JAVA图书馆库存管理系统程序代码(管理系统+用户购买结账系统)
Posted zhangyufeng0126
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了JAVA图书馆库存管理系统程序代码(管理系统+用户购买结账系统)相关的知识,希望对你有一定的参考价值。
JAVA图书馆库存管理系统(管理系统+用户购买结账系统)
package Library;
import java.io.Serializable;
public class Book implements Serializable
private int bookId;
private String bookName;
private String auther;
private String pubDate;
private double price;
private int store;
public int getBookId()
return bookId;
public void setBookId(int bookId)
this.bookId = bookId;
public String getBookName()
return bookName;
public void setBookName(String bookName)
this.bookName = bookName;
public String getAuther()
return auther;
public void setAuther(String auther)
this.auther = auther;
public String getPubDate()
return pubDate;
public void setPubDate(String pubDate)
this.pubDate = pubDate;
public double getPrice()
return price;
public void setPrice(double price)
this.price = price;
public int getStore()
return store;
public void setStore(int store)
this.store = store;
public Book(int bookId, String bookName, String auther, String pubDate,
double price, int store)
super();
this.bookId = bookId;
this.bookName = bookName;
this.auther = auther;
this.pubDate = pubDate;
this.price = price;
this.store = store;
public Book()
// TODO Auto-generated constructor stub
</strong></span><span style="font-size:18px;font-weight: bold;">
</span>
package Library;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.util.ArrayList;
import java.util.List;
public class BookBiz
private List<Book> bookList =new ArrayList<Book>();
public BookBiz()
readFile();
if(bookList.size()==0)
Book book=new Book(10001,"鬼吹灯","天下霸唱","2006-1-1",34,3);
insertBook(book);
book=new Book(10002,"魔戒","莫尔斯","2006-3-1",334,300);
insertBook(book);
book=new Book(10003,"哈利波特","罗琳","2006-1-1",34,3);
insertBook(book);
book=new Book(10004,"时间机器人","威尔","2006-3-1",90,30);
insertBook(book);
book=new Book(10005,"宇宙奥秘","霍金","2006-1-1",89,79);
insertBook(book);
public void insertBook(Book book)
bookList.add(book);
saveFile();
public void deleteBook(int id)
for(int i=0;i<bookList.size();i++)
Book book =bookList.get(i);
if(book.getBookId()==1)
bookList.remove(i);
saveFile();
return;
public void updateBook(Book book)
for(int i=0;i<bookList.size();i++)
Book bk=bookList.get(i);
if(bk.getBookId()==book.getBookId())
bk.setAuther(book.getAuther());
bk.setBookId(book.getBookId());
bk.setPrice(book.getPrice());
bk.setStore(book.getStore());
bk.setPubDate(book.getPubDate());
saveFile();
return;
public List<Book> selectBooks()
return bookList;
public Book selectBook(int id )
Book book=new Book();
for (int i = 0; i < bookList.size(); i++)
book=bookList.get(i);
if(book.getBookId()==id)
return book;
return null;
public Book selectBook2(int id,int store )
Book book=new Book();
for (int i = 0; i < bookList.size(); i++)
book=bookList.get(i);
if(book.getBookId()==id)
int st=book.getStore()-store;
book.setStore(st);
return book;
return null;
public void readFile()
ObjectInputStream ois=null;
FileInputStream fis=null;
String name="E:\\\\android\\\\workspace\\\\MyOopBook\\\\src\\\\book.txt";
try fis=new FileInputStream(name);
ois=new ObjectInputStream(fis);
bookList=(List<Book>) ois.readObject();
catch (FileNotFoundException e)
// TODO Auto-generated catch block
e.printStackTrace();
