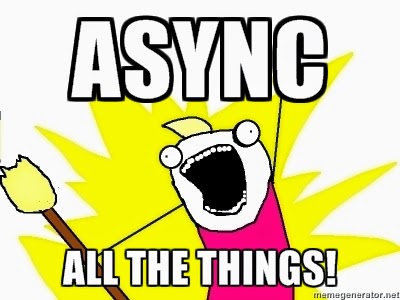
Hardest part
How do you think, how much changes you need to do to make your Swift service asynchronous? Do you afraid of it? You already scheduled a week for this refactoring? Oh, that‘s nice:)import com.facebook.swift.service.ThriftMethod; | |
import com.facebook.swift.service.ThriftService; | |
+import com.google.common.util.concurrent.ListenableFuture; | |
@ThriftService | |
public interface TCalculatorService { | |
@ThriftMethod | |
- int calculate(int num1, int num2, TOperation op) throws TDivisionByZeroException; | |
+ ListenableFuture<Integer> calculate(int num1, int num2, TOperation op) throws TDivisionByZeroException; | |
} |
Now it‘s time to update your service implementation. Are you ready?
import com.example.calculator.protocol.TOperation; | |
+import com.google.common.util.concurrent.*; | |
import org.springframework.stereotype.Component; | |
import com.example.calculator.service.CalculatorService; | |
import org.springframework.beans.factory.annotation.Autowired; | |
+import java.util.concurrent.*; | |
+ | |
@Component | |
public class CalculatorServiceHandler implements TCalculatorService { | |
@Autowired | |
CalculatorService calculatorService; | |
+ ScheduledExecutorService scheduledExecutorService = Executors.newScheduledThreadPool(10); | |
+ | |
@Override | |
- public int calculate(int num1, int num2, TOperation op) throws TDivisionByZeroException { | |
+ public ListenableFuture<Integer> calculate(int num1, int num2, TOperation op) throws TDivisionByZeroException { | |
+ return JdkFutureAdapters.listenInPoolThread( | |
+ scheduledExecutorService.schedule(() -> { | |
switch(op) { | |
case ADD: | |
return calculatorService.add(num1, num2); | |
@@ -32,5 +40,7 @@ | |
default: | |
throw new IllegalArgumentException("Unknown operation " + op); | |
} | |
+ }, 2, TimeUnit.SECONDS) | |
+ ); | |
} | |
} |
Hope you noticed this ScheduledExecutorService and 2 seconds delay. You shouldn‘t use it in your real application, this is just for example of promises (unless you want to simulate work for future optimizations like this guy: http://thedailywtf.com/articles/The-Speedup-Loop )
That‘s it, now your server is asynchronous. There is more - your clients can consume your service like before in non-async manner. How cool is that?
Full source code at GitHub: https://github.com/bsideup/spring-boot-swift/tree/async
Previous parts: