Spring boot入门之-Jpa- One to Many Many to one
Posted Wind_LPH
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了Spring boot入门之-Jpa- One to Many Many to one相关的知识,希望对你有一定的参考价值。
代码参考git。git地址:https://github.com/lidreamwind/Java-Jpa-Data
one to many是一张表的一条记录对应另一张表的多条记录。
Many to one 是一张表的多条记录对应另一张表的一条记录。
两张表之间以外键关系关联在一起。
文档参考:https://download.oracle.com/otn-pub/jcp/persistence-2_1-fr-eval-spec/JavaPersistence.pdf?AuthParam=1574061512_630dd51b88a4d03476be822a6903bad5
1、OneToMany配置如下

1 package com.lph.spring.dev.ManyToOne.pojo; 2 3 import javax.persistence.*; 4 import java.util.List; 5 6 @Entity 7 @Table(name = "student") 8 public class StudentEntity { 9 @Id 10 @GeneratedValue 11 @Column(name = "student_id") 12 private Integer studentId; 13 @Column(name = "name") 14 private String name; 15 @Column(name = "age") 16 private Integer age; 17 18 //studentId是实体类中的属性,mappedBy不能和@JoinTable一起 19 @OneToMany(targetEntity = CourseStudent.class,mappedBy = "studentId",fetch = FetchType.EAGER,cascade = CascadeType.ALL) 20 private List<CourseStudent> courseStudent ; 21 22 public Integer getStudentId() { 23 return studentId; 24 } 25 26 public void setStudentId(Integer studentId) { 27 this.studentId = studentId; 28 } 29 30 public String getName() { 31 return name; 32 } 33 34 public void setName(String name) { 35 this.name = name; 36 } 37 38 public Integer getAge() { 39 return age; 40 } 41 42 public void setAge(Integer age) { 43 this.age = age; 44 } 45 46 public List<CourseStudent> getCourseStudent() { 47 return courseStudent; 48 } 49 50 public void setCourseStudent(List<CourseStudent> courseStudent) { 51 this.courseStudent = courseStudent; 52 } 53 }
2、Many To One的配置如下

1 package com.lph.spring.dev.ManyToOne.pojo; 2 3 import com.fasterxml.jackson.annotation.JsonIgnore; 4 5 import javax.persistence.*; 6 7 @Entity 8 @Table(name = "couurse_student") 9 public class CourseStudent { 10 @Id 11 @GeneratedValue 12 @Column(name = "id") 13 private Integer id; 14 @Column(name = "course_id") 15 private Integer courseId; 16 @Column(name = "student_id") 17 private Integer studentId; 18 19 @ManyToOne() 20 @JoinColumn(name = "student_id",insertable = false,updatable = false,referencedColumnName = "student_id") 21 @JsonIgnore 22 private StudentEntity studentEntity; 23 24 public Integer getId() { 25 return id; 26 } 27 28 public void setId(Integer id) { 29 this.id = id; 30 } 31 32 public Integer getCourseId() { 33 return courseId; 34 } 35 36 public void setCourseId(Integer courseId) { 37 this.courseId = courseId; 38 } 39 40 public Integer getStudentId() { 41 return studentId; 42 } 43 44 public void setStudentId(Integer studentId) { 45 this.studentId = studentId; 46 } 47 48 public StudentEntity getStudentEntity() { 49 return studentEntity; 50 } 51 52 public void setStudentEntity(StudentEntity studentEntity) { 53 this.studentEntity = studentEntity; 54 } 55 }
3、One To Many的配置解析。
@OneToMany(targetEntity = CourseStudent.class,mappedBy = "studentId",fetch = FetchType.EAGER,cascade = CascadeType.ALL)
private List<CourseStudent> courseStudent ;
OneToMany的有两行,一行注解和一个List变量。List变量用来存放一对多的结果。
targetEntity的是用来注释需要和哪个实体类进行联合查询(实体类对应表),mappedBy指定的是实体类中的链接字段,对应表中的字段。
fetch有两个类型,LAZY和EAGER
参考:https://stackoverflow.com/questions/26601032/default-fetch-type-for-one-to-one-many-to-one-and-one-to-many-in-hibernate
cascade有ALL,DETACH,MERGE,PERSIST,REFRESH,REMOVE几种类型。
参考:https://vladmihalcea.com/a-beginners-guide-to-jpa-and-hibernate-cascade-types/
官网文档 ,mappedBy不能用于ManyToOnne注解。
The many side of one-to-many / many-to-one bidirectional relationships must be the owning
side, hence the mappedBy element cannot be specified on the ManyToOne annotation.
4、Many To One的配置解析。
@ManyToOne()
@JoinColumn(name = "student_id",insertable = false,updatable = false,referencedColumnName = "student_id")
@JsonIgnore
private StudentEntity studentEntity;
@ManyToOne是标注为多对一
@JoinColumn是标注匹配的外键列,insertable = false,不允许插入,updatable=false是不允许更新。referenceColumnName标注的是外键列。
@JsonIgnore,生成Json时不生成StudentEntity 的属性
5、结果展示
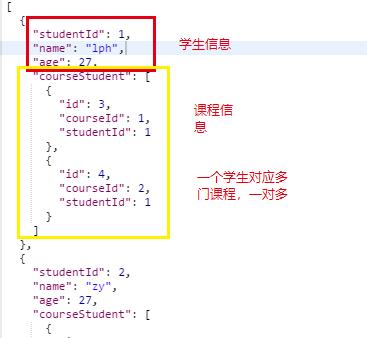
以上是关于Spring boot入门之-Jpa- One to Many Many to one的主要内容,如果未能解决你的问题,请参考以下文章
212. Spring Boot WebFlux:响应式Spring Data之MongoDB
使用Spring Data JPA查询时,报result returns more than one elements异常
spring boot 系列之四:spring boot 整合JPA