catch (IOException e)
// TODO Auto-generated catch block
e.printStackTrace();
catch (ClassNotFoundException e)
// TODO Auto-generated catch block
e.printStackTrace();
finally
try
if(ois!=null)
ois.close();
catch (IOException e)
// TODO: handle exception
e.printStackTrace();
public void saveFile()
ObjectOutputStream oos=null;
FileOutputStream fos=null;
String name="E:\\\\Android\\\\workspace\\\\MyOopBook\\\\src\\\\book.txt";
try fos=new FileOutputStream(name);
oos=new ObjectOutputStream(fos);
oos.writeObject(bookList);
catch (FileNotFoundException e)
// TODO Auto-generated catch block
e.printStackTrace();
catch (IOException e)
// TODO Auto-generated catch block
e.printStackTrace();
finally
try
if(oos!=null)
oos.close();
catch (IOException e)
// TODO: handle exception
e.printStackTrace();
package Library;
import java.util.List;
import java.util.Scanner;
public class BookTest
/**
* @param args
*/
private BookBiz bookbiz=new BookBiz();
public static void main(String[] args)
// TODO Auto-generated method stub
Scanner input=new Scanner(System.in);
System.out.println("欢迎使用图书书店");
System.out.println("请输入用户名");
String userName=input.next();
System.out.println("请输入密码");
String userPwd=input.next();
BookTest bt=new BookTest();
if(userName.equals("admin")&&userPwd.equals("123"))
System.out.println("登陆成功");
bt.showMainView();
bt.adminOption();
else if(userName.equals("user")&&userPwd.equals("123"))
System.out.println("登陆成功");
bt.showuser();
bt.adminOptionuser();
else
System.out.println("账号密码有错误");
public void showMainView()
System.out.println("============欢迎登陆库存管理系统===========");
System.out.println("书号\\t\\t书名\\t\\t作者\\t\\t日期\\t\\t价格\\t\\t数量\\t\\t");
List<Book> list=bookbiz.selectBooks();
for (Book book : list)
System.out.print(book.getBookId()+"\\t\\t");
System.out.print(book.getBookName()+"\\t\\t");
System.out.print(book.getAuther()+"\\t\\t");
System.out.print(book.getPubDate()+"\\t");
System.out.print(book.getPrice()+"\\t\\t");
System.out.print(book.getStore()+"\\n");
System.out.println("请选择进行的操作:1.图书入库2.图书出库3.查询全部图书4.新增图书5.退出");
public void showuser()
System.out.println("************欢迎光临图书系统*************");
System.out.println("书号\\t\\t书名\\t\\t作者\\t\\t日期\\t\\t价格\\t\\t数量\\t\\t");
List<Book> list=bookbiz.selectBooks();
for (Book book : list)
System.out.print(book.getBookId()+"\\t\\t");
System.out.print(book.getBookName()+"\\t\\t");
System.out.print(book.getAuther()+"\\t\\t");
System.out.print(book.getPubDate()+"\\t");
System.out.print(book.getPrice()+"\\t\\t");
System.out.print(book.getStore()+"\\n");
System.out.println("请选择进行的操作:1.查询全部图书2结账3.退出");
public void adminOptionuser()
Scanner input=new Scanner(System.in);
int which=input.nextInt();
switch(which)
case 1: showuser();
adminOptionuser(); //1.查询全部图书
break;
case 2: jiezhang();
adminOptionuser(); //2结账
break;
default:
System.out.println("谢谢使用");//3退出
break;
public void adminOption()
Scanner input=new Scanner(System.in);
int which=input.nextInt();
switch(which)
case 1: tushuruku(); //1.图书入库
break;
case 2: tushuchuku(); //2.图书出库
break;
case 3: //3查询全部图书
showMainView();
adminOption();
break;
case 4: insertbook(); //4.新增图书
break;
default:
System.out.println("谢谢使用");//5退出
break;
public void tushuruku()
System.out.println("请输入图书信息");
System.out.println("请输入图书ID:");
Scanner input=new Scanner(System.in);
