LeetCode 16. 3sum
Posted shiter
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了LeetCode 16. 3sum相关的知识,希望对你有一定的参考价值。
文章大纲
题目简介与解题思路
题目简介
Given an integer array nums of length n and an integer target, find three integers in nums such that the sum is closest to target.
Return the sum of the three integers.
You may assume that each input would have exactly one solution.
Example 1:
Input: nums = [-1,2,1,-4], target = 1
Output: 2
Explanation: The sum that is closest to the target is 2. (-1 + 2 + 1 = 2).
Example 2:
Input: nums = [0,0,0], target = 1
Output: 0
Constraints:
3 <= nums.length <= 1000
-1000 <= nums[i] <= 1000
-104 <= target <= 104
解法
主要的思路是排序后,移动两个指针的思路
Sort the vector and then no need to run O(N^3) algorithm as each index has a direction to move.
The code starts from this formation.
本题的解法不需要
/-------------------------------------------------------------------
^ ^ -------------------------------------------------------------- ^
| | --------------------------------------------------------------- |
| ± second ------------------------------------------------- third
±first
if nums[first] + nums[second] + nums[third] is smaller than the target, we know we have to increase the sum. so only choice is moving the second index forward.
/--------------------------------------------------------------------
^ ^ -------------------------------------------------------------- ^
| | ---------------------------------------------------------------- |
| — ± second ---------------------------------------------- third
±first
if the sum is bigger than the target, we know that we need to reduce the sum. so only choice is moving ‘third’ to backward. of course if the sum equals to target, we can immediately return the sum.
/----------------------------------------------------------------------------
^ ^ -------------------------------------------------------------- ^
| | ---------------------------------------------------------------- |
| ± second ------------------------------------------------------- third
±first
when second and third cross, the round is done so start next round by moving ‘first’ and resetting second and third.
/---------------------------------------------------------------------
— ^ — ^--------------------------------------------------------- ^
— | ---- | --------------------------------------------------------- |
— | ---- ± second ------------------------------------------- third
— ±first
while doing this, collect the closest sum of each stage by calculating and comparing delta. Compare abs(target-newSum) and abs(target-closest). At the end of the process the three indexes will eventually be gathered at the end of the array.
----------------------------------------------------------------- ^ ^ ^
------------------------------------------------------------------ | | `- third
------------------------------------------------------------------ | ± second
------------------------------------------------------------------ ±first
if no exactly matching sum has been found so far, the value in closest will be the answer.
c++ 代码
class Solution
public:
int threeSumClosest(vector<int>& nums, int target)
sort(nums.begin(), nums.end());
int n = nums.size(), ans = nums[0] + nums[1] + nums[2];
for (int i = 0; i < n-2; ++i)
int l = i + 1, r = n - 1;
while (l < r)
int sum3 = nums[i] + nums[l] + nums[r];
if (abs(ans - target) > abs(sum3 - target)) // Update better ans
ans = sum3;
if (sum3 == target) break;
if (sum3 > target)
--r; // Sum3 is greater than the target, to minimize the difference, we have to decrease our sum3
else
++l; // Sum3 is lesser than the target, to minimize the difference, we have to increase our sum3
return ans;
;
scala 代码
python 代码
class Solution:
def threeSumClosest(self, nums: [int], target: int) -> int:
nums.sort()
result = sum(nums[:3])
for i in range(len(nums)):
left,right = i+1,len(nums)-1
while left < right:
s = sum((nums[i],nums[left],nums[right]))
if abs(s - target) < abs(result - target):
result = s
if s < target:
left = left +1
elif s> target:
right = right -1
else:
return result
return result
if __name__ == "__main__":
s = Solution()
print(s.threeSumClosest([-1,2,1,-4],1))
参考文献
https://leetcode.com/problems/3sum-closest/
scala 版本题解
https://leetcode.com/problems/3sum-closest/discuss/?currentPage=1&orderBy=most_votes&query=&tag=scala
带有解释的题解
https://leetcode.com/problems/3sum-closest/discuss/7883/C%2B%2B-solution-O(n2)-using-sort
python 题解 2 个指针
https://leetcode.com/problems/3sum-closest/discuss/8026/Python-solution-(two-pointer).
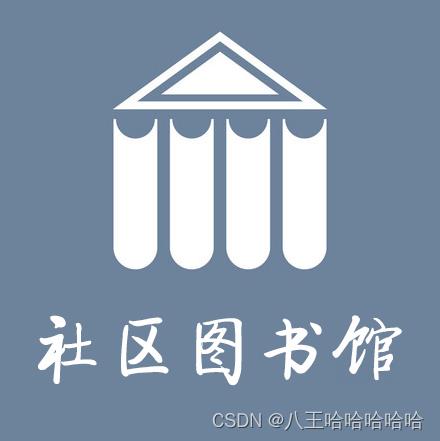

以上是关于LeetCode 16. 3sum的主要内容,如果未能解决你的问题,请参考以下文章