markdown 终极版
Posted
tags:
篇首语:本文由小常识网(cha138.com)小编为大家整理,主要介绍了markdown 终极版相关的知识,希望对你有一定的参考价值。
Redux is predictable state container for JavaScript apps.
# Redux principles
## All your data is contained in a single object
The all state of the app is a single Javascript object
## Redux state is read only
Redux state is *read only*, it means that the only way to change the state is to
dispatch an action. This action will return a new state with your changes and replace
the current state
## Some functions in Redux have to be 'pure'
*pure* functions, are functions that, given the same parameters, they always
return the same result and never modify the parameters.
## The state mutation needs to be described as a pure function
State mutation needs a reducer: a function that calculates and return the next
state tree based on the previous state tree and the action being dispatched.
## Combine reducers
You can combine several reducers with the method `combineReducers`
# Working
## Data flow
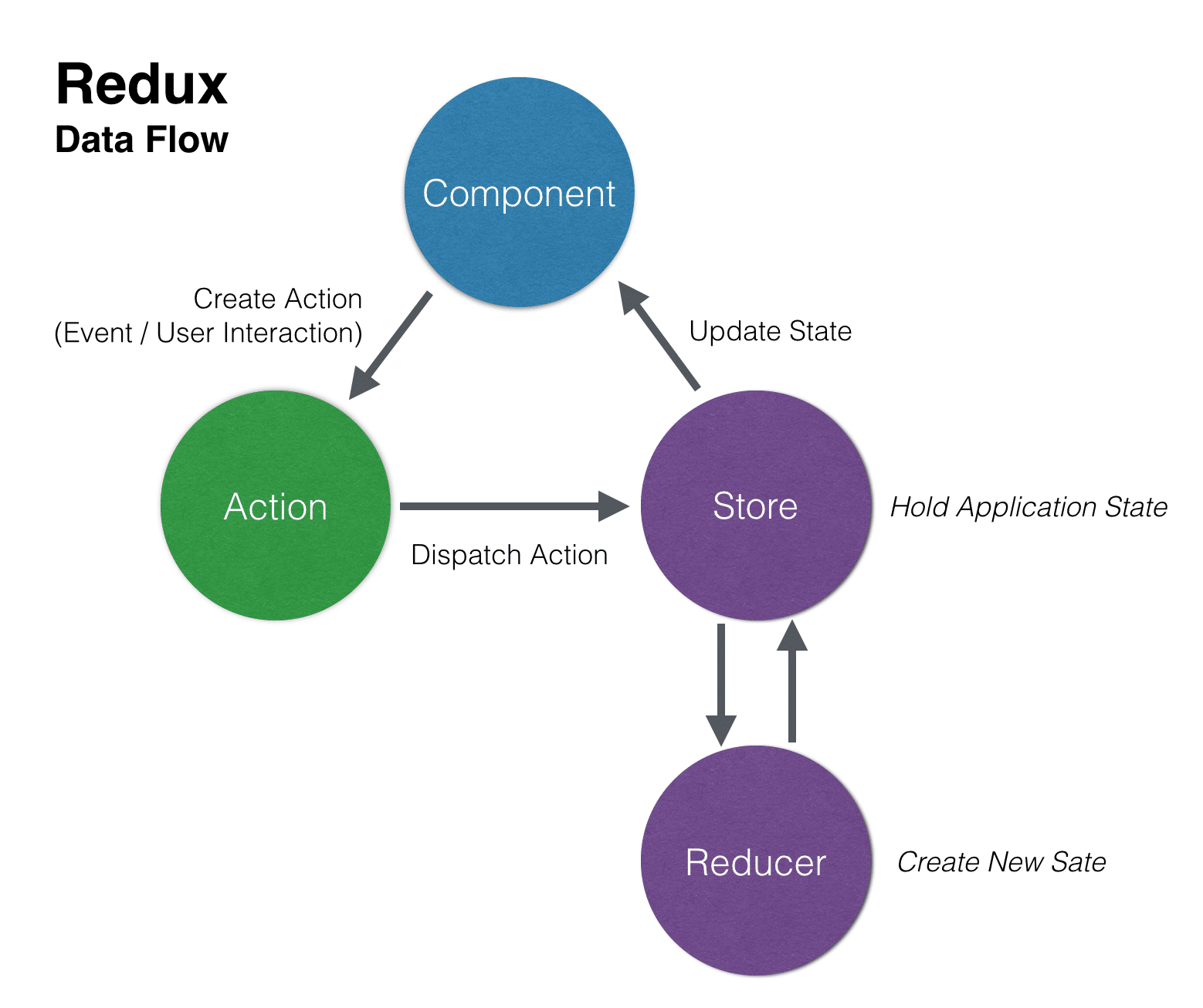
### Reducer
A reducer is a pure function that, given a state and an action, returns a new state.