int id=input.nextInt();
Book book=bookbiz.selectBook(id);
System.out.println("请输入入库库存数量:");
int store=input.nextInt();
store+=book.getStore();
book.setStore(store);
bookbiz.saveFile();
// bookbiz.readFile(); 一开始用第一次用读
showMainView();
adminOption();
public void tushuchuku()
System.out.println("请输入图书信息");
System.out.println("请输入图书ID:");
Scanner input=new Scanner(System.in);
int id=input.nextInt();
System.out.println("请输入出库库存数量:");
int store=input.nextInt();
bookbiz.selectBook2(id,store);
bookbiz.saveFile();
// bookbiz.readFile(); 一开始用第一次用读
showMainView();
adminOption();
private void insertbook()
// TODO Auto-generated method stub
Scanner a=new Scanner(System.in);
System.out.println("请输入增加图书信息");
System.out.println("请输入图书ID");
int m=a.nextInt();
System.out.println("请输入书名");
String e=a.next();
System.out.println("请输入作者");
String t=a.next();
System.out.println("请输入日期");
String y=a.next();
System.out.println("请输入价格");
double p=a.nextInt();
System.out.println("请输入库存");
int u=a.nextInt();
Book c=new Book(m,e,t,y,p,u);
bookbiz.insertBook(c);
showMainView();
adminOption();
public void jiezhang()
System.out.println("请输入欲购买的图书信息:");
System.out.println("请输入图书Id:");
Scanner input=new Scanner(System.in);
int id=input.nextInt();
System.out.println("请输入购买数量");
int store=input.nextInt();
bookbiz.selectBook2(id,store);
bookbiz.saveFile();
Book book=bookbiz.selectBook(id);
System.out.println(book.getBookName()+":"+book.getPrice());
System.out.println("数量:"+store);
System.out.println("小计:"+(store*book.getPrice()));
showuser();
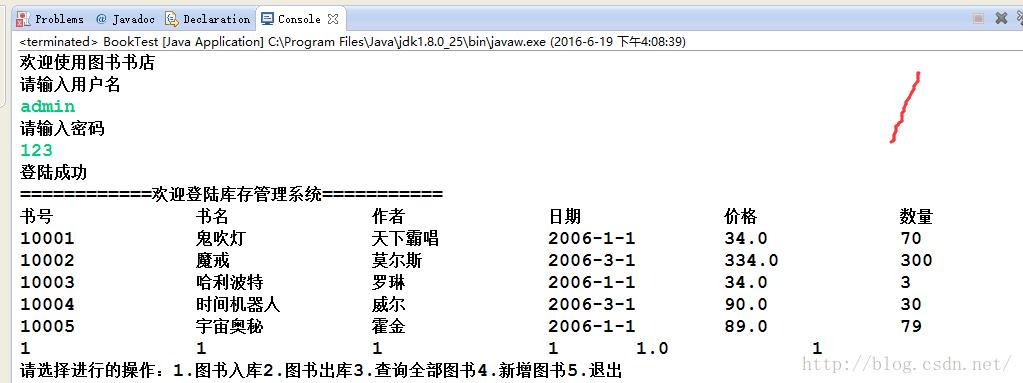
用户购买系统
图书馆用户购买系统优化
package biz;
import java.io.Serializable;
public class Book implements Serializable
private int bookId;
private String bookName;
private String author;
private String pubDate;
private double price;
private int store;
public Book()
public Book(int bookId, String bookName, String author, String pubDate,
double price, int store)
super();
this.bookId = bookId;
this.bookName = bookName;
this.author = author;
this.pubDate = pubDate;
this.price = price;
this.store = store;
public int getBookId()
return bookId;
public void setBookId(int bookId)
this.bookId = bookId;
public String getBookName()
return bookName;
public void setBookName(String bookName)
this.bookName = bookName;
public String getAuthor()
return author;
public void setAuthor(String author)
this.author = author;
public String getPubDate()
return pubDate;
public void setPubDate(String pubDate)
this.pubDate = pubDate;
public double getPrice()
return price;
public void setPrice(double price)
this.price = price;
public int getStore()
return store;
public void setStore(int store)
this.store = store;
package biz;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.util.ArrayList;
import java.util.List;
public class BookBiz
private List<Book> bookList = new ArrayList<Book>();
public BookBiz()
readFile();
if(bookList.size()==0)
Book book = new Book(10001,"鬼吹灯","天下霸唱","2006-01-01",87,100);
insertBook(book);
book = new Book(10002,"魔戒","莫尔斯","2006-01-01",22,55);
insertBook(book);
book = new Book(10003,"哈利波特","罗琳","2008-01-01",45,25);
insertBook(book);
book = new Book(10004,"时间机器","威尔","2009-01-01",66,64);
insertBook(book);
book = new Book(10005,"宇宙奥秘","霍金","2010-01-01",76,67);
insertBook(book);
