepage_featured:首页提供的餐点 -
num_orders:(目标)订单数 -
meal_info: -
meal_id:餐的唯一ID -
category:餐食类型(饮料/小吃/汤……) -
cuisine:美食(印度/意大利/…)
# 创建每周需求收集
database.create_collection("weekly_demand")
# 创建餐食信息
database.create_collection("meal_info")


-
将数据插入集合
# 获取collection weekly_demand
weekly_demand_collection = database.get_collection("weekly_demand")
# 打开weekly_demand json文件
with open("weekly_demand.json") as f:
file_data = json.load(f)
# 将数据插入集合
weekly_demand_collection.insert_many(file_data)
# 获取数据的总数量
weekly_demand_collection.find().count()
# >> 456548
# 获取收藏餐
meal_info_collection = database.get_collection("meal_info")
# 打开meat_info json文件
with open("meal_info.json") as f:
file_data = json.load(f)
# 将数据插入集合
meal_info_collection.insert_many(file_data)
# 获取数据的总数量
meal_info_collection.find().count()
# >> 51
weekly_demand_collection.find_one()

meal_info_collection.find_one()
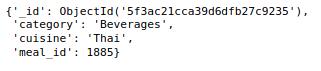
查询数据库
your_collection.find( {<< query >>} , { << fields>>} )
weekly_demand_collection.find_one( {}, { "week": 1, "checkout_price" : 1})

weekly_demand_collection.find_one( {}, {"num_orders" : 0, "meal_id" : 0})

weekly_demand_collection.find_one( {"center_id" : 55, "meal_id" : 1885}, {"_id" : 0, "week" : 0} )

|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
result_1 = weekly_demand_collection.find({
"center_id" : { "$eq" : 55},
"homepage_featured" : { "$ne" : 0}
})
for i in result_1:
print(i)
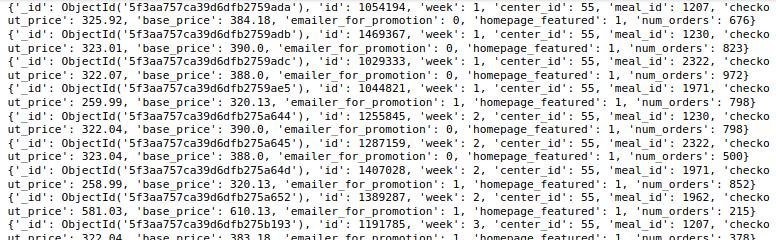
result_2 = weekly_demand_collection.find({
"center_id" : { "$in" : [ 24, 11] }
})
for i in result_2:
print(i)
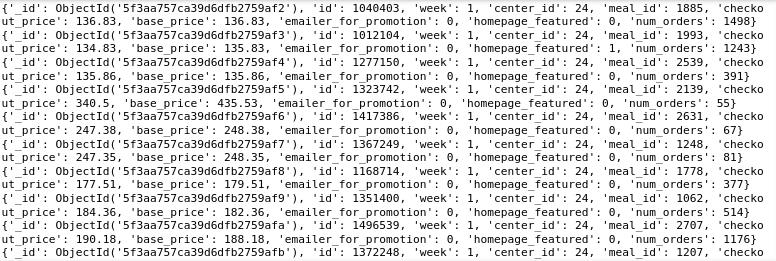
result_3 = weekly_demand_collection.find({
"center_id" : { "$nin" : [ 24, 11] }
})
for i in result_3:
print(i)

result_4 = weekly_demand_collection.find({
"center_id" : 55,
"checkout_price" : { "$lt" : 200, "$gt" : 100}
})
for i in result_4:
print(i)
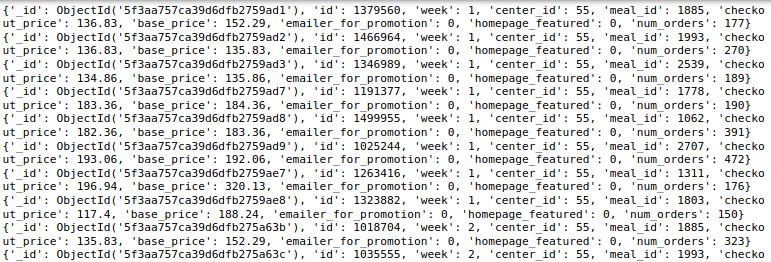
|
|
|
|
|
|
|
|
|
|
result_5 = weekly_demand_collection.find({
"$and" : [{
"center_id" : { "$eq" : 11}
},
{
"meal_id" : { "$ne" : 1778}
}]
})
for i in result_5:
print(i)

result_6 = weekly_demand_collection.find({
"$or" : [{
"center_id" : { "$eq" : 11}
},
{
"meal_id" : { "$in" : [1207, 2707]}
}]
})
for i in result_6:
print(i)

-
https://www.analyticsvidhya.com/blog/2015/06/regular-expression-python
result_7 = meal_info_collection.find({
"cuisine" : { "$regex" : "^C" }
})
for i in result_7:
print(i)

result_8 = meal_info_collection.find({
"$and" : [
{
"category" : {
"$regex" : "^S"
}},
{
"cuisine" : {
"$regex" : "ian$"
}}
]
})
for i in result_8:
print(i)

your_collection.aggregate( [ { <stage1> }, { <stage2> },.. ] )
result_9 = weekly_demand_collection.aggregate([
## stage 1
{
"$match" :
{"center_id" : {"$eq" : 11 } }
},
## stage 2
{
"$count" : "total_rows"
}
])
for i in result_9:
print(i)

result_10 = weekly_demand_collection.aggregate([
## stage 1
{
"$match" :
{"center_id" : {"$eq" : 11 } }
},
## stage 2
{
"$group" : { "_id" : 0 ,
"average_num_orders": { "$avg" : "$num_orders"},
"unique_meal_id" : {"$addToSet" : "$meal_id"}}
}
])
for i in result_10:
print(i)
尾注
-
https://courses.analyticsvidhya.com/courses/structured-query-language-sql-for-data-science
以上是关于使用PyMongo查询MongoDB数据库的主要内容,如果未能解决你的问题,请参考以下文章
遇到问题--mongodb---python---pymongo通过_id查询不到数据
遇到问题--mongodb---python---pymongo通过_id查询不到数据