````javascript
// REDUCER DECLARATION
const todo = (state, action) => {
switch (action.type) {
case 'ADD_TODO':
return {
id: action.id,
text: action.text,
completed: false
};
case 'TOGGLE_TODO':
if (state.id !== action.id) {
return state;
}
return {
...state,
completed: !state.completed
};
default:
return state;
}
};
// REDUCER CALL
store.dispatch({
type: 'ADD_TODO',
text: this.input.value,
id: nextTodoId++
});
````
Note that you can create multiple reducers that calls to each others and combine them in a single reducer using `combineReducers()`
````javascript
const myCombinedReducer = combineReducers({reducer1, reducer2});
````
### Store
A redux store is a javascript object that contains the entire state of the app.
You put in the store every data that you need to share between components.
````javascript
// Store creation
const myStore = createStore(myReducer);
```
### Component
A component is an UI element that contains reference to the redux state.
It can also contains event or user interactions that will dispatch an action to the redux store.
````javascript
// DECLARATION
class MyComponent extends Component {
render() {
return (
<div>
<button onClick={() => {
store.dispatch({ // Dispatch an action to the redux store
type: 'ADD_TODO',
text: this.input.value,
});
}}>
Add Todo
</button>
<ul>
{this.props.todos.map(todo => // Redux state references from the props
<li key={todo.id}>
{todo.text}
</li>
)}
</ul>
</div>
);
}
}
// USAGE
const render = () => {
ReactDOM.render(
<MyComponent todos={store.getState().todos}/>, //Redux state reference
document.getElementById('root')
);
};
````
To listen state changes, you need to suscribe to the store using `store.subscribe(mySubscriber)`
````javascript
store.subscribe(render); // subscribe
````
# Basics
## Actions
Actions are payloads of information that send data from your application to your store.You send them to the store using store.dispatch().
#### Exemple:
````javascript
{
type: SET_VISIBILITY_FILTER,
filter: SHOW_COMPLETED
}
````
## Reducers
Reducers specify how the application's state changes in response to actions sent to the store.
1. Add property to store: `myProperty: ""`
2. Add the action to export in the _index.js_ of actions folder
```javascript
export const myFunction = (myProperty) => {
return {type: "MY_FUNCTION", myProperty}
};
```
3.Add the action case into the reducer (often _AppContent.js_)
```javascript
switch (action.type) {
case "XXX":
return {...};
case "MY_FUNCTION":
return {
...state,
username: action.myProperty //update property in global state
};
}
```
4. In the scene, delete the internal state if needed
```javascript
delete constructor(props) {
super(props);
this.state = { username: '', password: '' };
}
```
5. Map redux state to props in the scene
```javascript
function mapStateToProps(state) {
return {
...
myProp: state.appContent.myProp
...
}
}
```
6. Import state properties and actions in the scene
```javascript
const {myProperty, myAction} : this.props;
```
7. Import the action in the scene
```javascript
import { ..., updateCredentials } from '@actions';
```
8. Export the functions in the scene
```javascript
export default connect(mapStateToProps, {..., updateCredentials});
```
以上是关于markdown 终极版的主要内容,如果未能解决你的问题,请参考以下文章
markdown 加速Android Studio的终极指南
markdown Mocha,Chai和Sinon的终极单元测试作弊表