public void insertBook(Book book)
bookList.add(book);
saveFile();
public void deleteBook(int id)
for(int i= 0 ; i<bookList.size(); i++)
Book book = bookList.get(i);
if(book.getBookId() == id)
bookList.remove(i);
saveFile();
return;
public void updateBook(Book book)
for(int i=0 ; i < bookList.size();i++)
Book bk = bookList.get(i);
if(bk.getBookId() == book.getBookId())
bk.setAuthor(book.getAuthor());
bk.setBookName(book.getBookName());
bk.setPrice(book.getPrice());
bk.setStore(book.getStore());
bk.setPubDate(book.getPubDate());
saveFile();
return;
public List<Book> selectBooks()
return bookList;
public Book selectBook(int id)
Book book = new Book();
for(int i =0;i<bookList.size();i++)
book = bookList.get(i);
if(book.getBookId() == id)
return book;
return null;
public void readFile()
ObjectInputStream ois = null;
FileInputStream fis = null;
String name = "E:\\\\Android\\\\workspace\\\\MyOopBookTeacher\\\\src\\\\biz\\\\book.txt";
try
fis = new FileInputStream(name);
ois = new ObjectInputStream(fis);
bookList = (List<Book>) ois.readObject();
catch (FileNotFoundException e)
// TODO Auto-generated catch block
e.printStackTrace();
catch (IOException e)
// TODO Auto-generated catch block
e.printStackTrace();
catch (ClassNotFoundException e)
// TODO Auto-generated catch block
e.printStackTrace();
finally
try
if(ois!=null)
ois.close();
catch (IOException e)
// TODO Auto-generated catch block
e.printStackTrace();
public void saveFile()
ObjectOutputStream oos = null;
FileOutputStream fos = null;
String name = "E:\\\\Android\\\\workspace\\\\MyOopBookTeacher\\\\src\\\\biz\\\\book.txt";
try
fos = new FileOutputStream(name);
oos = new ObjectOutputStream(fos);
oos.writeObject(bookList);
System.out.println("数据保存成功");
catch (FileNotFoundException e)
// TODO Auto-generated catch block
e.printStackTrace();
catch (IOException e)
// TODO Auto-generated catch block
e.printStackTrace();
finally
try
if(oos!=null)
oos.close();
catch (IOException e)
// TODO Auto-generated catch block
e.printStackTrace();
package biz;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Scanner;
import java.util.Set;
public class BookTest
private BookBiz bookbiz = new BookBiz();
public static void main(String[] args)
Scanner input = new Scanner(System.in);
System.out.println("欢迎使用图书书店");
System.out.println("请输入用户名:");
String userName = input.next();
System.out.println("请输入密码:");
String userPwd = input.next();
BookTest bt = new BookTest();
if(userName.equals("admin")&&userPwd.equals("123"))
System.out.println("登陆成功");
bt.showMainView("admin");
bt.adminOption();
else if(userName.equals("user")&&userPwd.equals("user"))
System.out.println("登陆成功");
bt.showMainView("user");
bt.userOption();
else
System.out.println("用户或密码");
public void showMainView(String arg)
System.out.println("==================欢迎登录库存管理系统===================");
System.out.println("书号\\t\\t书名\\t\\t作者\\t\\t发布日期\\t\\t价格\\t\\t库存");
List<Book> list = bookbiz.selectBooks();
for (Book book : list)
System.out.print(book.getBookId()+"\\t\\t");
System.out.print(book.getBookName()+"\\t\\t");
System.out.print(book.getAuthor()+"\\t\\t");
System.out.print(book.getPubDate()+"\\t\\t");
System.out.print(book.getPrice()+"\\t\\t");
System.out.print(book.getStore()+"\\n");
if(arg.equals("admin"))
System.out.println("请选择进行的操作:1.图书入库 2.图书出库 " +
"3.查询全部图书 4.新增图书 5.退出");
else
System.out.println("请选择进行的操作:1.查询 2.结账 3.退出");
public void adminOption()
Scanner input = new Scanner(System.in);
int which = input.nextInt();
switch(which)
case 1: //入库
inStore();
break;
case 2: //出库
outStore();
break;
case 3: //查询全部
showMainView("admin");
break;
case 4: //新增图书
addBook();
break;
default: //退出
bookbiz.saveFile();
break;
public void inStore()
Scanner input = new Scanner(System.in);
System.out.println("请输入图书信息");
System.out.println("请输入图书ID:");
int bookId = input.nextInt();
Book book = bookbiz.selectBook(bookId);
System.out.println("请输入入库的数量");
int qty = input.nextInt();
qty += book.getStore();
book.setStore(qty);
bookbiz.saveFile();
showMainView("admin");
adminOption();
public void outStore()
Scanner input = new Scanner(System.in);
System.out.println("请输入图书信息");
System.out.println("请输入图书ID:");
int bookId = input.nextInt();
Book book = bookbiz.selectBook(bookId);
System.out.println("请输入出库的数量");
int qty = input.nextInt();
book.setStore(book.getStore()-qty);
bookbiz.saveFile();
showMainView("admin");
adminOption();
public void addBook()
Scanner input = new Scanner(System.in);
System.out.println("请输入图书信息");
System.out.println("请输入图书ID:");
int bookId = input.nextInt();
System.out.println("请输入书名");
String bookName = input.next();
System.out.println("请输入作者");
String author = input.next();
System.out.println("请输入出版时间");
String pubDate = input.next();
System.out.println("请输入价格");
double price = input.nextDouble();
System.out.println("请输入数量");
int qty = input.nextInt();
Book book = new Book();
book.setBookId(bookId);
book.setBookName(bookName);
book.setAuthor(author);
book.setPubDate(pubDate);
book.setPrice(price);
book.setStore(qty);
bookbiz.insertBook(book);
showMainView("admin");
adminOption();
public void userOption()
Scanner input = new Scanner(System.in);
int which = input.nextInt();
switch(which)
case 1:
break;
case 2:
pay();
break;
default:
break;
public void pay()
Scanner input = new Scanner(System.in);
boolean flag = true;
double amt = 0;
Map map = new HashMap();
do
System.out.println("请输入要购买的图书编号:");
int bookId = input.nextInt();
Book book = bookbiz.selectBook(bookId);
System.out.println("请输入购买数量:");
int count = input.nextInt();
if(book.getStore()<count)
System.out.println("购买数量过大,请重新输入");
continue;
if(map.containsKey(book))
int temp = Integer.parseInt(map.get(book).toString());
map.put(book, count+temp);
else
map.put(book, count);
System.out.println(book.getBookName()+":"+book.getPrice());
System.out.println(count);
System.out.println("小计:"+(count*book.getPrice()));
amt += count*book.getPrice();
System.out.println("是否继续购买(y/n)");
String str = input.next();
if(!str.equals("y"))
flag = false;
while(flag);
System.out.println("总计:"+amt);
System.out.println("支付:");
double pay = input.nextDouble();
Set set = map.keySet();
Iterator it = set.iterator();
while(it.hasNext())
System.out.println("AAAAA");
Book book = (Book) it.next();
book = bookbiz.selectBook(book.getBookId());
int count = Integer.parseInt(map.get(book).toString());
book.setStore(book.getStore()-count);
bookbiz.saveFile();
System.out.println("找零:"+(pay-amt));
showMainView("user");
userOption();
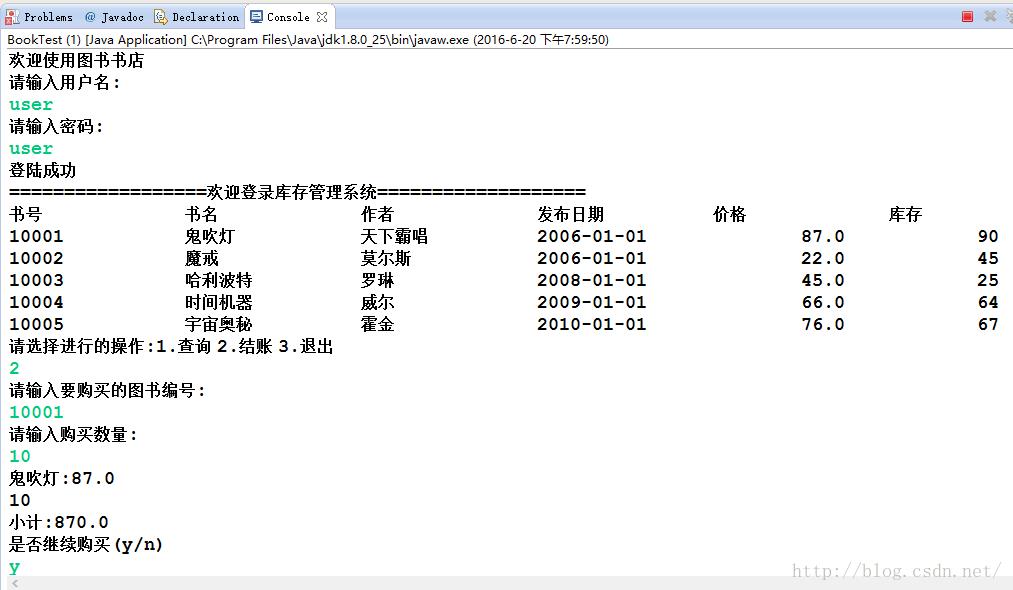
作者:冲天之峰 20160619
以上是关于JAVA图书馆库存管理系统程序代码(管理系统+用户购买结账系统)的主要内容,如果未能解决你的问题,请参考以下文章